










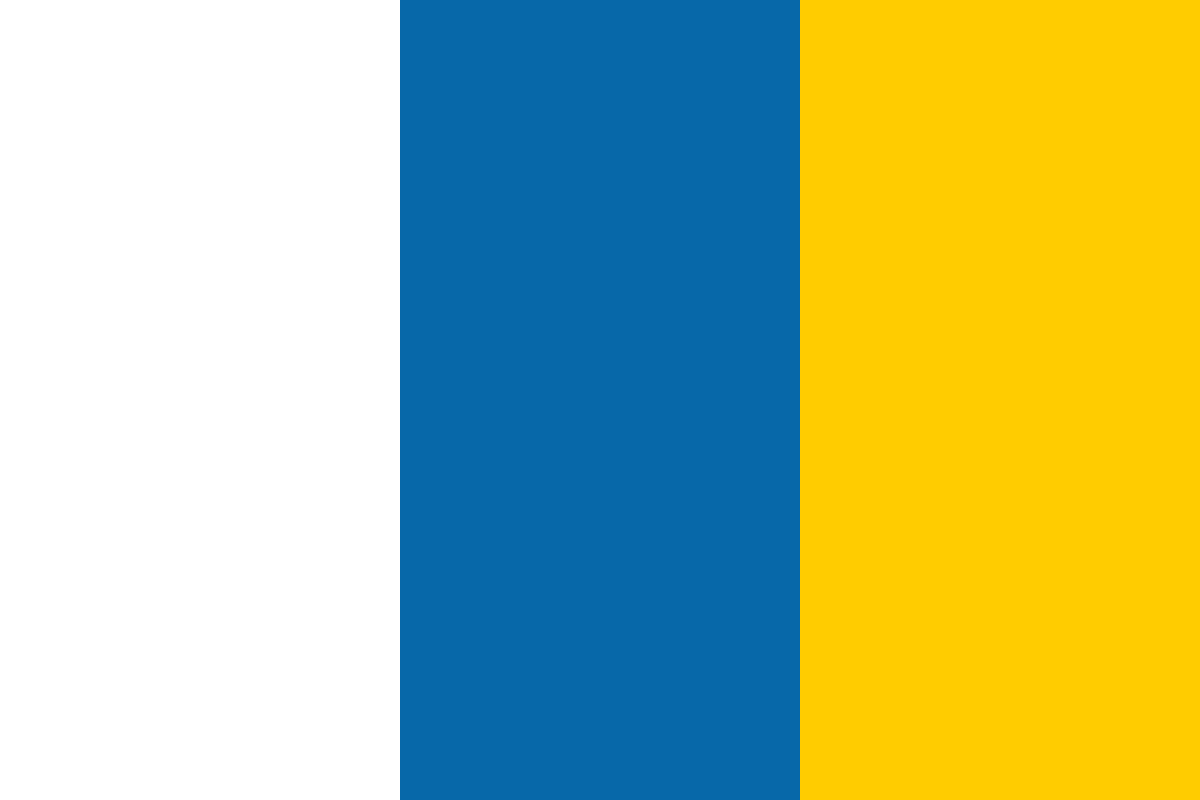

































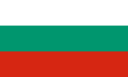








If you use Slack, you've probably added a handful of integrations for your team from the ever-growing App Directory, and maybe even had an idea for your own Slack app. While the Slack API is featureful and robust, writing your own integration can be exceptionally easy. Through the Slack RTM (Real Time Messaging) API, you can write our own basic integrations in just a few lines of Python using the SlackSocket library.
Want an accessible introduction to Python that's comprehensive enough to give you the confidence you need to dive deeper? This week, follow our Python Fundamentals course inside Mapt. It's completely free - so what have you got to lose?
Our integration will be structured with the following basic components:
The listener watches for one or more pre-defined "trigger" words, while the response posts the result of our intended task.
We'll start by setting up SlackSocket with our API token:
fromslacksocketimportSlackSocket
slack=SlackSocket('<slack-token>', event_filter=['message'])
By default, SlackSocket will listen for all Slack events. There are a lot of different events sent via RTM, but we're only concerned with 'message' events for our integration, so we've set an event_filter for only this type.
Using the SlackSocketevents() generator, we'll read each 'message' event that comes in and can act on various conditions:
for e inslack.events():
ife.event['text'] =='!hello':
slack.send_msg('it works!', channel_name=e.event['channel'])
If our message text matches the string '!hello', we'll respond to the source channel of the event with a given message('it works!').
At this point, we've created a complete integration that can connect to Slack as a bot user(or regular user), follow messages, and respond accordingly. Let's build something a bit more useful, like a password generator for throwaway accounts.
For this integration command, we'll write a simple function to generate a random alphanumeric string 15 characters long:
import random
import string
defrandomstr():
chars=string.ascii_letters+string.digits
return''.join(random.choice(chars) for _ inrange(15))
Now we're ready to provide our random string generator to the rest of the team using the same chat logic as before, responding to the source channel with our generated password:
for e inslack.events():
e.event['text'].startswith('!random'):
slack.send_msg(randomstr(), channel_name=e.event['channel'])
Altogether:
import random
import string
fromslacksocketimportSlackSocket
slack=SlackSocket('<slack-token>', event_filter=['message'])
defrandomstr():
chars=string.ascii_letters+string.digits
return''.join(random.choice(chars) for _ inrange(15))
for e inslack.events():
ife.event['text'].startswith('!random'):
slack.send_msg(randomstr(), channel_name=e.event['channel'])
And the results:
A complete integration in 10 lines of Python. Not bad!
Beyond simplicity, SlackSocket provides a great deal of flexibility for writing apps, bots, or integrations. In the case of massive Slack groups with several thousand users, messages are buffered locally to ensure that none are missed. Dropped websocket connections are automatically re-connected as well, making it an ideal base for chat client.
The code for SlackSocket is available on GitHub, and as always, we welcome any contributions or feature requests!
Bradley Cicenas is a New York City-based infrastructure engineer with an affinity for microservices, systems design, data science, and stoops.