










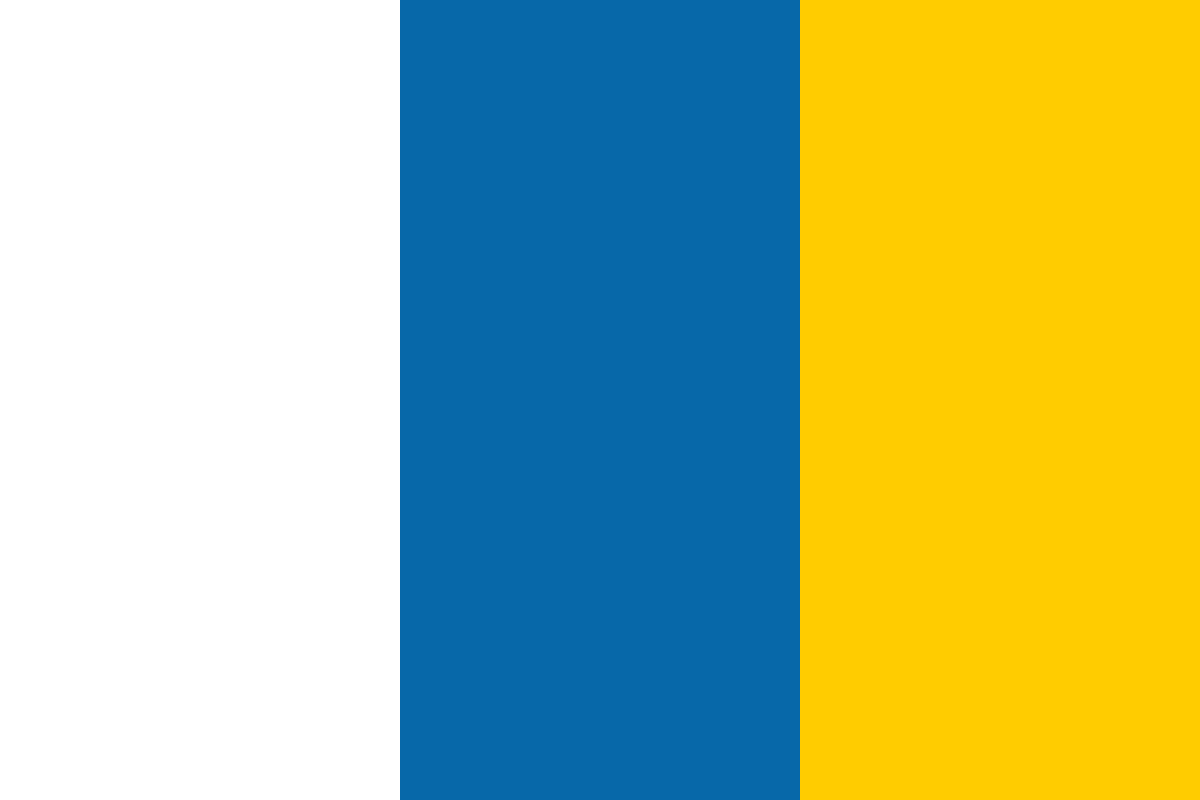

































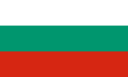








(For more resources related to this topic, see here.)
Here, we will see how to programmatically create an issue from a plugin. Prior to Version 4.1, JIRA used IssueManager to create an issue. From JIRA 4.1, there is this IssueService class that drives the issue operations. Since IssueService is recommended over IssueManager.
The main advantage of using IssueService over the IssueManager class is that it takes care of the validation and error handling. The following are the steps to create an issue using the IssueService class:
IssueService issueService = ComponentAccessor.getIssueService();
IssueInputParameters issueInputParameters = new
IssueInputParametersImpl();
issueInputParameters.setProjectId(10000L).
setIssueTypeId("5").setSummary("Test Summary").
setReporterId("admin").setAssigneeId("admin").
setDescription("Test Description").setStatusId("1").
setPriorityId("2").setFixVersionIds(10000L, 12121L);
CreateValidationResult createValidationResult = issueService.
validateCreate(user, issueInputParameters);
Here, the user is the one creating the issue. The validation is done based on the user permissions and the createValidationResult variable will have errors if the validation fails due to permission issues or due to invalid input parameters!
if (createValidationResult.isValid()) {
IssueResult createResult = issueService.create(user,
createValidationResult);
}
Here, we use the createValidationResult object to create the issue, as it already has the processed input parameters. If the result is not valid, handle the errors as shown in the following code:
if (!createValidationResult.isValid()) {
Collection<String> errorMessages = createValidationResult.
getErrorCollection().getErrorMessages();
for (String errorMessage : errorMessages) {
System.out.println(errorMessage);
}
Map<String, String> errors = createValidationResult.
getErrorCollection().getErrors();
Set<String> errorKeys = errors.keySet();
for (String errorKey : errorKeys) {
System.out.println(errors.get(errorKey));
}
}
Here, we just print the error to the console if the result is invalid. The errorMessages object will have all non-field-specific errors such as permission issue-related errors, and so on, but any field-specific errors, such as input validation errors, will appear in the errors map where the key will be the field name. We should handle both the error types as appropriate.
if (!createResult.isValid()) {
Collection<String> errorMessages = createResult.
getErrorCollection().getErrorMessages();
for (String errorMessage : errorMessages) {
System.out.println(errorMessage);
}
}
Here again, we just print the error to the console.
MutableIssue issue = createResult.getIssue();
By using IssueService, JIRA now validates the inputs we give it using the rules we have set up in JIRA via the user interfaces, such as the mandatory fields, permission checks, individual field validations, and so on. Behind the scenes, it still uses the IssueManager class.
As mentioned before, prior to JIRA 4.1, we needed to use the IssueManager class to create the issues. It can still be used in JIRA 4.1 and higher, but this is not recommended as it overrides all the validations.
But then again, what if you want to override those validations due to some reason? For example, to skip permission checks or field screen validations inside the plugin? In such cases, we might still need IssueManager.
Follow these steps to create an issue with the IssueManager class:
MutableIssue issue = ComponentAccessor.getIssueFactory().
getIssue();
issue.setProjectId(10000L);
issue.setIssueTypeId("5");
issue.setAssigneeId("admin");
Issue createdIssue = ComponentAccessor.getIssueManager().
createIssueObject(user, issue);
Here, we will demonstrate how to create a subtask from a JIRA plugin. It is very similar to the issue creation, but there are some notable differences.
Subtasks are useful for splitting up a parent issue into a number of tasks, which can be assigned and tracked separately. The progress on an issue is generally a sum of the progress on all its subtasks, although people use it for a lot of other purposes too.
There are two steps to creating a subtask:
Let's see these steps in more detail:
For this issue, we will use the validateSubTaskCreate method—which takes an extra parameter named parentId— instead of validateCreate:
CreateValidationResult createValidationResult = issueService.
validateSubTaskCreate(user, parent.getId(), issueInputParameters);
Here, parent is the issue object on which we are creating the subtask.
if (createValidationResult.isValid()) {
IssueResult createResult = issueService.create(user,
createValidationResult);
}
SubTaskManager subTaskManager = ComponentAccessor.
getSubTaskManager();
subTaskManager.createSubTaskIssueLink(parent, createResult.
getIssue(), user);
Here, let's look at editing an existing issue. Users can edit the issue to update one or more fields, and there are screen schemes or field configurations to define what a user can see while editing an issue. Moreover, there is the edit project permission option to limit editing to selected users, groups, or roles.
Programmatically editing an issue also takes these things into account.
Let's assume that we have an existing issue object. We will just modify the summary to a new summary. Following are the steps to do this:
IssueInputParameters issueInputParameters = new
IssueInputParametersImpl();
issueInputParameters.setSummary("Modified Summary");
If you do not want to retain the existing values and just want the summary on the issue to be updated, you can set the retainExistingValuesWhenParameterNotProvided flag as shown:
issueInputParameters.setRetainExistingValuesWhenParameterNotProvid
ed(false);
UpdateValidationResult updateValidationResult = issueService.
validateUpdate(user, issue.getId(), issueInputParameters);
Here, issue is the existing issue object.
if (updateValidationResult.isValid()) {
IssueResult updateResult = issueService.update(user,
updateValidationResult);
}
If it is not valid, handle the errors as we did when creating the issue.
