










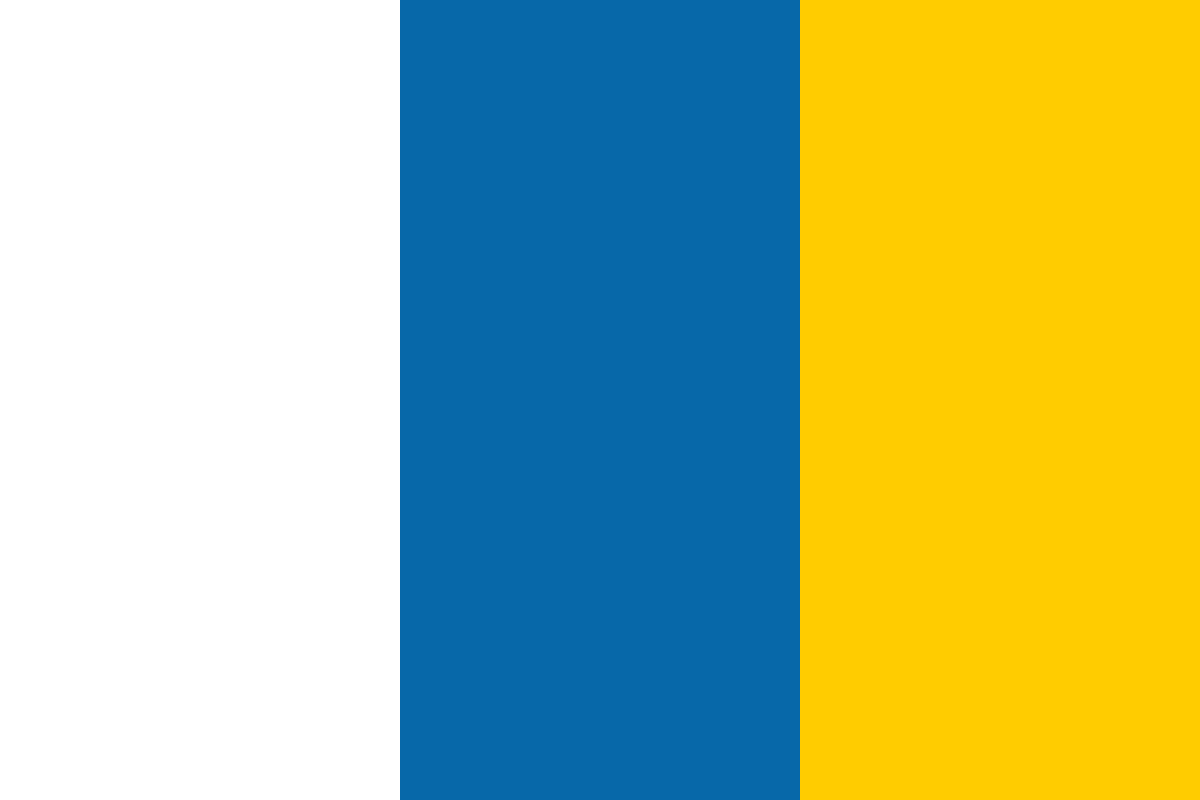

































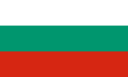








(For more resources on XNA 4.0, see here.)
We will define three different types of animated pieces: rotating, falling, and fading. The animation for each of these types will be accomplished by altering the parameters of the SpriteBatch.Draw() call.
In order to represent the three types of animated pieces, we will create three new classes. Each of these classes will inherit from the GamePiece class, meaning they will contain all of the methods and members of the GamePiece class, but add additional information to support the animation.
Child classes
Child classes inherit all of their parent's members and methods. The RotatingPiece class can refer to the pieceType and suffix of the piece without recreating them within RotatingPiece itself. Additionally, child classes can extend the functionality of their base class, adding new methods and properties or overriding old ones. In fact, Game1 itself is a child of the Micrsoft.Xna.Game class, which is why all of the methods we use (Update(), Draw(), LoadContent(), and so on) are declared as "override".
