










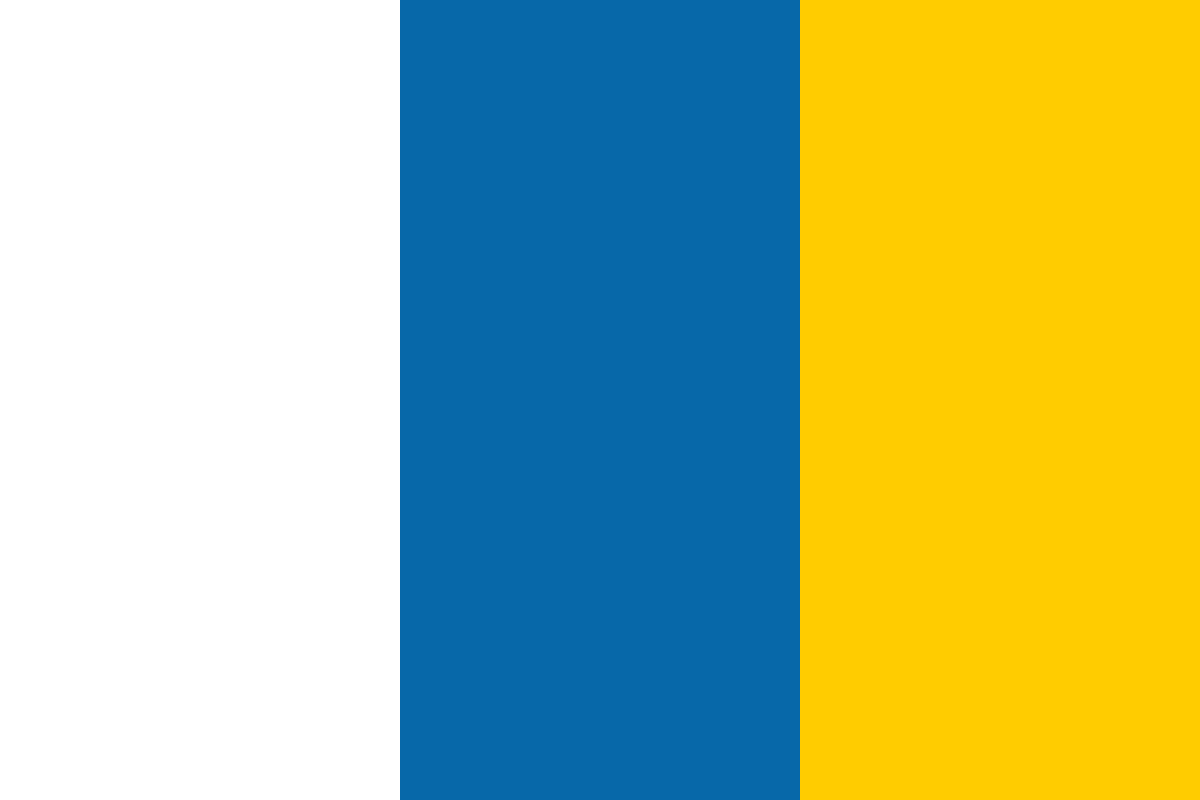

































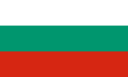








(For more resources related to this topic, see here.)
We are going to build a browsable database of cat profiles. Visitors will be able to create pages for their cats and fill in basic information such as the name, date of birth, and breed for each cat. This application will support the default Create-Retrieve-Update-Delete operations (CRUD). We will also create an overview page with the option to filter cats by breed. All of the security, authentication, and permission features are intentionally left out since they will be covered later.
Firstly, we need to define the entities of our application. In broad terms, an entity is a thing (person, place, or object) about which the application should store data. From the requirements, we can extract the following entities and attributes:
This information will help us when defining the database schema that will store the entities, relationships, and attributes, as well as the models, which are the PHP classes that represent the objects in our database.
We now need to think about the URL structure of our application. Having clean and expressive URLs has many benefits. On a usability level, the application will be easier to navigate and look less intimidating to the user. For frequent users, individual pages will be easier to remember or bookmark and, if they contain relevant keywords, they will often rank higher in search engine results.
To fulfill the initial set of requirements, we are going to need the following routes in our application:
Method |
Route |
Description |
GET |
/ |
Index |
GET |
/cats |
Overview page |
GET |
/cats/breeds/:name |
Overview page for specific breed |
GET |
/cats/:id |
Individual cat page |
GET |
/cats/create |
Form to create a new cat page |
POST |
/cats |
Handle creation of new cat page |
GET |
/cats/:id/edit |
Form to edit existing cat page |
PUT |
/cats/:id |
Handle updates to cat page |
GET |
/cats/:id/delete |
Form to confirm deletion of page |
DELETE |
/cats/:id |
Handle deletion of cat page |
We will shortly learn how Laravel helps us to turn this routing sketch into actual code. If you have written PHP applications without a framework, you can briefly reflect on how you would have implemented such a routing structure. To add some perspective, this is what the second to last URL could have looked like with a traditional PHP script (without URL rewriting): /index.php?p=cats&id=1&_action=delete&confirm=true.
The preceding table can be prepared using a pen and paper, in a spreadsheet editor, or even in your favorite code editor using ASCII characters. In the initial development phases, this table of routes is an important prototyping tool that forces you to think about URLs first and helps you define and refine the structure of your application iteratively.
If you have worked with REST APIs, this kind of routing structure will look familiar to you. In RESTful terms, we have a cats resource that responds to the different HTTP verbs and provides an additional set of routes to display the necessary forms.
If, on the other hand, you have not worked with RESTful sites, the use of the PUT and DELETE HTTP methods might be new to you. Even though web browsers do not support these methods for standard HTTP requests, Laravel uses a technique that other frameworks such as Rails use, and emulates those methods by adding a _method input field to the forms. This way, they can be sent over a standard POST request and are then delegated to the correct route or controller method in the application.
Note also that none of the form submissions endpoints are handled with a GET method. This is primarily because they have side effects; a user could trigger the same action multiple times accidentally when using the browser history. Therefore, when they are called, these routes never display anything to the users. Instead, they redirect them after completing the action (for instance, DELETE /cats/:id will redirect the user to GET/cats).
Now that we have the blueprints for the application, let's roll up our sleeves and start writing some code.
Start by opening a new terminal window and create a new project with Composer, as follows:
$ composer create-project laravel/laravel cats --prefer-dist $ cd cats
Once Composer finishes downloading Laravel and resolving its dependencies, you will have a directory structure identical to the one presented previously.
To start the application, unless you are running an older version of PHP (5.3.*), you will not need a local server such as WAMP on Windows or MAMP on Mac OS since Laravel uses the built-in development server that is bundled with PHP 5.4 or later.
To start the development server, we use the following artisan command:
$ php artisan serve
Artisan is the command-line utility that ships with Laravel and its features will be covered in more detail later.
Next, open your web browser and visit http://localhost:8000; you will be greeted with Laravel's welcome message.
If you get an error telling you that the php command does not exist or cannot be found, make sure that it is present in your PATH variable. If the command fails because you are running PHP 5.3 and you have no upgrade possibility, simply use your local development server (MAMP/WAMP) and set Apache's DocumentRoot to point to cats-app/public/.
Let's start by writing the first two routes of our application inside app/routes.php. This file already contains some comments as well as a sample route. You can keep the comments but you must remove the existing route before adding the following routes:
Route::get('/', function(){ return "All cats"; }); Route::get('cats/{id}', function($id){ return "Cat #$id"; });
The first parameter of the get method is the URI pattern. When a pattern is matched, the closure function in the second parameter is executed with any parameters that were extracted from the pattern. Note that the slash prefix in the pattern is optional; however, you should not have any trailing slashes. You can make sure that your routes work by opening your web browser and visiting http://localhost:8000/cats/123.
If you are not using PHP's built-in development server and are getting a 404 error at this stage, make sure that Apache's mod_rewrite configuration is enabled and works correctly.
In the pattern of the second route, {id} currently matches any string or number. To restrict it so that it only matches numbers, we can chain a where method to our route as follows:
Route::get('cats/{id}', function($id){ return "Cat #$id"; })->where('id', '[0-9]+');
The where method takes two arguments: the first one is the name of the parameter and the second one is the regular expression pattern that it needs to match.
We have covered a lot in this article. We learned how to define routes, prepare the models of the application, and interact with them. Moreover, we have had a glimpse at the many powerful features of Eloquent, Blade, as well as the other convenient helpers in Laravel to create forms and input fields: all of this in under 200 lines of code!
Further resources on this subject: