










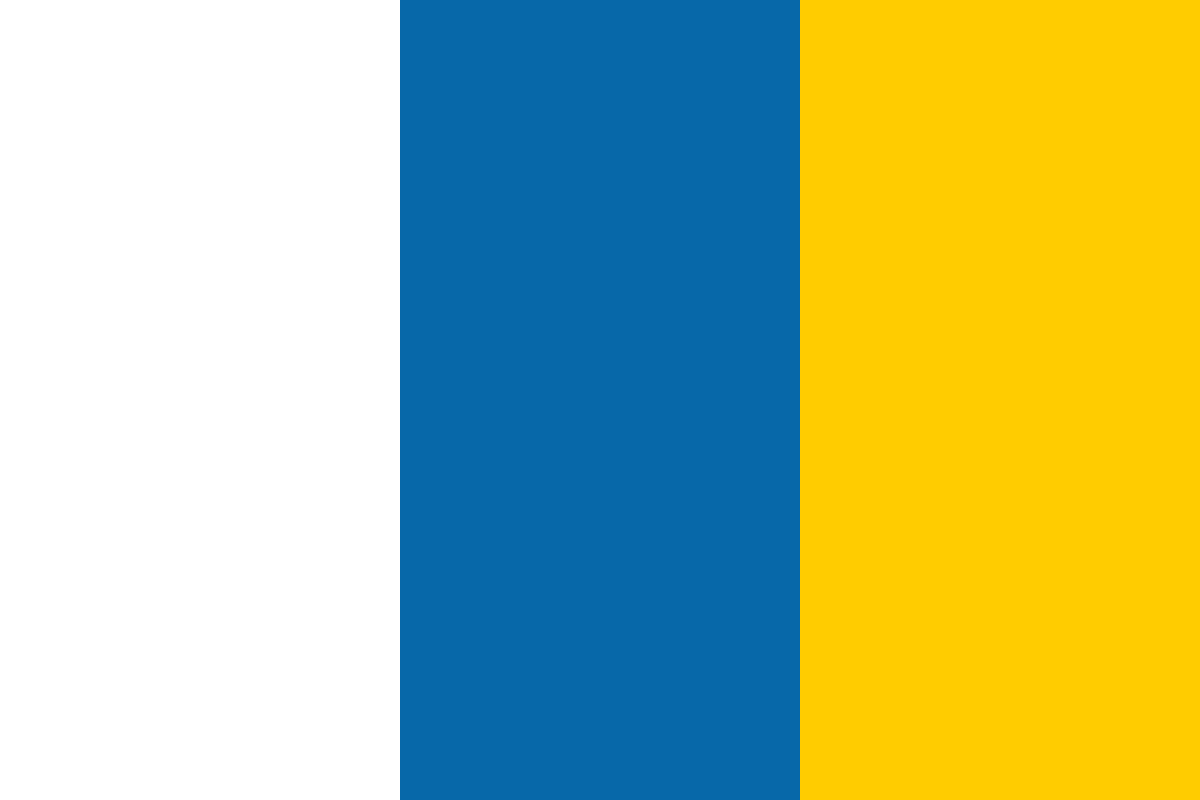

































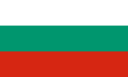








In this article, by Nishant Verma, author of the book Mobile Test Automation with Appium, you will learn about creating a new cucumber, appium Java project in IntelliJ. Next, you will learn to write a sample feature and automate, thereby learning how to start appium server session with an app using appium app, find locators using appium inspector and write java classes for each step implementation in the feature file. We will also discuss, how to write the test for mobile web and use Chrome Developer Tool to find the locators. Let's get started!
In this article, we will discuss the following topics:
(For more resources related to this topic, see here.)
Let's create a sample appium Java project in IntelliJ. Below steps will help you do the same:
compile group: 'info.cukes', name: 'cucumber-java', version:
'1.2.5'
compile group: 'io.appium', name: 'java-client', version:
'5.0.0-BETA6'
group 'com.test'
version '1.0-SNAPSHOT'
apply plugin: 'java'
sourceCompatibility = 1.5
repositories {
mavenCentral()
}
dependencies {
testCompile group: 'junit', name: 'junit', version: '4.11'
compile group: 'info.cukes', name: 'cucumber-java',
version:'1.2.5'
compile group: 'io.appium', name: 'java-client',
version:'5.0.0-BETA6'
}
We are now ready to write our first sample feature file but before that let's try to understand a brief about cucumber.
Cucumber is a test framework which supports behaviour driven development (or BDD). The core idea behind BDD is a domain specific language (known as DSL), where the tests are written in normal English, expressing how the application or system has to behave. DSL is an executable test, which starts with a known state, perform some action and verify the expected state. For e.g.
As a user I should be able to see Facebook/Google button. When I try to register myself in Quikr.
Dan North (creator of BDD) defined behaviour-driven development in 2009 as- BDD is a second-generation, outside-in, pull-based, multiple-stakeholder, multiple-scale, high-automation, agile methodology. It describes a cycle of interactions with well-defined outputs, resulting in the delivery of working, tested software that matters.
Cucumber feature files serve as a living documentation which can be implemented in many languages. It was first implemented in Ruby and later extended to Java. Some of the basic features of Cucumber are:
So in the above example, Feature, Scenario, Given, When, Then are keywords.
So I am hoping now we have a brief idea of what cucumber is. Further details can be read on their site (https://cucumber.io/). In the coming section, we will create a feature file, write a scenario, implement the code behind and execute it.
Till now we have created a sample Java project and added the appium dependency. Next we need to add a cucumber feature file and implement the code behind. Let's start that:
Feature: Hello World.
Scenario: Registration Flow Validation via App.
As a user I should be able to see my google account.
when I try to register myself in Quikr.
When I launch Quikr app.
And I choose to log in using Google.
Then I see account picker screen with my email address "testemail@gmail.com".
So the idea is that the steps will belong to a page and each page would typically have it's own step implementation class.
Now we need to implement these methods and write the code behind to launch Quikr app on emulator.
First thing we need to do, is to download a sample app (Quikr apk in this case). Download the Quikr app (version 9.16).
On the appium GUI app, click on the android icon and select the below options:
Once the above settings are done, click on General Settings icon and choose the below mentioned settings. Once the setup is done, click on the icon to close the pop up.
This would start the appium server session. Once you click on Appium Inspector, it will install the app on emulator and launch the same.
