










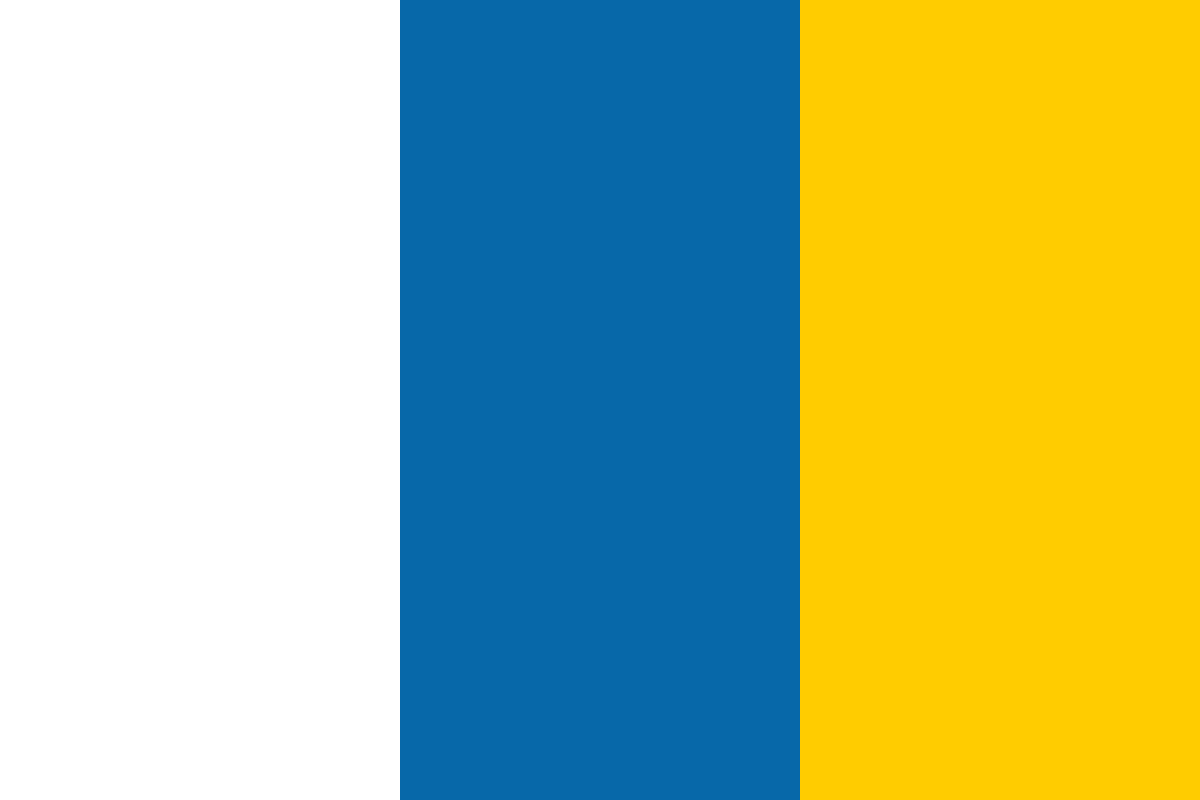

































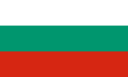








(Read more interesting articles on CodeIgniter 1.7 Professional Development here.)
You need to register a new Facebook Application so that you can get an API key and an Application Secret Key. Head on over to www.facebook.com/developers/ and click on the Set up New Application button in the upper right–hand corner.
This process is very similar to setting up a new Twitter application which we covered in the previous article, so I won't bore you with all of the details.
Once you've done that, you should have your API key and Application Secret Key. These two things will enable Facebook to recognize your application.
When you are on your applications page showing all your applications' information, scroll down the page to see a link to download the Client Library. Once you've downloaded it, simply untar it.
There are two folders inside the facebook-platform folder, footprints and php. We are only going to be using the php folder. Open up the php folder; there are two files here that we don't need, facebook_desktop.php and facebook_mobile.php—you can delete them.
Finally, we can copy this folder into our application. Place it in the system/application/libraries folder, and then rename the folder to facebook. This helps us to keep our code tidy and properly sorted.
Before we start coding, we need to know what we need to code in order to make the Facebook Client Library work with our CodeIgniter installation.
Our Wrapper library needs to instantiate the Facebook class with our API Key and Secret Application Key. We'll also want it to create a session for the user when they are logged in. If a session is found but the user is not authenticated, we will need to destroy the session.
You should create a new file in the system/application/libraries/ folder, called Facebook_connect.php. This is where the Library code given next should be placed.
The Base Class for our Facebook Connect Wrapper Library is very simple:
<?php
require_once(APPPATH . 'libraries/facebook/facebook.php');
class Facebook_connect
{
var $CI;
var $connection;
var $api_key;
var $secret_key;
var $user;
var $user_id;
var $client;
}
?>
The first thing that our Library needs to do is to load the Facebook library—the one we downloaded from facebook.com. We build the path for this by using APPPATH, a constant defined by CodeIgniter to be the path of the application folder.
Then, in our Class we have a set of variables. The $CI variable is the variable in which we will store the CodeIgniter super object; this allows us to load CodeIgniter resources (libraries, models, views, and so on) in our library. We'll only be using this to load and use the CodeIgniter Session library, however.
The $connection variable will contain the instance of the Facebook class. This will allow us to grab any necessary user data and perform any operations that we like, such as updating a user's status or sending a message to one of their friends.
The next few variables are pretty self-explanatory—they will hold our API Key and Secret Key.
The $user variable will be used to store all of the information about our user, including general details about the user such as their profile URL and their name. The $user_id variable will be used to store the user ID of our user.
Finally, the $client variable is used to store general information about our connection to Facebook, including the username of the user currently using the connection, amongst other things such as server addresses to query for things like photos.
Our class constructor has to do a few things in order to allow us to authenticate our users using Facebook Connect. Here's the code:
function Facebook_connect($data)
{
$this->CI =& get_instance();
$this->CI->load->library('session');
$this->api_key = $data['api_key'];
$this->secret_key = $data['secret_key'];
