










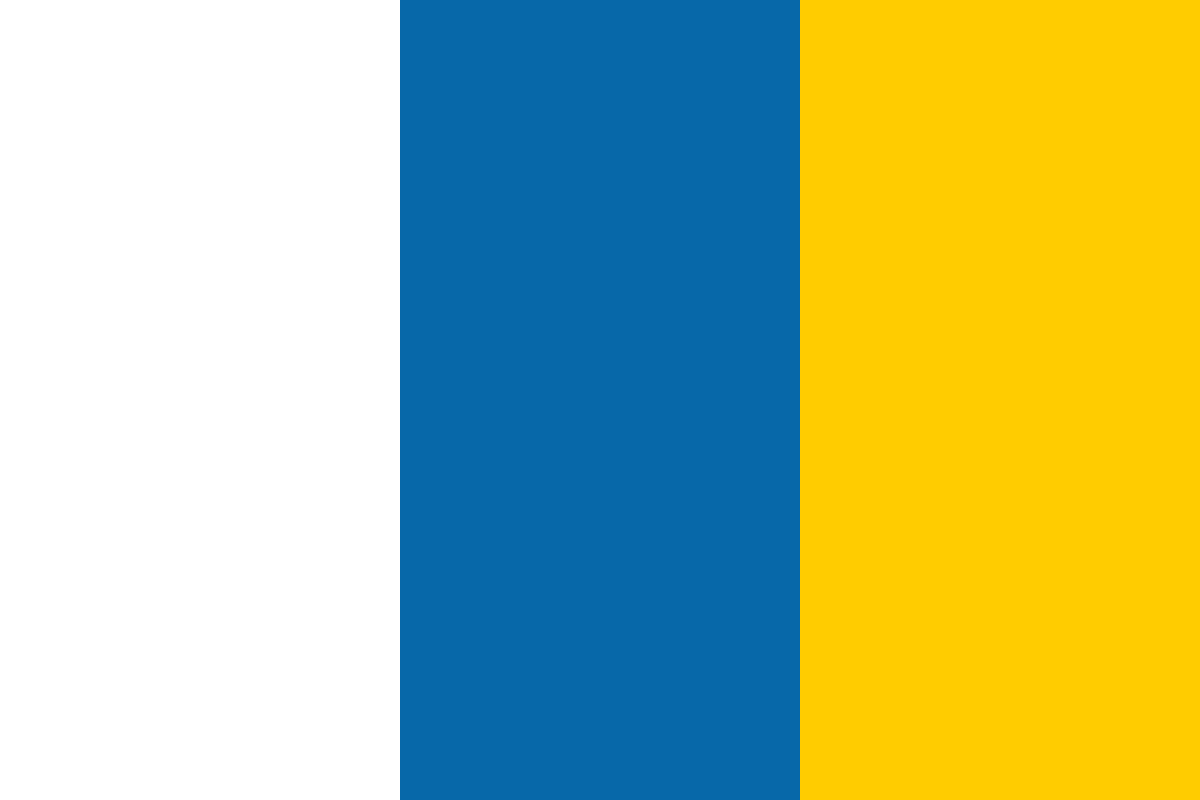

































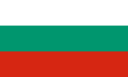








(For more resources on WordPress, see here.)
At its most basic, a simple implementation of the loop could work like this:
<?php if (have_posts()) : while (have_posts()) : the_post(); ?>
<?php the_title(); ?>
<?php the_content(); ?>
<?php endwhile; else: ?>
<p>Sorry, no posts matched your criteria.'</p>
<?php endif; ?>
In the real world, however, the WordPress loop is rarely that simple. This is one of those concepts best explained by referring to a real world example, so open up the index.php file of your system's TwentyEleven theme. Look for the following lines of code:
<?php if ( have_posts() ) : ?>
<?php twentyeleven_content_nav( 'nav-above' ); ?>
<?php /* Start the Loop */ ?>
<?php while ( have_posts() ) : the_post(); ?>
<?php get_template_part( 'content', get_post_format()
); ?>
<?php endwhile; ?>
<?php twentyeleven_content_nav( 'nav-below' ); ?>
<?php else : ?>
<article id="post-0" class="post no-results not-found">
<header class="entry-header">
<h1 class="entry-title"><?php _e( 'Nothing Found',
'twentyeleven' ); ?></h1>
</header><!-- .entry-header -->
<div class="entry-content">
3
<p><?php _e( 'Apologies, but no results were found
for the requested archive. Perhaps searching will help
find a related post.', 'twentyeleven' ); ?></p>
<?php get_search_form(); ?>
</div><!-- .entry-content -->
</article><!-- #post-0 -->
<?php endif; ?>
Most of the extra stuff seen in the loop from TwentyEleven is there to add in additional page elements, including content navigation; there's also some code to control what happens if there are no posts to display. The nature of the WordPress loops means that theme authors can add in what they want to display and thereby customize and control the output of their site.
As you would expect, the WordPress Codex includes an extensive discussion of the WordPress loop. Visit http://codex.wordpress.org/The_Loop.
In this recipe, we look at how to create a custom template that includes your own implementation of the WordPress loop.
All you need to execute this recipe is your favorite code editor and access to the WordPress files on your server. You will also need a theme template file, which we will use to hold our modified WordPress loop.
Let's assume you have created a custom template. Inside of that template you will want to include the WordPress loop. Follow these steps to add the loop, along with a little customization:
<?php if ( have_posts() ) : while ( have_posts() ) : the_post();
?>
<h2><?php the_title() ?></h2>
<?php the_author_posts_link() ?>
<div class="thecontent">
<?php the_content(); ?>
</div>
<?php endwhile; else: ?>
<p>Oops! There are no posts to display.</p>
<?php endif; ?>
That's all there is to it. Your code should look like this:
?php if ( have_posts() ) : while ( have_posts() ) : the_post(); ?>
<h2><?php the_title() ?></h2>
<?php the_author_posts_link() ?>
<div class="thecontent">
<?php the_content(); ?>
</div>
<?php endwhile; else: ?>
<p>Oops! There are no posts to display.</p>
<?php endif; ?>
This basic piece of code first checks if there are posts in your site. If the answer is yes, the loop will repeat until every post title and their contents are displayed on the page. The post title is displayed using the_title(). The author's name and link are added with the_ author_posts_link() function. The content is displayed with the_content() function and styled by the div named thecontent. Finally, if there are no posts to display, the code will display the message Oops! There are no posts to display.
In the preceding code you saw the use of two template tags: the_author_posts_link and the_content. These are just two examples of the many template tags available in WordPress. The tags make your life easier by reducing an entire function to just a short phrase. You can find a full list of the template tags at: http://codex.wordpress.org/ Template_Tags.
The template tags can be broken down into a number of categories:
There are times when you might wish to display only those posts that belong to a specific category, for example, perhaps you want to show only the featured posts. With a small modification to the WordPress loop, it's easy to grab only those posts you want to display.
In this recipe we introduce query_posts(),which can be used to control which posts are displayed by the loop.
To execute this recipe, you will need a code editor and access to the WordPress files on your server. You will also need a theme template file, which we will use to hold our modified WordPress loop. To keep this recipe short and to the point, we use the loop we created in the preceding recipe.
Let's create a situation where the loop shows only those posts assigned to the Featured category. To do this, you will need to work through two different processes.
First, you need to find the category ID number of the Featured category. To do this, follow these steps:
Category IDs vary from site to site. The ID used in this recipe may not be the same as the ID for your site!
Next, we need to add a query to our loop that will extract only those posts that belong to the Featured category, that is, to those posts that belong to the category with the ID of 9. Follow these steps:
<?php if ( have_posts() ) : while ( have_posts() ) :
the_post(); ?>
<h2><?php the_title() ?></h2>
<?php the_author_posts_link() ?>
<div class="thecontent">
<?php the_content(); ?>
</div>
<?php endwhile; else: ?>
<p>Oops! There are no posts to display.</p>
<?php endif; ?>
<?php query_posts($query_string.'&cat=9'); ?>
That's all there is to it. If you visit your site, you will now see that the page displays only the posts that belong to the category with the ID of 9.
The query_posts() function modifies the default loop. When used with the cat parameter, it allows you to specify one or more categories that you want to use as filters for the posts.
For example:
For more information, visit the WordPRess Codex page on query posts: http://codex.wordpress.org/Function_Reference/query_posts