










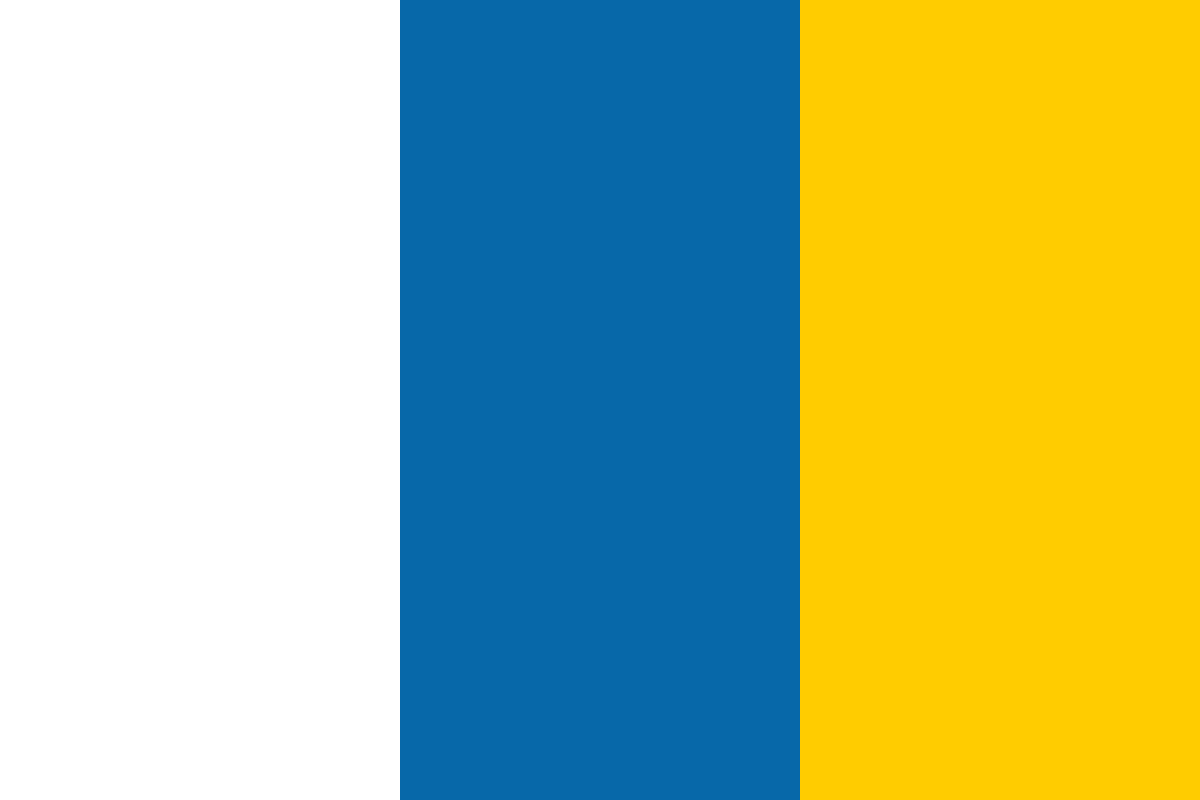

































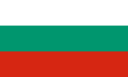








(For more resources related to this topic, see here.)
When developing an iOS game, we need to know which device to target. Besides the obvious technical differences between all of the iOS devices, there are two factors we need to actively take care of: screen size and texture size limit.
Let's take a closer look at how to deal with the texture size limit and screen sizes.
Every graphics card has a limit for the maximum size texture it can display. If a texture is bigger than the texture size limit, it can't be loaded and will appear black on the screen. A texture size limit has power-of-two dimensions and is a square such as 1024 pixels in width and in height or 2048 x 2048 pixels.
When loading a texture, they don't need to have power-of-two dimensions. In fact, the texture does not have to be a square. However, it is a best practice for a texture to have power-of-two dimensions.
This limit holds for big images as well as a bunch of small images packed into a big image. The latter is commonly referred to as a sprite sheet. Take a look at the following sample sprite sheet to see how it's structured:
While the screen size is always measured in pixels, the iOS coordinate system is measured in points.
The screen size of an iPhone 3GS is 320 x 480 pixels and also 320 x 480 points. On an iPhone 4, the screen size is 640 x 960 pixels, but is still 320 by 480 points. So, in this case, each point represents four pixels: two in width and two in height. A 100-point wide rectangle will be 200 pixels wide on an iPhone 4 and 100 pixels on an iPhone 3GS.
It works similarly for the devices with large display screens, such as the iPhone 5. Instead of 480 points, it's 568 points.
Let's explain the term viewport first: the viewport is the visible portion of the complete screen area.
We need to be clear about which devices we want our game to run on. We take the biggest resolution that we want to support and scale it down to a smaller resolution. This is the easiest option, but it might not lead to the best results; touch areas and the user interface scale down as well. Apple recommends for touch areas to be at least a 40-point square; so, depending on the user interface, some elements might get scaled down so much that they get harder to touch.
Take a look at the following screenshot, where we choose the iPad Retina resolution (2048 x 1536 pixels) as our biggest resolution and scale down all display objects on the screen for the iPad resolution (1024 x 768 pixels):
Scaling is a popular option for non-iOS environments, especially for PC and Mac games that support resolutions from 1024 x 600 pixels to full HD.
Sparrow and the iOS SDK provide some mechanisms that will facilitate handling Retina and non-Retina iPad devices without the need to scale the whole viewport.
Some games in the past have been designed for a 4:3 resolution display but then made to run on a widescreen device that had more screen space.
So, the option was to either scale a 4:3 resolution to widescreen, which will distort the whole screen, or put some black borders on either side of the screen to maintain the original scale factor.
Showing black borders is something that is now considered as bad practice, especially when there are so many games out there which scale quite well across different screen sizes and platforms.
If our pirate game is a multiplayer, we may have a player on an iPad and another on an iPhone 5. So, the player with the iPad has a bigger screen and more screen space to maneuver their ship. The worst case will be if the player with the iPad is able to move their ship outside the visual range for the iPhone player to see, which will result in a serious advantage for the iPad player.
Luckily for us, we don't require competitive multiplayer functionality. Still, we need to keep a consistent screen space for players to move their ship in for game balance purposes. We wouldn't want to tie the difficulty level to the device someone is playing on.
Let's compare the previous screenshot to the black border example. Instead of the ugly black borders, we just show more of the background.
In some cases, it's also possible to move some user interface elements to the areas which are not visible on other devices. However, we will need to consider whether we want to keep the same user experience across devices and whether moving these elements will result in a disadvantage for users who don't have this extra screen space on their devices.
Rearranging screen elements is probably the most time-intensive and sophisticated way of solving this issue. In this example, we have a big user interface at the top of the screen in the portrait mode. Now, if we were to leave it like this in the landscape mode, the top of the screen will be just the user interface, leaving very little room for the game itself.
In this case, we have to be deliberate about what kind of elements we need to see on the screen and which elements are using up too much screen estate. Screen real estate (or screen estate) is the amount of space available on a display for an application or a game to provide output. We will then have to reposition them, cut them up in to smaller pieces, or both.
The most prominent example of this technique is Candy Crush (a popular trending game) by King. While this concept applies particularly to device rotation, this does not mean that it can't be used for universal applications.
None of these options are mutually exclusive. For our purposes, we are going to show non-interactive screen space, and if things get complicated, we might also resort to rearranging screen elements depending on our needs.
Let's take a look at the differences in the screen size and the texture size limit between the different iOS devices:
Device |
Screen size (in pixels) |
Texture size limit (in pixels) |
iPhone 3GS |
480 x 360 |
2048 x 2048 |
iPhone 4 (including iPhone 4S) and iPod Touch 4th generation |
960 x 640 |
2048 x 2048 |
iPhone 5 (including iPhone 5C and iPhone 5S) and iPod Touch 5th generation |
1136 x 640 |
2048 x 2048 |
iPad 2 |
1024 x 768 |
2048 x 2048 |
iPad (3rd and 4th generations) and iPad Air |
2048 x 1536 |
4096 x 4096 |
iPad Mini |
1024 x 768 |
4096 x 4096 |
Both the iOS SDK and Sparrow can aid us in creating a universal application. Universal application is the term for apps that target more than one device, especially for an app that targets the iPhone and iPad device family.
The iOS SDK provides a handy mechanism for loading files for specific devices. Let's say we are developing an iPhone application and we have an image that's called my_amazing_image.png. If we load this image on our devices, it will get loaded—no questions asked. However, if it's not a universal application, we can only scale the application using the regular scale button on iPad and iPhone Retina devices. This button appears on the bottom-right of the screen.
If we want to target iPad, we have two options:
The first option is to load the image as is. The device will scale the image. Depending on the image quality, the scaled image may look bad. In this case, we also need to consider that the device's CPU will do all the scaling work, which might result in some slowdown depending on the app's complexity.
The second option is to add an extra image for iPad devices. This one will use the ~ipad suffix, for example, my_amazing_image~ipad.png. When loading the required image, we will still use the filename my_amazing_image.png. The iOS SDK will automatically detect the different sizes of the image supplied and use the correct size for the device.
Beginning with Xcode 5 and iOS 7, it is possible to use asset catalogs. Asset catalogs can contain a variety of images grouped into image sets. Image sets contain all the images for the targeted devices. These asset catalogs don't require files with suffixes any more. These can only be used for splash images and application icons. We can't use asset catalogs for textures we load with Sparrow though.
The following table shows which suffix is needed for which device:
Device |
Retina |
File suffix |
iPhone 3GS |
No |
None |
iPhone 4 (including iPhone 4S) and iPod Touch (4th generation) |
Yes |
@2x @2x~iphone |
iPhone 5 (including iPhone 5C and iPhone 5S) and iPod Touch (5th generation) |
Yes |
-568h@2x |
iPad 2 |
No |
~ipad |
iPad (3rd and 4th generations) and iPad Air |
Yes |
@2x~ipad |
iPad Mini |
No |
~ipad |
How does this affect the graphics we wish to display? The non-Retina image will be 128 pixels in width and 128 pixels in height. The Retina image, the one with the @2x suffix, will be exactly double the size of the non-Retina image, that is, 256 pixels in width and 256 pixels in height.
Sparrow supports all the filename suffixes shown in the previous table, and there is a special case for iPad devices, which we will take a closer look at now.
When we take a look at AppDelegate.m in our game's source, note the following line:
[_viewController startWithRoot:[Game class] supportHighResolutions:YES
doubleOnPad:YES];
The first parameter, supportHighResolutions, tells the application to load Retina images (with the @2x suffix) if they are available.
The doubleOnPad parameter is the interesting one. If this is set to true, Sparrow will use the @2x images for iPad devices. So, we don't need to create a separate set of images for iPad, but we can use the Retina iPhone images for the iPad application.
In this case, the width and height are 512 and 384 points respectively. If we are targeting iPad Retina devices, Sparrow introduces the @4x suffix, which requires larger images and leaves the coordinate system at 512 x 384 points.
If we are talking about images of different sizes for the actual game content, app icons and splash images are also required to be in different sizes.
Splash images (also referred to as launch images) are the images that show up while the application loads. The iOS naming scheme applies for these images as well, so for Retina iPhone devices such as iPhone 4, we will name an image as Default@2x.png, and for iPhone 5 devices, we will name an image as Default-568h@2x.png.
For the correct size of app icons, take a look at the following table:
Device |
Retina |
App icon size |
iPhone 3GS |
No |
57 x 57 pixels |
iPhone 4 (including iPhone 4S) and iPod Touch 4th generation |
Yes |
120 x 120 pixels |
iPhone 5 (including iPhone 5C and iPhone 5S) and iPod Touch 5th generation |
Yes |
120 x 120 pixels |
iPad 2 |
No |
76 x 76 pixels |
iPad (3rd and 4th generation) and iPad Air |
Yes |
152 x 152 pixels |
iPad Mini |
No |
76 x 76 pixels |
The more devices we want to support, the more graphics we need, which directly increases the application file size, of course. Adding iPad support to our application is not a simple task, but Sparrow does some groundwork.
One thing we should keep in mind though: if we are only targeting iOS 7.0 and higher, we don't need to include non-Retina iPhone images any more. Using @2x and @4x will be enough in this case, as support for non-Retina devices will soon end.
This article deals with setting up our game to work on iPhone, iPod Touch, and iPad in the same manner.
Further resources on this subject: