










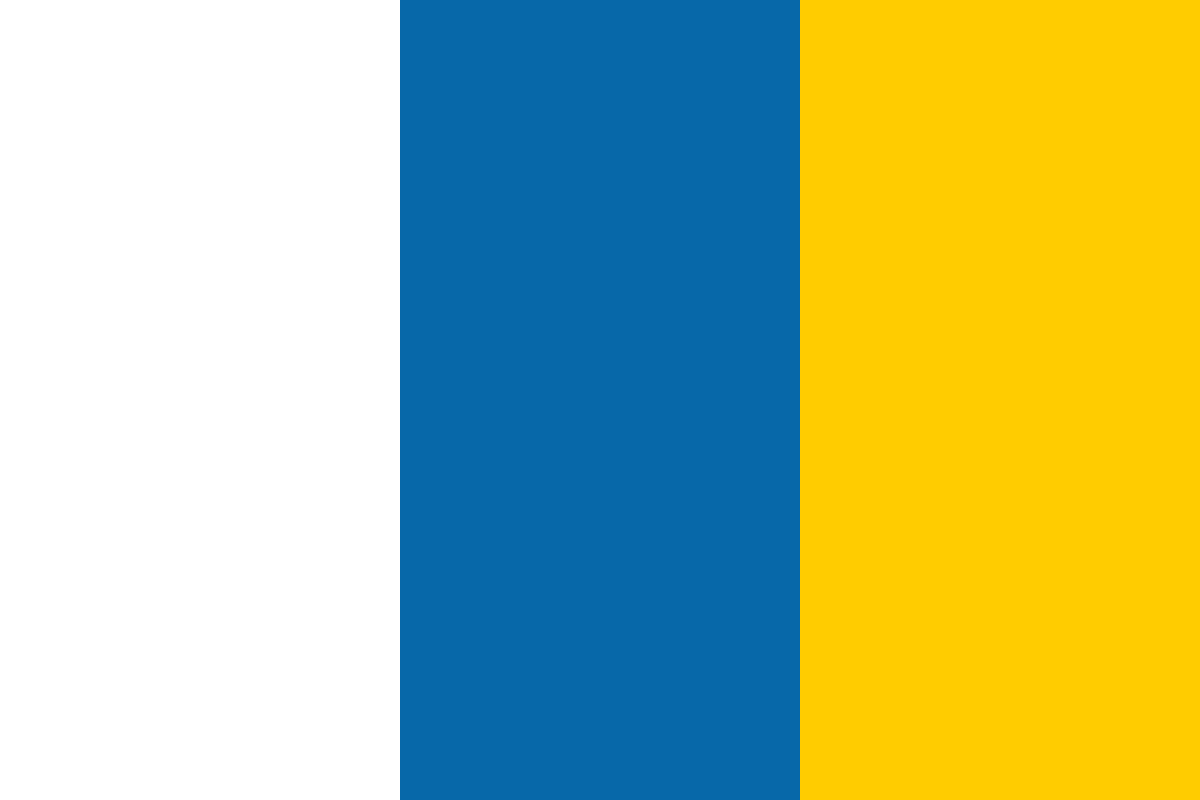

































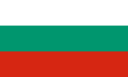








(For more resources on Oracle, see here.)
An EJB 3.0 entity bean is required to have a persistence.xml configuration file, which defines the database persistence properties. A persistence.xml file gets added to the META-INF folder when a JPA project is defined. Copy the following listing to the persistence.xml file in Eclipse:
<?xml version="1.0" encoding="UTF-8" ?>
<persistence
xsi_schemaLocation=
"http://java.sun.com/xml/ns/persistence
http://java.sun.com/xml/ns/persistence/persistence_1_0.xsd"
version="1.0">
<persistence-unit name="em">
<provider>org.eclipse.persistence.jpa.PersistenceProvider</provider>
<jta-data-source>jdbc/MySQLDS</jta-data-source>
<class>ejb3.Catalog</class>
<properties>
<property name="eclipselink.target-server" value="WebLogic_10"
/>
<property name="javax.persistence.jtaDataSource" value="jdbc/
MySQLDS" />
<property name="eclipselink.ddl-generation"
value="create-tables" />
<property name="eclipselink.target-database" value="MySQL" />
</properties>
</persistence-unit>
</persistence>
The persistence-unit is required to be named and may be given any name. We had configured a JDBC data source with JNDI jdbc/MySQLDS in WebLogic Server. Specify the JNDI name in the jta-data-source element. The properties element specifies vendor-specific properties. The eclipselink.ddl-generation property is set to create-tables, which implies that the required database tables will be created unless they are already created . The persistence.xml configuration file is shown in the Eclipse project in the following illustration:
(Move the mouse over the image to enlarge.)
For better performance, one of the best practices in developing EJBs is to access entity beans from session beans. Wrapping an entity bean with a session bean reduces the number of remote method calls as a session bean may invoke an entity bean locally. If a client accesses an entity bean directly, each method invocation is a remote method call and incurs an overhead of additional network resources. We shall use a stateless session bean, which consumes less resources than a stateful session bean, to invoke entity bean methods. In this section, we create a session bean in Eclipse. A stateless session bean class is just a Java class annotated with the @Stateless annotation. Therefore, we create Java classes for the session bean and session bean remote interface in Eclipse. To create a Java class, select File | New. In the New window, select Java | Class and click on Next>
In the New Java Class window, select the Source folder as EJB3JPA/src, EJB3JPA being the project name. Specify Class Name as CatalogTestBean and click on Finish.
Similarly, create a CatalogTestBeanRemote interface by selecting Java | Interface in the New window. The session bean class and the remote interface get added to the EJB3JPA project.
The stateless session bean class, CatalogTestBean implements the CatalogTestRemote interface. We shall use the EntityManager API to create, find, query, and remove entity instances. Inject an EntityManager using the @PersistenceContext annotation. Specify unitName as the same as the persistence-unit name in the persistence.xml configuration file:
@PersistenceContext(unitName = "em")
EntityManager em;
Next, create a test() method, which we shall invoke from a test client. In the test() method we shall create and persist entity instances, query an entity instance, and delete an entity instance, all using an EntityManager object, which we had injected earlier in the session bean class. Injecting an EntityManager implies that an instance of EntityManager is made available to the session bean. Create an instance of the Entity bean class:
Catalog catalog = new Catalog(new Integer(1), "Oracle Magazine",
"Oracle Publishing", "September-October 2009",
"Put Your Arrays in a Bind","Mark Williams");
Persist the entity instance to the database using the persist() method:
em.persist(catalog);
Similarly, persist two more entity instances. Next, create a query using the createQuery() method of the EntityManager object. The query string may be specified as a EJB-QL query. Unlike HQL, the SELECT clause is not optional in EJB-QL. Execute the query and return the query result as a List using the getResultList() method. As an example, select the catalog entry corresponding to author David Baum. The FROM clause of a query is directed towards the mapped entity bean class, not the underlying database.
List catalogEntry =em.createQuery("SELECT c from Catalog c where
c.author=:name").setParameter("name","David Baum").
getResultList();
Iterate over the result list to output the properties of the entity instance:
for (Iterator iter = catalogEntry.iterator(); iter.hasNext(); ) {
Catalog element = (Catalog)iter.next();
retValue =retValue + "<br/>" + element.getJournal() +
"<br/>" + element.getPublisher() +"<br/>" +
element.getDate() + "<br/>" + element.getTitle() +
"<br/>" + element.getAuthor() +"<br/>";
}
The variable retValue is a String that is returned by the test() method. Similarly, create and run a EJB-QL query to return all titles in the Catalog database:
List allTitles =em.createQuery("SELECT c from Catalog c").
getResultList();
An entity instance may be removed using the remove() method:
em.remove(catalog2);
The corresponding database row gets deleted from the Catalog table. Subsequently, create and run a query to list all the entity instances mapped to the database. The session bean class, CatalogTestBean, is listed next:
package ejb3;
import java.util.Iterator;
import java.util.List;
import javax.ejb.Stateless;
import javax.persistence.EntityManager;
import javax.persistence.PersistenceContext;
/**
* Session Bean implementation class CatalogTestBean
*/
@Stateless(mappedName = "EJB3-SessionEJB")
public class CatalogTestBean implements CatalogTestBeanRemote {
@PersistenceContext(unitName = "em")
EntityManager em;
/**
* Default constructor.
