










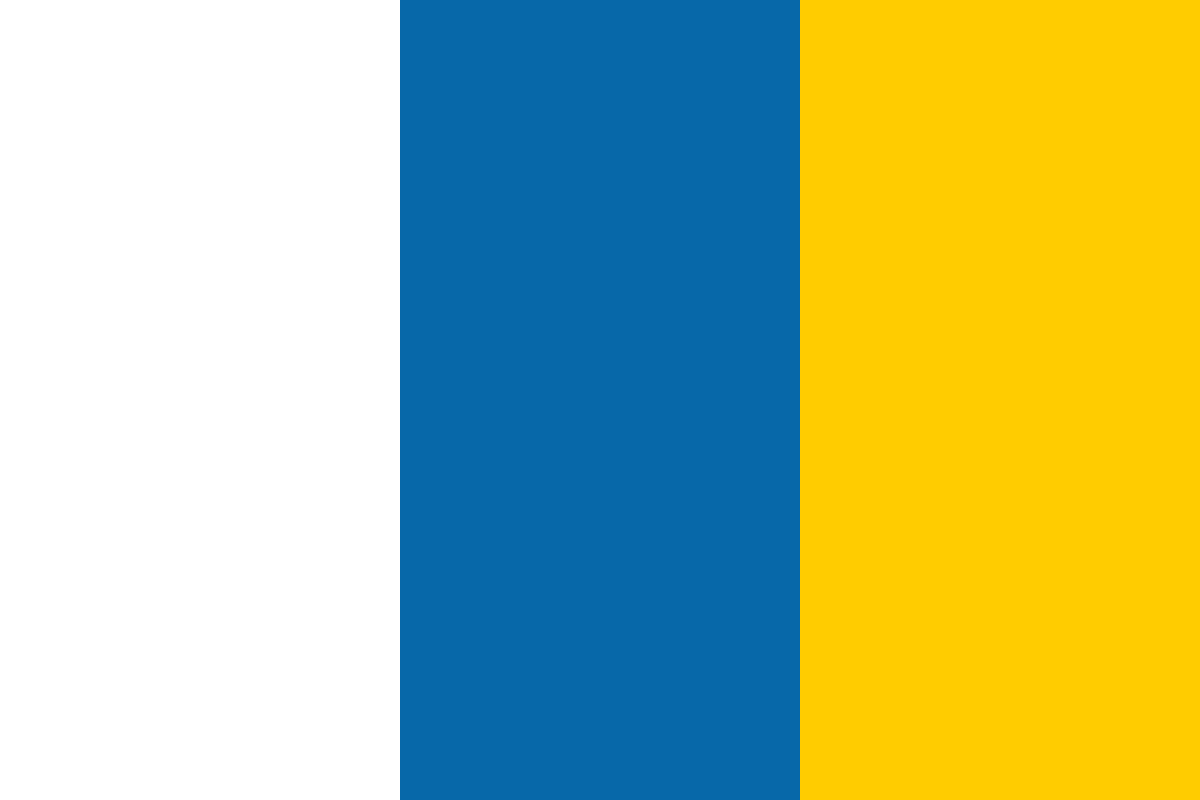

































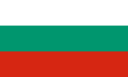








The choice of validation method depends on the additional functionality required in the validation application. SAXParser is recommended if SAX parsing event notification is required in addition to validation with a schema. DOMParser is recommended if the DOM tree structure of an XML document is required for random access and modification of the XML document.
In this section we shall validate the example XML document catalog.xml with XML schema document catalog.xsd, with the SAXParser class. Import the oracle.xml.parser.schema and oracle.xml.parser.v2 packages.
Create a SAXParser object and set the validation mode of the SAXParser object to SCHEMA_VALIDATION, as shown in the following listing:
SAXParser saxParser=new SAXParser();
saxParser.setValidationMode(XMLParser.SCHEMA_VALIDATION);
The different validation modes that may be set on a SAXParser are discussed in the following table; but we only need the SCHEMA-based validation modes:
Validation Mode |
Description |
NONVALIDATING |
The parser does not validate the XML document. |
PARTIAL_VALIDATION |
The parser validates the complete or a partial XML document with a DTD or an XML schema if specified. |
DTD_VALIDATION |
The parser validates the XML document with a DTD if any. |
SCHEMA_VALIDATION |
The parser validates the XML document with an XML schema if any specified. |
SCHEMA_LAX_VALIDATION |
Validates the complete or partial XML document with an XML schema if the parser is able to locate a schema. The parser does not raise an error if a schema is not found. |
SCHEMA_STRICT_VALIDATION |
Validates the complete XML document with an XML schema if the parser is able to find a schema. If the parser is not able find a schema or if the XML document does not conform to the schema, an error is raised. |
Next, create an XMLSchema object from the schema document with which an XML document is to be validated. An XMLSchema object represents the DOM structure of an XML schema document and is created with an XSDBuilder class object. Create an XSDBuilder object and invoke the build(InputSource) method of the XSDBuilder object to obtain an XMLSchema object. The InputSource object is created with an InputStream object created from the example XML schema document, catalog.xsd. As discussed before, we have used an InputSource object because most SAX implementations are InputSource based. The procedure to obtain an XMLSchema object is shown in the following listing:
XSDBuilder builder = new XSDBuilder();
InputStream inputStream=new FileInputStream(new File("catalog.xsd"));
InputSource inputSource=new InputSource(inputStream);
XMLSchema schema = builder.build(inputSource);
Set the XMLSchema object on the SAXParser object with setXMLSchema(XMLSchema) method:
saxParser.setXMLSchema(schema);
As in the previous section, define an error handling class, CustomErrorHandler that extends DefaultHandler class. Create an object of type CustomErrorHandler, and register the ErrorHandler object with the SAXParser as shown here:
CustomErrorHandler errorHandler = new CustomErrorHandler();
saxParser.setErrorHandler(errorHandler);
The SAXParser class extends the XMLParser class, which provides the overloaded parse methods discussed in the following table to parse an XML document:
Method |
Description |
parse(InputSource in) |
Parses an XML document from an org.xml.sax.InputSouce object. The InputSource-based parse method is the preferred method because SAX parsers convert the input to InputSource no matter what the input type is. |
