










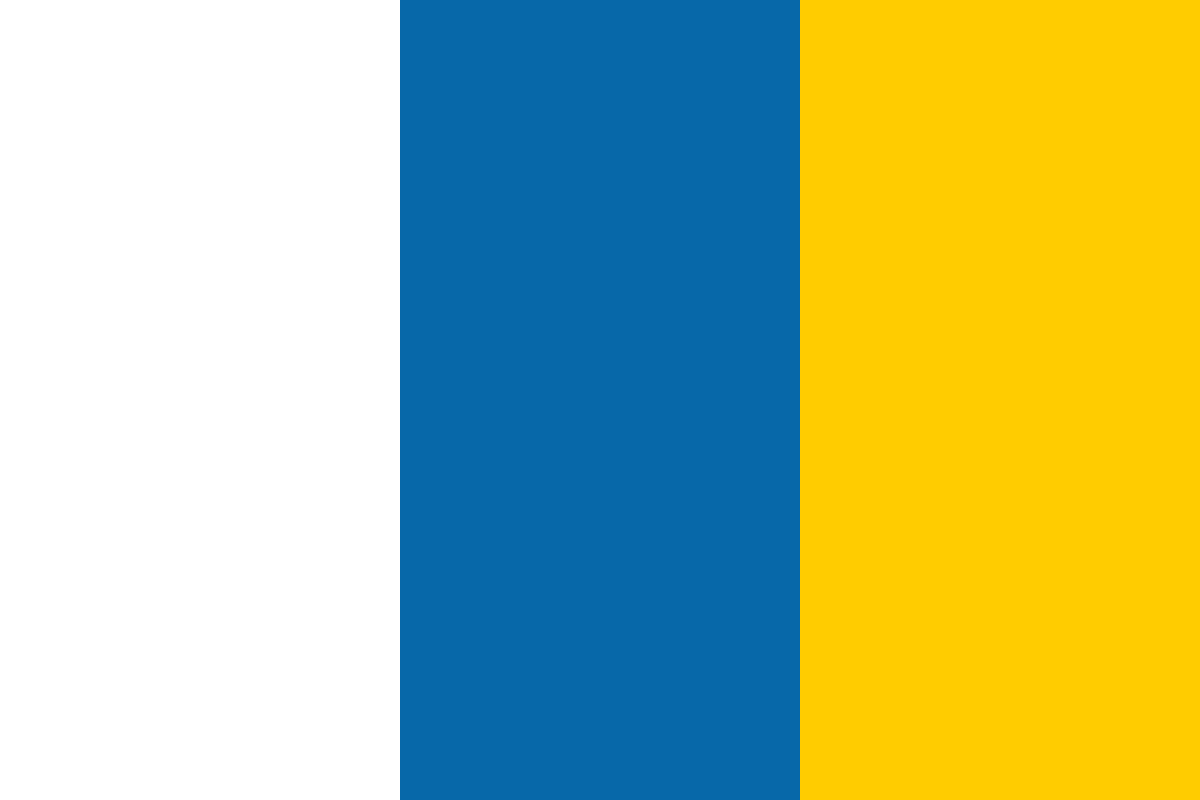

































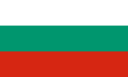








In this article by Ashwin Pajankar and Arush Kakkar, the author of the book Raspberry Pi By Example we will learn how to use different types and uses of cameras with our Pi. Let's take a look at the topics we will study and implement in this article:
(For more resources related to this topic, see here.)
USB webcams are a great way to capture images and videos. Raspberry Pi supports common USB webcams.
To be on the safe side, here is a list of the webcams supported by Pi: http://elinux.org/RPi_USB_Webcams.
I am using a Logitech HD c310 USB Webcam.
You can purchase it online, and you can find the product details and the specifications at http://www.logitech.com/en-in/product/hd-webcam-c310.
Attach your USB webcam to Raspberry Pi through the USB port on Pi and run the lsusb command in the terminal. This command lists all the USB devices connected to the computer. The output should be similar to the following output depending on which port is used to connect the USB webcam:
pi@raspberrypi ~/book/chapter04 $ lsusb
Bus 001 Device 002: ID 0424:9514 Standard Microsystems Corp.
Bus 001 Device 001: ID 1d6b:0002 Linux Foundation 2.0 root hub
Bus 001 Device 003: ID 0424:ec00 Standard Microsystems Corp.
Bus 001 Device 004: ID 148f:2070 Ralink Technology, Corp. RT2070 Wireless Adapter
Bus 001 Device 007: ID 046d:081b Logitech, Inc. Webcam C310
Bus 001 Device 006: ID 1c4f:0003 SiGma Micro HID controller
Bus 001 Device 005: ID 1c4f:0002 SiGma Micro Keyboard TRACER Gamma Ivory
Then, install the fswebcam utility by running the following command:
sudo apt-get install fswebcam
The fswebcam is a simple command-line utility that captures images with webcams for Linux computers. Once the installation is done, you can use the following command to create a directory for output images:
mkdir /home/pi/book/output
Then, run the following command to capture the image:
fswebcam -r 1280x960 --no-banner ~/book/output/camtest.jpg
This will capture an image with a resolution of 1280 x 960. You might want to try another resolution for your learning. The --no-banner command will disable the timestamp banner. The image will be saved with the filename mentioned. If you run this command multiple times with the same filename, the image file will be overwritten each time. So, make sure that you change the filename if you want to save previously captured images. The text output of the command should be similar to the following:
--- Opening /dev/video0...
Trying source module v4l2...
/dev/video0 opened.
No input was specified, using the first.
--- Capturing frame...
Corrupt JPEG data: 2 extraneous bytes before marker 0xd5
Captured frame in 0.00 seconds.
--- Processing captured image...
Disabling banner.
Writing JPEG image to '/home/pi/book/output/camtest.jpg'.
A cron is a time-based job scheduler in Unix-like computer operating systems. It is driven by a crontab (cron table) file, which is a configuration file that specifies shell commands to be run periodically on a given schedule. It is used to schedule commands or shell scripts to run periodically at a fixed time, date, or interval.
The syntax for crontab in order to schedule a command or script is as follows:
1 2 3 4 5 /location/command
Here, the following are the definitions:
The crontab entry to run any script or command every minute is as follows:
* * * * * /location/command 2>&1
In the next section, we will learn how to use crontab to schedule a script to capture images periodically in order to create the timelapse sequence.
You can refer to this URL for more details oncrontab: http://www.adminschoice.com/crontab-quick-reference.
Timelapse photography means capturing photographs in regular intervals and playing the images with a higher frequency in time than those that were shot. For example, if you capture images with a frequency of one image per minute for 10 hours, you will get 600 images. If you combine all these images in a video with 30 images per second, you will get 10 hours of timelapse video compressed in 20 seconds. You can use your USB webcam with Raspberry Pi to achieve this. We already know how to use the Raspberry Pi with a Webcam and the fswebcam utility to capture an image. The trick is to write a script that captures images with different names and then add this script in crontab and make it run at regular intervals.
Begin with creating a directory for captured images:
mkdir /home/pi/book/output/timelapse
Open an editor of your choice, write the following code, and save it as timelapse.sh:
#!/bin/bash
DATE=$(date +"%Y-%m-%d_%H%M")
fswebcam -r 1280x960 --no-banner
/home/pi/book/output/timelapse/garden_$DATE.jpg
Make the script executable using:
chmod +x timelapse.sh
This shell script captures the image and saves it with the current timestamp in its name. Thus, we get an image with a new filename every time as the file contains the timestamp. The second line in the script creates the timestamp that we're using in the filename. Run this script manually once, and make sure that the image is saved in the /home/pi/book/output/timelapse directory with the garden_<timestamp>.jpg name.
To run this script at regular intervals, we need to schedule it in crontab.
The crontab entry to run our script every minute is as follows:
* * * * * /home/pi/book/chapter04/timelapse.sh 2>&1
Open the crontab of the Pi user with crontab –e. It will open crontab with nano as the editor. Add the preceding line to crontab, save it, and exit it.
Once you exit crontab, it will show the following message:
no crontab for pi - using an empty one
crontab: installing new crontab
Our timelapse webcam setup is now live. If you want to change the image capture frequency, then you have to change the crontab settings. To set it every 5 minutes, change it to */5 * * * *. To set it for every 2 hours, use 0 */2 * * *. Make sure that your MicroSD card has enough free space to store all the images for the time duration for which you need to keep your timelapse setup.
Once you capture all the images, the next part is to encode them all in a fast playing video, preferably 20 to 30 frames per second. For this part, the mencoder utility is recommended. The following are the steps to create a timelapse video with mencoder on a Raspberry Pi or any Debian/Ubuntu machine:
cd /home/pi/book/output/timelapse
ls garden_*.jpg > timelapse.txt
mencoder -nosound -ovc lavc -lavcopts vcodec=mpeg4:aspect=16/9:vbitrate=8000000 -vf scale=1280:960 -o timelapse.avi -mf type=jpeg:fps=30 mf://@timelapse.txt
This will create a video with name timelapse.avi in the current directory with all the images listed in timelapse.txt with a 30 fps frame rate. The statement contains the details of the video codec, aspect ratio, bit rate, and scale. For more information, you can run man mencoder on Command Prompt. We will cover how to play a video in the next section.
We can use a webcam to record live videos using avconv. Install avconv using sudo apt-get install libav-tools. Use the following command to record a video:
avconv -f video4linux2 -r 25 -s 1280x960 -i /dev/video0 ~/book/output/VideoStream.avi
It will show following output on the screen.
pi@raspberrypi ~ $ avconv -f video4linux2 -r 25 -s 1280x960 -i /dev/video0 ~/book/output/VideoStream.avi
avconv version 9.14-6:9.14-1rpi1rpi1, Copyright (c) 2000-2014 the Libav developers
built on Jul 22 2014 15:08:12 with gcc 4.6 (Debian 4.6.3-14+rpi1)
[video4linux2 @ 0x5d6720] The driver changed the time per frame from 1/25 to 2/15
[video4linux2 @ 0x5d6720] Estimating duration from bitrate, this may be inaccurate
Input #0, video4linux2, from '/dev/video0':
Duration: N/A, start: 629.030244, bitrate: 147456 kb/s
Stream #0.0: Video: rawvideo, yuyv422, 1280x960, 147456 kb/s, 1000k tbn, 7.50 tbc
Output #0, avi, to '/home/pi/book/output/VideoStream.avi':
Metadata:
ISFT : Lavf54.20.4
Stream #0.0: Video: mpeg4, yuv420p, 1280x960, q=2-31, 200 kb/s, 25 tbn, 25 tbc
Stream mapping:
Stream #0:0 -> #0:0 (rawvideo -> mpeg4)
Press ctrl-c to stop encoding
frame= 182 fps= 7 q=31.0 Lsize= 802kB time=7.28 bitrate= 902.4kbits/s
video:792kB audio:0kB global headers:0kB muxing overhead 1.249878%
Received signal 2: terminating.
You can terminate the recording sequence by pressing Ctrl + C.
We can play the video using omxplayer. It comes with the latest raspbian, so there is no need to install it. To play a file with the name vid.mjpg, use the following command:
omxplayer ~/book/output/VideoStream.avi
It will play the video and display some output similar to the one here:
pi@raspberrypi ~ $ omxplayer ~/book/output/VideoStream.avi
Video codec omx-mpeg4 width 1280 height 960 profile 0 fps 25.000000
Subtitle count: 0, state: off, index: 1, delay: 0
V:PortSettingsChanged: 1280x960@25.00 interlace:0 deinterlace:0 anaglyph:0 par:1.00 layer:0
have a nice day ;)
Try playing timelapse and record videos using omxplayer.
These camera modules are specially manufactured for Raspberry Pi and work with all the available models. You will need to connect the camera module to the CSI port, located behind the Ethernet port, and activate the camera using the raspi-config utility if you haven't already.
You can find the video instructions to connect the camera module to Raspberry Pi at http://www.raspberrypi.org/help/camera-module-setup/.
This page lists the types of camera modules available: http://www.raspberrypi.org/products/.
Two types of camera modules are available for the Pi. These are Pi Camera and Pi NoIR camera, and they can be found at https://www.raspberrypi.org/products/camera-module/ and https://www.raspberrypi.org/products/pi-noir-camera/, respectively.
The following image shows Pi Camera and Pi NoIR Camera boards side by side:
The following image shows the Pi Camera board connected to the Pi:
The following is an image of the Pi camera board placed in the camera case:
The main difference between Pi Camera and Pi NoIR Camera is that Pi Camera gives better results in good lighting conditions, whereas Pi NoIR (NoIR stands for No-Infra Red) is used for low light photography. To use NoIR Camera in complete darkness, we need to flood the object to be photographed with infrared light.
This is a good time to take a look at the various enclosures for Raspberry Pi Models. You can find various cases available online at https://www.adafruit.com/categories/289.
An example of a Raspberry Pi case is as follows:
To capture images and videos using the Raspberry Pi camera module, we need to use raspistill and raspivid utilities.
To capture an image, run the following command:
raspistill -o cam_module_pic.jpg
This will capture and save the image with name cam_module_pic.jpg in the current directory.
To capture a 20 second video with the camera module, run the following command:
raspivid –o test.avi –t 20000
This will capture and save the video with name test.avi in the current directory. Unlike fswebcam and avconv, raspistill and raspivid do not write anything to the console. So, you need to check the current directory for the output. Also, one can run the echo $? command to check whether these commands executed successfully. We can also mention the complete location of the file to be saved in these command, as shown in the following example:
raspistill -o /home/pi/book/output/cam_module_pic.jpg
Just like fswebcam, raspistill can be used to record the timelapse sequence. In our timelapse shell script, replace the line that contains fswebcam with the appropriate raspistill command to capture the timelapse sequence and use mencoder again to create the video. This is left as an exercise for the readers.
Now, let's take a look at the images taken with the Pi camera under different lighting conditions.
The following is the image with normal lighting and the backlight:
The following is the image with only the backlight:
The following is the image with normal lighting and no backlight:
For NoIR camera usage in the night under low light conditions, use IR illuminator light for better results. You can get it online. A typical off-the-shelf LED IR illuminator suitable for our purpose will look like the one shown here:
picamera is a Python package that provides a programming interface to the Pi Camera module. The most recent version of raspbian has picamera preinstalled. If you do not have it installed, you can install it using:
sudo apt-get install python-picamera
The following program quickly demonstrates the basic usage of the picamera module to capture an image:
import picamera
import time
with picamera.PiCamera() as cam:
cam.resolution=(1024,768)
cam.start_preview()
time.sleep(5)
cam.capture('/home/pi/book/output/still.jpg')
We have to import time and picamera modules first. cam.start_preview()will start the preview, and time.sleep(5) will wait for 5 seconds before cam.capture() captures and saves image in the specified file.
There is a built-in function in picamera for timelapse photography. The following program demonstrates its usage:
import picamera
import time
with picamera.PiCamera() as cam:
cam.resolution=(1024,768)
cam.start_preview()
time.sleep(3)
for count,
imagefile in enumerate(cam.capture_continuous
('/home/pi/book/output/image{counter:
02d}.jpg')):
print 'Capturing and saving ' + imagefile
time.sleep(1)
if count == 10:
break
In the preceding code, cam.capture_continuous()is used to capture the timelapse sequence using the Pi camera module.
Checkout more examples and API references for the picamera module at http://picamera.readthedocs.org/.
Now, after using the webcam and the Pi camera, it's a good time to understand the differences, the pros, and the cons of using these.
The Pi camera board does not use a USB port and is directly interfaced to the Pi. So, it provides better performance than a webcam in terms of the frame rate and resolution. We can directly use the picamera module in Python to work on images and videos. However, the Pi camera cannot be used with any other computer.
A webcam uses an USB port for interface, and because of that, it can be used with any computer. However, compared to the Pi camera its performance, it is lower in terms of the frame rate and resolution.
In this article, we learned how to use a webcam and the Pi camera. We also learned how to use utilities such as fswebcam, avconv, raspistill, raspivid, mencoder, and omxplayer. We covered how to use crontab. We used the Python picamera module to programmatically work with the Pi camera board. Finally, we compared the Pi camera and the webcam. We will be reusing all the code examples and concepts for some real-life projects soon.
Further resources on this subject: