










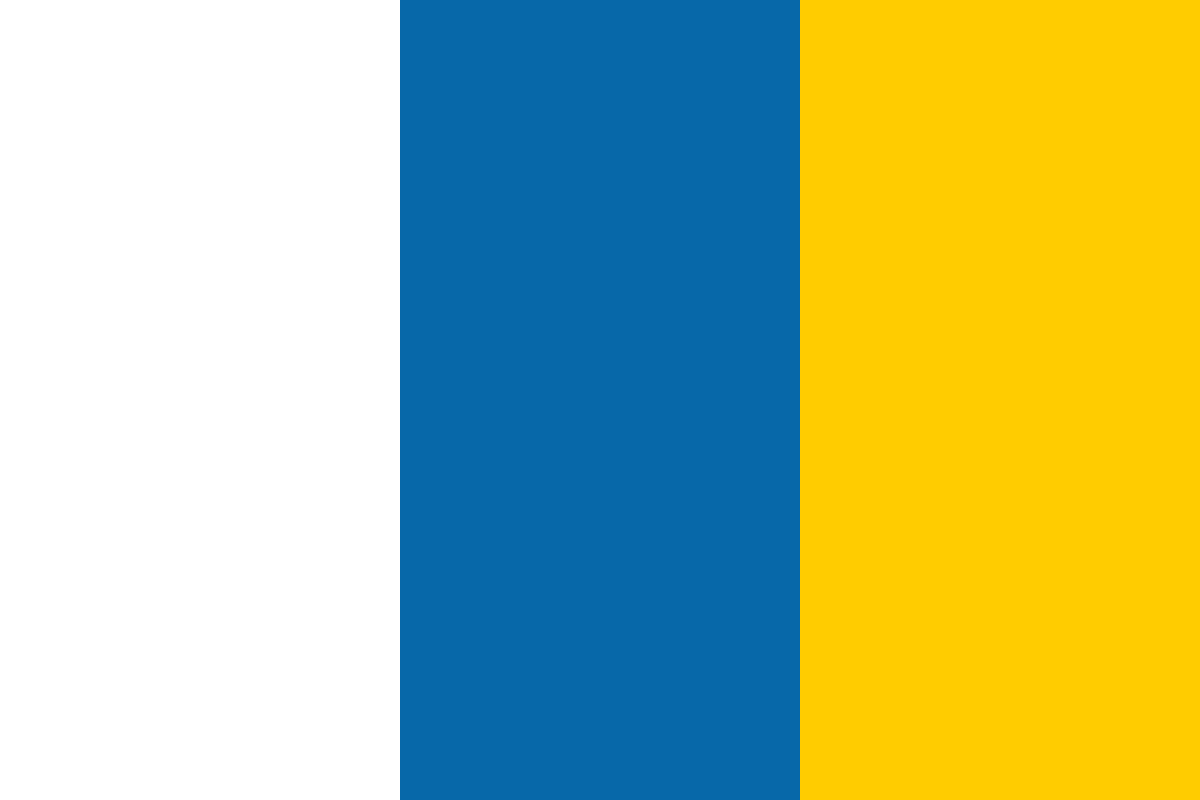

































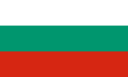








The Flickr API is very powerful and gives access to just about everything a user can do manually. You can write scripts to automatically download latest pictures from a photostream, download photos or videos tagged with a certain keyword, or post comments on photos. In this recipe, we will make use of the phpFlickr library to perform some basic listing functions for photos in Flickr.
Before you start, you should sign up for a free Flickr account, or use an existing one. Once you have the account, you need to sign up for an API key. You can go to Your Account, and select the Extending Flickr tab. After filling in a short form, you should be given two keys—API key and secret key. We will use these in all Flickr operations. We will not go through the steps required for integration into extensions, and will leave this exercise to the reader. The code we present can be used in both frontend plugins and backend modules. As was previously mentioned, we will be using the phpFlickr library. Go to http://phpflickr.com/ to download the latest version of the library and read the complete documentation.
require_once("phpFlickr.php");
$flickrService = new phpFlickr('api-key');
$photos = $flickrService->people_getPublicPhotos('7542705@N08');
foreach ($photos['photos']['photo'] as $photo) {
$imgURL = $flickrService->buildPhotoURL($photo, 'thumbnail');
print '<a href="http://www.flickr.com/photos/' .
$photo['owner'] . '/' . $photo['id'] . '">' .
'<img src="' . $imgURL . '" /></a><br />';
}
The Flickr API is exposed as a set of REST services, which we can issue calls to. The tough work of signing the requests and parsing the results is encapsulated by phpFlickr, so we don't have to worry about it. Our job is to gather the parameters, issue the request, and process the response. In the example above, we got a list of public photos from a user 7542705@N08. You may not know the user ID of the person you want to get photos for, but Flickr API offers several methods for finding the ID:
$userID = $flickrService->people_findByEmail($email);
$userID = $flickrService->people_findByUsername($username);
If you have the user ID, but want to get more information about the user, you can do it with the following calls:
// Get more info about the user:
$flickrService->people_getInfo($userID);
// Find which public groups the user belongs to:
$flickrService->people_getPublicGroups($userID);
// Get user's public photos:
$flickrService->people_getPublicPhotos($userID);
We utilize the people_getPublicPhotos method to get the user's photostream. The returned array has the following structure:
Array
(
[photos] => Array
(
[page] => 1
[pages] => 8
[perpage] => 100
[total] => 770
[photo] => Array
(
[0] => Array
(
[id] => 3960430648
[owner] => 7542705@N08
[secret] => 9c4087aae3
[server] => 3423
[farm] => 4
[title] => One Cold Morning
[ispublic] => 1
[isfriend] => 0
[isfamily] => 0
)
[…]
)
)
)
We loop over the $photos['photos']['photo'] array, and for each image, we build a URL for the thumbnail using the buildPhotoURL method, and a link to the image page on Flickr.
There are lots of other things we can do, but we will only cover a few basic operations.
Occasionally, you might encounter an output you do not expect. It's possible that the Flickr API returned an error, but by default, it's not shown to the user. You need to call the following functions to get more information about the error:
$errorCode = $flickrService->getErrorCode();
$errorMessage = $flickrService->getErrorMsg();
You can get a list of the most recent photos uploaded to Flickr using the following call:
$recentPhotos = $flickrService->photos_getRecent();
In this recipe, we will take a look at how to upload files to Flickr, as well as how to access other authenticated operations. Although many operations don't require authentication, any interactive functions do. Once you have successfully authenticated with Flickr, you can upload files, leave comments, and make other changes to the data stored in Flickr that you wouldn't be allowed to do without authentication.
If you followed the previous example, you should have everything ready to go. We'll assume you have the $flickrService object instantiated ready.
$frob = t3lib_div::_GET('frob');
if (empty($frob)) {
$flickrService->auth('write', false);
} else {
$flickrService->auth_getToken($frob);
}
$flickrService->sync_upload($filePath);
Flickr applications can access any user's data if the user authorizes them. For security reasons, users are redirected to Yahoo! to log into their account, and confirm access for your application. Once your application is authorized by a user, a token is stored in Flickr, and can be retrieved at any other time.
$flickrService->auth() requests permissions for the application. If the application is not yet authorized by the user, he/she will be redirected to Flickr. After giving the requested permissions, Flickr will redirect the user to the URL defined in the API key settings.
The redirected URL will contain a parameter frob. If present, $flickrService->auth_getToken($frob); is executed to get the token and store it in session. Future calls within the session lifetime will not require further calls to Flickr. If the session is expired, the token will be requested from Flickr service, transparent to the end user.
Successful authentication allows you to access other operations that you would not be able to access using regular authentication.
There are different levels of permissions that the service can request. You should not request more permissions than your application will use.
API call
|
Permission level
|
$flickrService->auth('read', false);
|
Permissions to read users' files, sets, collections, groups, and more.
|
$flickrService->auth('write', false);
|
Permissions to write (upload, create new, and so on).
|
$flickrService->auth('delete', false);
|
Permissions to delete files, groups, associations, and so on.
|
There are two functions that perform a file upload:
$flickrService->sync_upload($filePath);
$flickrService->async_upload($filePath);
The first function continues execution only after the file has been accepted and processed by Flickr. The second function returns after the file has been submitted, but not necessarily processed.
Why would you use the asynchronous method? Flickr service may have a large queue of uploaded files waiting to be processed, and your application might timeout while it's waiting. If you don't need to access the uploaded file right after it was uploaded, you should use the asynchronous method.
In this recipe, we will make use of our knowledge of the Flickr API and the phpFlickr interface to build a Flickr upload service into DAM. We will create a new action class, which will add our functionality into a DAM file list and context menus.
For simplicity, we will skip the process of creating the extension. You can download the extension dam_flickr_upload and view the source code. We will examine it in more detail in the How it works... section.
You're likely to experience trouble with the callback URL if you're doing it on a local installation with no public URI.
Let's examine in detail how the extension works. First, examine the file tree. The root contains the now familiar ext_tables.php and ext_conf_template.txt files.The Res directory contains icons used in the DAM. The Lib directory contains the phpFlickr library. The Mod1 directory contains the module for uploading.
This file contains the global extension configuration variables. The two variables defined in this file are the Flickr API key and the Flickr secret key. Both of these are required to upload files.
As was mentioned previously, ext_tables.php is a configuration file that is loaded when the TYPO3 framework is initializing.
tx_dam::register_action ('tx_dam_action_flickrUpload', 'EXT:dam_
flickr_upload/class.tx_dam_flickr_upload_action.php:&tx_dam_flickr_
upload_action_flickrUpload');
This line registers a new action in DAM. Actions are provided by classes extending the tx_dam_actionbase class, and define operations that can be performed on files and directories. Examples of actions include view, cut, copy, rename, delete, and more. The second parameter of the function defines where the action class is located.
$GLOBALS['TYPO3_CONF_VARS']['EXTCONF']['dam_flickr_upload']
['allowedExtensions'] = array('avi', 'wmv', 'mov', 'mpg', 'mpeg',
'3gp', 'jpg', 'jpeg', 'tiff', 'gif', 'png');
We define an array of file types that can be uploaded to Flickr. This is not hardcoded in the extension, but stored in ext_tables.php, so that it can be overwritten by extensions wanting to limit or expand the functionality to other file types.