










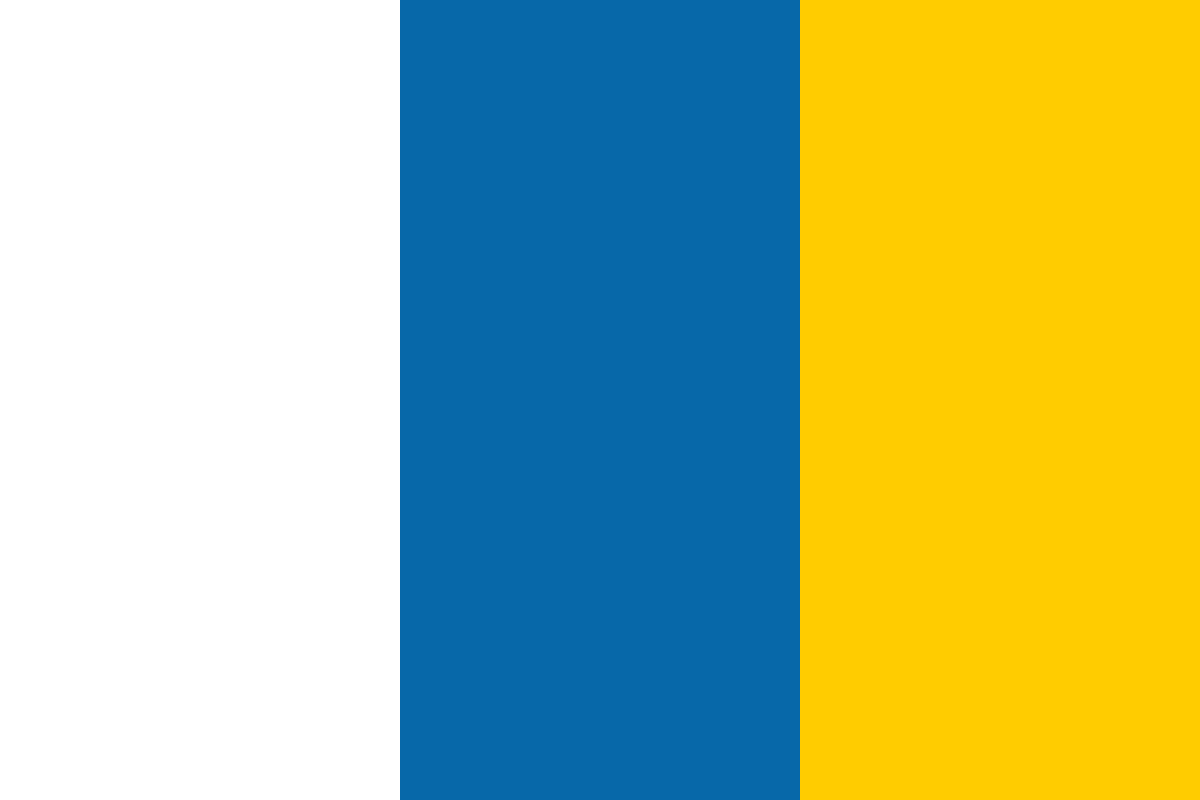

































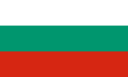








(For more resources on this subject, see here.)
Considering workflow programs as imperative program, we need to think of three fundamental things:
In WF4, we can define a workflow in either managed .NET code or in XAML. There are two kinds of code workflow authoring styles:
There are also two ways to author workflow in XAML:
Essentially, workflow program is .NET program, no matter how we create it.
After defining workflows, we can build workflow applications as we build normal .NET applications.
When it comes to workflow execution, we need to consider three basic things:
This article is going to focus on answering these questions.
Before moving ahead, make sure we have the following installed on our computer:
We can also use Windows XP; however, its usage is not recommended.
In this task we will create our first workflow to print "Hello Workflow" to console application.
When we press Ctrl+F5, Visual Studio saves the current project, and then it runs the project from Main method in Program.cs file.
WorkflowInvoker.Invoke(new Workflow1());
The preceding statement starts the workflow. After the workflow starts running, WriteLine activity prints the "Hello Workflow" to Console Application.
The workflow we created in WF designer is actually an XML file. We can open Workflow1.xaml with XML editor to check it.
Right-click on Workflow1.xaml then click Open With..., and choose XML Editor to open the Workflow1.xaml as XML file.
All XAML files will be compiled to .dll or .exe file. That is why when we press Ctrl+F5, the program just runs like a normal C# program.
So far, there are no officially published WF4 Designer add-ins for Visual Studio 2008. We need a copy of Visual Studio 2010 installed on our computer to use WF4 designer, otherwise we can only create workflows by imperative code or by writing pure XAML file.
In this task, we will create the same "HelloWorkflow" function workflow using pure C# code, beginning from a Console Application.
Open Program.cs file and change the code present as follows:
using System.Activities;
using System.Activities.Statements;
namespace HelloCodeWorkflow {
class Program {
static void Main(string[] args) {
WorkflowInvoker.Invoke(new HelloWorkflow());
}
}
public class HelloWorkflow:Activity {
public HelloWorkflow() {
this.Implementation = () => new Sequence {
Activities = {
new WriteLine(){Text="Hellow Workflow"}
}
};
}
}
}
We use the following namespace:
using System.Activities;
using System.Activities.Statements;
Because WorflowInvoker class belongs to System.Activities namespace and Sequence activity, WriteLine activity belongs to System.Activities.Statements.
public class HelloWorkflow:Activity {
public HelloWorkflow() {
this.Implementation = () => new Sequence {
Activities = {
new WriteLine(){Text="Hellow Workflow"}
}
};
}
}
By implementing a class inherited from Activity, we define a workflow using imperative code.
WorkflowInvoker.Invoke(s);
This code statement loads a workflow instance up and runs it automatically. WorkflowInvoker.Invoke method is synchronous and invokes the workflow on the same thread as the caller.
WF4 also provide us a class DynamicActivity by which we can create a workflow instance dynamically in the runtime. In other words, by using DynamicActivity, there is no need to define a workflow class before initializing a workflow instance. Here is a sample code:
public static DynamicActivity GetWF() {
return new DynamicActivity() {
Implementation = () => new Sequence() {
Activities ={
new WriteLine(){Text="Hello Workflow"}
}
}
};
}