










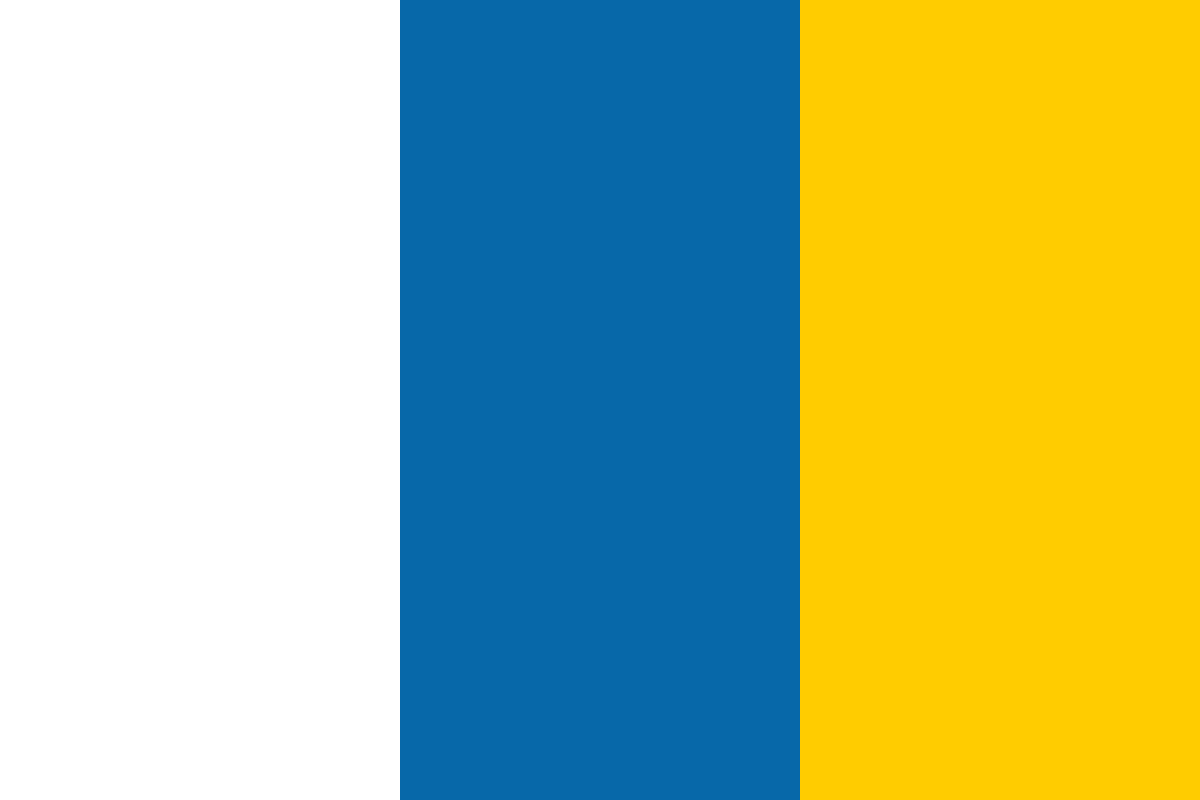

































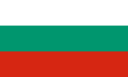








Personal community is a dynamic feature of Liferay portal. By default, the personal community is a portal-wide setting that will affect all of the users. It would be nice to have more features in the personal community such as showing the most popular journal articles. This article by Jonas Yuan will address how to set up the most popular journal articles in you personalized community and view the counter for other assets.
In a web site, we will have a lot of journal articles (that is, web content) for a given article type. For example, for the article type Article Content, we will have articles talking about product family. We may want to know how many times the end users read each article. Meanwhile, it would be nice if we could show the most popular articles (for example, TOP 10 articles) for this given article type.
As shown in the following screenshot, a journal article My EDI Product I is shown via a portlet Ext Web Content Display. Rating and comments on this article are also exhibited. At the same time, the medium-size image, polls, and related content of this article are listed, too. A view counter of this article is especially displayed under the ratings. Moreover, the most popular articles are exhibited with article title and number of views under related content. All these articles belong to the article type article-content. That is, the article in the current portlet Ext Web Content Display has the most popular articles only for the article type article-content.
Of course, you can customize the portlet Web Content Display directly through changing JSP files. For demo purposes, we will implement the view counter in the portlet Ext Web Content Display. Meanwhile, we will implement the mostly popular articles via VM services and article templates. In addition, we will analyze the view counter for other assets such as Image Gallery images, Document Library documents, Wiki articles, Blog entries, Message Boards threads, and so on.
First of all, let's add a view counter in the Ext Web Content Display portlet. As the function of view counter for assets (including journal articles) is provided in the model TagsAssetModel of the com.liferay.portlet.tags.model package in the /portal/portal-service/src folder, we could use this feature in this portlet directly. To do so, use the following steps:
JournalArticleDisplay articleDisplay = (JournalArticleDisplay)
request.getAttribute(
WebKeys.JOURNAL_ARTICLE_DISPLAY);
if (articleDisplay != null) {
TagsAssetLocalServiceUtil.incrementViewCounter(
JournalArticle.class.getName(),
articleDisplay.getResourcePrimKey());
}
<span class="view-count">
<% TagsAsset asset = TagsAssetLocalServiceUtil.getAsset
(JournalArticle.class.getName(),
articleDisplay.getResourcePrimKey());%>
<c:choose>
<c:when test="<%= asset.getViewCount() == 1 %>">
<%= asset.getViewCount() %>
<liferay-ui:message key="view" />,
</c:when>
<c:when test="<%= asset.getViewCount() > 1 %>">
<%= asset.getViewCount() %>
<liferay-ui:message key="views" />,
</c:when>
</c:choose>
</span>
The code above shows a way to increase the view counter via the TagsAssetLocalServiceUtil.incrementViewCounter method. This method takes two parameters className and classPK as inputs. For the current journal article, the two parameters are JournalArticle.class.getName() and articleDisplay.getResourcePrimKey(). Then, this code shows a way to display view counted through the TagsAssetLocalServiceUtil.getAsset method. Similarly, this method also takes two parameters, className and classPK, as inputs. This approach would be useful for other assets, as the className parameter could be Image Gallery, Document Library, Wiki, Blogs, Message Boards, Bookmark, and so on.
We can set up the VM service to exhibit the most popular articles. We can also add the getMostPopularArticles method in the custom velocity tool ExtVelocityToolUtil.
To do so, first add the following method in the ExtVelocityToolService interface:
public List<TagsAsset> getMostPopularArticles(String companyId,
String groupId, String type, int limit);
And then add an implementation of the getMostPopularArticles method in the ExtVelocityToolServiceImpl class as follows:
public List<TagsAsset> getMostPopularArticles(String companyId,
String groupId, String type, int limit) {
List<TagsAsset> results = Collections.synchronizedList(new
ArrayList<TagsAsset>());
DynamicQuery dq0 = DynamicQueryFactoryUtil.forClass(
JournalArticle.class, "journalarticle").
setProjection(ProjectionFactoryUtil.property
("resourcePrimKey")).add(PropertyFactoryUtil.
forName("journalarticle.companyId").
eqProperty("tagsasset.companyId")).
add(PropertyFactoryUtil.forName(
"journalarticle.groupId").eqProperty(
"tagsasset.groupId")).add(PropertyFactoryUtil.
forName("journalarticle.type").eq(
"article-content"));
DynamicQuery query = DynamicQueryFactoryUtil.forClass(
TagsAsset.class, "tagsasset")
.add(PropertyFactoryUtil.forName(
"tagsasset.classPK").in(dq0))
.addOrder(OrderFactoryUtil.desc(
"tagsasset.viewCount"));
try{ List<Object> assets = TagsAssetLocalServiceUtil.
dynamicQuery(query);
int index = 0; for (Object obj: assets) {
TagsAsset asset = (TagsAsset) obj;
results.add(asset);
index ++;
if(index == limit)
break;
}
}
catch (Exception e){
return results;
}
return results;
}
The preceding code shows a way to get the most popular articles by company ID, group ID, article type, and limited articles to be returned. DynamicQuery API allows us to leverage the existing mapping definitions through access to the Hibernate session. For example, DynamicQuery dq0 selects the journal articles by companyID, groupId, and type; DynamicQuery query selects tagsassets by classPK, which exists in DynamicQuery dq0; and tagsassets are ordered by viewCount as well.
Finally, add the following method to register the above method in ExtVelocityToolUtil:
public List<TagsAsset> getRelatedArticles(String companyId, String
groupId, String articleId, int limit){
return _extVelocityToolService.getRelatedArticles(companyId,
groupId, articleId, limit);
}
The code above shows a generic approach to get TOP 10 articles for any article types. Of course, you can extend this approach to find TOP 10 assets. This can include Image Gallery images, Document Library documents, Wiki articles, Blog entries, Message Boards threads, Bookmark entries, slideshow, videos, games, video queue, video list, playlist, and so on. You may practice these TOP 10 assets feature.
We have added view counter on journal articles. We have already built VM service for the most popular articles too. Now let's build an article template for them.
As mentioned earlier, there is a set of types of journal articles, for example, announcements, blogs, general, news, press-release, updates, article-tout, article-content, and so on. In real case, only some of these types will require view counter, for example article-content. Let's configure the default article type for mostly popular articles. We can add the following line at the end of portal-ext.properties.
ext.most_popular_articles.article_type=article-content
The code above shows that the default article type for most_popular_articles is article-content.