










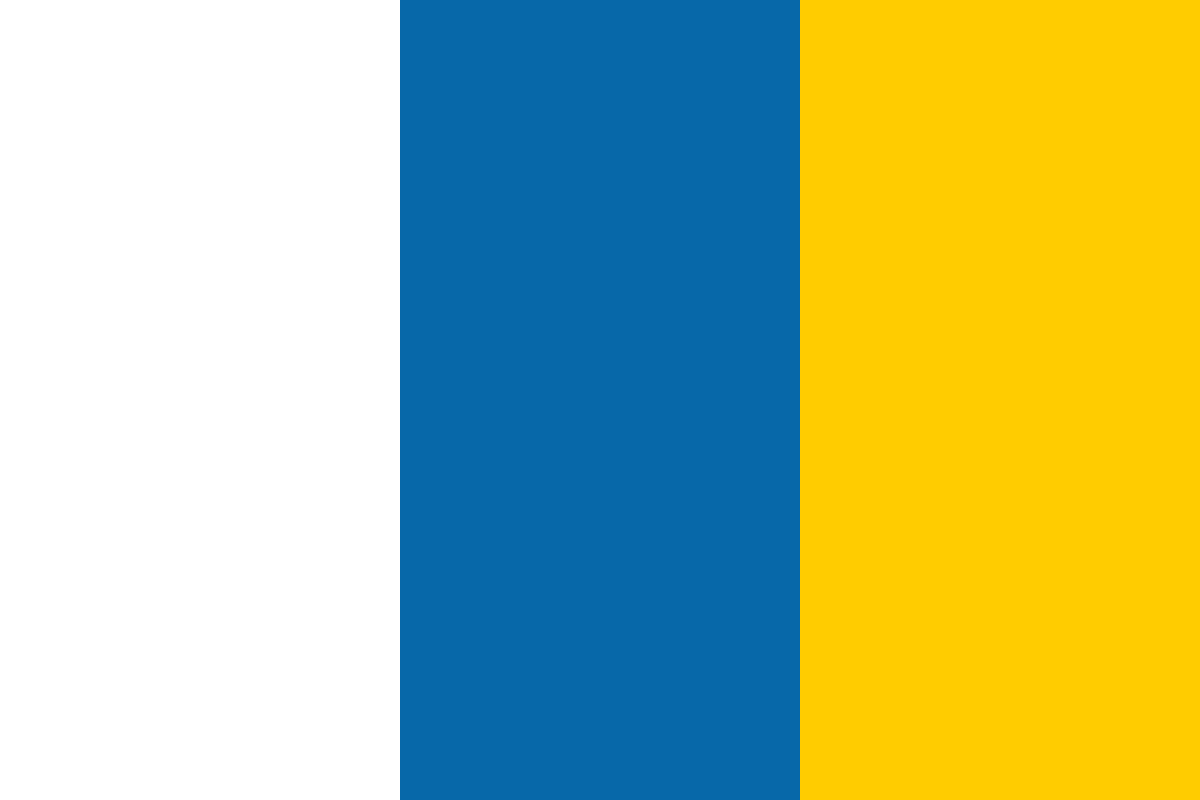

































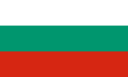








(For more resources related to this topic, see here.)
RavenDB provides a set of .NET client libraries for interacting with it, which can be accessed from any .NET-based programming languages. By using these libraries, you can manage RavenDB, construct requests, send them to the server, and receive responses.
The .NET Client API exposes all aspects of the RavenDB and allows developers to easily integrate RavenDB services into their own applications.
The .NET Client API is involved with loading and saving the data, and it is responsible for integrating with the .NET System.Transactions namespace. With System.Transactions, there is already a general way to work transactionally across different resources. Also the .NET Client API is responsible for batching requests to the server, caching, and more.
The .NET Client API is easy to use and uses several conventions to control how it works. These conventions can be modified by the user to meet its application needs.
It is not recommended to use System.Transactions. In fact, it is recommended to avoid it. System.Transactions is supported in RavenDB mostly to allow integration while using collaboration tools between services for example, NServiceBus.
Before you can start developing an application for RavenDB, you must first set up your development environment. This setup involves installing the following software packages on your development computer:
You may download and install the latest Visual Studio version from the Microsoft website: http://msdn.microsoft.com/En-us/library/vstudio/e2h7fzkw.aspx
In order to use RavenDB in your own .NET application, you have to add a reference to the Raven.Client.Lightweight.dll and the Raven.Abstractions.dll files, (which you can find in the ~Client folder of the distribution package) into your Visual Studio project.
The easiest way to add a reference to these DLLs is by using the NuGet Package Manager, which you may use to add the RavenDB.Client package.
NuGet Package Manager is a Visual Studio extension that makes it easy to add, remove, and update libraries and tools in Visual Studio projects that use the .NET framework.
You can find more information on the NuGet Package Manager extension by visiting the official website at http://nuget.org/.
The NuGet Package Manager extension is the easiest way to add the RavenDB Client library to a Visual Studio project. If you do not have NuGet Package Manager already installed, you can install it as follows:
We installed the NuGet Package Manager extension to Visual Studio, which you will use to add RavenDB Client to your Visual Studio project.
Let’s write a simple application to learn how to interact with RavenDB.
Let’s go ahead and create a new Visual Studio project. You will add the RavenDB Client using the Nuget Package Manager extension to this project to be able to connect to RavenDB and begin using it:
If you do not see this menu item, make sure that the NuGet Package Manager extension has been installed correctly.
We created the RavenDB_Ch03 Visual Studio project and added the RavenDB Client to get connected to the RavenDB server.
Once the RavenDB Client is installed, by expanding the References node of your project in Visual Studio, you can see the RavenDB DLLs (Raven.Abstractions, Raven.Client.Lightweight) added automatically to your project by the Nuget Package Manager extension.
You should ensure that the RavenDB Client version matches the server version you are running. This can lead to some really frustrating runtime errors when the versions don’t match.
You can also install RavenDB Client using the Package Manager console (Visual Studio | Tools | Library Package Manager | Package Manager Console). To install the latest RavenDB Client, run the following command in the Package Manager console: Install-Package RavenDB.Client or Install-Package RavenDB.Client–Version {version number} to add a specific version.
To begin using RavenDB we need to get a new instance of the DocumentStore object, which points to the server and acts as the main communication channel manager.
Once a new instance of DocumentStore has been created, the next step is to create a new session against that Document Store. The session object is responsible for implementing the Unit of Work pattern. A Unit of Work keeps track of everything you do during a business transaction that can affect the database. When you’re done, it figures out everything that needs to be done to alter the database as a result of your work.
The session object is used to interact with RavenDB and provides a fully transactional way of performing database operations, and allows the consumer to store data into the database, and load it back when necessary using queries or by document ID.
In order to perform an action against the RavenDB store, we need to ensure that we have an active and valid RavenDB session before starting the action, and dispose it off once it ends.
Basically, before disposing of the session, we will call the SaveChanges() method on the session object to ensure that all changes will be persisted.
To create a new RavenDB session, we will call the OpenSession() method on the DocumentStore object, which will return a new instance of the IDocumentSession object.
Your Visual Studio project now has a reference to all needed RavenDB Client DLLs and is ready to get connected to the RavenDB server. You will add a new class and necessary code to create a communication channel with the RavenDB server:
We just added the DocumentStore object initialization code to the Main() method of the RavenDB_Ch03 project.
Within the Main() method, you create a new instance of the DocumentStore object, which is stored in the DocumentStore variable. The DocumentStore object basically acts as the connection manager and must be created only once per application.
It is important to point out that this is a heavyweight and thread safe object. Note that, when you create many of these your application will run slow and will have a larger memory footprint.
To create an instance of the DocumentStore object, you need to specify the URL that points to the RavenDB server. The URL has to include the TCP port number (8080 is the default port number) on which RavenDB server is listening (line 17 in the previous code snippet).
In order to point to the Orders database, you set the value of DefaultDatabase to Orders, which is the name of our database (line 18).
To get the new instance of the IDocumentStore object, you have to call the Initialize() method on the DocumentStore object. With this instance, you can establish the connection to the RavenDB server (line 19).
The whole DocumentStore initialization code is surrounded by a using statement. This is used to specify when resources should be released. Basically, the using statement calls the Dispose() method of each object for which the resources should be released.
RavenDB stores documents in JSON format. It converts the .NET objects into their JSON equivalent when storing documents, and back again by mapping the .NET object property names to the JSON property names and copies the values when loading documents. This process that makes writing and reading data structures to and from a document file extremely easy is called Serialization and Deserialization.
Interacting with RavenDB is very easy. You might create a new DocumentStore object and then open a session, do some operations, and finally apply the changes to the RavenDB server.
The session object will manage all changes internally, but changes will be committed to the underlying document database only when the SaveChanges() method is called. This is important to note because all changes to the document will be discarded if this method is not invoked.
RavenDB is safe by default. This unique feature means that the database is configured to stop users querying for large amount of data. It is never a good idea to have a query that returns thousands of records, which is inefficient and may take a long time to process.
By default, RavenDB limits the number of documents on the client side to 128 (this is a configurable option) if you don't make use of the Take() method on the Query object.
Basically, if you need to get data beyond the 128 documents limit, you need to page your query results (by using Take() and Skip() methods). RavenDB also has a very useful option to stop you from creating the dreaded SELECT N+1 scenario—this feature stops after 30 requests are sent to the server per session, (which also is a configurable option).
The recommended practice is to keep the ratio of SaveChanges() calls to session instance at 1:1. Reaching the limit of 30 remote calls while maintaining the 1:1 ratio is typically a symptom of a significant N+1 problem.
To retrieve or store documents, you might create a class type to hold your data document and use the session instance to save or load that document, which will be automatically serialized to JSON.
To load a single document from a RavenDB database, you need to invoke the Load() method on the Session object you got from the DocumentStore instance. Then you need to specify the type of the document you will load from the RavenDB database.
You will create the Order class to hold the order data information and will add the LoadOrder()method, which you will call to load an Order document from the RavenDB server:
You just wrote the necessary code to load your first document from the RavenDB server. Let’s take a look at the code you added to the RavenDB_Ch03 project.
You added the Order class to the project. This class will hold the data information for the Order document you will load from the server. It contains six fields (lines 11 to 16 in the previous code snippet) that will be populated with values from the JSON Order document stored on the server.
By adding a field named Id, RavenDB will automatically populate this field with the document ID when the document is retrieved from the server.
You added the DisplayOrder() method to the Program class. This method is responsible for displaying the Order field’s values to the output window.
You also added the Load<Order>() method (lines 26 to 30) to the Program class. This method is surrounded by a using statement to ensure that the resources will be disposed at the end of execution of this method.
To open a RavenDB session you call the OpenSession() method on the IDocumentStore object. This session is handled by the session variable (line 26).
The Load() method is a generic method. It will load a specific entity with a specific ID, which you need to provide when calling the method. So in the calling code to the Load() method (line 28), you provide the Order entity and the document ID that we want to retrieve from the server which is Orders/A179854.
Once the Order document with the Id field as Orders/A179854 is retrieved from the server, you send it to the DisplayOrder() method to be displayed (line 29).
Finally, you build and run the RavenDB_Ch03 project.
You know now how to load any single document from the RavenDB server using the Load() method. What if you have more than one document to load? You can use the Load() method to load more than one document in a single call. It seems easy to do. Well give it a go!
Arrays are very useful!