










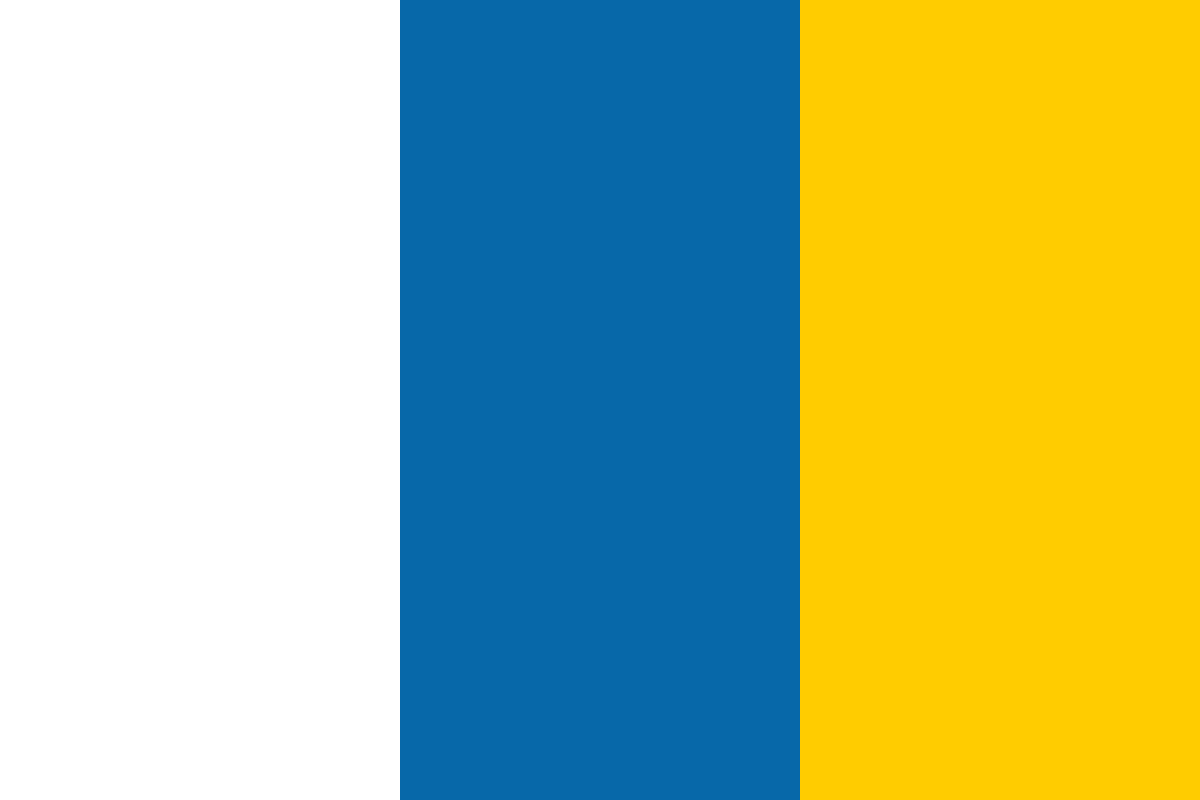

































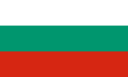








Kotlin has been eating up the Java world. It has already become a hit in the Android Ecosystem which was dominated by Java and is welcomed with open arms. Kotlin is not limited to Android development and can be used to develop server-side and client-side web applications as well. Kotlin is 100% compatible with the JVM so you can use any existing frameworks such as Spring Boot, Vert.x, or JSF for writing Java applications.
In this tutorial, we will learn how to implement RESTful web services using Kotlin.
This article is an excerpt from the book 'Kotlin Programming Cookbook', written by, Aanand Shekhar Roy and Rashi Karanpuria.
In this recipe, we will lay the foundation for developing the RESTful service. We will see how to set up dependencies and run our first SpringBoot web application. SpringBoot provides great support for Kotlin, which makes it easy to work with Kotlin. So let's get started.
We will be using IntelliJ IDEA and Gradle build system. If you don't have that, you can get it from https://www.jetbrains.com/idea/.
Let's follow the given steps to set up the dependencies for building RESTful services:
buildscript { ext.kotlin_version = '1.1.60' // Required for Kotlin integration ext.spring_boot_version = '1.5.4.RELEASE' repositories { jcenter() } dependencies { classpath "org.jetbrains.kotlin:kotlin-gradle-plugin:$kotlin_version" // Required for Kotlin integration classpath "org.jetbrains.kotlin:kotlin-allopen:$kotlin_version" // See https://kotlinlang.org/docs/reference/compiler-plugins.html#kotlin-spring-compiler-plugin classpath "org.springframework.boot:spring-boot-gradle-plugin:$spring_boot_version" } }
apply plugin: 'kotlin' // Required for Kotlin integration
apply plugin: "kotlin-spring" // See https://kotlinlang.org/docs/reference/compiler-plugins.html#kotlin-spring-compiler-plugin
apply plugin: 'org.springframework.boot'
jar {
baseName = 'gs-rest-service'
version = '0.1.0'
}
sourceSets {
main.java.srcDirs += 'src/main/kotlin'
}
repositories {
jcenter()
}
dependencies {
compile "org.jetbrains.kotlin:kotlin-stdlib:$kotlin_version" // Required for Kotlin integration
compile 'org.springframework.boot:spring-boot-starter-web'
testCompile('org.springframework.boot:spring-boot-starter-test')
}
It is important to keep the App.kt file in a package (we've used the college package). Otherwise, you will get an error that says the following:
** WARNING ** : Your ApplicationContext is unlikely to start due to a `@ComponentScan` of the default package.
The reason for this error is that if you don't include a package declaration, it considers it a "default package," which is discouraged and avoided.
@SpringBootApplication
open class App {
}
fun main(args: Array<String>) {
SpringApplication.run(App::class.java, *args)
}
Started AppKt in 5.875 seconds (JVM running for 6.445)
In the previous recipe, we learned how to set up dependencies for creating RESTful services. Finally, we launched our backend on the http://localhost:8080 endpoint but got 404 error as our application wasn't configured to handle requests at that path (/). We will start from that point and learn how to create a REST controller. Let's get started!
We will be using IntelliJ IDE for coding purposes. For setting up of the environment, refer to the previous recipe. You can also find the source in the repository at https://gitlab.com/aanandshekharroy/kotlin-webservices.
In this recipe, we will create a REST controller that will fetch us information about students in a college. We will be using an in-memory database using a list to keep things simple:
package college
class Student() {
lateinit var roll_number: String
lateinit var name: String
constructor(
roll_number: String,
name: String): this() {
this.roll_number = roll_number
this.name = name
}
}
@Component class StudentDatabase { private val students = mutableListOf<Student>() }
Note that we have annotated the StudentDatabase class with @Component, which means its lifecycle will be controlled by Spring (because we want it to act as a database for our application).
@PostConstruct private fun init() { students.add(Student("2013001","Aanand Shekhar Roy")) students.add(Student("2013165","Rashi Karanpuria")) }
fun getStudents()=students
fun addStudent(student: Student): Boolean { students.add(student) return true }
@RestController class StudentController { @Autowired private lateinit var database: StudentDatabase }
@RequestMapping("", method = arrayOf(RequestMethod.GET)) fun students() = database.getStudents()
package college
import org.springframework.beans.factory.annotation.Autowired
import org.springframework.web.bind.annotation.RequestMapping
import org.springframework.web.bind.annotation.RequestMethod
import org.springframework.web.bind.annotation.RestController
@RestController
class StudentController {
@Autowired
private lateinit var database: StudentDatabase
@RequestMapping("", method = arrayOf(RequestMethod.GET))
fun students() = database.getStudents()
}
As you can see, Spring is intelligent enough to provide the response in the JSON format, which makes it easy to design APIs.
@GetMapping("/student/{roll_number}") fun studentWithRollNumber( @PathVariable("roll_number") roll_number:String) = database.getStudentWithRollNumber(roll_number)
{"roll_number":"2013001","name":"Aanand Shekhar Roy"}
@RequestMapping("/add", method = arrayOf(RequestMethod.POST)) fun addStudent(@RequestBody student: Student) = if (database.addStudent(student)) student else throw Exception("Something went wrong")
So far, our server has been dependent on IDE. We would definitely want to make it independent of an IDE. Thanks to Gradle, it is very easy to create a runnable JAR just with the following:
./gradlew clean bootRepackage
The preceding command is platform independent and uses the Gradle build system to build the application. Now, you just need to type the mentioned command to run it:
java -jar build/libs/gs-rest-service-0.1.0.jar
You can then see the following output as before:
Started AppKt in 4.858 seconds (JVM running for 5.548)
This means your server is running successfully.
The SpringApplication class is used to bootstrap our application. We've used it in the previous recipes; we will see how to create the Application class for Spring Boot in this recipe.
We will be using IntelliJ IDE for coding purposes. To set up the environment, read previous recipes, especially the Setting up dependencies for building RESTful services recipe.
If you've used Spring Boot before, you must be familiar with using @Configuration, @EnableAutoConfiguration, and @ComponentScan in your main class. These were used so frequently that Spring Boot provides a convenient @SpringBootApplication alternative. The Spring Boot looks for the public static main method, and we will use a top-level function outside the Application class.
If you noted, while setting up the dependencies, we used the kotlin-spring plugin, hence we don't need to make the Application class open.
Here's an example of the Spring Boot application:
package college
import org.springframework.boot.SpringApplication
import org.springframework.boot.autoconfigure.SpringBootApplication
@SpringBootApplication
class Application
fun main(args: Array<String>) {
SpringApplication.run(Application::class.java, *args)
}
The Spring Boot application executes the static run() method, which takes two parameters and starts a autoconfigured Tomcat web server when Spring application is started.
When everything is set, you can start the application by executing the following command:
./gradlew bootRun
If everything goes well, you will see the following output in the console:
This is along with the last message—Started AppKt in xxx seconds. This means that your application is up and running.
In order to run it as an independent server, you need to create a JAR and then you can execute as follows:
./gradlew clean bootRepackage
Now, to run it, you just need to type the following command:
java -jar build/libs/gs-rest-service-0.1.0.jar
We learned how to set up dependencies for building RESTful services, creating a REST controller, and creating the application class for Spring boot. If you are interested in learning more about Kotlin then be sure to check out the 'Kotlin Programming Cookbook'.
Build your first Android app with Kotlin
5 reasons to choose Kotlin over Java
Getting started with Kotlin programming
Forget C and Java. Learn Kotlin: the next universal programming language