Let's begin by creating the class we will use for rotating pieces.
public bool clockwise;
public static float rotationRate = (MathHelper.PiOver2 / 10);
private float rotationAmount = 0;
public int rotationTicksRemaining = 10;
public float RotationAmount
{
get
{
if (clockwise)
return rotationAmount;
else
return (MathHelper.Pi*2) - rotationAmount;
}
}
public RotatingPiece(string pieceType, bool clockwise)
: base(pieceType)
{
this.clockwise = clockwise;
}
public void UpdatePiece()
{
rotationAmount += rotationRate;
rotationTicksRemaining = (int)MathHelper.Max(0,
rotationTicksRemaining-1);
}
In step 2, we modified the declaration of the RotatingPiece class by adding : GamePiece to the end of it. This indicates to Visual C# that the RotatingPiece class is a child of the GamePiece class.
The clockwise variable stores a "true" value if the piece will be rotating clockwise and "false" if the rotation is counter-clockwise.
When a game piece is rotated, it will turn a total of 90 degrees (or pi/2 radians) over 10 animation frames. The MathHelper class provides a number of constants to represent commonly used numbers, with MathHelper.PiOver2 being equal to the number of radians in a 90 degree angle. We divide this constant by 10 and store the result as the rotationRate for use later. This number will be added to the rotationAmount float, which will be referenced when the animated piece is drawn.
Working with radians
All angular math is handled in radians from XNA's point of view. A complete (360 degree) circle contains 2*pi radians. In other words, one radian is equal to about 57.29 degrees. We tend to relate to circles more often in terms of degrees (a right angle being 90 degrees, for example), so if you prefer to work with degrees, you can use the MathHelper.ToRadians() method to convert your values when supplying them to XNA classes and methods.
The final declaration, rotationTicksRemaining, is reduced by one each time the piece is updated. When this counter reaches zero, the piece has finished animating.
When the piece is drawn, the RotationAmount property is referenced by a spriteBatch. Draw() call and returns either the rotationAmount property (in the case of a clockwise rotation) or 2*pi (a full circle) minus the rotationAmount if the rotation is counter-clockwise.
The constructor in step 7 illustrates how the parameters passed to a constructor can be forwarded to the class' parent constructor via the :base specification. Since the GamePiece class has a constructor that accepts a piece type, we can pass that information along to its constructor while using the second parameter (clockwise) to update the clockwise member that does not exist in the GamePiece class. In this case, since both the clockwise member and the clockwise parameter have identical names, we specify this.clockwise to refer to the clockwise member of the RotatingPiece class. Simply clockwise in this scope refers only to the parameter passed to the constructor.
this notation
You can see that it is perfectly valid C# code to have method parameter names that match the names of class variables, thus potentially hiding the class variables from being used in the method (since referring to the name inside the method will be assumed to refer to the parameter). To ensure that you can always access your class variables even when a parameter name conflicts, you can preface the variable name with this. when referring to the class variable. this. indicates to C# that the variable you want to use is part of the class, and not a local method parameter.
Lastly, the UpdatePiece() method simply increases the rotationAmount member while decreasing the rotationTicksRemaining counter (using MathHelper.Max() to ensure that the value does not fall below zero).
public int VerticalOffset;
public static int fallRate = 5;
public FallingPiece(string pieceType, int verticalOffset)
: base(pieceType)
{
VerticalOffset = verticalOffset;
}
public void UpdatePiece()
{
VerticalOffset = (int)MathHelper.Max(
0,
VerticalOffset - fallRate);
}
Simpler than a RotatingPiece, a FallingPiece is also a child of the GamePiece class. A falling piece has an offset (how high above its final destination it is currently located) and a falling speed (the number of pixels it will move per update).
As with a RotatingPiece, the constructor passes the pieceType parameter to its base class constructor and uses the verticalOffset parameter to set the VerticalOffset member. Note that the capitalization on these two items differs. Since VerticalOffset is declared as public and therefore capitalized by common C# convention, there is no need to use the "this" notation, since the two variables technically have different names.
Lastly, the UpdatePiece() method subtracts fallRate from VerticalOffset, again using the MathHelper.Max() method to ensure the offset does not fall below zero.
public float alphaLevel = 1.0f;
public static float alphaChangeRate = 0.02f;
public FadingPiece(string pieceType, string suffix)
: base(pieceType, suffix)
{
}
public void UpdatePiece()
{
alphaLevel = MathHelper.Max(
0,
alphaLevel - alphaChangeRate);
}
The simplest of our animated pieces, the FadingPiece only requires an alpha value (which always starts at 1.0f, or fully opaque) and a rate of change. The FadingPiece constructor simply passes the parameters along to the base constructor.
When a FadingPiece is updated, alphaLevel is reduced by alphaChangeRate, making the piece more transparent.
Now that we can create animated pieces, it will be the responsibility of the GameBoard class to keep track of them. In order to do that, we will define a Dictionary object for each type of piece.
A Dictionary is a collection object similar to a List, except that instead of being organized by an index number, a dictionary consists of a set of key and value pairs. In an array or a List, you might access an entity by referencing its index as in dataValues[2] = 12; With a Dictionary, the index is replaced with your desired key type. Most commonly this will be a string value. This way, you can do something like fruitColors["Apple"]="red";
public Dictionary<string, FallingPiece> fallingPieces =
new Dictionary<string, FallingPiece>();
public Dictionary<string, RotatingPiece> rotatingPieces =
new Dictionary<string, RotatingPiece>();
public Dictionary<string, FadingPiece> fadingPieces =
new Dictionary<string, FadingPiece>();
public void AddFallingPiece(int X, int Y,
string PieceName, int VerticalOffset)
{
fallingPieces[X.ToString() + "_" + Y.ToString()] = new
FallingPiece(PieceName, VerticalOffset);
}
public void AddRotatingPiece(int X, int Y,
string PieceName, bool Clockwise)
{
rotatingPieces[X.ToString() + "_" + Y.ToString()] = new
RotatingPiece(PieceName, Clockwise);
}
public void AddFadingPiece(int X, int Y, string PieceName)
{
fadingPieces[X.ToString() + "_" + Y.ToString()] = new
FadingPiece(PieceName,"W");
}
{
if ((fallingPieces.Count == 0) &&
(rotatingPieces.Count == 0) &&
(fadingPieces.Count == 0))
{
return false;
}
else
{
return true;
}
}
private void UpdateFadingPieces()
{
Queue<string> RemoveKeys = new Queue<string>();
foreach (string thisKey in fadingPieces.Keys)
{
fadingPieces[thisKey].UpdatePiece();
if (fadingPieces[thisKey].alphaLevel == 0.0f)
RemoveKeys.Enqueue(thisKey.ToString());
}
while (RemoveKeys.Count > 0)
fadingPieces.Remove(RemoveKeys.Dequeue());
}
private void UpdateFallingPieces()
{
Queue<string> RemoveKeys = new Queue<string>();
foreach (string thisKey in fallingPieces.Keys)
{
fallingPieces[thisKey].UpdatePiece();
if (fallingPieces[thisKey].VerticalOffset == 0)
RemoveKeys.Enqueue(thisKey.ToString());
}
while (RemoveKeys.Count > 0)
fallingPieces.Remove(RemoveKeys.Dequeue());
}
private void UpdateRotatingPieces()
{
Queue<string> RemoveKeys = new Queue<string>();
foreach (string thisKey in rotatingPieces.Keys)
{
rotatingPieces[thisKey].UpdatePiece();
if (rotatingPieces[thisKey].rotationTicksRemaining == 0)
RemoveKeys.Enqueue(thisKey.ToString());
}
while (RemoveKeys.Count > 0)
rotatingPieces.Remove(RemoveKeys.Dequeue());
}
public void UpdateAnimatedPieces()
{
if (fadingPieces.Count == 0)
{
UpdateFallingPieces();
UpdateRotatingPieces();
}
else
{
UpdateFadingPieces();
}
}
After declaring the three Dictionary objects, we have three methods used by the GameBoard class to create them when necessary. In each case, the key is built in the form "X_Y", so an animated piece in column 5 on row 4 will have a key of "5_4". Each of the three Add... methods simply pass the parameters along to the constructor for the appropriate piece types after determining the key to use.
When we begin drawing the animated pieces, we want to be sure that animations finish playing before responding to other input or taking other game actions (like creating new pieces). The ArePiecesAnimating() method returns "true" if any of the Dictionary objects contain entries. If they do, we will not process any more input or fill empty holes on the game board until they have completed.
The UpdateAnimatedPieces() method will be called from the game's Update() method and is responsible for calling the three different update methods above (UpdateFadingPiece(), UpdateFallingPiece(), and UpdateRotatingPiece()) for any animated pieces currently on the board. The first line in each of these methods declares a Queue object called RemoveKeys. We will need this because C# does not allow you to modify a Dictionary (or List, or any of the similar "generic collection" objects) while a foreach loop is processing them.
A Queue is yet another generic collection object that works like a line at the bank. People stand in a line and await their turn to be served. When a bank teller is available, the first person in the line transacts his/her business and leaves. The next person then steps forward. This type of processing is known as FIFO, or First In, First Out.
Using the Enqueue() and Dequeue() methods of the Queue class, objects can be added to the Queue (Enqueue()) where they await processing. When we want to deal with an object, we Dequeue() the oldest object in the Queue and handle it. Dequeue() returns the first object waiting to be processed, which is the oldest object added to the Queue.
Collection classes
C# provides a number of different "collection" classes, such as the Dictionary, Queue, List, and Stack objects. Each of these objects provides different ways to organize and reference the data in them. For information on the various collection classes and when to use each type, see the following MSDN entry: http://msdn.microsoft.com/en-us/library/6tc79sx1(VS.80).aspx
Each of the update methods loops through all of the keys in its own Dictionary and in turn calls the UpdatePiece() method for each key. Each piece is then checked to see if its animation has completed. If it has, its key is added to the RemoveKeys queue. After all of the pieces in the Dictionary have been processed, any keys that were added to RemoveKeys are then removed from the Dictionary, eliminating those animated pieces.
If there are any FadingPieces currently active, those are the only animated pieces that UpdateAnimatedPieces() will update. When a row is completed, the scoring tiles fade out, the tiles above them fall into place, and new tiles fall in from above. We want all of the fading to finish before the other tiles start falling (or it would look strange as the new tiles pass through the fading old tiles).
In the discussion of UpdateAnimatedPieces(), we stated that fading pieces are added to the board whenever the player completes a scoring chain. Each piece in the chain is replaced with a fading piece.
gameBoard.AddFadingPiece(
(int)ScoringSquare.X,
(int)ScoringSquare.Y,
gameBoard.GetSquare(
(int)ScoringSquare.X,
(int)ScoringSquare.Y));
Adding fading pieces is simply a matter of getting the square (before it is replaced with an empty square) and adding it to the FadingPieces dictionary. We need to use the (int) typecasts because the ScoringSquare variable is a Vector2 value, which stores its X and Y components as floats.
Falling pieces are added to the game board in two possible locations: From the FillFromAbove() method when a piece is being moved from one location on the board to another, and in the GenerateNewPieces() method, when a new piece falls in from the top of the game board.
AddFallingPiece(x, y, GetSquare(x, y),
GamePiece.PieceHeight *(y-rowLookup));
AddFallingPiece(x, y, GetSquare(x, y),
GamePiece.PieceHeight * GameBoardHeight);
When FillFromAbove() moves a piece downward, we now create an entry in the FallingPieces dictionary that is equivalent to the newly moved piece. The vertical offset is set to the height of a piece (40 pixels) times the number of board squares the piece was moved. For example, if the empty space was at location 5,5 on the board, and the piece above it (5,4) is being moved down one block, the animated piece is created at 5,5 with an offset of 40 pixels (5-4 = 1, times 40).
When new pieces are generated for the board, they are added with an offset equal to the height (in pixels) of the game board, determined by multiplying the GamePiece.PieceHeight value by the GameBoardHeight. This means they will always start above the playing area and fall into it.