










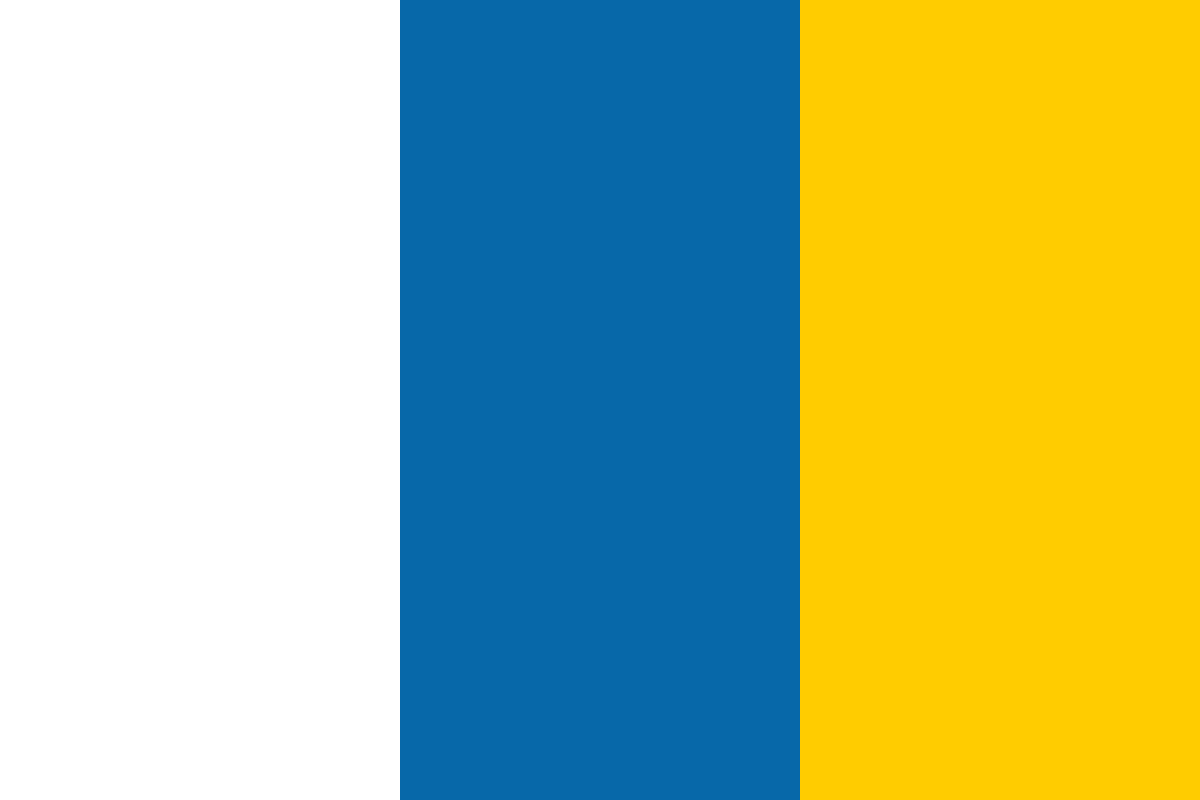

































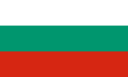








(For more resources related to this topic, see here.)
We are going to implement a simple scenario: given a deck of 52 cards, we want to pick a random number of cards, and then take out all of the hearts. From this stack of hearts, we will discard the first two and take the next five cards (if possible), and order them by their face value for display.
You can try it in a C# program query in LINQPad:
public static Random random = new Random();
void Main()
{
var deck = CreateDeck();
var randomCount = random.Next(52);
var hearts = new Card[randomCount];
var j = 0;
// take all hearts out
for(var i=0;i<randomCount;i++)
{
if(deck[i].Suit == "Hearts")
{
hearts[j++] = deck[i];
}
}
// resize the array to avoid null references
Array.Resize(ref hearts, j);
// check that we have at least 2 cards. If not, stop
if(hearts.Length <= 2)
return;
var count = 0;
// check how many cards we can take
count = hearts.Length - 2;
// the most we need to take is 5
if(count > 5) { count = 5; }
// take the cards
var finalDeck = new Card[count];
Array.Copy(hearts, 2, finalDeck, 0, count);
// now order the cards
Array.Sort(finalDeck, new CardComparer());
// Display the result
finalDeck.Dump();
}
public class Card
{
public string Suit { get; set; }
public int Value { get; set; }
}
// Create the cards' deck
public Card[] CreateDeck()
{
var suits = new [] { "Spades", "Clubs",
"Hearts", "Diamonds" };
var deck = new Card[52];
for(var i = 0; i < 52; i++)
{
deck[i] = new Card { Suit = suits[i / 13],
FaceValue = i-(13*(i/13))+1 };
}
// randomly shuffle the deck
for (var i = deck.Length - 1; i > 0; i--)
{
var j = random.Next(i + 1);
var tmp = deck[j];
deck[j] = deck[i];
deck[i] = tmp;
}
return deck;
}
// CardComparer compare 2 cards against their face value
public class CardComparer : Comparer<Card>
{
public override int Compare(Card x, Card y)
{
return x.FaceValue.CompareTo(y.FaceValue);
}
}
Even if we didn't consider the CreateDeck() method, we had to do quite a few operations to produce the expected result (your values might be different as we are using random cards). The output is as follows:
Depending on the data, LINQPad will add contextual information. For example, in this sample it will add the bottom row with the sum of all the values (here, only FaceValue).
Also, if you click on the horizontal graph button, you will get a visual representation of your data, as shown in the following screenshot:
This information is not always relevant but it can help you explore your data.
In this article we saw how LINQ queries can be used in LINQPad. The powerful query capabilitiesof LINQ has been utilized to the maximum in LINQPad.
Further resources on this subject: