










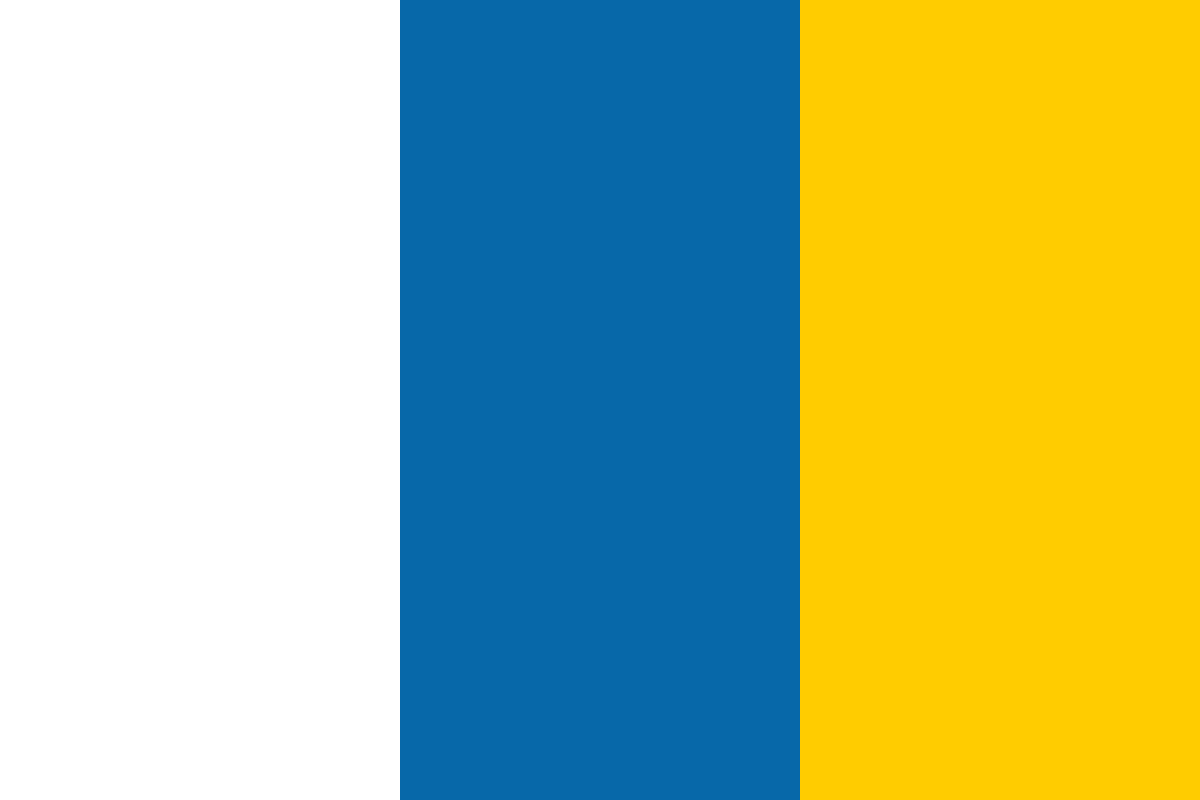

































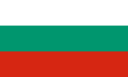








Imports: First we will import the required Pygame modules. If Pygame is installed properly, we should get no errors:
import pygame, sys from pygame.locals import *
Initialization: We will initialize Pygame by creating a display of 400 by 300 pixels and setting the window title to Hello world:
pygame.init() screen = pygame.display.set_mode((400, 300)) pygame.display.set_caption('Hello World!')
The main game loop: Games usually have a game loop, which runs forever until, for instance, a quit event occurs. In this example, we will only set a label with the text Hello world at coordinates (100, 100). The text has a font size of 19, red color, and falls back to the default font:
while True: sys_font = pygame.font.SysFont("None", 19) rendered = sys_font.render('Hello World', 0, (255, 100, 100)) screen.blit(rendered, (100, 100)) for event in pygame.event.get(): if event.type == QUIT: pygame.quit() sys.exit() pygame.display.update()
We get the following screenshot as the end result:
The following is the complete code for the Hello World example:
import pygame, sys from pygame.locals import * pygame.init() screen = pygame.display.set_mode((400, 300)) pygame.display.set_caption('Hello World!') while True: sysFont = pygame.font.SysFont("None", 19) rendered = sysFont.render('Hello World', 0, (255, 100, 100)) screen.blit(rendered, (100, 100)) for event in pygame.event.get(): if event.type == QUIT: pygame.quit() sys.exit() pygame.display.update()
It might not seem like much, but we learned a lot in this recipe. The functions that passed the review are summarized in the following table:
Function |
Description |
pygame.init() |
This function performs the initialization and needs to be called before any other Pygame functions are called. |
pygame.display.set_mode((400, 300)) |
This function creates a so-called Surface object to draw on. We give this function a tuple representing the width and height of the surface. |
pygame.display.set_caption('Hello World!') |
This function sets the window title to a specified string value. |
pygame.font.SysFont("None", 19) |
This function creates a system font from a comma-separated list of fonts (in this case none) and a font size parameter. |
sysFont.render('Hello World', 0, (255, 100, 100)) |
This function draws text on a surface. The second parameter indicates whether anti-aliasing is used. The last parameter is a tuple representing the RGB values of a color. |
screen.blit(rendered, (100, 100)) |
This function draws on a surface. |
pygame.event.get() |
This function gets a list of Event objects. Events represent some special occurrence in the system, such as a user quitting the game. |
pygame.quit() |
This function cleans up resources used by Pygame. Call this function before exiting the game. |
pygame.display.update() |
This function refreshes the surface. |
Before we start creating cool games, we need an introduction to the drawing functionality of Pygame. As we noticed in the previous section, in Pygame we draw on the Surface objects. There is a myriad of drawing options—different colors, rectangles, polygons, lines, circles, ellipses, animation, and different fonts.
The following steps will help you diverge into the different drawing options you can use with Pygame:
Imports: We will need the NumPy library to randomly generate RGB values for the colors, so we will add an extra import for that:
import numpy
Initializing colors: Generate four tuples containing three RGB values each with NumPy:
colors = numpy.random.randint(0, 255, size=(4, 3))
Then define the white color as a variable:
WHITE = (255, 255, 255)
Set the background color: We can make the whole screen white with the following code:
screen.fill(WHITE)
Drawing a circle: Draw a circle in the center with the window using the first color we generated:
pygame.draw.circle(screen, colors[0], (200, 200), 25, 0)
Drawing a line: To draw a line we need a start point and an end point. We will use the second random color and give the line a thickness of 3:
pygame.draw.line(screen, colors[1], (0, 0), (200, 200), 3)
Drawing a rectangle: When drawing a rectangle, we are required to specify a color, the coordinates of the upper-left corner of the rectangle, and its dimensions:
pygame.draw.rect(screen, colors[2], (200, 0, 100, 100))
Drawing an ellipse: You might be surprised to discover that drawing an ellipse requires similar parameters as for rectangles. The parameters actually describe an imaginary rectangle that can be drawn around the ellipse:
pygame.draw.ellipse(screen, colors[3], (100, 300, 100, 50), 2)
The resulting window with a circle, line, rectangle, and ellipse using random colors:
The code for the drawing demo is as follows:
import pygame, sys from pygame.locals import * import numpy pygame.init() screen = pygame.display.set_mode((400, 400)) pygame.display.set_caption('Drawing with Pygame') colors = numpy.random.randint(0, 255, size=(4, 3)) WHITE = (255, 255, 255) #Make screen white screen.fill(WHITE) #Circle in the center of the window pygame.draw.circle(screen, colors[0], (200, 200), 25, 0) # Half diagonal from the upper-left corner to the center pygame.draw.line(screen, colors[1], (0, 0), (200, 200), 3) pygame.draw.rect(screen, colors[2], (200, 0, 100, 100)) pygame.draw.ellipse(screen, colors[3], (100, 300, 100, 50), 2) while True: for event in pygame.event.get(): if event.type == QUIT: pygame.quit() sys.exit() pygame.display.update()
Here we saw how to create a basic game to get us started. The game demonstrated fonts and screen management in the time-honored tradition of Hello world examples.
The next section of drawing with Pygame taught us how to draw basic shapes such as rectangles, ovals, circles, lines, and others. We also learned important information about colors and color management.
Further resources on this subject: