










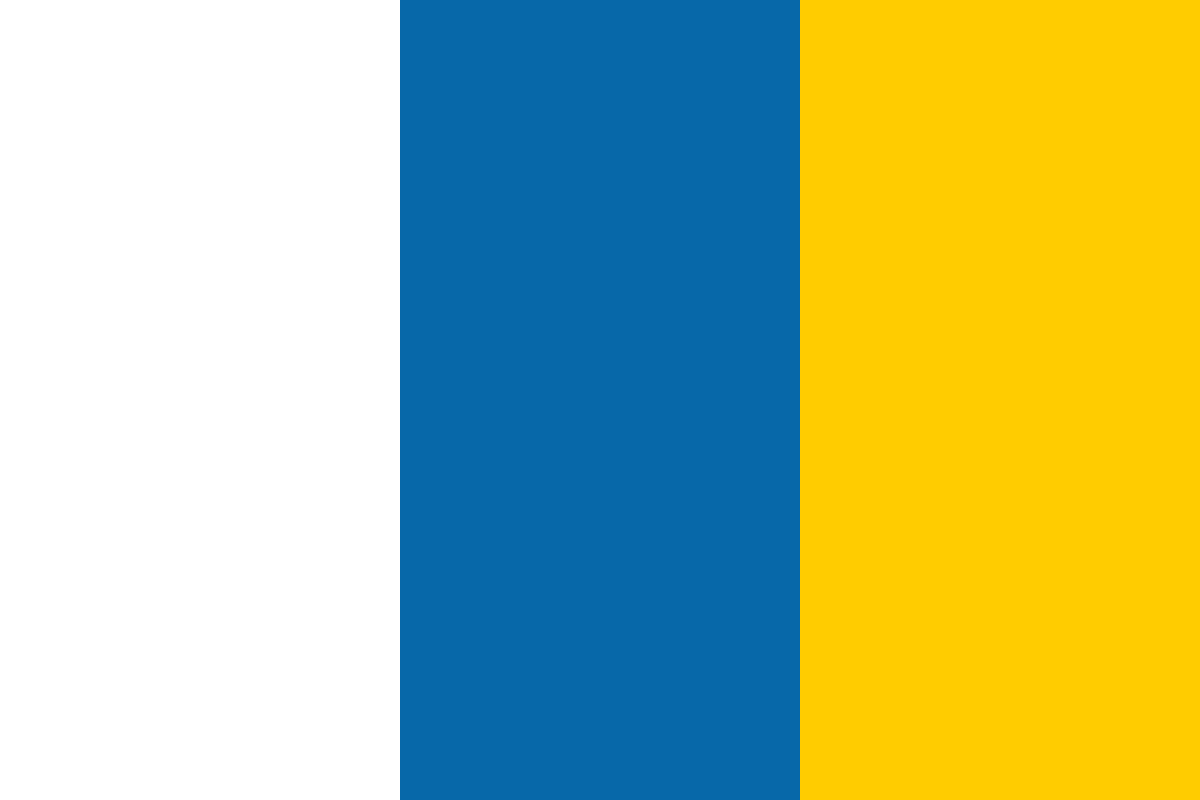

































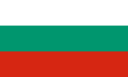








Besides adding event listeners, other operations you may want to do are removing events from an element, cloning events from other elements, and firing off events for elements manually. We'll go through each one of these operations.
There are instances when you want to remove an event listener that you've added to an element. One reason would be that you only want to an element to be triggered once, and after that event has been triggered, you no longer want to trigger it again. To ensure it only fires once, you should remove the event once certain conditions have been met.
There are two methods available to you for removing events from elements. The first is removeEvent() which removes a single specified event.
Let's say you have some hyperlinks on a page, that when clicked, will alert the user that they have clicked a hyperlink, but you only wanted to do it once. To ensure that the warning message appears only once, we'll have to remove the event after it has been fired.
This type of thing may be utilized for instructional tips: when the user sees an unfamiliar interface element, you can display a help tip for them, but only once - because you don't want the tip to keep showing up every single time they perform an action.
<a href="#">Hyperlink 1</a> <a href="#">Hyperlink 2</a>
// Create an function object
var warning = function() {
alert('You have clicked a link. This is your only warning');
// After warning has executed, remove it
$$('a').removeEvent('click', warning);
};
// Add a click event listener which when triggered, executes the
//warning function
$$('a').addEvent('click', warning);
window.addEvent('domready', function(){
// Create an function object that will be executed when a
//click happens
var warning = function() {
alert('You have clicked a link. This is your only warning');
// After warning has executed, remove it from all <a>
//elements on the web page
$$('a').removeEvent('click', warning);
};
// Add a click event listener which when triggered, executes the
//warning function
$$('a').addEvent('click', warning);
});
If you want to remove a type of event on an element (or set of elements), or if you want to remove all events regardless of its type from an element, you have to use the removeEvents method.
To remove a type of event from an element, you pass the type of event you want to remove as a parameter of the removeEvents method. For example, if you wanted to remove all click events that were added using MooTools addEvent method from an element called myElement, you would do the following:
$('myElement').removeEvents('click');
If instead, you wanted to remove all events that myElement has regardless of the type of event it has, then you would simply run removeEvents as follows:
$('myElement').removeEvents();
What if you wanted to copy all event listeners from another element. This could be useful in situations where you clone an element using the clone MooTools element method. Cloning an element doesn't copy the event listeners attached to it, so you also have to run the cloneEvents method on the element being cloned if you wanted to also port the event listeners to the copy.
To clone the events of an element, follow the format:
// clone the element
var original = $(‘originalElement’);
var myClone = original.clone();
// clone the events from the original
myClone.cloneEvents(original);
Sometimes you want to fire off events manually. This is helpful in many situations, such as manually firing off an event listener functions that is triggered by another event. For example, to fire off a click event on myElement without having the user actually clicking on myElement, you would do the following:
$('myElement').fireEvent('click');
Imagine that you have a hyperlink with a click event listener attached to it, that when triggered, alerts the user with a message. But you also want to fire off this alert message when the user presses the Ctrl key. Here's how you'd do this:
<body>
<p>Show a warning by clicking on this link: <a href="#">Click
me</a>. Alternatively, you can show the warning by pressing the
<strong>Ctrl</strong> key on your keyboard.</p>
</body>
// Add a click event
$$('a').addEvent('click', function(){
alert('You either clicked a link or pressed the Ctrl key.');
});
If the key that was pressed is the Ctrl key, we ask it to fire the click event function that we set in all our a elements by using the fireEvent method with click as its parameter.
// Add a keydown event on our web page
window.addEvent('keydown', function(e){
// If the keypress is the Ctrl key
// manually fire off the click event
if(e.control) {
$$('a').fireEvent('click');
}
});
window.addEvent('domready', function(){
// Add a click event
$$('a').addEvent('click', function(){
alert('You either clicked a link or pressed the Ctrl key.');
});
// Add a keydown event on our web page
window.addEvent('keydown', function(e){
// If the keypress is the Ctrl key
// manually fire off the click event
if(e.control) {
$$('a').fireEvent('click');
}
});
});
The MooTools Event object, which is part of the Native component, is what allows us to create and work with events. It's therefore worth it to take a bit of time to explore the Events object.
There are three Event methods: preventDefault, stopPropagation, stop.
Events usually has a default behavior; that is, it has a predefined reaction in the instance that the event is triggered. For example, clicking on a hyperlink will direct you to the URL that href property is assigned to. Clicking on a submit input field will submit the form to the value that the action property of the form element is assigned to.
Perhaps you want to open the page in a new window, but instead of using the non-standard target property on an <a> element, you can use JavaScript to open the page in a new window. Or maybe you need to validate a form before submitting it. You will want to prevent the default behaviors of an event doing either one of these things. You can use the preventDefault method to do so.
Imagine that you have a list of hyperlinks that go to popular sites. The thing is, you don't want your website visitors to ever get to see them (at least coming from your site). You can prevent the default behavior of your hyperlinks using the preventDefault method.
<h1>A list of links you can't go to.</h1>
<ul>
<li><a href="http://www.google.com/">Google</a></li>
<li><a href="http://www.yahoo.com/">Yahoo!</a></li>
<li><a href="http://digg.com/">Digg</a></li>
</ul>
$$('a').addEvent('click', function(e){
alert('Sorry you can't go there. At least not from this page.');
});
e.preventDefault();
Event bubbling occurs when you have an element inside another element. When an event is triggered from the child element, the same event is triggered for the parent element, with the child element taking precedence by being triggered first.
You can prevent event bubbling using the stopPropagation method. Let's explore the concept of event bubbling and how to prevent it from occurring (if you want to), using the stopPropagation event method.