










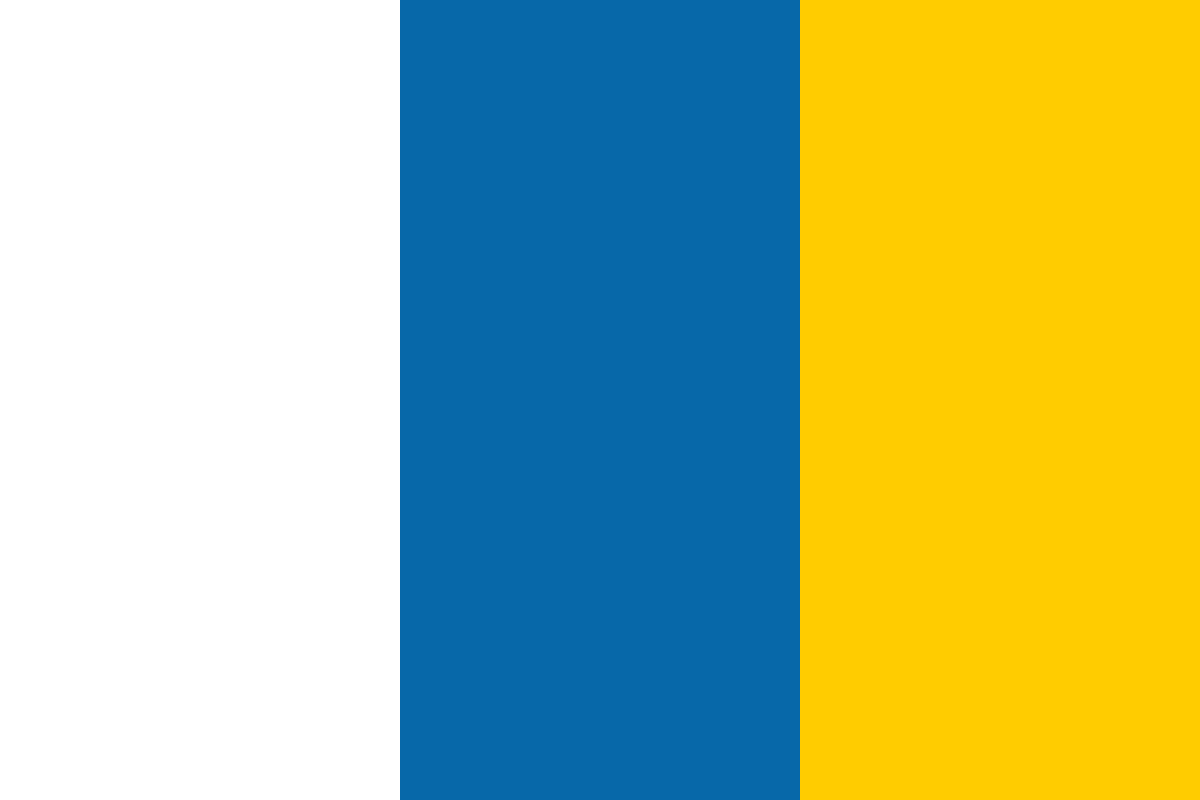

































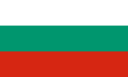








We will need to create an Android app, get it all set up, then add a test project to it. Let's begin.
1. Start Android Studio and select new project.
2. Change the Application name to UTest. Click Next .
3. Click Next again.
4. Click Finish.
Now that we have the project started, let’s set it up. Open the layout resource file:activity_main.xml. Add an ID to TextView. It should look as follows:
<RelativeLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:paddingLeft="@dimen/activity_horizontal_margin"
android:paddingRight="@dimen/activity_horizontal_margin"
android:paddingTop="@dimen/activity_vertical_margin"
android:paddingBottom="@dimen/activity_vertical_margin"
tools:context="com.tekadept.utest.app.MainActivity" >
<TextView
android:id="@+id/greeting"
android:text="@string/hello_world"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</RelativeLayout>
Next we modify the MainActivity class. We are going to add some code that will display a random greeting message to the user. Modify MainActivity so that it looks like the following code:
TextViewtxtGreeting;
@Override
protected void onCreate(Bundle savedInstanceState) {
super .onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
txtGreeting = (TextView)findViewById(R.id.greeting);
Random rndGenerator = new Random();
int rnd = rndGenerator.nextInt(4);
String greeting = getGreeting(rnd);
txtGreeting.setText(greeting);
}
private String getGreeting(intmsgNumber) {
String greeting;
switch (msgNumber){
case 0:
greeting = "Holamundo";
break ;
case 1:
greeting = "Bonjour tout le monde";
break ;
case 2:
greeting = "Ciao mondo";
break ;
case 3:
greeting = "Hallo Welt";
break ;
default :
greeting = "Hello world";
break ;
}
return greeting;
}
At this point, if you run the app, it should display one of four random greetings each time you run. We want to test the getGreeting method. We need to be sure that the string it returns matches the number we sent it. Currently, however, we have no way to know that.
In order to add a test package, we need to hover over the package name. For my app, the package name is com.tekadept.utest.app . It is the line directly below the Java directory. The rest of the steps are as follows:
Currently, we are not extending from the proper base class. Let's fix that. Change the MainActivityTest class so it looks like the following code:
package com.tekadept.utest.app.tests;
import android.test.ActivityInstrumentationTestCase2;
import com.tekadept.utest.app.MainActivity;
public class MainActivityTestextends ActivityInstrumentationTestCase2<MainActivity>{
public MainActivityTest() {
super (MainActivity.class);
}
}
We've done two things. First, we changed the base class to ActivityInstrumentationTestCase2. Secondly, we added a constructor method. Before we can test the logic of the getGreeting method, we need to make it visible to outside classes by changing its modifier from private to public. Once we've done that, return to the MainActivityTest class and add a new method, testGetGreetings. This is shown in the following code:
public void testGetGreeting() throws Exception {
MainActivity activity = getActivity();
int count = 0;
String result = activity.getGreeting(count);
Assert.assertEquals("Holamundo", result);
count = 1;
result = activity.getGreeting(count);
Assert.assertEquals("Bonjour tout le monde", result);
count = 2;
result = activity.getGreeting(count);
Assert.assertEquals("Ciao mondo", result);
count = 3;
result = activity.getGreeting(count);
Assert.assertEquals("Hallo Welt", result);
}
All we need to do now is create a configuration for our test package.
The Android unit test must run on a device or emulator. I prefer having the choose dialog come up, so I've selected that option. You should select whichever option works best for you. Then click OK.
At this point, you have a working app complete with a functioning unit test. To run the unit test, choose the test configuration from the drop-down menu to the left of the run button. Then click the run button. After building your app and running it on your selected device, Android Studio will show the test results. If you don't see the results, click the run button in the lower left hand corner of Android Studio. Green is good. Red means one or more tests have failed. Currently our one test should be passing, so everything should be green. In order to see a test fail, let's make a temporary change to the getGreeting method. Change the first greeting from "Holamundo" to "Adios mundo". Save your change and click the run button to run the tests again. This time the test should fail. You should see a message something like the following:
The test runner shows the failure message and includes a stack trace of the failure. The first line of the stack trace shows that the test failed on line 17 of MainActivityTest . Don't forget to restore the MainActivity class' getGreeting method back to fix the failing unit test.
That is it for this post. You now know how to add a unit test package to Android Studio. If you had any trouble with this post, be sure to check out the complete source code to the UTest project on my GitHub repo at: https://github.com/Rockncoder/UTest.
From 14th-20th March we're throwing the spotlight on iOS and Android, and asking you which one you think will win out in the future. Tell us - then save 50% on a selection of our very best Android and iOS titles!
Troy Miles, also known asthe Rockncoder, currently has fun writing full stack code with ASP.NET MVC or Node.js on the backend and web or mobile up front. He started coding over 30 years ago, cutting his teeth writing games for C64, Apple II, and IBM PCs. After burning out, he moved on to Windows system programming before catching Internet fever just before the dot net bubble burst. After realizing that mobile devices were the perfect window into backend data, he added mobile programming to his repertoire. He loves competing in hackathons and randomly posting interesting code nuggets on his blog: http://therockncoder.blogspot.com/.