










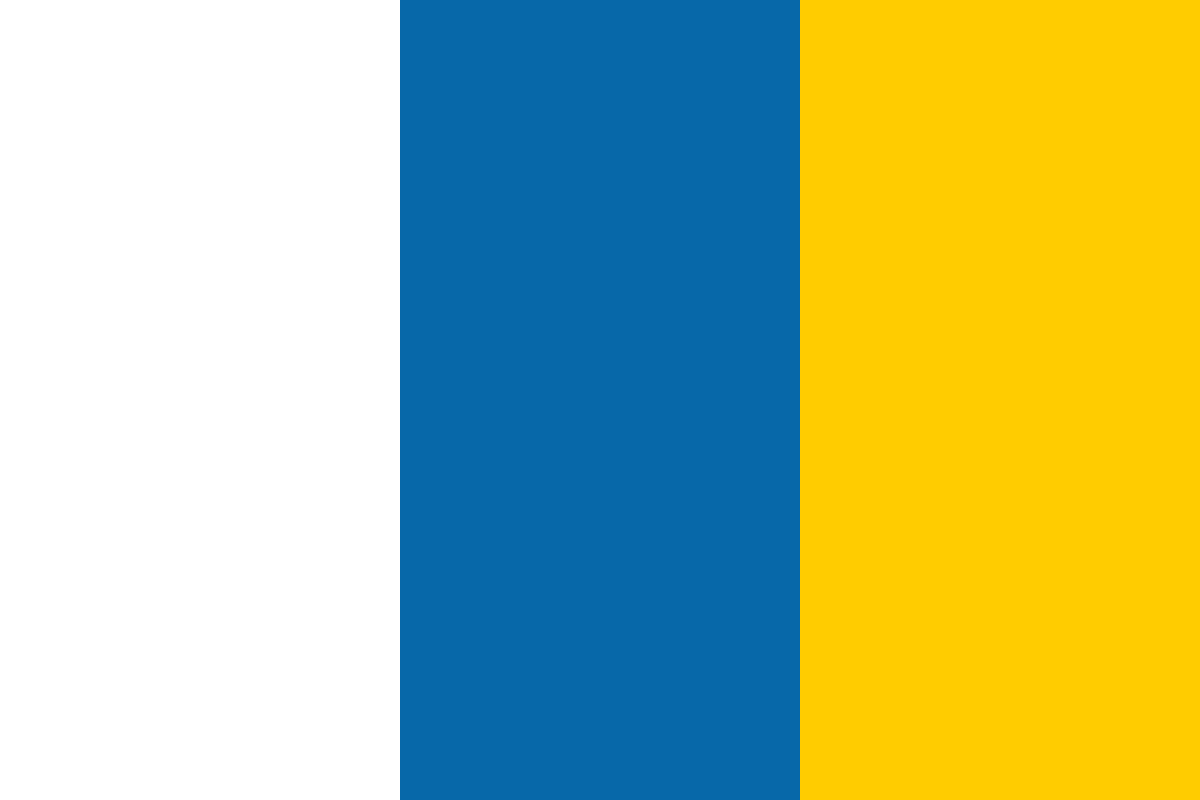

































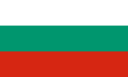








(For more resources on this topic, see here.)
The reader can benefit from the previous article on Sencha Touch: Layouts Revisited.
In our last application, we used buttons and a card layout to create an application that switched between different child items. While it is often desirable for your application to do this programmatically (with your own buttons and code), you can also choose to have Sencha Touch set this up automatically, using TabPanel or Carousel.
TabPanel is useful when you have a number of views the user needs to switch between, such as, contacts, tasks, and settings. The TabPanel component autogenerates the navigation for the layout, which makes it very useful as the main container for an application.
The following is a code example:
new Ext.Application({
name: 'TouchStart',
launch: function() {
this.viewport = new Ext.TabPanel({
fullscreen: true,
cardSwitchAnimation: 'slide',
tabBar:{
dock: 'bottom',
layout: {
pack: 'center'
}
},
items: [{
xtype: 'container',
title: 'Item 1',
fullscreen: false,
html: 'TouchStart container 1',
iconCls: 'info'
}, {
xtype: 'container',
html: 'TouchStart container 2',
iconCls: 'home',
title: 'Item 2'
}, {
xtype: 'container',
html: 'TouchStart container 3',
iconCls: 'favorites',
title: 'Item 3'
}]
});
}
});
TabPanel, in this code, automatically generates a card layout; you don't have to declare a layout. You do need to declare a configuration for the tabBar component. This is where your tabs will automatically appear.
In our previous code example, we dock the toolbar at the bottom. This will generate a large square button for each child item in the items list. The button will also use the iconCls value to assign an icon to the button. The title configuration is used to name the button.
If you dock the tabBar component at the top, it makes the buttons small and round. It also eliminates the icons, even if you declare a value for iconCls, in your child items. Only the title configuration is used when the bar is docked at the top.
The Carousel component is similar to TabPanel, but the navigation it generates is more appropriate for things such as slide shows. It probably would not work as well as a main interface for your application, but it does work well as a way to display multiple items in a single swipeable container.
Similar to TabPanel, Carousel gathers its child items and automatically arranges them in a card layout. In fact, we can actually make just some simple modifications to our previous code to make it into a Carousel:
new Ext.Application({
name: 'TouchStart',
launch: function() {
this.viewport = new Ext.Carousel({
fullscreen: true,
direction: 'horizontal',
items: [{
html: 'TouchStart container 1'
}, {
html: 'TouchStart container 2'
}, {
html: 'TouchStart container 3'
}]
});
}
});
The first thing we did was create a new Ext.Carousel class instead of a new Ext.TabPanel class. We also added a configuration for direction, which can be either horizontal (scrolling from left to right) or vertical (scrolling up or down).
We removed the docked toolbar, because, as we will see, Carousel doesn't use one. We also removed iconClass and title from each of our child items for the same reason. Finally, we removed the xtype configuration, since the Carousel automatically creates a panel for each of its items.
Unlike TabPanel, Carousel has no buttons, only a series of dots at the bottom, with one dot for each child item. While it is possible to navigate using the dots, the Carousel component automatically sets itself up to respond to a swipe on a touch screen. You can duplicate this gesture in the browser by clicking and holding with the mouse, while moving it horizontally. If you declare a direction: vertical configuration in your Carousel, you can swipe vertically, to move between the child items.
TabPanel and the Carousel components understand the activeItem configuration. This lets you set which item appears when the application first loads. Additionally, they all understand the setActiveItem() method that allows you to change the selected child item after the application loads.
Carousel also has methods for next() and previous(), which allow you to step through the items in order.
It should also be noted that, since TabPanel and Carousel both inherit from the panel, they also understand any methods and configurations that panels and containers understand.
Along with containers and panels, TabPanel and Carousel will serve as the main starting point for most of your applications. However, there is another type of panel you will likely want to use at some point: the FormPanel.
The FormPanel panel is a very specialized version of the panel, and as the name implies, it is designed to handle form elements. Unlike panels and containers, you don't need to specify the layout for FormPanel. It automatically uses its own special form layout.
A basic example of creating a FormPanel would look something like this:
var form = new Ext.form.FormPanel({
items: [
{
xtype: 'textfield',
name : 'first',
label: 'First name'
},
{
xtype: 'textfield',
name : 'last',
label: 'Last name'
},
{
xtype: 'emailfield',
name : 'email',
label: 'Email'
}
]
});
For this example, we just create the panel and add items for each field in the form. Our xtype tells the form what type of field to create. We can add this to our Carousel and replace our first container, as follows:
this.viewport = new Ext.Carousel({
fullscreen: true,
direction: 'horizontal',
items: [form, {
layout: 'fit',
html: 'TouchStart container 2'
}, {
layout: 'fit',
html: 'TouchStart container 3'
}]
});
Anyone who has worked with forms in HTML should be familiar with all of the standard field types, so the following xtype attribute names will make sense to anyone who is used to standard HTML forms:
These field types all match their HTML cousins, for the most part. Sencha Touch also offers a few specialized text fields that can assist with validating the user's input:
These special fields will only submit if the input is valid.
All of these basic form fields inherit from the main container class, so they have all of the standard height, width, cls, style, and other container configuration options.
They also have a few field-specific options:
Form field placement
While FormPanel is typically the container you will use when displaying form elements, you can also place them in any panel or toolbar, if desired. FormPanel has the advantage of understanding the submit() method that will post the form values via AJAX request or POST.
If you include a form field in something that is not a FormPanel, you will need to get and set the values for the field using your own custom JavaScript method.
In addition to the standard HTML fields, there are a few specialty fields available in Sencha Touch. These include the datepicker, slider, spinner, and toggle fields.
datepickerfield places a clickable field in the form with a small triangle on the far right side.
You can add a date picker to our form by adding the following code after the emailfield item:
,{
xtype: 'datepickerfield',
name : 'date',
label: 'Date'
}
When the user clicks the field, a DatePicker will appear, allowing the user to select a date by rotating the month, day, and year wheels, by swiping up or down.
Sliders allow for the selection of a single value from a specified numerical range. The sliderfield value displays a bar, with an indicator, that can be slid horizontally to select a value. This can be useful for setting volume, color values, and other ranged options.
Like the slider, a spinner allows for the selection of a single value from a specified numerical range. The spinnerfield value displays a form field with a numerical value with + and - buttons on either side of the field.
A toggle allows for a simple selection between one and zero (on and off) and displays a toggle-style button on the form.
Add the following new components to the end of our list of items:
,{
xtype : 'sliderfield',
label : 'Volume',
value : 5,
minValue: 0,
maxValue: 10
},
{
xtype: 'togglefield',
name : 'turbo',
label: 'Turbo'
},
{
xtype: 'spinnerfield',
minValue: 0,
maxValue: 100,
incrementValue: 2,
cycle: true
}
The following screenshot shows how the new components will look:
Our sliderfield and spinnerfield have configuration options for minValue and maxValue. We also added an incrementValue attribute, to spinnerfield, that will cause it to move in increments of 2 whenever the + or - button is pressed.