parse(java.io.InputStream in) |
Parses an XML document from an InputStream. |
parse(java.io.Reader r) |
Parses an XML document from a Reader. |
parse(java.lang.String in) |
Parses an XML document from a String URL for the XML document. |
parse(java.net.URL url) |
Parses an XML document from the specified URL object for the XML document. |
Create an InputSource object from the XML document to be validated, and parse the XML document with the parse(InputSource) object:
InputStream inputStream=new FileInputStream(new
File("catalog.xml"));
InputSource inputSource=new InputSource(inputStream);
saxParser.parse(inputSource);
The validation application SAXValidator.java is listed in the following listing with additional explanations:
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import oracle.xml.parser.schema.*;
import oracle.xml.parser.v2.*;
import java.io.IOException;
import java.io.InputStream;
import org.xml.sax.SAXException;
import org.xml.sax.SAXParseException;
import org.xml.sax.helpers.DefaultHandler;
import org.xml.sax.InputSource;
public class SAXValidator {
public void validateXMLDocument(InputSource input) {
try {
SAXParser saxParser = new SAXParser();
saxParser.setValidationMode(XMLParser.SCHEMA_VALIDATION);
XMLSchema schema=getXMLSchema();
saxParser.setXMLSchema(schema);
CustomErrorHandler errorHandler = new CustomErrorHandler();
saxParser.setErrorHandler(errorHandler);
saxParser.parse(input);
if (errorHandler.hasValidationError == true) {
System.err.println("XML Document has
Validation Error:" + errorHandler.saxParseException.getMessage());
} else {
System.out.println("XML Document validates
with XML schema");
}
} catch (IOException e) {
System.err.println("IOException " + e.getMessage());
} catch (SAXException e) {
System.err.println("SAXException " + e.getMessage());
}
}
try {
XSDBuilder builder = new XSDBuilder();
InputStream inputStream =
new FileInputStream(new File("catalog.xsd"));
InputSource inputSource = new
InputSource(inputStream);
XMLSchema schema = builder.build(inputSource);
return schema;
} catch (XSDException e) {
System.err.println("XSDException " + e.getMessage());
} catch (FileNotFoundException e) {
System.err.println("FileNotFoundException " +
e.getMessage());
}
return null;
}
public static void main(String[] argv) {
try {
InputStream inputStream =
new FileInputStream(new File("catalog.xml"));
InputSource inputSource=new InputSource(inputStream);
SAXValidator validator = new SAXValidator();
validator.validateXMLDocument(inputSource);
} catch (FileNotFoundException e) {
System.err.println("FileNotFoundException " +
e.getMessage());
}
}
private class CustomErrorHandler extends DefaultHandler {
protected boolean hasValidationError = false;
protected SAXParseException saxParseException = null;
public void error(SAXParseException exception)
{
hasValidationError = true;
saxParseException = exception;
}
public void fatalError(SAXParseException exception)
{
hasValidationError = true;
saxParseException = exception;
}
public void warning(SAXParseException exception)
{
}
}
}
Copy the SAXValidator.java application to SAXValidator.java in the SchemaValidation project. To demonstrate error handling, add a title element to the journal element.
To run the SAXValidator.java application, right-click on SAXValidator.java in Application Navigator, and select Run. A validation error gets outputted. The validation error indicates that the title element is not expected.