If you click on the Record button, it will generate the boilerplate code which has Desired Capabilities respective to the run environment and app location:
We can copy the above line and put into the code template generated for the step When I launch Quikr app.
This is how the code should look like after copying it in the method:
@When("^I launch Quikr app$")
public void iLaunchQuikrApp() throws Throwable {
DesiredCapabilities capabilities = new DesiredCapabilities();
capabilities.setCapability("appium-version", "1.0");
capabilities.setCapability("platformName", "Android");
capabilities.setCapability("platformVersion", "5.1");
capabilities.setCapability("deviceName", "Nexus6");
capabilities.setCapability("app",
"/Users/nishant/Development/HelloAppium/app/quikr.apk");
AppiumDriver wd = new AppiumDriver(new
URL("http://0.0.0.0:4723/wd/hub"), capabilities);
wd.manage().timeouts().implicitlyWait(60, TimeUnit.
SECONDS
);
}
Now the above code only sets the Desired Capabilities, appium server is yet to be started. For now, we can start it from outside like terminal (or command prompt) by running the command appium.
We can close the appium inspector and stop the appium server by click in onStop on the appium GUI app.
To run the above test, we need to do the following:
Now the scope of AppiumDriver is local to the method, hence we can refactor and extract appiumDriver as field.
To continue with the other steps automation, we can use the appium inspector to find the element handle. We can launch appium inspector using the above mentioned steps, then click on the element whose locator we want to find out as shown in the below mentioned screen.
Once we have the locator, we can use the appium api (as shown below) to click it:
appiumDriver.findElement(By.id("sign_in_button")).click();
This way we can implement the remaining steps.
To automate mobile web app, we don't need to install the app on the device. We need a browser and the app url which is sufficient to start the test automation. We can tweak the above written code by adding a desired capability browserName. We can write a similar scenario and make it mobile web specific:
Scenario: Registration Flow Validation via web
As a User I want to verify that
I get the option of choosing Facebook when I choose to register
When I launch Quikr mobile web
And I choose to register
Then I should see an option to register using Facebook
So the method for mobile web would look like:
@When("^I launch Quikr mobile web$")
public void iLaunchQuikrMobileWeb() throws Throwable {
DesiredCapabilities desiredCapabilities = new
DesiredCapabilities();
desiredCapabilities.setCapability("platformName", "Android");
desiredCapabilities.setCapability("deviceName", "Nexus");
desiredCapabilities.setCapability("browserName", "Browser");
URL url = new URL("http://127.0.0.1:4723/wd/hub");
appiumDriver = new AppiumDriver(url, desiredCapabilities);
appiumDriver.get("http://m.quikr.com");
}
So in the above code, we don't need platformVersion and we need a valid value for browserName parameter.
Possible values for browserName are:
We can follow the same steps as above to run the test.
To implement the remaining steps of above mentioned feature, we need to find locators for the elements we want to interact with. Once the locators are found then we need to perform the desired operation which could be click, send keys etc.
Below mentioned are the steps which will help us find the locators for a mobile web app:
appiumDriver.findElement(By.id("query")).click();
Using the above way, we can find the locator of the various elements we need to interact with and proceed with our test automation.
So in this article, we briefly described how we would go about writing test for a native app as well as a mobile web. We discussed how to create a project in IntelliJ and write a sample feature file. We also learned how to start the appium inspector and look for locator. We learned about the chrome dev tool and how can use the same to find locator for mobile web.
Further resources on this subject: