










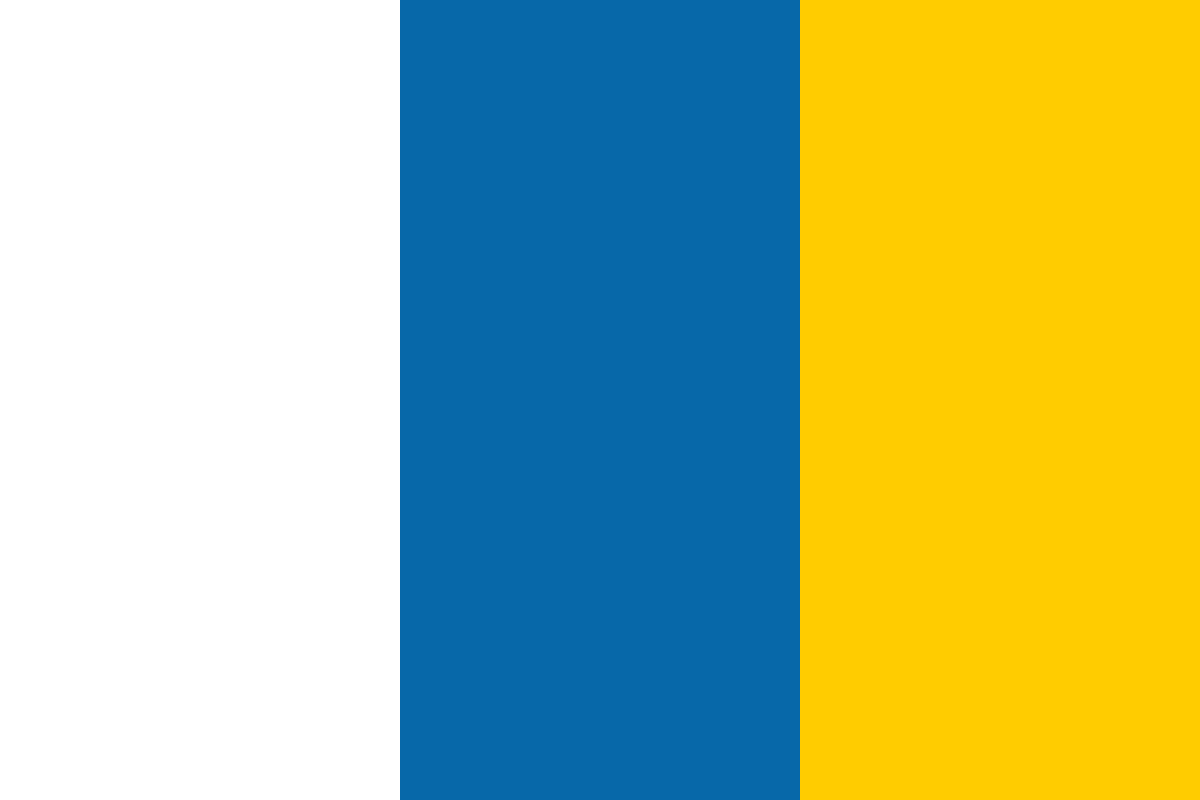

































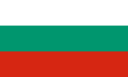








When users choose to install an application on Android, they are always presented with a warning about which permissions the application will have within their particular system. From Internet access to full Geolocation, Camera, or External Storage permissions; the user is explicitly told what rights the application will have on their system. If it seems as though the application is asking for more permissions than necessary, the user will usually refuse the install and look for another application to perform the task they need. It is very important to only require the permissions your application truly needs, or else users might be suspicious of you and the applications you make available.
There are three ways in which we can modify the Android Manifest file to set application permissions for compiling our application with Adobe AIR.
Within an AIR for Android project, open the Properties panel and click the little wrench icon next to Player selection:
The AIR for Android Settings dialog window will appear. You will be presented with a list of permissions to either enable or disable for your application. Check only the ones your application will need and click OK when finished.
<uses-permission name="android.permission.INTERNET"/>
<uses-permission name="android.permission.WRITE_EXTERNAL_
STORAGE"/>
<!--<uses-permission name="android.permission.READ_PHONE_
STATE"/>-->
<!--<uses-permission name="android.permission.ACCESS_FINE_
LOCATION"/>-->
<!--<uses-permission name="android.permission.DISABLE_
KEYGUARD"/>-->
<!--<uses-permission name="android.permission.WAKE_LOCK"/>--
>
<uses-permission name="android.permission.CAMERA"/>
<!--<uses-permission name="android.permission.RECORD_
AUDIO"/>-->
<!--<uses-permission name="android.permission.ACCESS_
NETWORK_STATE"/>-->
<!--<uses-permission name="android.permission.ACCESS_WIFI_
STATE"/>-->
The permissions you define within the AIR descriptor file will be used to create an Android Manifest file to be packaged within the .apk produced by the tool used to compile the project. These permissions restrict and enable the application, once installed on a user's device, and also alert the user as to which activities and resources the application will be given access to prior to installation. It is very important to provide only the permissions necessary for an application to perform the expected tasks once installed upon a device.
The following is a list of the possible permissions for the Android manifest document:
The Android operating system will dim, and eventually turn off the device screen after a certain amount of time has passed. It does this to preserve battery life, as the display is the primary power drain on a device. For most applications, if a user is interacting with the interface, that interaction will prevent the screen from dimming. However, if your application does not involve user interaction for lengthy periods of time, yet the user is looking at or reading something upon the display, it would make sense to prevent the screen from dimming.
There are two settings in the AIR descriptor file that can be changed to ensure the screen does not dim. We will also modify properties of our application to complete this recipe:
<uses-permission android_name="android.permission.WAKE_LOCK" />
<uses-permission android_name="android.permission.DISABLE_
KEYGUARD" />
import flash.desktop.NativeApplication;
import flash.desktop.SystemIdleMode;
import flash.display.Sprite;
import flash.display.StageAlign;
import flash.display.StageScaleMode;
import flash.text.TextField;
import flash.text.TextFormat;
private var traceField:TextField;
private var traceFormat:TextFormat;
protected function setIdleMode():void {
NativeApplication.nativeApplication.systemIdleMode =
SystemIdleMode.KEEP_AWAKE;
}
protected function setupTraceField():void {
traceFormat = new TextFormat();
traceFormat.bold = true;
traceFormat.font = "_sans";
traceFormat.size = 24;
traceFormat.align = "left";
traceFormat.color = 0xCCCCCC;
traceField = new TextField();
traceField.defaultTextFormat = traceFormat;
traceField.selectable = false;
traceField.multiline = true;
traceField.wordWrap = true;
traceField.mouseEnabled = false;
traceField.x = 20;
traceField.y = 20
traceField.width = stage.stageWidth-40;
traceField.height = stage.stageHeight - traceField.y;
addChild(traceField);
}
protected function checkIdleMode():void {
traceField.text = "System Idle Mode: " + NativeApplication.
nativeApplication.systemIdleMode;
}
There are two things that must be done in order to get this to work correctly and both are absolutely necessary. First, we have to be sure the application has correct permissions through the Android Manifest file. Allowing the application permissions for WAKE_LOCK and DISABLE_KEYGUARD within the AIR descriptor file will do this for us. The second part involves setting the NativeApplication.systemIdleMode property to keepAwake. This is best accomplished through use of the SystemIdleMode.KEEP_AWAKE constant. Ensuring that these conditions are met will enable the application to keep the device display lit and prevent Android from locking the device after it has been idle.