










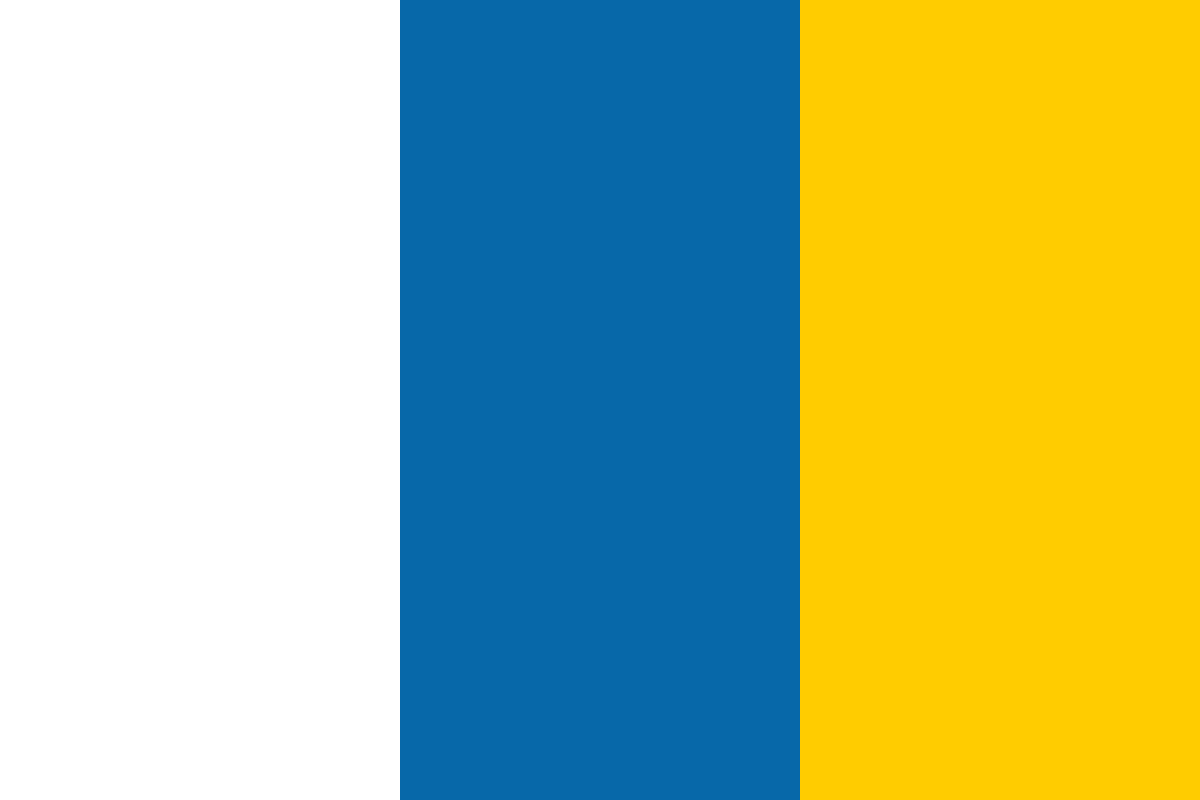

































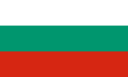








Depending on the interaction scenario in which a Web service is involved, it can either act as a service provider or a service requestor. In the following sections, you will see how to build a Web service provider and a requestor that will consume the service provider.
To start with, let's look at a simple example. Suppose that you need to implement an application based on Web services that performs the following sequence of steps:
In a real-world situation, to build such an application, you would have to design more than one service and pull these services together into a composite solution. However, for simplicity's sake, the example discussed here uses the only service to handle all of the above tasks.
Of course, the above service would be only a part of an entire real-world solution. A service requestor sending a PO document for processing to this service would act as a service provider itself towards another requestor, or would be part of a composite service built, for example, with WS-BPEL.
Diagrammatically, the scenario involving the PO Web service that performs the tasks described above might look like the following figure:
Here is the detailed explanation of the steps in the figure:
To build the PO Web service depicted in the previous figure, you need to accomplish the following five general steps:
The following sections take you through each of the above steps. First, you will see how to create a simple PO Web service that actually performs no validation. Then, you will learn how the XML Schema feature can be used with WSDL to define types in messages being transmitted, making sure that transmitted data is valid with respect to a specific XML schema.
B efore we go any further, let's take a moment to set up the database required for this example. This example assumes that you are using Oracle Database Express Edition (XE)—a free edition of Oracle Database, or any other edition of Oracle database.
You can download a copy of Oracle Database from the Download page of the Oracle Technology Network (OTN) Website at http://www.oracle.com/technology/software/index.html. For this particular example, Oracle is used because it provides native support for XML, which makes it easier for you to get the job done, allowing you to concentrate on using the PHP SOAP extension while building the application.
To keep things simple, this section actually discusses how to create a minimal set of the database objects required only to store incoming PO documents.
The Oracle XML Schema is part of the Oracle XML DB, which is a set of Oracle XML features available in any edition of Oracle Database by default.
With Oracle database, you have several options when it comes to creating, accessing and manipulating the database objects. You can use both the graphical and command-line tools shipped with Oracle Database. As for Oracle Database XE, you might use the Oracle Database XE graphical user interface, a browser-based tool that allows you to administer the database.
However, to create the database objects required for this example, it is assumed that you will make use of Oracle SQL*Plus, a command-line SQL tool, which is installed by default with every Oracle database installation.
With SQL*Plus, you interact with the database server by entering appropriate SQL statements at the SQL> prompt.
Assuming that you have an Oracle database server installed and running, launch SQL*Plus and then follow these steps:
Set up a database account that will be used as a container for the database objects by issuing the following SQL statements:
//connect to the database as sysdba to be able to create a new
account
CONN /as sysdba
//create a user identified as xmlusr with the same password
CREATE USER xmlusr IDENTIFIED BY xmlusr;
//grant privileges required to connect and create resources
GRANT connect, resource TO xmlusr;
Issue the following SQL statements to create a table that will be used to store PO XML documents:
//connect to the database using the newly created account
CONN xmlusr/xmlusr;
//create a purchaseOrders table to be used for storing POs
CREATE TABLE purchaseOrders(
doc VARCHAR2(4000));
As you can see, the purchaseOrders table created by the above statement contains only one column, namely doc of VARCHAR2. Using the VARCHAR2 Oracle data type is the simplest option when it comes to storing XML documents in an Oracle database. In fact, Oracle provides much more powerful options for storing XML data in the database.
N ow that you have set up the database to store the incoming PO documents, it's time to create the PHP code that will perform just that operation: storing incoming POs into the database. Consider the purchaseOrder PHP class containing the PO Web service underlying logic. It is assumed that you will save this class in the purchaseOrder.php file in the WebServicesch2 directory within the document directory of your Web server, so that it will be available at http://localhost/ WebServices/ch2/purchaseOrder.php.
<?php
//File purchaseOrder.php
class purchaseOrder {
function placeOrder($po) {
if(!$conn = oci_connect('xmlusr', 'xmlusr', '//localhost/xe')){
throw new SoapFault("Server","Failed to connect to
database");
};
$sql = "INSERT INTO purchaseOrders VALUES(:po)";
$query = oci_parse($conn, $sql);
oci_bind_by_name($query, ':po', $po);
if (!oci_execute($query)) {
throw new SoapFault("Server","Failed to insert PO");
};
$msg='<rsltMsg>PO inserted!</rsltMsg>';
return $msg;
}
}
?>
Looking through the code, you may notice that the purchaseOrder class actually contains the only method, namely placeOrder. As its name implies, the placeOrder method is responsible for placing an incoming PO document. What this method actually does is take a PO XML document as the parameter and then store it in the purchaseOrders table created in the preceding section. Upon failure to connect to the database or execute the INSERT statement, the placeOrder method stops execution and throws a SOAP exception.
Another important point to note here is that the placeOrder method doesn't contain any code required to validate an incoming PO document. For simplicity, this example assumes no validation for the moment.