










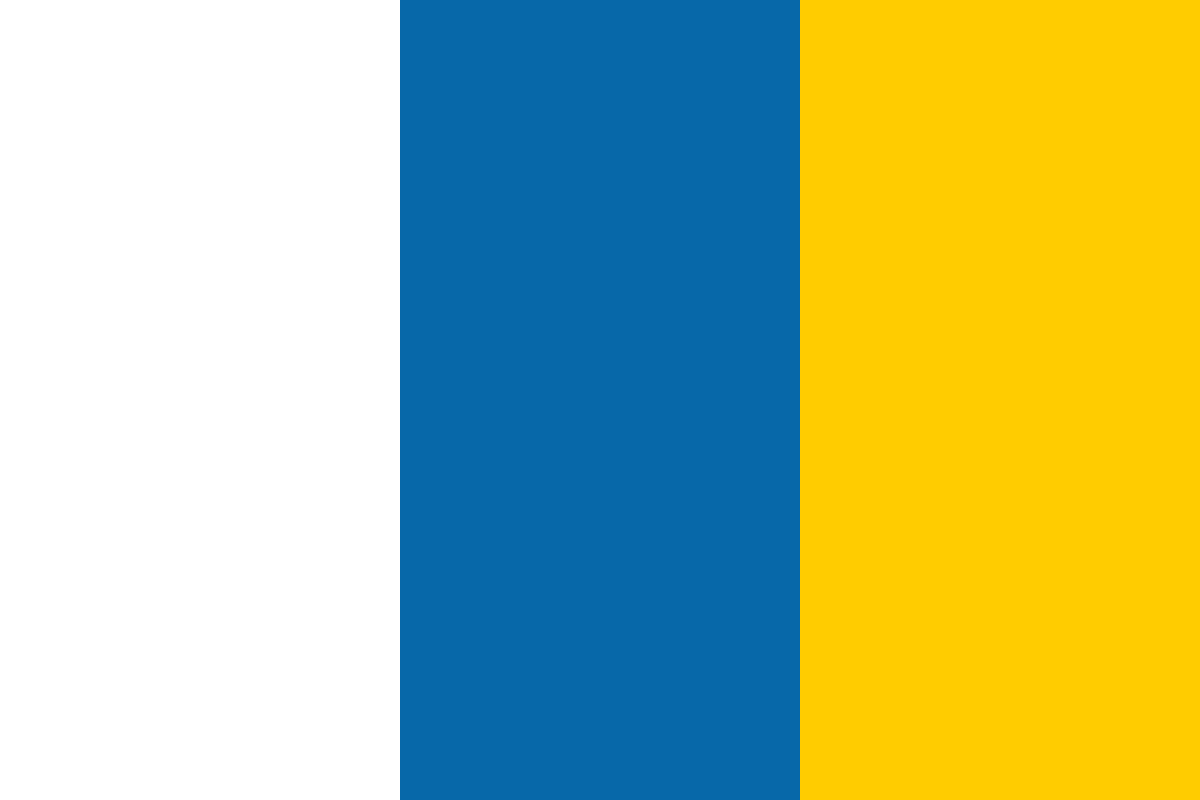

































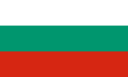








In order to get started, we will make a directory to store our code for this chapter. We'll make one on the desktop using mkdir and we'll call this directory node-tests:
mkdir node-tests
Then we'll change directory inside it using cd, so we can go ahead and run npm init. We'll
be installing modules and this will require a package.json file:
cd node-tests
npm init
We'll run npm init using the default values for everything, simply hitting enter throughout every single step:
Now once that package.json file is generated, we can open up the directory inside Atom. It's on the desktop and it's called node-tests.
From here, we're ready to actually define a function we want to test. The goal in this section is to learn how to set up testing for a Node project, so the actual functions we'll be testing are going to be pretty trivial, but it will help illustrate exactly how to set up our tests.
To get started, let's make a fake module. This module will have some functions and we'll test those functions. In the root of the project, we'll create a brand new directory and I'll call this directory utils:
We can assume this will store some utility functions, such as adding a number to another number, or stripping out whitespaces from a string, anything kind of hodge-podge that doesn't really belong to any specific location. We'll make a new file in the utils folder called utils.js, and this is a similar pattern to what we did when we created the weather and location directories in our weather app:
You're probably wondering why we have a folder and a file with the same name. This will be clear when we start testing.
