










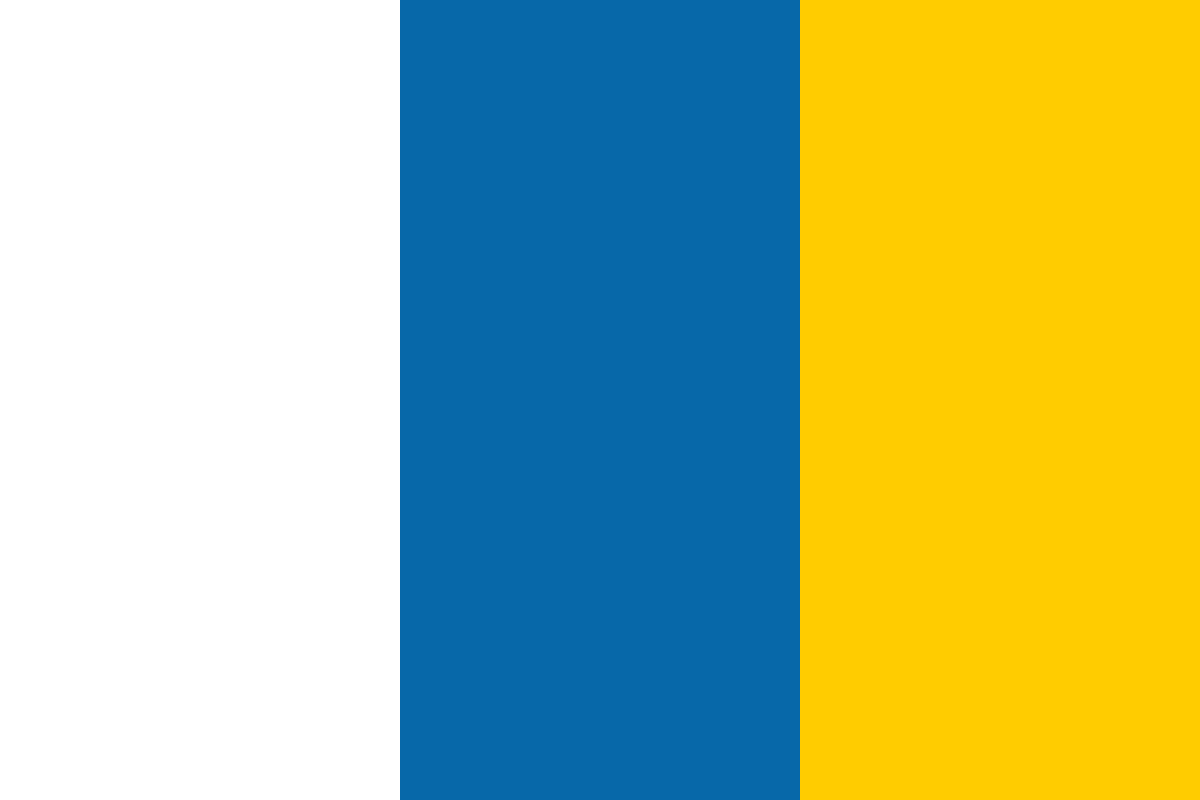

































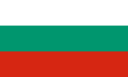








When you are done with this article, you should be able to use .NET classes as reference classes in AX through the Common Language Runtime (CLR). The article will also guide you through the process of creating a .NET class in Visual Studio, and how to use it in AX. You will also learn how to use AX logic from external applications by using the .NET Business Connector.
All the Visual Studio examples in this article are written in C#.
You might have done some .NET development before looking into X++ right? Maybe you're even a .NET expert? If so, you must have heard of CLR before. The CLR is a component of .NET that enables objects written in different languages to communicate with each other. CLR can be used in AX to combine functionality from .NET classes and libraries, including the ones you have yourself created in .NET. However, you cannot consume AX objects in .NET by using the CLR. Instead, you will then have to use the .NET Business Connector.
To learn more about the CLR, check out the following link: http://msdn.microsoft.com/en-us/library/ddk909ch(VS.71).aspx
One very useful feature in AX when dealing with integration between AX and .NET is the way AX implicitly converts the most common data types. For the data types listed in the next table you do not need to convert the data manually. For all other data types, you will have to convert them manually.
.NET Common Language Runtime |
X++ |
System.String |
str |
System.Int32 |
int |
System.Int64 |
int64 |
System.Single |
real |
System.Double |
real |
System.Boolean |
Boolean |
System.DateTime |
date |
System.Guid |
guid |
Enums are stored as integers in AX and are treated as integers when they are implicitly converted between .NET and AX.
We prove this by executing the next example that shows the conversion between System.String and str. The same can be done for any of the other data types in the above table.
static void ImplicitDataConversion(Args _args)
{
System.String netString;
str xppString;
;
// Converting from System.String to str
netString = "Hello AX!";
xppString = netString;
info(xppString);
// Converting from str to System.String
xppString = "Hello .NET!";
netString = xppString;
info(netString);
}
X++ is case insensitive, except when dealing with CLR. This means that writing System.string in the previous example will result in a compile error, whereas writing Str instead of str will not.
The result will look like this:
To be able to use .NET classes in AX you have to make sure that the .NET assembly that you would like to use in AX exists under the References node in the AOT. If you can't find it there, you have to add it by adding a reference to the DLL file that contains the assembly in the AOT under References.
When adding a reference node in the AOT you have to make sure that the DLL exists on all client computers. If there is a client computer in which the DLL does not exist, it will result in compile errors when compiling code on that client computer.
Follow these steps to add a reference that exists in the Global Assembly Cache:
If the file does not exist in the Global Assembly Cache follow these steps:
Another option is to add the DLL to the Global Assembly Cache first and select it as described in the previous section, Assembly exist in the Global Assembly Cache.