










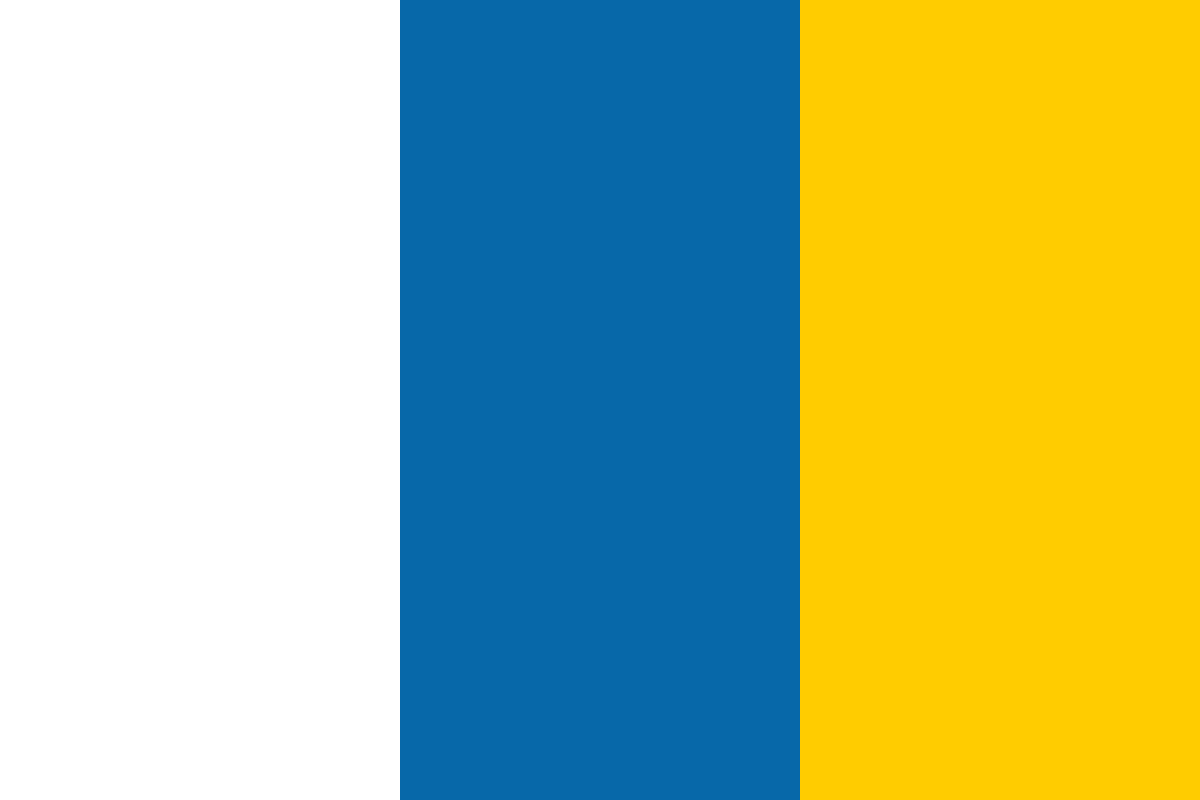

































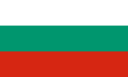








Over 60 simple but highly effective recipes to create interactive web applications using PHP with jQuery
Extensible Markup Language—also known as XML—is a structure for representation of data in human readable format. Contrary to its name, it's actually not a language but a markup which focuses on data and its structure. XML is a lot like HTML in syntax except that where HTML is used for presentation of data, XML is used for storing and data interchange.
Moreover, all the tags in an XML are user-defined and can be formatted according to one's will. But an XML must follow the specification recommended by W3C.
With a large increase in distributed applications over the internet, XML is the most widely used method of data interchange between applications. Web services use XML to carry and exchange data between applications. Since XML is platform-independent and is stored in string format, applications using different server-side technologies can communicate with each other using XML.
Consider the following XML document:
From the above document, we can infer that it is a list of websites containing data about the name, URL, and some information about each website.
PHP has several classes and functions available for working with XML documents. You can read, write, modify, and query documents easily using these functions.
In this article, we will discuss SimpleXML functions and DOMDocument class of PHP for manipulating XML documents. You will learn how to read and modify XML files, using SimpleXML as well as DOM API. We will also explore the XPath method, which makes traversing documents a lot easier.
Note that an XML must be well-formed and valid before we can do anything with it. There are many rules that define well-formedness of XML out of which a few are given below:
To know more about validity of an XML, you can refer to this link:
http://en.wikipedia.org/wiki/XML#Schemas_and_validation
For most of the recipes in this article, we will use an already created XML file. Create a new file, save it as common.xml in the Article3 directory. Put the following contents in this file.
<?xml version="1.0"?>
<books>
<book index="1">
<name year="1892">The Adventures of Sherlock Holmes</name>
<story>
<title>A Scandal in Bohemia</title>
<quote>You see, but you do not observe. The distinction
is clear.</quote>
</story>
<story>
<title>The Red-headed League</title>
<quote>It is quite a three pipe problem, and I beg that you
won't speak to me for fifty minutes.</quote>
</story>
<story>
<title>The Man with the Twisted Lip</title>
<quote>It is, of course, a trifle, but there is nothing so
important as trifles.</quote>
</story>
</book>
<book index="2">
<name year="1927">The Case-book of Sherlock Holmes</name>
<story>
<title>The Adventure of the Three Gables</title>
<quote>I am not the law, but I represent justice so far as
my feeble powers go.</quote>
</story>
<story>
<title>The Problem of Thor Bridge</title>
<quote>We must look for consistency. Where there is a want
of it we must suspect deception.</quote>
</story>
<story>
<title>The Adventure of Shoscombe Old Place</title>
<quote>Dogs don't make mistakes.</quote>
</story>
</book>
<book index="3">
<name year="1893">The Memoirs of Sherlock Holmes</name>
<story>
<title>The Yellow Face</title>
<quote>Any truth is better than indefinite doubt.</quote>
</story>
<story>
<title>The Stockbroker's Clerk</title>
<quote>Results without causes are much more impressive. </quote>
</story>
<story>
<title>The Final Problem</title>
<quote>If I were assured of your eventual destruction I would,
in the interests of the public, cheerfully accept my
own.</quote>
</story>
</book>
</books>
True to its name, SimpleXML functions provide an easy way to access data from XML documents. XML files or strings can be converted into objects, and data can be read from them.
We will see how to load an XML from a file or string using SimpleXML functions. You will also learn how to handle errors in XML documents.
Create a new directory named Article3. This article will contain sub-folders for each recipe. So, create another folder named Recipe1 inside it.
<?php
libxml_use_internal_errors(true);
$objXML = simplexml_load_file('../common.xml');
if (!$objXML)
{
$errors = libxml_get_errors();
foreach($errors as $error)
{
echo $error->message,'<br/>';
}
}
else
{
foreach($objXML->book as $book)
{
echo $book->name.'<br/>';
}
}
?>
In the first line, passing a true value to the libxml_use_internal_errors function will suppress any XML errors and will allow us to handle errors from the code itself. The second line tries to load the specified XML using the simplexml_load_file function. If the XML is loaded successfully, it is converted into a SimpleXMLElement object otherwise a false value is returned.
We then check for the return value. If it is false, we use the libxml_get_errors() function to get all the errors in the form of an array. This array contains objects of type LibXMLError. Each of these objects has several properties. In the previous code, we iterated over the errors array and echoed the message property of each object which contains a detailed error message.
If there are no errors in XML, we get a SimpleXMLElement object which has all the XML data loaded in it.
More parameters are available for the simplexml_load_file method, which are as follows:
Similar to simplexml_load_file is simplexml_load_string, which also creates a SimpleXMLElement on successful execution. If a valid XML string is passed to it we get a SimpleXMLElement object or a false value otherwise.
$objXML = simplexml_load_string('<?xml version="1.0"?><book><name>
Myfavourite book</name></book>');
The above code will return a SimpleXMLElement object with data loaded from the XML string. The second and third parameters of this function are same as that of simplexml_load_file.
You can also use the constructor of the SimpleXMLElement class to create a new object.
$objXML = new SimpleXMLElement('<?xml version="1.0"?><book><name>
Myfavourite book</name></book>');
You can read about SimpleXML in more detail on the PHP site at http://php.net/manual/en/book.simplexml.php and about libxml at http://php.net/manual/en/book.libxml.php.