Now before we can write our first test case to make sure something works, we need something to test. I'll make a very basic function that takes two numbers and adds them together. We'll create an adder function as shown in the following code block:
module.exports.add = () => {
}
This arrow function (=>) will take two arguments, a and b, and inside the function, we'll
return the value a + b. Nothing too complex here:
module.exports.add = () => {
return a + b;
};
Now since we just have one expression inside our arrow function (=>) and we want to return it, we can actually use the arrow function (=>) expression syntax, which lets us add our expression as shown in the following code, a + b, and it'll be implicitly returned:
module.exports.add = (a, b) => a + b;
There's no need to explicitly add a return keyword on to the function. Now that we have utils.js ready to go, let's explore testing.
We'll be using a framework called Mocha in order to set up our test suite. This will let us configure our individual test cases and also run all of our test files. This will be really important for creating and running tests. The goal here is to make testing simple and we'll use Mocha to do just that. Now that we have a file and a function we actually want to test, let's explore how to create and run a test suite.
We'll be doing the testing using the super popular testing framework Mocha, which you can find at mochajs.org. This is a fantastic framework for creating and running test suites. It's super popular and their page has all the information you'd ever want to know about setting it up, configuring it, and all the cool bells and whistles it has included:
If you scroll down on this page, you'll be able to see a table of contents:
Here you can explore everything Mocha has to offer. We'll be covering most of it in this article, but for anything we don't cover, I do want to make you aware you can always learn about it on this page.
Now that we've explored the Mocha documentation page, let's install it and start using it. Inside the Terminal, we'll install Mocha. First up, let's clear the Terminal output. Then we'll install it using the npm install command. When you use npm install, you can also use the shortcut npm i. This has the exact same effect. I'll use npm i with mocha, specifying the version @3.0.0. This is the most recent version of the library as of this filming:
npm i mocha@3.0.0
Now we do want to save this into the package.json file. Previously, we've used the save flag, but we'll talk about a new flag, called save-dev. The save-dev flag is will save this package for development purposes only—and that's exactly what Mocha will be for. We don't actually need Mocha to run our app on a service like Heroku. We just need Mocha locally on our machine to test our code.
When you use the save-dev flag, it installs the module much the same way:
npm i mocha@5.0.0 --save-dev
But if you explore package.json, you'll see things are a little different. Inside our package.json file, instead of a dependencies attribute, we have a devDependencies attribute:
In there we have Mocha, with the version number as the value. The devDependencies are fantastic because they're not going to be installed on Heroku, but they will be installed locally. This will keep the Heroku boot times really, really quick. It won't need to install modules that it's not going to actually need. We'll be installing both devDependencies and dependencies in most of our projects from here on out.
Now that we have Mocha installed, we can go ahead and create a test file. In the utils folder, we'll make a new file called utils.test.js:
This file will store our test cases. We'll not store our test cases in utils.js. This will be our application code. Instead, we'll make a file called utils.test.js. When we use this test.js extension, we're basically telling our app that this will store our test cases. When Mocha goes through our app looking for tests to run, it should run any file with this Extension.
Now we have a test file, the only thing left to do is create a test case. A test case is a function that runs some code, and if things go well, great, the test is considered to have passed. And if things do not go well, the test is considered to have failed. We can create a new test case, using it. It is a function provided by Mocha. We'll be running our project test files through Mocha, so there's no reason to import it or do anything like that. We simply call it just like this:
it();
Now it lets us define a new test case and it takes two arguments. These are:
First up, we'll have a string description of what exactly the test is doing. If we're testing that
the adder function works, we might have something like:
it('should add two numbers');
Notice here that it plays into the sentence. It should read like this, it should add two numbers; describes exactly what the test will verify. This is called behavior-driven development, or BDD, and that's the principles that Mocha was built on.
Now that we've set up the test string, the next thing to do is add a function as the second argument:
it('should add two numbers', () => {
});
Inside this function, we'll add the code that tests that the add function works as expected. This means it will probably call add and check that the value that comes back is the appropriate value given the two numbers passed in. That means we do need to import the util.js file up at the top. We'll create a constant, call utils, setting it equal to the return result from requiring utils. We're using ./ since we will be requiring a local file. It's in the same directory so I can simply type utils without the js extension as shown here:
const utils = require('./utils');
it('should add two numbers', () => {
});
Now that we have the utils library loaded in, inside the callback we can call it. Let's make a
variable to store the return results. We'll call this one results. And we'll set it equal to
utils.add passing in two numbers. Let's use something like 33 and 11:
const utils = require('./utils');
it('should add two numbers', () => {
var res = utils.add(33, 11);
});
We would expect it to get 44 back. Now at this point, we do have some code inside of our test suites so we run it. We'll do that by configuring the test script.
Currently, the test script simply prints a message to the screen saying that no tests exist. What we'll do instead is call Mocha. As shown in the following code, we'll be calling Mocha, passing in as the one and only argument the actual files we want to test. We can use a globbing pattern to specify multiple files. In this case, we'll be using ** to look in every single directory. We're looking for a file called utils.test.js:
"scripts": {
"test": "mocha **/utils.test.js"
},
Now this is a very specific pattern. It's not going to be particularly useful. Instead, we can swap out the file name with a star as well. Now we're looking for any file on the project that has a file name ending in .test.js:
"scripts": {
"test": "mocha **/*.test.js"
},
And this is exactly what we want. From here, we can run our test suite by saving package.json and moving to the Terminal. We'll use the clear command to clear the Terminal output and then we can run our test script using command shown as follows:
npm test
When we run this, we'll execute that Mocha command:
It'll go off. It'll fetch all of our test files. It'll run all of them and print the results on the screen inside Terminal as shown in the preceding screenshot. Here we can see we have a green checkmark next to our test, should add two numbers. Next, we have a little summary, one passing test, and it happened in 8 milliseconds.
It'll go off. It'll fetch all of our test files. It'll run all of them and print the results on the
screen inside Terminal as shown in the preceding screenshot. Here we can see we have a
green checkmark next to our test, should add two numbers. Next, we have a little
summary, one passing test, and it happened in 8 milliseconds.
Now in our case, we don't actually assert anything about the number that comes back. It could be 700 and we wouldn't care. The test will always pass. To make a test fail what we have to do is throw an error. That means we can throw a new error and we pass into the constructor function whatever message we want to use as the error as shown in the following code block. In this case, I could say something like Value not correct:
const utils = require('./utils');
it('should add two numbers', () => {
var res = utils.add(33, 11);
throw new Error('Value not correct')
});
Now with this in place, I can save the test file and rerun things from the Terminal by rerunning npm test, and when we do that now we have 0 tests passing and we have 1 test failing:
Next we can see the one test is should add two numbers, and we get our error message, Value not correct. When we throw a new error, the test fails and that's exactly what we want to do for add.
Now, we'll create an if statement for the test. If the response value is not equal to 44, that means we have a problem on our hands and we'll throw an error:
const utils = require('./utils');
it('should add two numbers', () => {
var res = utils.add(33, 11);
if (res != 44){
}
});
Inside the if condition, we can throw a new error and we'll use a template string as our message string because I do want to use the value that comes back in the error message. I'll say Expected 44, but got, then I'll inject the actual value, whatever happens to come back:
const utils = require('./utils');
it('should add two numbers', () => {
var res = utils.add(33, 11);
if (res != 44){
throw new Error(`Expected 44, but got ${res}.`);
}
});
Now in our case, everything will line up great. But what if the add method wasn't working correctly? Let's simulate this by simply tacking on another addition, adding on something like 22 in utils.js:
module.exports.add = (a, b) => a + b + 22;
I'll save the file, rerun the test suite:
Now we get an error message: Expected 44, but got 66. This error message is fantastic. It lets us know that something is going wrong with the test and it even tells us exactly what we got back and what we expected. This will let us go into the add function, look for errors, and hopefully fix them.
Creating test cases doesn't need to be something super complex. In this case, we have a simple test case that tests a simple function.
To summarize, we looked into basic testing of a node app. We explored the testing framework, Mocha which can be used for creating and running test suites.
You read an excerpt from a book written by Andrew Mead, titled Learning Node.js Development. In this book, you will learn how to build, deploy, and test Node apps.
Developing Node.js Web Applications
How is Node.js Changing Web Development?