*/
public CatalogTestBean() {
// TODO Auto-generated constructor stub
}
public String test() {
Catalog catalog = new Catalog(new Integer(1), "Oracle Magazine",
"Oracle Publishing", "September-October 2009",
"Put Your Arrays in a Bind","Mark Williams");
em.persist(catalog);
Catalog catalog2 = new Catalog(new Integer(2), "Oracle Magazine",
"Oracle Publishing", "September-October 2009",
"Oracle Fusion Middleware 11g: The Foundation for Innovation",
"David Baum");
em.persist(catalog2);
Catalog catalog3 = new Catalog(new Integer(3), "Oracle Magazine",
"Oracle Publishing", "September-October 2009",
"Integrating Information","David Baum");
em.persist(catalog3);
String retValue = "<b>Catalog Entries: </b>";
List catalogEntry = em.createQuery("SELECT c from Catalog c
where c.author=:name").setParameter("name",
"David Baum").getResultList();
for (Iterator iter = catalogEntry.iterator(); iter.hasNext(); ) {
Catalog element = (Catalog)iter.next();
retValue = retValue + "<br/>" + element.getJournal() + "<br/>" +
element.getPublisher() + "<br/>" + element.getDate()
+ "<br/>" + element.getTitle() + "<br/>" +
element.getAuthor() + "<br/>";
}
retValue = retValue + "<b>All Titles: </b>";
List allTitles =
em.createQuery("SELECT c from Catalog c").getResultList();
for (Iterator iter = allTitles.iterator(); iter.hasNext(); ) {
Catalog element = (Catalog)iter.next();
retValue = retValue + "<br/>" + element.getTitle() + "<br/>";
}
em.remove(catalog2); );
retValue = retValue + "<b>All Entries after removing an entry:
</b>";
List allCatalogEntries =
em.createQuery("SELECT c from Catalog c").
getResultList();
for (Iterator iter = allCatalogEntries.iterator(); iter.hasNext();
) {
Catalog element = (Catalog)iter.next();
retValue = retValue + "<br/>" + element + "<br/>";
}
return retValue;
}
}
We also need to add a remote or a local interface for the session bean:
package ejb3;
import javax.ejb.Remote;
@Remote
public interface CatalogTestBeanRemote {
public String test();
}
The session bean class and the remote interface are shown next:
We shall be packaging the entity bean and the session bean in a EJB JAR file, and packaging the JAR file with a WAR file for the EJB 3.0 client into an EAR file as shown next:
EAR File
|
|
|-WAR File
|
|-EJB 3.0 Client
|-JAR File
|
|-EJB 3.0 Entity Bean
EJB 3.0 Session Bean
Next, we create an application.xml for the EAR file. Create a META-INF folder for the application.xml. Right-click on the EJB3JPA project in Project Explorer and select New>Folder. In the New Folder window, select the EJB3JPA folder and specify the new Folder name as META-INF. Click on Finish. Right-click on the META-INF folder and select New | Other. In the New window, select XML | XML and click on Next. In the New XML File window, select the META-INF folder and specify File name as application.xml. Click on Next. Click on Finish.
An application.xml file gets created. Copy the following listing to application.xml:
<?xml version = '1.0' encoding = 'windows-1252'?>
<application>
<display-name></display-name>
<module>
<ejb>ejb3.jar</ejb>
</module>
<module>
<web>
<web-uri>weblogic.war</web-uri>
<context-root>weblogic</context-root>
</web>
</module>
</application>
The application.xml in the Project Explorer is shown next: