










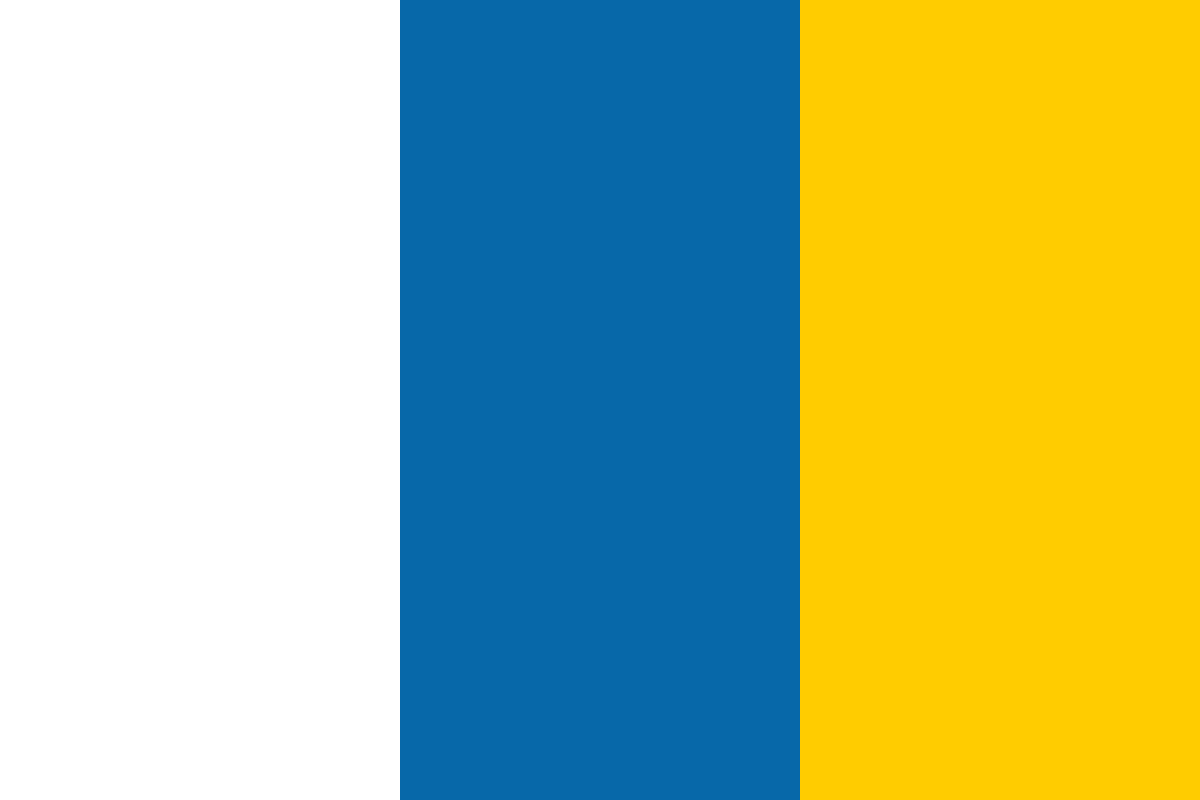

































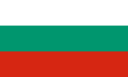








(For more resources related to this topic, see here.)
A column bar chart widget is slightly different from the regular bar chart widget we previously created. Remember we also had a look at some of the useful and common options used by most Wijmo developers. The last one we listed was the horizontal option of type Boolean. The default value of the horizontal option is true. This implies that the bar chart will be rendered in a horizontal layout by default.
For the creation of our first column bar chart, we shall proceed by setting the horizontal option to false, using the same code used for the previous bar chart we had created. Here's our new complete code:
<html>
<head>
<!--jQuery References-->
<script src = "http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.7.1.min.
js" type="text/javascript"></script>
<script src = "http://ajax.aspnetcdn.com/ajax/jquery.ui/1.8.17/jqueryui.
min.js" type="text/javascript"></script>
<!--Wijmo Widgets JavaScript-->
<script src = "http://cdn.wijmo.com/jquery.wijmo-open.all.2.0.0.min.js"
type="text/javascript"></script>
<script src = "http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.
js" type="text/javascript"></script>
<!--Theme-->
<link href = "http://cdn.wijmo.com/themes/rocket/jquery-wijmo.css"
rel="stylesheet" type="text/css" title="rocket-jqueryui" />
<!--Wijmo Widgets CSS-->
<link href = "http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.
css" rel="stylesheet" type="text/css" />
</head>
<body>
<div id="wijbarchart" class="ui-widget ui-widget-content ui-cornerall"
style="width: 400px;
height: 300px">
</div>
<script id="scriptInit" type="text/javascript">
$(document).ready(function () {
//activating the wijbarchart function on #wijbarchart
$("#wijbarchart").wijbarchart({
horizontal: false,//makes it vertical
axis: { //set up the x and y axes
y: {
text: "Total Automation Sales",
},
x: {
text: "",
labels: {
style: {
rotation: -45
}
}
}
},
hint: { //hint text when hovering over chart
content: function () {
return this.data.label + 'n ' + this.y + '';
}
},
header: {//chart title
text: "US Toyota Automobile Statistics (Dummy
Data)"
},
//data for chart representing each column
seriesList: [{
label: "US",
legendEntry: true,
data: { x: ['Toyota Camry', 'Toyota Corolla',
'Toyota Sienna'], y: [12.35, 21.50, 30.56] }
}],
seriesStyles: [{
fill: "40-#BD0070-#718680", stroke: "#1261C0",
opacity: 0.7
}],
seriesHoverStyles: [{ "stroke-width": "1.5", opacity:
1
}]
});
});
</script>
</body>
</html>
Notice from the preceding code, which we will hereon refer to as the main code, that we set the horizontal option to false. Now, when we run the main code, we should see a column bar chart widget as follows:
That's how simple switching from a regular bar chart to a column bar chart is. The choice between either of the two bar charts is usually dependent on user preference.
We reference the Wijmo dependencies as follows:
After the page loads, we set the horizontal property to false.
The x and y properties are set, and y is rotated at -45 degrees, which rotates the labels for Toyota Camry, Toyota Corolla, and Toyota Sienna.
We set the hint property, which is displayed on hovering over the chart.
We set the header property, which is displayed atop the grid.
For the seriesList property, we have a data subproperty that holds data for x and y. These values are mapped one-to-one in such a way that x['Toyota Camry'] gets y[0] or y[12.35].
For the seriesStyles property, we set the gradient color as "40-#BD0070- #718680" and set the opacity value as 0.7.
A bubble chart is a chart whose data points are replaced with bubbles of various shapes and scattered across the chart. It is like a scatter chart. The Wijmo widget representing a bubble chart is referred to as a wijbubblechart object.
The data points or bubbles each have three non-dependent values, x, y, and y1 as follows:
The value x defines the Cartesian position along the x axis
The value y defines the Cartesian position along the y axis
The value y1 defines the bubble size at each point
Having understood a bubble chart and the three values that define the positions of the bubble, we can now happily proceed with an implementation. Let us create a wijbubblechart object of the percentage of college graduates in six major cities around the world. This is dummy data and doesn't reflect the actual relationship between college graduates and the health of the corresponding economy. However, this dummy data is based on the assumption that a city with more graduates per thousand will have a smarter economy. Also, this depends on the overall population of that city.
Enter the following code into your favorite code editor:
<html>
<head>
<!--jQuery References-->
<script src = "http://ajax.aspnetcdn.com/ajax/jquery/jquery-1.7.1.min.
js" type="text/javascript"></script>
<script src = "http://ajax.aspnetcdn.com/ajax/jquery.ui/1.8.17/jqueryui.
min.js" type="text/javascript"></script>
<!--Wijmo Widgets JavaScript-->
<script src = "http://cdn.wijmo.com/jquery.wijmo-open.all.2.0.0.min.js"
type="text/javascript"></script>
<script src = "http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.
js" type="text/javascript"></script>
<!--Theme-->
<link href = "http://cdn.wijmo.com/themes/rocket/jquery-wijmo.css"
rel="stylesheet" type="text/css" title="rocket-jqueryui" />
<!--Wijmo Widgets CSS-->
<link href = "http://cdn.wijmo.com/jquery.wijmo-complete.all.2.0.0.min.
css" rel="stylesheet" type="text/css" />
<script type = "text/javascript">
$(document).ready(function () {
$("#myWijbubblechart").wijbubblechart({
showChartLabels: false,
axis: {
y: {
text: "Smart Economy Rating"
},
x: {
text: "College Graduates(Per Thousand)"
}
},
hint: {
content: function () {
return this.data.label;
}
},
header: {
text: "College Graduates Vs. Health of the Economy
- 2012"
},
seriesList: [{
label: "Beijing",
legendEntry: true,
data: { y: [85], x: [150], y1: [1340] },
markers: {
type: "tri"
}
}, {
label: "New Delhi",
legendEntry: true,
data: { y: [80], x: [167], y1: [1150] },
markers: {
type: "diamond"
}
}, {
label: "Los Angeles",
legendEntry: true,
data: { y: [92], x: [400], y1: [309] },
markers: {
type: "circle"
}
}, {
label: "Tokyo",
legendEntry: true,
data: { y: [94], x: [250], y1: [126] },
markers: {
type: "invertedTri"
}
}, {
label: "London",
legendEntry: true,
data: { y: [82], x: [200], y1: [140] },
markers: {
type: "cross"
}
}, {
label: "Lagos",
legendEntry: true,
data: { y: [48], x: [374], y1: [72] },
markers: {
type: "box"
}
}]
});
});
</script>
</head>
<body>
<div id="myWijbubblechart" class="ui-widget ui-widget-content uicorner-
all" style="width: 500px;
height: 300px">
</div>
</body>
</html>
If the preceding code is copied correctly and run in a browser, we should have a Wijmo bubble chart similar to the following screenshot:
To see how the wijbubblechart object works, we can simply examine one of the objects in the seriesList property, like this one:
{
label: "Beijing",
legendEntry: true,
data: { y: [85], x: [150], y1: [1340] },
markers: {
type: "tri"
}
},
Remember that we already defined x, y, and y1 as the values of a point on the x axis, a point on the y axis, and the size of the bubble respectively. So in this case, for Beijing the value for y is set to 85, and the size of the bubble, y1, is 1340. The legendEntry property is set to true so we can see Beijing in the legend area by the right of the chart.
We can see the various bubbles in different shapes and a legend that tells what city each bubble represents. The type property of the markers object is responsible for setting the shape of the bubble.
markers: {
type: "tri"
}
One more common aspect of the wijbubblechart object is the possibility of changing its appearance, for example, applying gradient colors, using some options, and so on. To achieve this we simply have to include a seriesStyles property as follows:
<script type="text/javascript">
$(document).ready(function () {
//instantiating wijbubblechart on #myWijbubblechart
$("#myWijbubblechart").wijbubblechart({
showChartLabels: false,
//setup the x and y axis labels
axis: {
y: {
text: "Smart Economy Rating"
},
x: {
text: "College Graduates(Per Thousand)"
}
},
//Display hint text on chart hover
hint: {
content: function () {
return this.data.label;
}
},
//title of chart
header: {
text: "College Graduates Vs. Health of the Economy
- 2012"
},
//chart color fill styles
seriesStyles: [{
fill: "180-#8F8F8F-#C462AC", stroke: "#8F8F8F"
}, {
fill: "45-#C462AC-#2371B0", stroke: "#C462AC"
}, {
fill: "90-#4A067D-#0B7D19", stroke: "#4A067D"
}, {
fill: "270-#2371B0-#6AABA7", stroke: "#2371B0"
}, {
fill: "45-#0C85F0-#852E85", stroke: "#0C85F0"
}, {
fill: "180-#6AABA7-#AB6A9C", stroke: "#6AABA7"
}],
//data values for each bubble
seriesList: [{
label: "Beijing",
legendEntry: true,
data: { y: [85], x: [150], y1: [1340] },
markers: {
type: "tri"
}
}, {
label: "New Delhi",
legendEntry: true,
data: { y: [80], x: [167], y1: [1150] },
markers: {
type: "diamond"
}
}, {
label: "Los Angeles",
legendEntry: true,
data: { y: [92], x: [400], y1: [309] },
markers: {
type: "circle"
}
}, {
label: "Tokyo",
legendEntry: true,
data: { y: [94], x: [250], y1: [126] },
markers: {
type: "invertedTri"
}
}, {
label: "London",
legendEntry: true,
data: { y: [82], x: [200], y1: [140] },
markers: {
type: "cross"
}
}, {
label: "Lagos",
legendEntry: true,
data: { y: [48], x: [374], y1: [72] },
markers: {
type: "box"
}
}]
});
});
</script>
The script tags should be embedded within the HTML tags for a successful run.
If we successfully run the preceding code, we should have a bubble chart that looks like this:
You can visit http://wijmo.com/wiki/index. php/Bubblechart for more details about Wijmo bubble charts and more advanced options available for customizing your charts.
In this Article, we have discussed the Column Bar Chart and Bubble Chart wijmos. Column bar chart (Simple) explains how to convert a regular Wijmo bar chart to a column bar chart widget. Bubble chart (Intermediate) shows how to create and customize a bubble chart widget
Further resources on this subject: