










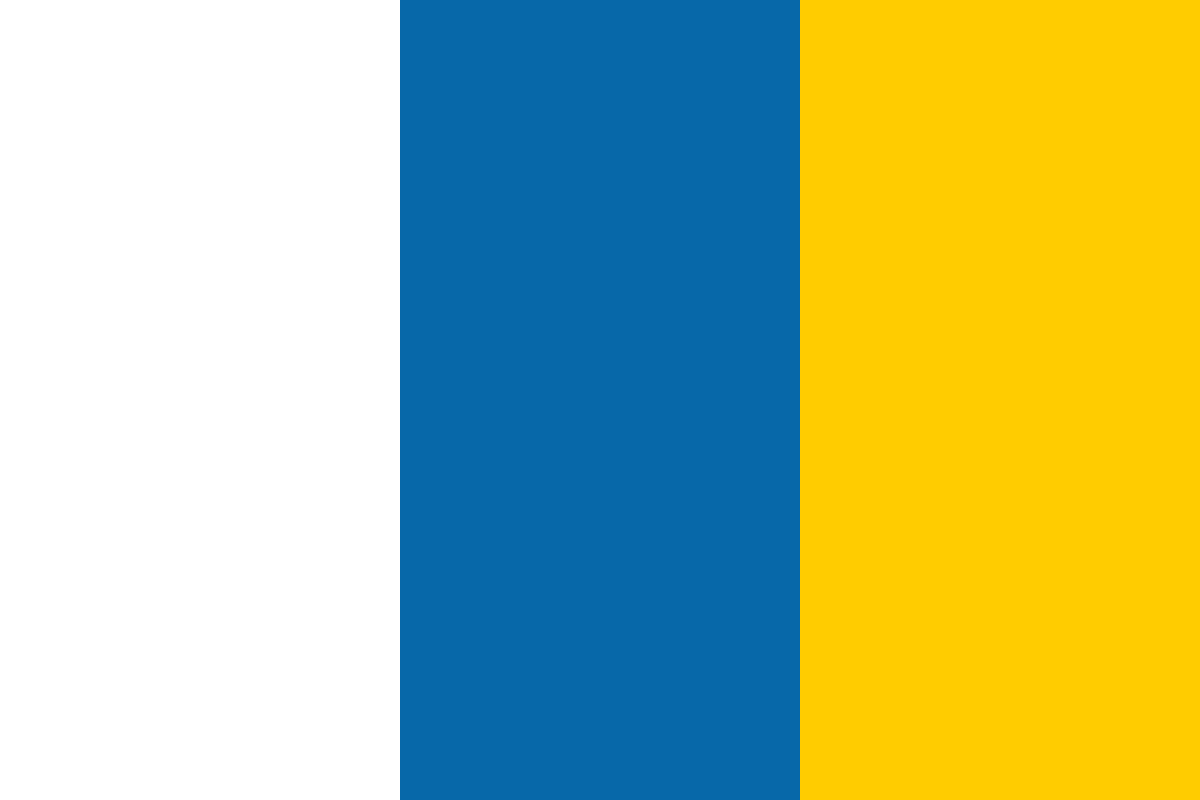

































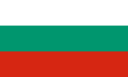








First of all, let us understand what a web feed is. Basically, it is a data format that provides frequently updated content to users. Content distributors syndicate the web feed, allowing users to subscribe, by using feed aggregator. RSS feeds contain data in an XML format.
RSS is the term used for describing Really Simple Syndication, RDF Site Summary, or Rich Site Summary, depending upon the different versions.
RDF (Resource Description Framework), a family of W3C specification, is a data model format for modelling various information such as title, author, modified date, content etc through variety of syntax format. RDF is basically designed to be read by computers for exchanging information.
Since, RSS is an XML format for data representation, different authorities defined different formats of RSS across different versions like 0.90, 0.91, 0.92, 0.93, 0.94, 1.0 and 2.0. The following table shows when and by whom were the different RSS versions proposed.
RSS Version
|
Year
|
Developer's Name
|
RSS 0.90
|
1999
|
Netscape introduced RSS 0.90.
|
RSS 0.91
|
1999
|
Netscape proposed the simpler format of RSS 0.91.
|
1999
|
UserLand Software proposed the RSS specification.
|
|
RSS 1.0
|
2000
|
O'Reilly released RSS 1.0.
|
RSS 2.0
|
2000
|
UserLand Software proposed the further RSS specification in this version and it is the most popular RSS format being used these days.
|
Meanwhile, Harvard Law school is responsible for the further development of the RSS specification.
There had been a competition like scenario for developing the different versions of RSS between UserLand, Netscape and O'Reilly before the official RSS 2.0 specification was released. For a detailed history of these different versions of RSS you can check http://www.rss-specifications.com/history-rss.htm
The current version RSS is 2.0 and it is the common format for publishing RSS feeds these days.
Like RSS, there is another format that uses the XML language for publishing web feeds. It is known as ATOM feed, and is most commonly used in Wiki and blogging software. Please refer to http://en.wikipedia.org/wiki/ATOM for detail.
The following is the RSS icon that denotes links with RSS feeds.
If you're using Mozilla's Firefox web browser then you're likely to see the above image in the address bar of the browser for subscribing to an RSS feed link available in any given page.
Web browsers like Firefox and Safari discover available RSS feeds in web pages by looking at the Internet media type application/rss+xml. The following tag specifies that this web page is linked with the RSS feed URL:
http://www.example.com/rss.xml
<link href="http://www.example.com/rss.xml" rel="alternate"
type="application/rss+xml" title="Sitewide RSS Feed" />
First of all, let’s look at a simple example of the RSS format.
<?xml version="1.0" encoding="UTF-8" ?>
<rss version="2.0">
<channel>
<title>Title of the feed</title>
<link>http://www.examples.com</link>
<description>Description of feed</description>
<item>
<title>News1 heading</title>
<link>http://www.example.com/news-1</link>
<description>detail of news1 </description>
</item>
<item>
<title>News2 heading</title>
<link>http://www.example.com/news-2</link>
<description>detail of news2 </description>
</item>
</channel>
</rss>
The first line is the XML declaration that indicates its version is 1.0. The character encoding is UTF-8. UTF-8 characters support many European and Asian characters so it is widely used as character encoding in web. The next line is the rss declaration, which declares that it is a RSS document of version 2.0
The next line contains the <channel> element which is used for describing the detail of the RSS feed. The <channel> element must have three required elements <title>, <link> and <description>. The title tag contains the title of that particular feed. Similarly, the link element contains the hyperlink of the channel and the description tag describes or carries the main information of the channel. This tag usually contains the information in detail.
Furthermore, each <channel> element may have one or more <item> elements which contain the story of the feed. Each <item> element must have the three elements <title>, <link> and <description> whose use is similar to those of channel elements, but they describe the details of each individual items.
Finally, the last two lines are the closing tags for the <channel> and <rss> elements.
The RSS widget we're going to build is a simple one which displays the headlines from the RSS feed, along with the title of the RSS feed. This is another widget which uses some JavaScript, PHP CSS and HTML. The content of the widget is displayed within an Iframe so when you set up the widget, you've to adjust the height and width.
To parse the RSS feed in XML format, I've used the popular PHP RSS Parser – Magpie RSS. The homepage of Magpie RSS is located at http://magpierss.sourceforge.net/.
Before writing the code, let's understand what the benefits of using the Magpie framework are, and how it works.
Now, let's quickly understand how Magpie RSS is used to parse the RSS feed. I'm going to pick the example from their homepage for demonstration.
require_once 'rss_fetch.inc';
$url = 'http://www.getacoder.com/rss.xml';
$rss = fetch_rss($url);
echo "Site: ", $rss->channel['title'], "<br>
";
foreach ($rss->items as $item ) {
$title = $item[title];
$url = $item[link];
echo "<a href=$url>$title</a></li><br>
";
}
If you're more interested in trying other PHP RSS parsers then you might like to check out SimplePie RSS Parser (http://simplepie.org/) and LastRSS (http://lastrss.oslab.net/).
You can see in the first line how the rss_fetch.inc file is included in the working file. After that, the URL of the RSS feed from getacoder.com is assigned to the $url variable. The fetch_rss() function of Magpie is used for fetching data and converting this data into RSS Objects. In the next line, the title of RSS feed is displayed using the code $rss->channel['title'].
The other lines are used for displaying each of the RSS feed's items. Each feed item is stored within an $rss->items array, and the foreach() loop is used to loop through each element of the array.
As I've already discussed, this widget is going to use Iframe for displaying the content of the widget, so let's look at the JavaScript code for embedding Iframe within the HTML code.
var widget_string = '<iframe
src="http://www.yourserver.com/rsswidget/rss_parse_handler.php?rss_url=';
widget_string += encodeURIComponent(rss_widget_url);
widget_string += '&maxlinks='+rss_widget_max_links;
widget_string += '" height="'+rss_widget_height+'" width="'+rss_widget_width+'"';
widget_string += ' style="border:1px solid #FF0000;"';
widget_string +=' scrolling="no" frameborder="0"></iframe>';
document.write(widget_string);
In the above code, the widget string variable contains the string for displaying the widget. The source of Iframe is assigned to rss_parse_handler.php. The URL of the RSS feed, and the headlines from the feed are passed to rss_parse_handler.php via the GET method, using rss_url and maxlinks parameters respectively. The values to these parameters are assigned from the Javascript variables rss_widget_url and rss_widget_max_links.
The width and height of the Iframe are also assigned from JavaScript variables, namely rss_widget_width and rss_widget_height. The red border on the widget is displayed by assigning 1px solid #FF0000 to the border attribute using the inline styling of CSS. Since, Inline CSS is used, the frameborder property is set to 0 (i.e. the border of the frame is zero).
Displaying borders from the CSS has some benefit over employing the frameborder property. While using CSS code, 1px dashed #FF0000 (border-width border-style border-color) means you can display a dashed border (you can't using frameborder), and you can use the border-right, border-left, border-top, border-bottom attributes of CSS to display borders at specified positions of the object.
The scrolling property is set to no here, which means that the scroll bar will not be displayed in the widget if the widget content overflows. If you want to show a scroll bar, then you can set this property to yes.
The values of JavaScript variables like rss_widget_url, rss_widget_max_links etc come from the page where we'll be using this widget. You'll see how the values of these variables will be assigned from the section at the end where we'll look at how to use this RSS widget.