MutableIssue updatedIssue = updateResult.getIssue();
Here, let us look at deleting an issue programmatically.
Let us assume that we have an existing issue object. For deletion as well, we will use the IssueService class. Following are the steps to do this:
DeleteValidationResult deleteValidationResult = issueService.
validateDelete(user, issue.getId());
Here, issue is the existing issue object that needs to be deleted.
if (deleteValidationResult.isValid()) {
ErrorCollection deleteErrors = issueService.delete(user,
deleteValidationResult);
}
if (deleteErrors.hasAnyErrors()){
Collection<String> errorMessages = deleteErrors.
getErrorMessages();
for (String errorMessage : errorMessages) {
System.out.println(errorMessage);
}
} else {
System.out.println("Deleted Succesfully!");
}
Here, we will look at adding new operations to an issue. The existing issue operations include Edit Issue, Clone Issue, and so on, but most of the time, people tend to look for similar operations with variations or entirely new operations that they can perform on an issue.
Prior to JIRA 4.1, the issue operations were added using the Issue Operations Plugin Module (http://confluence.atlassian.com/display/JIRADEV/Issue+Operations+Plugin+Module). But since JIRA 4.1, new issue operations are added using the Web Item Plugin Module (http://confluence.atlassian.com/display/JIRADEV/Web+Item+Plugin+Module).
A Web Item Plugin module is a generic module that is used to define links in various application menus. One such menu is the issue operations menu. Here, we will only concentrate on using the web-item module to create issue operations.
Create a skeleton plugin using Atlassian Plugin SDK.
Creating a web item is pretty easy! All we need to do is to place it in the appropriate section. There are already defined web sections in JIRA, and we can add more sections using the Web Section module if needed.
Let us create a new operation that lets us administer the project of an issue when we are on the View Issue page. All we need here is to add an operation that takes us to the Administer Project page. Following are the steps to create the new operation:
For issue operations, JIRA already has multiple web sections defined. We can add our new operation on any one of these sections. The following is a diagram from the Atlassian documentation detailing with each of the available web sections for the issue operations:
<web-item key="manage-project" name="Manage Project"
section="operations-operations" weight="100">
<label>Manage Project</label>
<tooltip>Manages the Project in which the issue belongs </
tooltip>
<link linkId="manage-project-link">/plugins/servlet/projectconfig/${
issue.project.key}</link>
</web-item>
As you can see, this has a unique key and a human-readable name. The section here is operations-operations. The weight attribute is used to reorder the operations as we saw earlier, and here we use weight as 100 to put it at the bottom of the list.
label is the name of the operation that will appear to the user. We can add a tooltip as well, which can have a friendly description of the operation. The next part, that is, the link attribute, is the most important one, as it links us to the operation that we want to perform. Essentially it is just a link, and hence you can use it to redirect to anywhere; the Atlassian site, for example.
In our example, we need to take the user to the administer project area. Luckily, in this case, we know the servlet to be invoked, as it is an existing servlet in JIRA. All we need to do is to invoke the project-config servlet by passing the project key as well. The issue object is available on the view issue page as $issue, and hence we can retrieve the project ID on the link as ${issue.project.key}.
In cases where we need to do new things, we will have to create an action or servlet by ourselves and point the link to that action/servlet.
At runtime, you will see a new operation on the View Issue page on the More Actions drop-down menu, as shown in the next screenshot:
After clicking on the link, the Administer Project screen will appear as expected. As you might have noticed, the URL is populated with the correct key from the expression ${issue.project.key}.
Also, just change the section or weight and see how the operation appears at various places on the screen!
Prior to JIRA 4.1, the Issue Operations module was used in the creation of new issue operations. You can find details about it in the Atlassian documentation at http://confluence.atlassian.com/display/JIRADEV/Issue+Operations+Plugin+Module.