










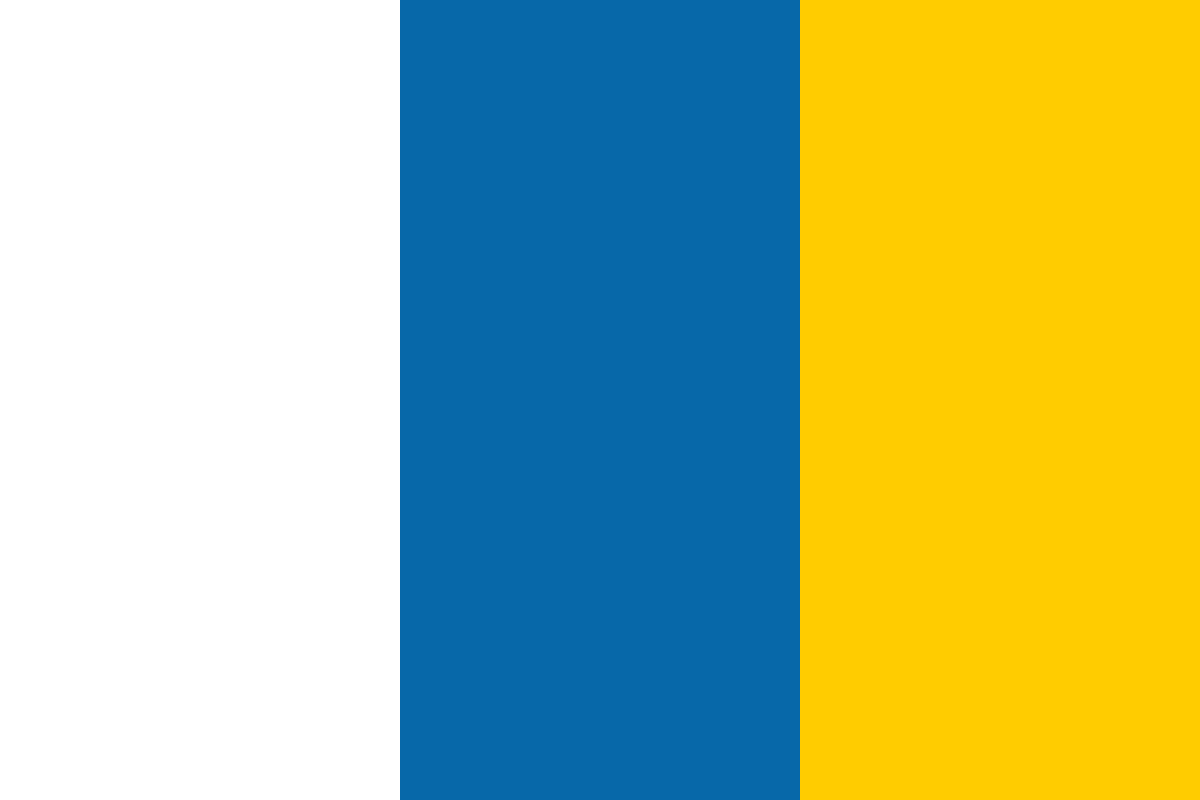

































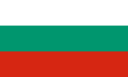








(For more resources related to this topic, see here.)
We must declare the jquery alias name within our Require.js configuration file.
require.config({
// 3rd party script alias names
paths: {
// Core Libraries
// --------------
// jQuery
"jquery": "libs/jquery",
// Plugins
// -------
"somePlugin": "libs/plugins/somePlugin"
}
});
If a jQuery plugin does not register itself as AMD compatible, we must also create a Require.js shim configuration to make sure Require.js loads jQuery before the jQuery plugin.
shim: {
// Twitter Bootstrap plugins depend on jQuery
"bootstrap": ["jquery"]
}
We will now be able to dynamically load a jQuery plugin with the require() method.
// Dynamically loads a jQuery plugin using the require() method
require(["somePlugin"], function() {
// The callback function is executed after the plugin
is loaded
});
We will also be able to list a jQuery plugin as a dependency to another module.
// Sample file
// -----------
// The define method is passed a dependency array and a callback
function
define(["jquery", "somePlugin"], function ($) {
// Wraps all logic inside of a jQuery.ready event
$(function() {
});
});
When using a jQueryUI Widget Factory plugin, we create Require.js path names for both the jQueryUI Widget Factory and the jQueryUI Widget Factory plugin:
"jqueryui": "libs/jqueryui",
"selectBoxIt": "libs/plugins/selectBoxIt"
Next, create a shim configuration property:
// The jQueryUI Widget Factory depends on jQuery
"jqueryui": ["jquery"],
// The selectBoxIt plugin depends on both jQuery and the jQueryUI
Widget Factory
"selectBoxIt": ["jqueryui"]
We will now be able to dynamically load the jQueryUI Widget Factory plugin with the require() method:
// Dynamically loads the jQueryUI Widget Factory plugin, selectBoxIt,
using the Require() method
require(["selectBoxIt"], function() {
// The callback function is executed after selectBoxIt.js
(and all of its dependencies) have been loaded
});
We will also be able to list the jQueryUI Widget Factory plugin as a dependency to another module:
// Sample file
// -----------
// The define method is passed a dependency array and a callback
function
define(["jquery", "selectBoxIt"], function ($) {
// Wraps all logic inside of a jQuery.ready event
$(function() {
});
});
Luckily for us, jQuery adheres to the AMD specification and registers itself as a named AMD module. If you are confused about how/why they are doing that, let's take a look at the jQuery source:
// Expose jQuery as an AMD module
if ( typeof define === "function" && define.amd && define.amd.jQuery )
{
define( "jquery", [], function () { return jQuery; } );
}
jQuery first checks to make sure there is a global define() function available on the page. Next, jQuery checks if the define function has an amd property, which all AMD loaders that adhere to the AMD API should have.
Remember that in JavaScript, functions are first class objects, and can contain properties.
Finally, jQuery checks to see if the amd property contains a jQuery property, which should only be there for AMD loaders that understand the issues with loading multiple versions of jQuery in a page that all might call the define() function.
Essentially, jQuery is checking that an AMD script loader is on the page, and then registering itself as a named AMD module (jquery).
Since jQuery exports itself as the named AMD module, jquery, you must use this exact name when setting the path configuration to your own version of jQuery, or Require.js will throw an error.
If a jQuery plugin registers itself as an anonymous AMD module and jQuery is also listed with the proper lowercased jquery alias name within your Require.js configuration file, using the plugin with the require() and define() methods will work as you expect.
Unfortunately, most jQuery plugins are not AMD compatible, and do not wrap themselves in an optional define() method and list jquery as a dependency. To get around this issue, we can use the Require.js shim object configuration like we have seen before to tell Require. js that a file depends on jQuery. The shim configuration is a great solution for jQuery plugins that do not register themselves as AMD modules.
Unfortunately, unlike jQuery, the jQueryUI does not currently register itself as a named AMD module, which means that plugin authors that use the jQueryUI Widget Factory cannot provide AMD compatibility. Since the jQueryUI Widget Factory is not AMD compatible, we must use a workaround involving the paths and shim configuration objects to properly define the plugin as an AMD module.
You will most likely always register your own files as anonymous AMD modules, but jQuery is a special case. Registering itself as a named AMD module allows other third-party libraries that depend on jQuery, such as jQuery plugins, to become AMD compatible by calling the define() method themselves and using the community agreed upon module name, jquery, to list jQuery as a dependency.
This article demonstrates how to use jQuery and jQueryUI Widget Factory plugins with Require.js.
Further resources on this subject: