










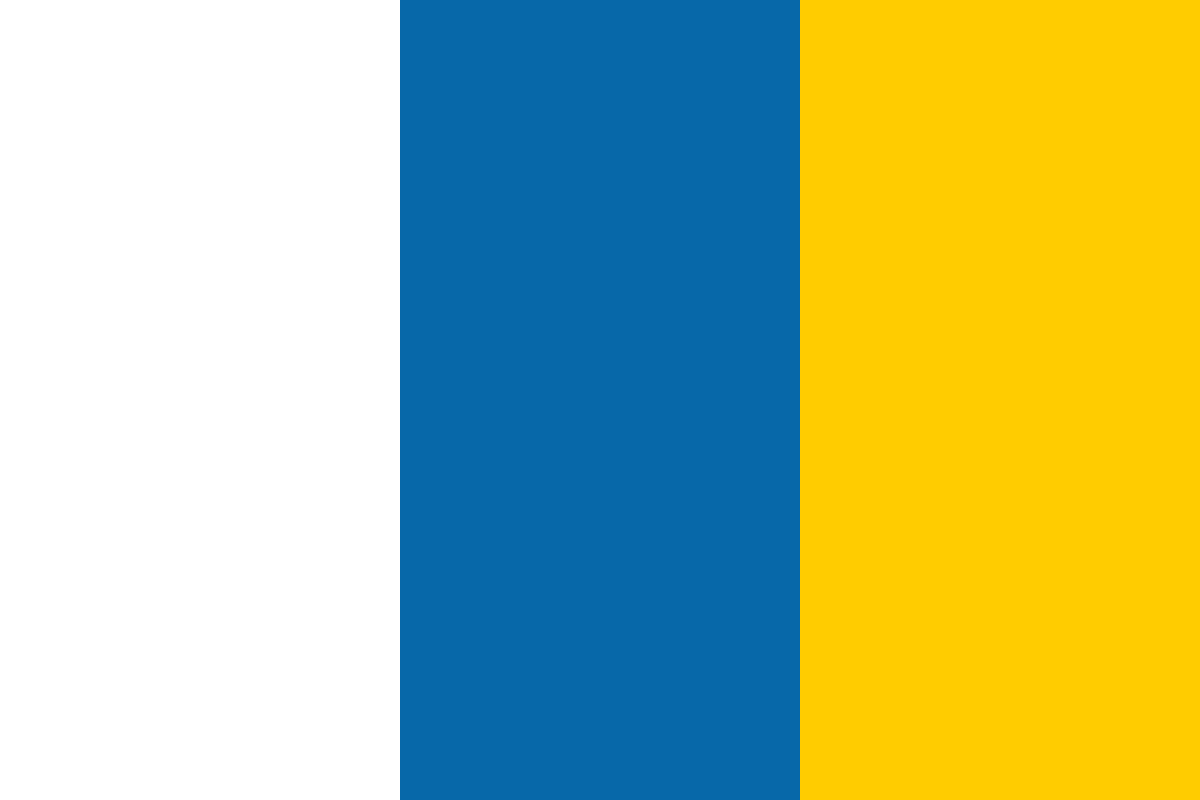

































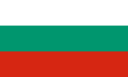








After the release of React 16.5.0 last month, the React team announced the release of React 16.6.0 yesterday. This release comes with a few new convenient features including support for code splitting, easier way to consume Context from class components, and more.
Let’s see what changes have been made in this release:
React.memo() is similar to React.PureComponent but for function components instead of class. It can be used in cases when the function component renders the same result given the same props.
You can wrap the function component in a call to React.memo() for a performance boost in some cases by memorizing the result. This will make React skip rendering the component and reuse the last rendered result.
You can now render a dynamic import as a regular component with the help of React.lazy() function. To do code splitting you can use the Suspense component by wrapping a dynamic import in a call to React.lazy().
Library authors can leverage this Suspense component to start building data fetching with Suspense support in the future. Note that the feature is not yet available for server-side rendering.
In React 16.3 a new Context API was introduced as a replacement to the previous Legacy Context API, which will be removed in a future major version.
React 16.6 comes with contextType, which makes it convenient to consume a context value from within a class component. A Context object created by React.createContext() can be assigned to the contextType property. This enables you to consume the nearest current value of that Context type using this.context. This update is done because developers were having some difficulties adopting the new render prop API in class components.
React 16 introduced error boundaries to solve the problem of a JavaScript error in a part of the UI breaking the whole app. What Error boundaries do is, they:
An already existing method, componentDidCatch() gets fired after an error has occurred. But before it is fired, null is rendered in place of the tree that causes an error. This sometimes results in breaking the parent components that don’t expect their refs to be empty.
This version introduces another lifecycle method called getDerivedStateFromError() that lets you render the fallback UI before the render completes.
The StrictMode component was released in React 16.3 that lets you opt-in early warnings for any deprecations. Two more APIs are now added to the deprecated APIs list: ReactDOM.findDOMNode() and Legacy Context. The reason these APIs are deprecated are:
To read the entire changelog of React 16.6, check out their official announcement and also React’s GitHub repository.
React 16.5.0 is now out with a new package for scheduling, support for DevTools, and more!
React Native 0.57 released with major improvements in accessibility APIs, WKWebView-backed implementation, and more!
InfernoJS v6.0.0, a React-like library for building high-performance user interfaces, is now out