










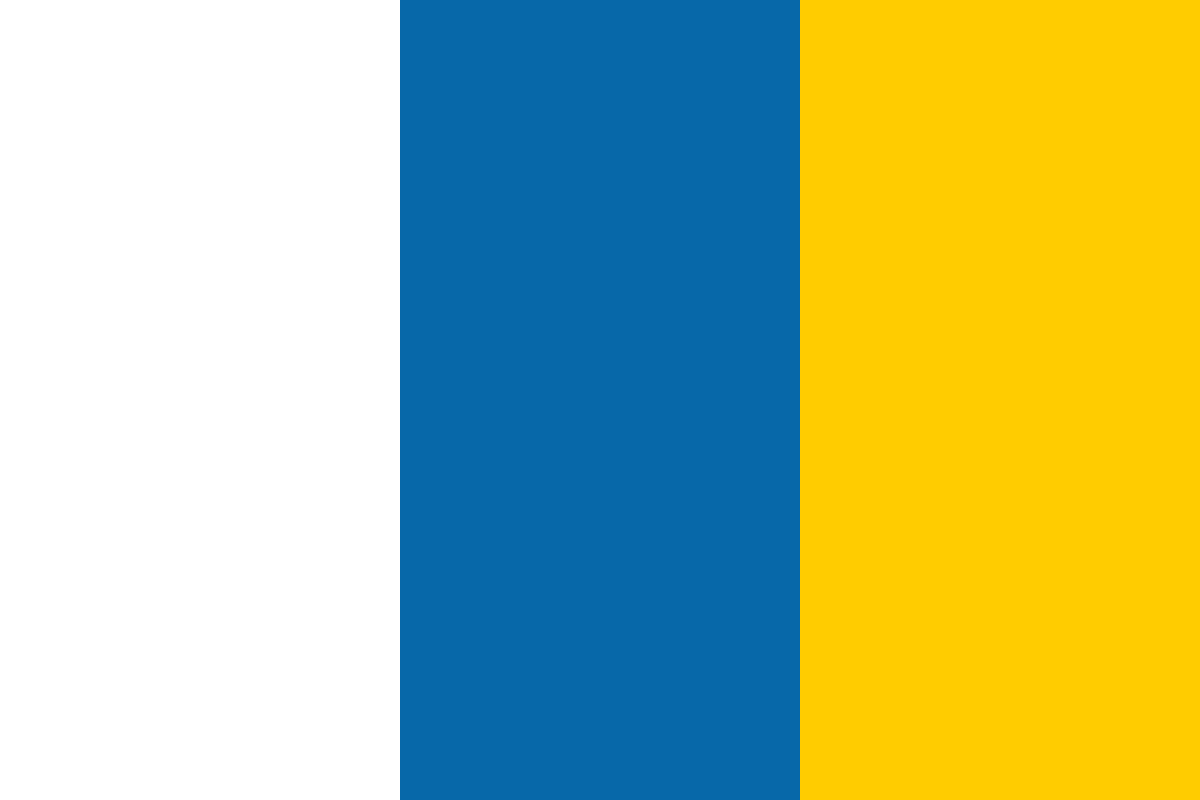

































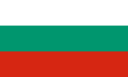








In today's world, many server-side applications make use of XML to structure data because XML is a standard way of representing structured information. It is easy to work with, and people can easily read, write, and understand XML without the need of any specialized skills. The XML standard is widely accepted and used in server communications such as Simple Object Access Protocol (SOAP) based web services. XML stands for eXtensible Markup Language. The XML standard specification is available at http://www.w3.org/XML/.
Adobe Flex provides a standardized ECMAScript-based set of API classes and functionality for working with XML data. This collection of classes and functionality provided by Flex are known as E4X. You can use these classes provided by Flex to build sophisticated Rich Internet Applications using XML data.
XML is a standard way to represent categorized data into a tree structure similar to HTML documents. XML is written in plain-text format, and hence it is very easy to read, write, and manipulate its data.
A typical XML document looks like this:
<book> <title>Flex 3 with Java</title> <author>Satish Kore</author> <publisher>Packt Publishing</publisher> <pages>300</pages> </book>
Generally, XML data is known as XML documents and it is represented by tags wrapped in angle brackets (< >). These tags are also known as XML elements. Every XML document starts with a single top-level element known as the root element. Each element is distinguished by a set of tags known as the opening tag and the closing tag. In the previous XML document, <book> is the opening tag and </book> is the closing tag. If an element contains no content, it can be written as an empty statement (also called self-closing statement). For example, <book/> is as good as writing <book></book>.
XML documents can also be more complex with nested tags and attributes, as shown in the following example:
<book ISBN="978-1-847195-34-0"> <title>Flex 3 with Java</title> <author country="India" numberOfBooks="1"> <firstName>Satish</firstName> <lastName>Kore</lastName> </author> <publisher country="United Kingdom">Packt Publishing</publisher> <pages>300</pages> </book>
Notice that the above XML document contains nested tags such as <firstName> and <lastName> under the <author> tag. ISBN, country, and numberOfBooks, which you can see inside the tags, are called XML attributes.
To learn more about XML, visit the W3Schools' XML Tutorial at http://w3schools.com/xml/.
Flex provides a set of API classes and functionality based on the ECMAScript for XML (E4X) standards in order to work with XML data. The E4X approach provides a simple and straightforward way to work with XML structured data, and it also reduces the complexity of parsing XML documents.
Earlier versions of Flex did not have a direct way of working with XML data. The E4X provides an alternative to DOM (Document Object Model) interface that uses a simpler syntax for reading and querying XML documents. More information about other E4X implementations can be found at http://en.wikipedia.org/wiki/E4X.
The key features of E4X include:
ActionScript 3.0 includes the following E4X classes: XML, XMLList, QName, and Namespace. These classes are designed to simplify XML data processing into Flex applications.
Let's see one quick example:
Define a variable of type XML and create a sample XML document. In this example, we will assign it as a literal. However, in the real world, your application might load XML data from external sources, such as a web service or an RSS feed.
private var myBooks:XML = <books publisher="Packt Pub"> <book title="Book1" price="99.99"> <author>Author1</author> </book> <book title="Book2" price="59.99"> <author>Author2</author> </book> <book title="Book3" price="49.99"> <author>Author3</author> </book> </books>;
Now, we will see some of the E4X approaches to read and parse the above XML in our application. The E4X uses many operators to simplify accessing XML nodes and attributes, such as dot (.) and attribute identifier (@), for accessing properties and attributes.
private function traceXML():void { trace(myBooks.book.(@price < 50.99).@title); //Output: Book3 trace(myBooks.book[1].author); //Output: Author2 trace(myBooks.@publisher); //Output: Packt Pub //Following for loop outputs prices of all books for each(var price in myBooks..@price) { trace(price); } }
In the code above, we are using a conditional expression to extract the title of the book(s) whose price is set below 50.99$ in the first trace statement. If we have to do this manually, imagine how much code would have been needed to parse the XML. In the second trace, we are accessing a book node using index and printing its author node's value. And in the third trace, we are simply printing the root node's publisher attribute value and finally, we are using a for loop to traverse through prices of all the books and printing each price.
The following is a list of XML operators:
Operator | Name | Description | |
@
|
attribute identifier | Identifies attributes of an XML or XMLList object.
|
|
{ }
|
braces(XML) | Evaluates an expression that is used in an XML or XMLList initializer. | |
[ ]
|
brackets(XML) | Accesses a property or attribute of an XML or XMLList object, for example myBooks.book["@title"].
|
|
+ | concatenation(XMLList) | Concatenates (combines) XML or XMLList values into an XMLList object.
|
|
+= | concatenation assignment (XMLList) | Assigns expression1 |
An XML class represents an XML element, attribute, comment, processing instruction, or a text element.
We have used the XML class in our example above to initialize the myBooks variable with an XML literal. The XML class is included into an ActionScript 3.0 core class, so you don't need to import a package to use it.
The XML class provides many properties and methods to simplify XML processing, such as ignoreWhitespace and ignoreComments properties, used for ignoring whitespaces and comments in XML documents respectively. You can use the prependChild() and appendChild() methods to prepend and append XML nodes to existing XML documents. Methods such as toString() and toXMLString() allow you to convert XML to a string.
An example of an XML object:
private var myBooks:XML = <books publisher="Packt Pub"> <book title="Book1" price="99.99"> <author>Author1</author> </book> <book title="Book2" price="120.00"> <author>Author2</author> </book> </books>;
In the above example, we have created an XML object by assigning an XML literal to it. You can also create an XML object from a string that contains XML data, as shown in the following example:
private var str:String = "<books publisher="Packt Pub"> <book title="Book1" price="99.99"> <author>Author1</author> </book> <book title="Book2" price="59.99"> <author>Author2</author> </book> </books>"; private var myBooks:XML = new XML(str); trace(myBooks.toXMLString()); //outputs formatted xml as string
If the XML data in string is not well-formed (for example, a closing tag is missing), then you will see a runtime error.
You can also use binding expressions in the XML text to extract contents from a variable data. For example, you could bind a node's name attribute to a variable value, as in the following line:
private var title:String = "Book1" var aBook:XML = <book title="{title}">;
To read more about XML class methods and properties, go through Flex 3 LiveDocs at http://livedocs.adobe.com/flex/3/langref/XML.html.
As the class name indicates, XMLList contains one or more XML objects. It can contain full XML documents, XML fragments, or the results of an XML query.
You can typically use all of the XML class's methods and properties on the objects from XMLList. To access these objects from the XMLList collection, iterate over it using a for each… statement.
The XMLList provides you with the following methods to work with its objects:
For details on these methods, see the ActionScript 3.0 Language Reference.
Let's return to the example of the XMLList:
var xmlList:XMLList = myBooks.book.(@price == 99.99); var item:XML; for each(item in xmlList) { trace("item:"+item.toXMLString()); }
Output:
item:<book title="Book1" price="99.99">
<author>Author1</author>
</book>
In the example above, we have used XMLList to store the result of the myBooks.book.(@price == 99.99); statement. This statement returns an XMLList containing XML node(s) whose price is 99.99$.
The XML class provides many useful methods to work with XML objects, such as the appendChild() and prependChild() methods to add an XML element to the beginning or end of an XML object, as shown in the following example:
var node1:XML = <middleInitial>B</middleInitial> var node2:XML = <lastName>Kore</lastName> var root:XML = <personalInfo></personalInfo> root = root.appendChild(node1); root = root.appendChild(node2); root = root.prependChild(<firstName>Satish</firstName>);
The output is as follows:
<personalInfo>
<firstName>Satish</firstName>
<middleInitial>B</middleInitial>
<lastName>Kore</lastName>
</personalInfo>
You can use the insertChildBefore() or insertChildAfter() method to add a property before or after a specified property, as shown in the following example:
var x:XML = <count> <one>1</one> <three>3</three> <four>4</four> </count>; x = x.insertChildBefore(x.three, "<two>2</two>"); x = x.insertChildAfter(x.four, "<five>5</five>"); trace(x.toXMLString());
The output of the above code is as follows:
<count>
<one>1</one>
<two>2</two>
<three>3</three>
<four>4</four>
<five>5</five>
</count>