










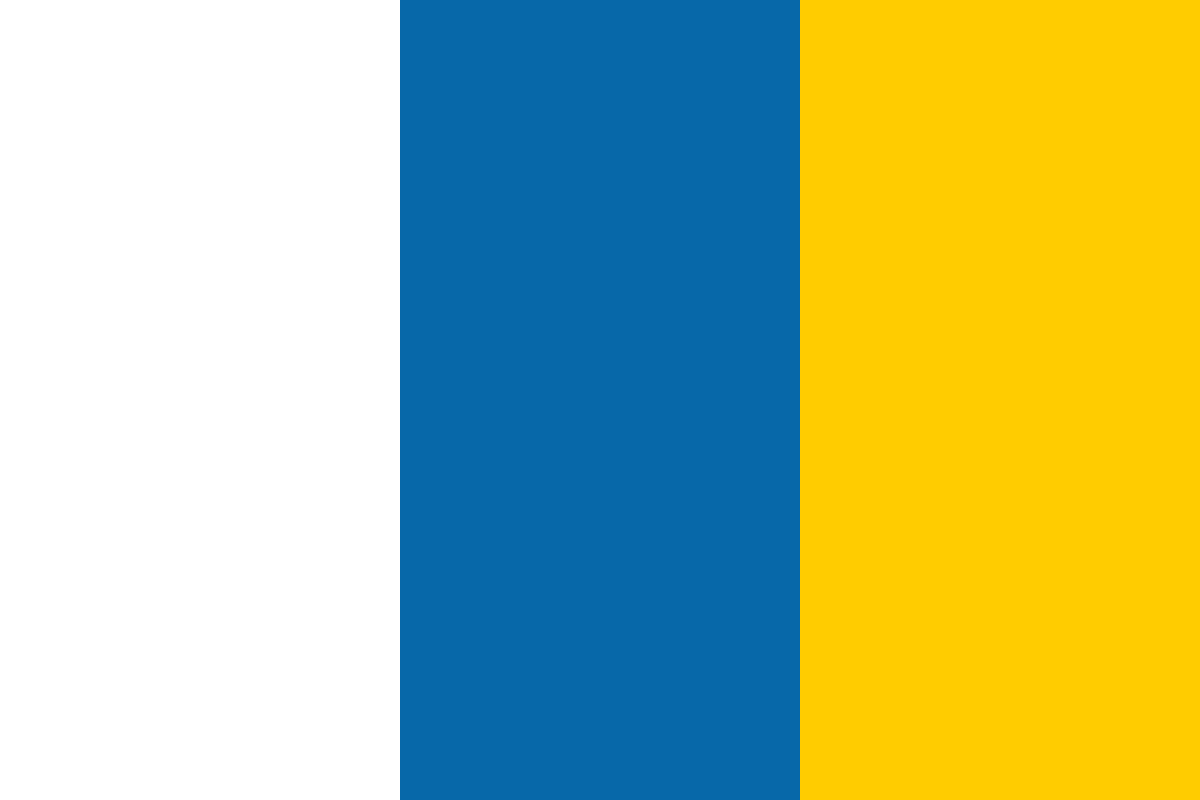

































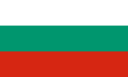








In this two-part series, we're programing littleBits circuits using the Johnny-Five JavaScript Robotics Framework. Be sure to read over Part 1 before continuing here.
Let's create a circuit to play with, using all of the modules from the littleBits Arduino Coding Kit.
Attach a button to the Arduino connector labelled d0. Attach a dimmer to the connector marked a0 and a second dimmer to a1. Turn the dimmers all the way to the right (max) to start with.
Attach a power module to the single connector on the left-hand side of the fork module, and the three output connectors of the fork module to all of the input modules.
The bargraph should be connected to d5, and the servo to d9, and both set to PWM output mode using the switches on board of the Arduino. The servo module has two modes: turn and swing. Swing mode makes the servo sweep betwen maximum and minimum. Set it to swing mode using the onboard switch.
We'll create an instance of the Johnny-Five Button class to respond to button press events. Our button is connected to the connector labelled d0 (i.e. digital "pin" 0) on our Arduino, so we'll need to specify the pin as an argument when we create the button.
var five = require("johnny-five");
var board = new five.Board();
board.on("ready", function() {
var button = new five.Button(0);
});
Our dimmers are connected to analog pins (A0 and A1), so we'll specify these as strings when we create Sensor objects to read their values. We can also provide options for reading the values; for example, we'll set the frequency to 250 milliseconds, so we'll be receiving 4 readings per second for both dimmers.
var dimmer1 = new five.Sensor({
pin: "A0",
freq: 250
});
var dimmer2 = new five.Sensor({
pin: "A1",
freq: 250
});
We can attach a function that will be run any time the value changes (on "change") or anytime we get a reading (on "data"):
dimmer1.on("change", function() {
// raw value (between 0 and 1023)
console.log("dimmer 1 is " + this.raw);
});
Run the code and try turning dimmer 1. You should see the value printed to the console whenever the dimmer value changes.
Now we can use code to hook our input components up to our output components. To use, for example, the dimmer to control the brightness of the bargraph, change the code in the event handler:
var led = new five.Led(5);
dimmer1.on("change", function() {
// set bargraph brightness to one quarter
// of the raw value from dimmer
led.brightness(Math.floor(this.raw / 4));
});
You'll see the bargraph brightness fade as you turn the dimmer. We can use the JavaScript Math library and operators to manipulate the brightness value before we send it to the bargraph. Writing code gives us more control over the mapping between input values and output behaviors than if we'd snapped our littleBits modules directly together without going via the Arduino. We set our d5 output to PWM mode, so all of the LEDs should fade in and out at the same time. If we set the output to analog mode instead, we'd see the behavior change to light up more or fewer LEDs depending on value of the brightness.
Let's use the button to trigger the servo stop and start functions. Add a button press handler to your code, and a variable to keep track of whether the servo is running or not. We'll toggle this variable between true and false using JavaScript's boolean not operator (!). We can determine whether to stop or start the servo each time the button is pressed via a conditional statement based on the value of this variable.
var servo = new five.Motor(9);
servo.start();
var button = new five.Button(0);
var toggle = false;
var speed = 255;
button.on("press", function(value){
toggle = !toggle;
if (toggle) {
servo.start(speed);
}
else {
servo.stop();
}
});
The other dimmer can be used to change the servo speed:
dimmer2.on("change", function(){
speed = Math.floor(this.raw / 4);
if (toggle) {
servo.start(speed);
}
});
There are many input and output modules available within the littleBits system for you to experiment with. You can use the Sensor class with input modules, and check out the Johnny-Five API docs to see examples of types of outputs supported by the API. You can always fall back to using the Pin class to program any littleBits module.
Johnny-Five includes a Read-Eval-Print-Loop (REPL) so you can interactively write code to control components instantly - no waiting for code to compile and upload! Any of the JavaScript objects from your program that you want to access from the REPL need to be "injected". The following code, for example, injects our servo and led objects.
this.repl.inject({
led: led,
servo: servo
});
After running the program using Node.js, you'll see a >> prompt in your terminal. Try some of the following functions (hit Enter after each to see it take effect):
LittleBits are fantastic for prototyping, and pairing the littleBits Arduino with JavaScript makes prototyping interactive electronic projects even faster and easier.
Anna Gerber is a full-stack developer with 15 years of experience in the university sector. Specializing in Digital Humanities, she was a Technical Project Manager at the University of Queensland’s eResearch centre, and she has worked at Brisbane’s Distributed System Technology Centre as a Research Scientist. Anna is a JavaScript robotics enthusiast who enjoys tinkering with soft circuits and 3D printers.