$this->connection =
new Facebook($this->api_key, $this->secret_key);
$this->client = $this->connection->api_client;
$this->user_id = $this->connection->get_loggedin_user();
$this->_session();
}
The first line in our function should be new to everyone reading this article. The function get_instance() allows us to assign the CodeIgniter super object by reference to a local variable. This allows us to use all of CodeIgniter's syntax for loading libraries, and so on; but instead of using $this->load we would use $this->CI->load. But of course it doesn't just allow us to use the Loader—it allows us to use any CodeIgniter resource, as we normally would inside a Controller or a Model. The next line of code gives us a brilliant example of this: we're loading the session library using the variable $this->CI rather than the usual $this.
The next two lines simply set the values of the API key and Secret Application Key into a class variable so that we can reference it throughout the whole class. The $data array is passed into the constructor when we load the library in our Controller. More on that when we get there.
Next up, we create a new instance of the Facebook Class (this is contained within the Facebook library that we include before our own class code) and we pass the API Key and Secret Application Key through to the class instance. This is all assigned to the class variable $this->connection, so that we can easily refer to it anywhere in the class.
The next two lines are specific parts of the overall Facebook instance. All of the client details and the data that helps us when using the connection are stored in a class variable, in order to make it more accessible. We store the client details in the variable $this->client. The next line of code stores all of the details about the user that were provided to us by the Facebook class. We store this in a class variable for the same reason as storing the client data: it makes it easier to get to. We store this data in $this->user_id.
The next line of code calls upon a function inside our class. The underscore at the beginning tells CodeIgniter that we only want to be able to use this function inside this class; so you couldn't use it in a Controller, for example. I'll go over this function shortly.
This function manages the user's CodeIgniter session. Take a look at the following code:
function _session()
{
$user = $this->CI->session->userdata('facebook_user');
if($user === FALSE && $this->user_id !== NULL)
{
$profile_data = array('uid','first_name', 'last_name',
'name', 'locale', 'pic_square', 'profile_url');
$info = $this->connection->api_client->
users_getInfo($this->user_id, $profile_data);
$user = $info[0];
$this->CI->session->set_userdata('facebook_user', $user);
}
elseif($user !== FALSE && $this->user_id === NULL)
{
$this->CI->session->sess_destroy();
}
if($user !== FALSE)
{
$this->user = $user;
}
}
This function initially creates a variable and sets its value to that of the session data from the CodeIgniter session library.
Then we go through a check to see if the session is empty and the $this->user_id variable is false. This means that the user has not yet logged in using Facebook Connect. So we create an array of the data that we want to get back from the Facebook class, and then use the function users_getInfo() provided by the class to get the information in the array that we created. Then we store this data into the $user variable and create a new session for the user.
The next check that we do is that if the $user variable is not empty, but the $this->user_id variable is empty, then the user is not authenticated on Facebook's side so we should destroy the session. We do this by using a function built in to the Session Library sess_destroy();
Finally, we check to see if the $user variable is not equal to FALSE. If it passes this check, we set the $this->user class variable to that of the local $user variable.