










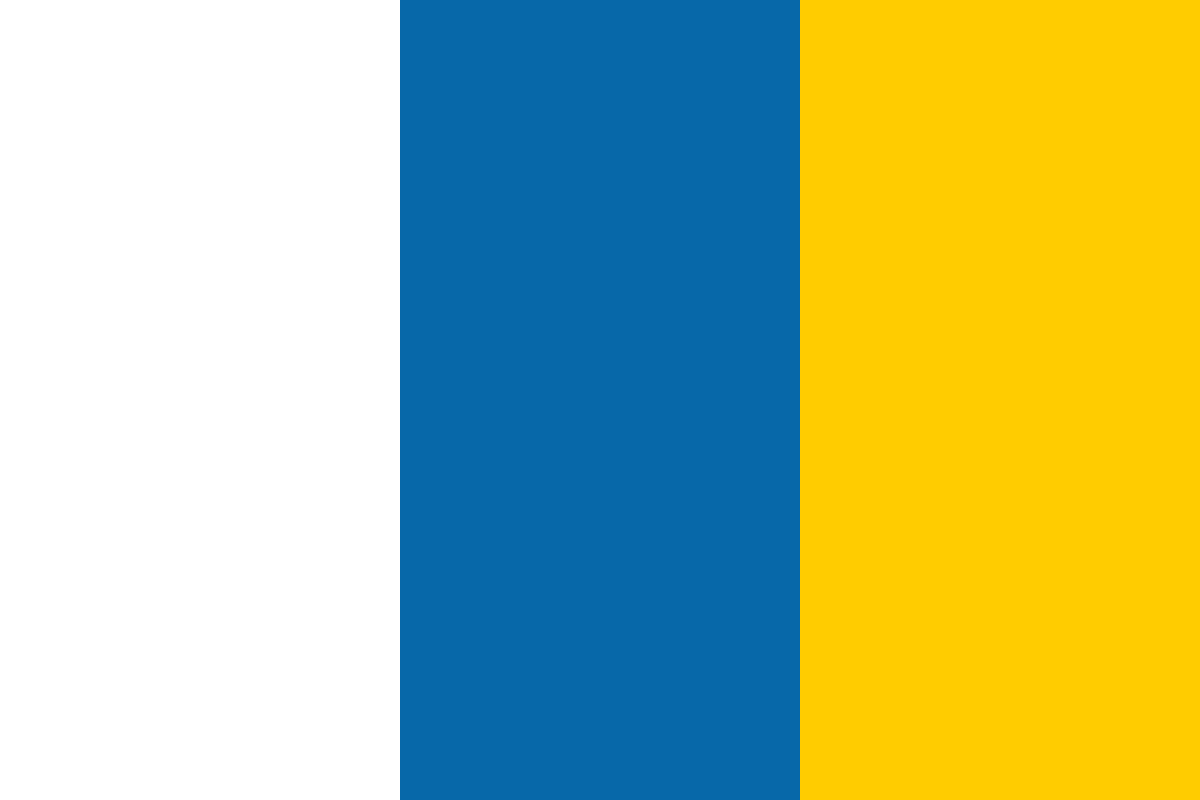

































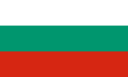








(For more resources related to this topic, see here.)
A Maven project is simply a folder in your filesystem (also known as the project root folder) that contains a file called pom.xml, the XML representation of your Project Object Model (POM); this is the first—and most important—Maven convention.
This minimal structure allows you to run a mvn command from the project root folder. By default, the mvn command searches for a pom.xml file in the local folder and it stops immediately if it is not able to find it.
By convention, all artifacts generated by the build are delivered in a folder—relative to the pom.xml location—known as Build Directory (the target by default). Since the target is generated on each build, it is:
A Maven project defines a packaging, which identifies the main objective of the build, which in turn specifies the artifact that is going to be produced by the invocation of the build.Default JAR (other values are EAR, EJB, RAR, PAR, WAR, and POM).
POM packaging is an exception, since:
A POM Maven project can be useful for the following activities:
Every Maven POM implicitly inherits from Super POM (more information is available at http://maven.apache.org/ref/3.0.5/maven-model-builder/super-pom.html), which contains all the default values that are needed to perform built-in Maven features, as we will see later in this book. Super POM is provided by the Maven installation.
It is not intended to be changed—as it would cause build portability issues (more information is available at http://www.devx.com/Java/Article/32386)–but it is definitely interesting to read and investigate it further (more information is available at http://maven.apache.org/ guides/introduction/introduction-to-the-pom.html) in order to be more confident when using/overriding values in your pom.xml file.
An artifact–in a Maven context–is a file that is (or has been) produced by a build execution and represents an application binary (of a specific version) that is subject to a lifecycle.
An artifact can have diff erent purposes, listed as follows:
The following coordinates uniquely identify an artifact:
For more information about naming conventions, check the official Maven documentation available at http://maven.apache.org/guides/mini/guide-naming-conventions.html
An example of a minimal pom.xml file is as follows:
<project>
<modelVersion>4.0.0</modelVersion>
<groupId>com.mycompany.app</groupId>
<artifactId>app-web</artifactId>
<version>1.0-SNAPSHOT</version>
<type>war</type>
</project>
The pom.xml file is the central configuration file for a Maven project, and it is fundamental to understand it deeply; it contains information about the project structure, metadata, and confi guration related with the plugin's executions.
The modelVersion element is in every pom.xml; it represents the pom.xml XML schema version and is set to 4.0.0 for all Maven 2.x and 3.x-based builds.
The–SNAPSHOT suffix in a pom.xml file specifies that the artifacts produced by this build are unreleased, and therefore they considered nightly builds.
In this section we will introduce the most important elements of a POM; some will not be mentioned, while some others will be briefl y introduced.
A pom.xml file can define a parent as a pointer to a POM artifact. As a result, all parent's Maven configurations will be inherited.
<parent>
<groupId>com.mycompany.myproject</groupId>
<artifactId>my-parent-pom</artifactId>
<version>1.0-SNAPSHOT</version>
</parent>
A Maven plugin is a JAR Maven artifact containing Java classes that implement one or more goals using the Maven Plugin API (more information is available at http://maven.apache. org/ref/3.0.5/maven-plugin-api), and declares a public short name (that is, tomcat7x).
<build>
<plugins>
<plugin>
<groupId>org.apache.tomcat.maven</groupId>
<artifactId>tomcat7-maven-plugin</artifactId>
<version>2.1</version>
<configuration>
<url>http://127.0.0.1:8080/manager</url>
<server>Tomcat</server>
<path>/app-web</path>
</configuration>
</plugin>
</plugins>
</build>
The goal identifies a build task; it has a unique name (that is, run) within the same plugin. It can access and change the POM and provide a wide range of operations, from zipping a folder to performing a remote deployment on a Tomcat server.
A goal can be executed by the mvn command using the following syntax:
<plugin_shortname>:<goal_name>, for example mvn tomcat7x:run
A goal can be invoked by the lifecycle phase; you will read more about it in the Maven lifecycle section.
All plugins having an artifactId coordinate starting with maven- are directly supported by Maven projects (more information is available at http://maven.apache.org/plugins/).
In order to understand how to use a Maven Plugin, search for its official documentation page; the Usage page (available at http://tomcat.apache.org/maven-plugin-2.0/tomcat7- maven-plugin/usage.html) explains how to add the plugin to your build; the Goals page (available at http://tomcat.apache.org/maven-plugin-2.0/tomcat7-maven-plugin/plugin-info.html) lists all goals and parameters that you can set.
A Maven Repository is a folder with a specific layout that can optionally be located remotely:
<repositories>
<repository>
<id>my-custom-repo</id>
<url>http://artifacts.mysite.com/repository</url>
</repository>
</repositories>
The Repository layout is a key convention in Maven that allows you to uniquely locate an artifact:
<repository_url>/<groupId>/<artifactId>/<version>/<artifactId>-
<classifier>-<version>.<type>
For example, you can have the following coordinates for the preceding artifact:
For a remote URL, the Repository URL can be:
The layout key will hence be:
For a local URL, the Repository URL can be:
The layout key will hence be:
A Maven Repository is the source and the destination of artifacts in the following scenarios:
A Maven Repository can restrict the download/upload artifact operations depending whether the artifact's version is a -SNAPSHOT literal or not. This way, you can easily defi ne nightly builds, repositories, and defi ne tailored maintenance operations (that is, remove -SNAPSHOT artifacts after 30 days).
-SNAPSHOT artifacts are a special case for Maven Repositories:
The Super POM defines two very special repositories:
Thus this article helps us understand Maven vocabulary more closely as it is very important to have all the concepts clear in order to gain a complete understanding of Maven.