










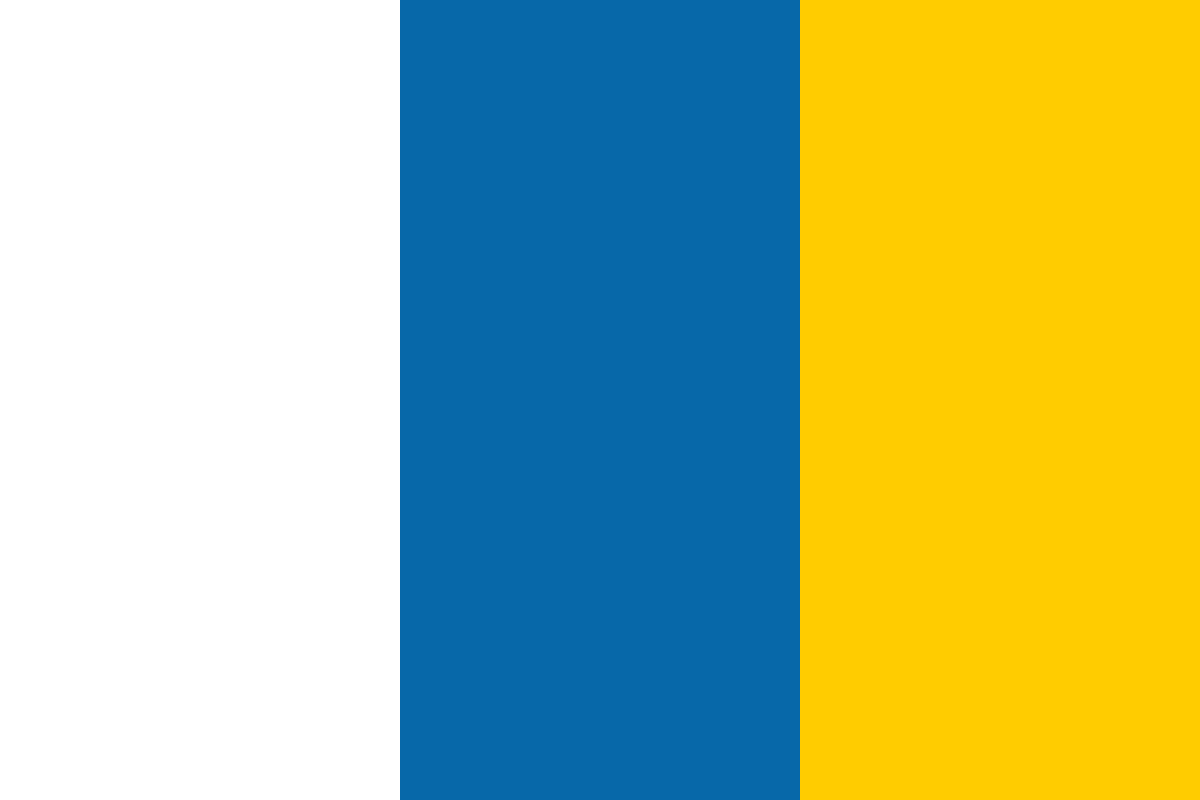

































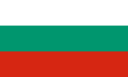








The UI tabs widget is used to toggle visibility across a set of different elements, each element containing content that can be accessed by clicking on its heading which appears as an individual tab. The tabs are structured so that they line up next to each other, whereas the content sections are layered on top of each other, with only the top one visible. Clicking a tab will highlight the tab and bring its associated content panel to the top of the stack. Only one content panel can be open at a time.
The following screenshot shows the different components of a set of UI tabs:
The structure of the underlying HTML elements, on which tabs are based, is rigid and widgets require a certain number of elements for them to work.
The tabs must be created from a list element (ordered or unordered) and each list item must contain an <a> element. Each link will need to have a corresponding element with a specified id that it is associated with the link's href attribute. We'll clarify the exact structure of these elements after our first example.
In a new file in your text editor, create the following page:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"
"http://www.w3.org/TR/html4/strict.dtd">
<html lang="en">
<head>
<link rel="stylesheet" type="text/css"
href="development-bundle/themes/smoothness/ui.all.css">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>jQuery UI Tabs Example 1</title>
</head>
<body>
<div id="myTabs">
<ul>
<li><a href="#a">Tab 1</a></li>
<li><a href="#b">Tab 2</a></li>
</ul>
<div id="a">This is the content panel linked to the first tab,
it is shown by default.</div>
<div id="b">This content is linked to the second tab and
will be shown when its tab is clicked.</div>
</div>
<script type="text/javascript" src="development-bundle/jquery-1.3.2.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.core.js"></script>
<script type="text/javascript" src="development-bundle/ui/ui.tabs.js"></script>
<script type="text/javascript">
$(function(){
$("#myTabs").tabs();
});
</script>
</body>
</html>
Save the code as tabs1.html in your jqueryui working folder. Let's review what was used. The following script and CSS resources are needed for the default tab widget instantiation:
A tab widget consists of several standard HTML elements arranged in a specific manner (these can be either hardcoded into the page, or added dynamically, or can be a mixture of both depending on the requirements of your implementation).
The first two elements within the outer container make the clickable tab headings, which are used to show the content section that is associated with the tab. Each tab should include a list item containing the link.
The href attribute of the link should be set as a fragment identifier, prefixed with #. It should match the id attribute of the element that forms the content section, with which it is associated. The content sections of each tab are created by the elements. The id attribute is required and will be targeted by its corresponding element. We've used elements in this example as the content panels for each tab, but other elements, such as elements can also be used. The elements discussed so far, along with their required attributes, are the minimum that are required from the underlying markup.We link to several <script> resources from the library at the bottom of the <body> before its closing tag. Loading scripts last, after stylesheets and page elements is a proven technique for improving performance. After linking first to jQuery, we link to the ui.core.js file that is required by all components (except the effects, which have their own core file). We then link to the component's source file that in this case is ui.tabs.js.After the three required script files from the library, we can turn to our custom < script> element in which we add the code that creates the tabs. We simply use the $(function(){}); shortcut to execute our code when the document is ready.
We then call the tabs() widget method on the jQuery object, representing our tabs container element (the <ul> with an id of myTabs).When we run this file in a browser, we should see the tabs as they appeared in the first screenshot of this article(without the annotations of course).
Using Firebug for Firefox (or another generic DOM explorer) we can see that a variety of class names are added to the different underlying HTML elements that the tabs widget is created from, as shown in the following screenshot:
Let's review these briefly. To the outer container < div> the following class names are added:
Class name |
Purpose |
ui-tabs |
Allows tab-specific structural CSS to be applied. |
ui-widget |
Sets generic font styles that are inherited by nested elements. |
ui-widget-content |
Provides theme-specific styles. |
ui-corner-all |
Applies rounded corners to container. |
The first element within the container is the < ul> element. This element receives the following class names:
Class name |
Purpose |
ui-tabs-nav |
Allows tab-specific structural CSS to be applied. |
ui-helper-reset |
Neutralizes browser-specific styles applied to <ul> elements. |
ui-helper-clearfix |
Applies the clear-fix as this element has children that are floated. |
ui-widget-header |
Provides theme-specific styles. |
ui-corner-all |
Applies rounded corners. |
The individual < li> elements are given the following class names:
Class name |
Purpose |
ui-state-default |
Applies theme-specific styles. |
ui-corner-top |
Applies rounded corners to the top edges of the elements. |
ui-tabs-selected |
This is only applied to the active tab. On page load of the default implementation this will be the first tab. Selecting another tab will remove this class from the currently selected tab and apply it to the new tab. |
ui-state-active |
Applies theme-specific styles to the currently selected tab. This class name will be added to the tab that is currently selected, just like the previous class name. The reason there are two class names is that ui-tabs-selected provides the functional CSS, while ui-state-active provides the visual, decorative styles. |
The < a> elements within each < li> are not given any class names, but they still have both structural and theme-specific styles applied to them by the framework.
Finally, the elements that hold each tab's content are given the following class names:
Class name |
Purpose |
ui-tabs-panel |
Applies structural CSS to the content panels |
ui-widget-content |
Applies theme-specific styles |
ui-corner-bottom |
Applied rounded corners to the bottom edges of the content panels |
All of these classes are added to the underlying elements automatically by the library, we don't need to manually add them when coding the page.
As these tables illustrate, the CSS framework supplies the complete set of both structural CSS styles that control how the tabs function and theme-specific styles that control how the tabs appear, but not how they function. We can easily see which selectors we'll need to override if we wish to tweak the appearance of the widget, which is what we'll do in the following section.