










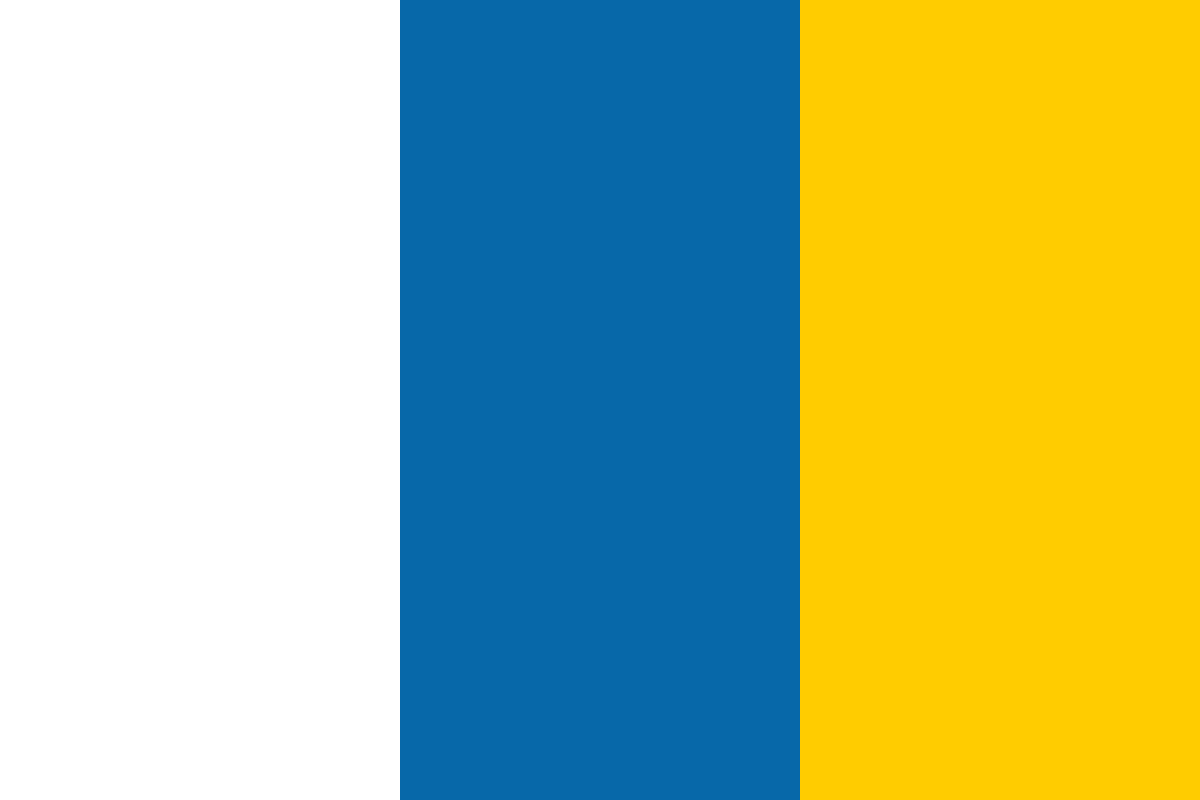

































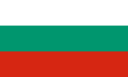








(For more resources on JavaScript, see here.)
So without further ado, let us get started with a lighter topic—the difference between validating and testing.
There's a thin line separating validating and testing. If you have some idea about sets (as in sets from mathematics), I would say that validation can lead to better testing results, while testing does not necessarily lead to a valid code.
Let us consider the scenario—you wrote a JavaScript program and tested it on major browsers such as the Internet Explorer and Firefox; and it worked. In this case, you have tested the code to make sure that it is functional.
However, the same code that you have created may or may not be valid; valid code is akin to writing a code that has the following characteristics:
There may come a point in time where you will notice that good coding style is highly subjective—there are various validators that may have different opinions or standards as to what is known as "good coding style". Therefore, if you do use different validators to validate your code, do not freak out if you see different advice for your coding style.
This does not mean that valid code leads to code that is functional (as you will see later) and that code that is functional leads to validated code as both have different standards for comparison.
However, valid code often leads to less errors, and code that is both functional and valid is often quality code. This is due to the fact that writing a piece of JavaScript code, that is both valid and correct, is much more difficult than just writing a code that is correct.
Testing often means that we are trying to get the code working correctly; while validation is making sure that the code is syntactically correct, with good style and that it meets the specification of the language. While good coding styles may be subjective, there is often a coding style that is accepted by most programmers, such as, making sure that the code is properly commented, indented, and there is no pollution of the global namespace (especially in the case of JavaScript).
To make the case clearer, following are three situations that you can consider:
This form of errors would most probably be caused by logic errors in JavaScript. Logic errors can be syntactically correct but they may be logically flawed.
A classic example would be an infinite for loop or infinite while loop.
This would most probably be the case for most functional code; a piece of JavaScript may be functionally correct and working, but it may be invalid. This may be due to poor coding style or any other characteristics in a valid code that are missing.
Later on in this article, you will see a full working example of a piece of JavaScript code that is right but invalid.
In this case, the code error can be caused by all three forms of JavaScript errors that are mentioned in the article such as loading errors, runtime errors, and logic errors. While it is more likely that errors caused by syntax errors might be spotted by good validators, it is also possible that some errors are buried deep inside the code, such that it is difficult to spot them using manual methods.
Now that we have some common understanding as to what validation and testing is about, let us move on to the next section which discusses the issues surrounding quality code.
While there are many views as to what is quality code, I personally believe that there are a few agreed standards. Some of the most commonly mentioned standards may include code readability, ease of extension, efficiency, good coding style, and meeting language specifications, and so on.
For our purpose here, we will focus on the factors that make a piece of code valid—coding style and meeting specifications. In general, good coding style almost guarantees that the code is highly readable (even to third parties) and this will help us to spot errors manually.
Most importantly, having a good coding style allows us to quickly understand the code, specially if we need to work in teams or are required to debug the code on our own.
You will notice that we will focus on the importance of code validity for testing purposes in later parts of the article. But now, let us start with the first building block of quality code—valid HTML and CSS.
We have a common understanding that JavaScript breathes life into a web page by manipulating the Document Object Model (DOM) of the HTML documents. This means that the DOM must be present in the code before JavaScript can operate on it.
Here's an important fact that is directly related to HTML, CSS, and browsers—browsers are generally forgiving towards invalid HTML and CSS code as compared to compilers for languages like C or Python. This is because, all browsers have to do is parse the HTML and CSS so as to render the web page for its browsers. On the other hand, compilers are generally unforgiving towards invalid code. Any missing tag, declarations, and so on will lead to a compilation error. Therefore, it is ok to write invalid or even buggy HTML and CSS, yet get a "usual" looking web page.
Based on the previous explanation, we should see that we would need to have valid HTML and CSS in order to create quality JavaScript code.
A short list of reasons, based on my personal experience, as to why valid HTML and CSS is an important prerequisite before you start working on JavaScript are as follows:
In short, one of the most important building blocks of creating quality JavaScript code is to have valid HTML and CSS.
You may disagree with me on the previous section as to why HTML and CSS should be valid. In general, validation helps you to prevent errors that are related to coding style and specifications. However, do take note that using different validators may give you different results since validators might have different standards in terms of code style.
In case you are wondering if invalid code can affect your JavaScript code, I would advise you to make your code as valid as possible; invalid code may lead to sticky issues such as cross-browser incompatibility, difficulty in reading code, and so on.
Invalidated code means that your code may not be foolproof; in the early days of the Internet, there were websites that were dependent on the quirks of the early Netscape browser. Back track to the time where the Internet Explorer 6 was widely used, there were also many websites that worked in quirks mode to support Internet Explorer 6.
Now, most browsers are supporting or are moving towards supporting web standards (though slightly different, they are supporting in subtle manners), writing valid code is one of the best ways to ensure that your website works and appears the way it is intended to.
While invalid code may not cause your code to be dysfunctional, valid code often simplifies testing. This is due to the focus on coding style and specifications; codes that are valid and have met specifications are typically more likely to be correct and much easier to debug. Consider the following code that is stylistically invalid:
function checkForm(formObj){
alert(formObj.id)
//alert(formObj.text.value);
var totalFormNumber = document.forms.length;
// check if form elements are empty and are digits
var maxCounter = formObj.length; // this is for checking for empty
values
alert(totalFormNumber);
// check if the form is properly filled in order to proceed
if(checkInput(formObj)== false){
alert("Fields cannot be empty and it must be digits!");
// stop executing the code since the input is invalid
return false;
}
else{
;
}
var i = 0;
var formID;
while(i < totalFormNumber){
if(formObj == document.forms[i]){
formID = i;alert(i);
}
i++;
}
if(formID<4){
formID++;
var formToBeChanged = document.forms[formID].id;
// alert(formToBeChanged);
showForm(formToBeChanged);
}
else{
// this else statement deals with the last form
// and we need to manipulate other HTML elements
document.getElementById("formResponse").style.visibility = "visible";
}
return false;
}
The previous code is an extreme example of poor code style, especially in terms of indentation. Imagine if you have to manually debug the second code snippet that you saw earlier! I am pretty sure that you will find it frustrating to check the code, because you will have little visual sense of what is going on.
More importantly, if you are working in a team, you will be required to write legible code; in short, writing valid code typically leads to code that is more legible, easier to follow, and hence, less erroneous.
As mentioned in the previous section, browsers are in general forgiving towards invalid HTML and CSS. While this is true, there may be errors that are not caught, or are not rendered correctly or gracefully. This means that while the invalid HTML and CSS code may appear fine on a certain platform or browser, it may not be supported on others.
This means that using valid code (valid code typically means standard code set by international organizations such as W3C) will give you a much greater probability of having your web page rendered correctly on different browsers and platforms.
With valid HTML and CSS, you can safely write your JavaScript code and expect it to work as intended, assuming that your JavaScript code is equally valid and error free.
Valid code typically requires coding using good practices. As mentioned frequently in this article, good practices include the proper enclosing of tags, suitable indentation to enhance code readability, and so on.
If you need more information about good practi ces when using JavaScript, feel free to check out the creator of JSLint, Douglas Crockford, at http://crockford.com.. Or you can read up John Resigs blog (the creator of JQuery) at http://ejohn.org. Both are great guys who know what great JavaScript is about.
To summarize the above sections, the DOM is provided by HTML, and both CSS and JavaScript are applied to the DOM. This means that if there is an invalid DOM, there is a chance that the JavaScript that is operating on the DOM (and sometimes the CSS) might result in errors.
With this summary in mind, we'll focus on how you can spot validation errors by using color coding editors.