










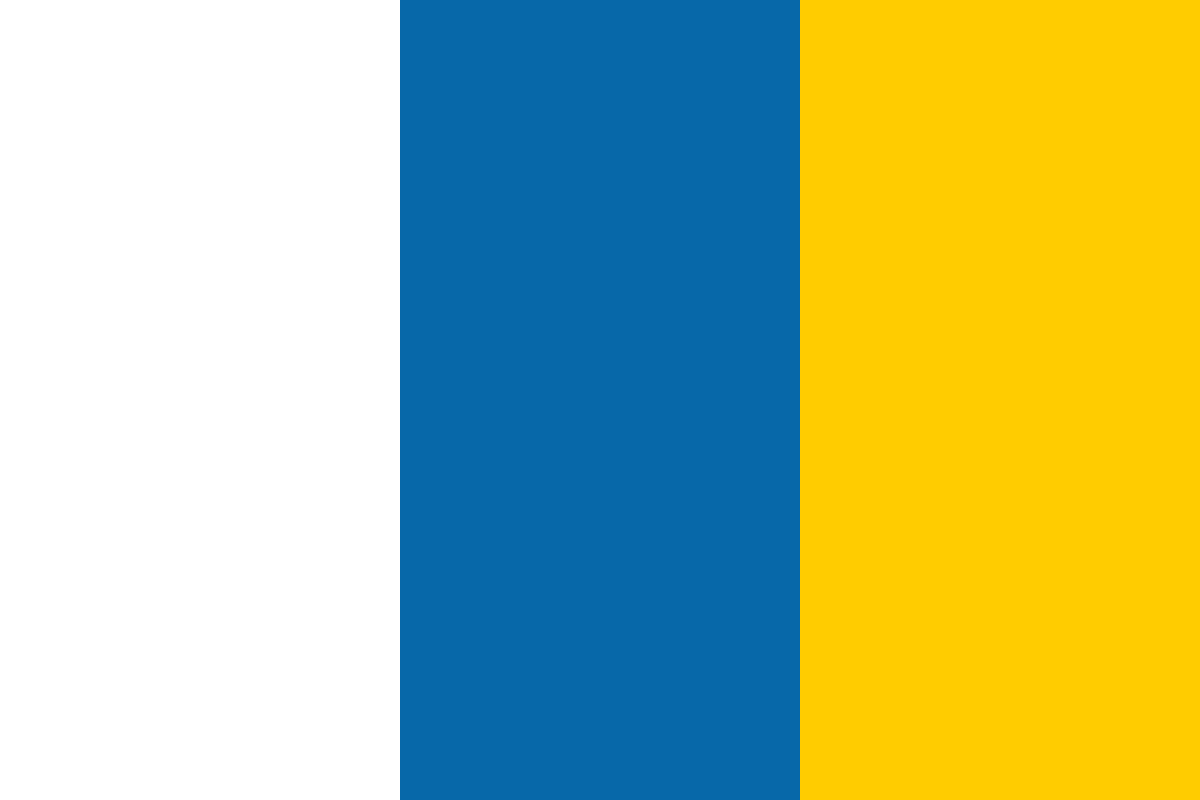

































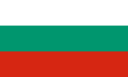








(For more resources related to this topic, see here.)
Create a new Single View Application in Xamarin Studio and name it BackgroundFetchApp. Add a label to the controller.
Perform the following steps:
public UILabel LabelStatus { get { return this.lblStatus; } }
UIApplication.SharedApplication.SetMinimumBackgroundFetchIn
terval(UIApplication.BackgroundFetchIntervalMinimum);
Enter the following code, again, in the AppDelegate class:
private int updateCount;
public override void PerformFetch (UIApplication application,
Action<UIBackgroundFetchResult> completionHandler)
{
try {
HttpWebRequest request = WebRequest.Create
("http://software.tavlikos.com") as HttpWebRequest;
using (StreamReader sr = new StreamReader
(request.GetResponse().GetResponseStream())) {
Console.WriteLine("Received response: {0}",
sr.ReadToEnd());
}
this.viewController.LabelStatus.Text =
string.Format("Update count: {0}/n{1}",
++updateCount, DateTime.Now);
completionHandler(UIBackgroundFetchResult.NewData);
} catch {
this.viewController.LabelStatus.Text =
string.Format("Update {0} failed at {1}!",
++updateCount, DateTime.Now);
completionHandler(UIBackgroundFetchResult.Failed);
}
}
The UIBackgroundModes key with the fetch value enables the background fetch functionality for our app. Without setting it, the app will not wake up in the background.
After setting the key in Info.plist, we override the PerformFetch method in the AppDelegate class, as follows:
public override void PerformFetch (UIApplication application, Action<UIBackgroundFetchResult> completionHandler)
This method is called whenever the system wakes up the app. Inside this method, we can connect to a server and retrieve the data we need. An important thing to note here is that we do not have to use iOS-specific APIs to connect to a server. In this example, a simple HttpWebRequest method is used to fetch the contents of this blog: http://software.tavlikos.com.
After we have received the data we need, we must call the callback that is passed to the method, as follows:
completionHandler(UIBackgroundFetchResult.NewData);
We also need to pass the result of the fetch. In this example, we pass UIBackgroundFetchResult.NewData if the update is successful and UIBackgroundFetchResult.Failed if an exception occurs.
If we do not call the callback in the specified amount of time, the app will be terminated. Furthermore, it might get fewer opportunities to fetch the data in the future.
Lastly, to make sure that everything works correctly, we have to set the interval at which the app will be woken up, as follows:
UIApplication.SharedApplication.SetMinimumBackgroundFetchInterval(
UIApplication.BackgroundFetchIntervalMinimum);
The default interval is UIApplication.BackgroundFetchIntervalNever, so if we do not set an interval, the background fetch will never be triggered.
Except for the functionality we added in this project, the background fetch is completely managed by the system. The interval we set is merely an indication and the only guarantee we have is that it will not be triggered sooner than the interval. In general, the system monitors the usage of all apps and will make sure to trigger the background fetch according to how often the apps are used.
Apart from the predefined values, we can pass whatever value we want in seconds.
We can update the UI in the PerformFetch method. iOS allows this so that the app's screenshot is updated while the app is in the background. However, note that we need to keep UI updates to the absolute minimum.
Thus, this article covered the things to keep in mind to make use of iOS 7's background fetch feature.
Further resources on this subject: