










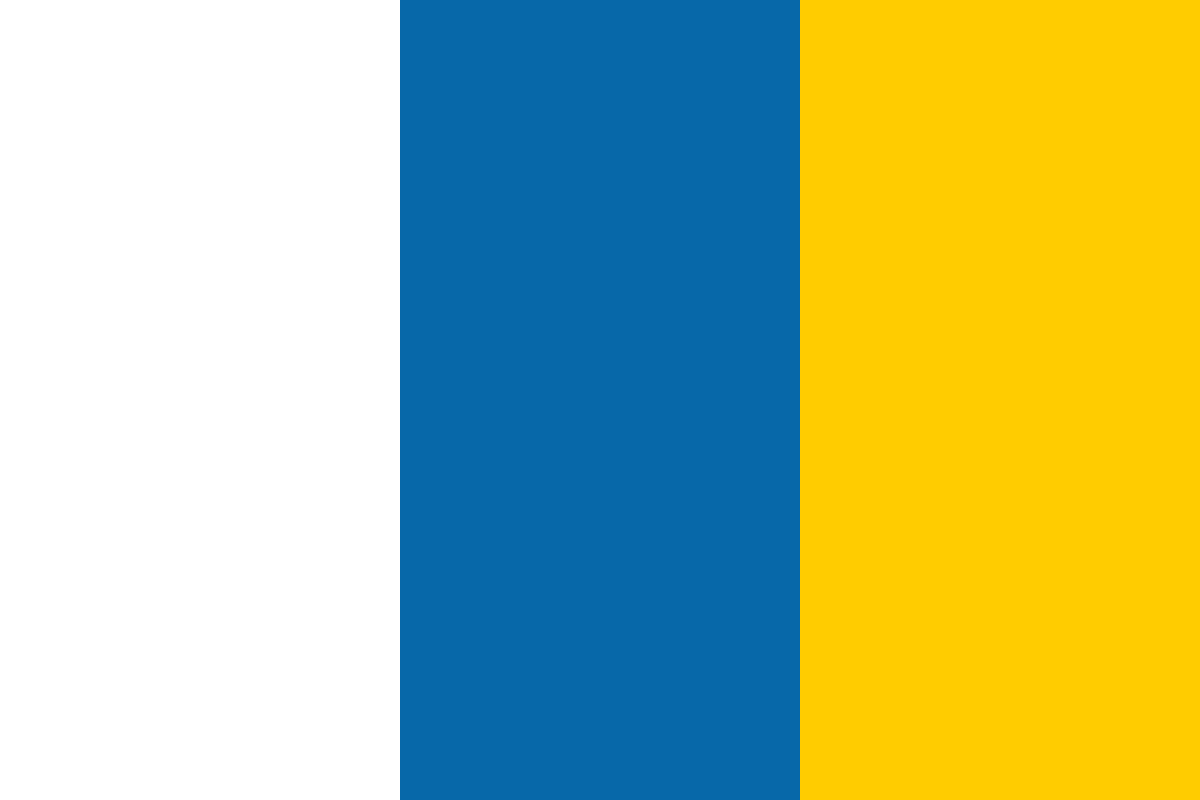

































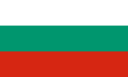








(For more resources related to this topic, see here.)
Let's begin with a simple web page that is not mobile optimized. To be clear, we aren't saying it can't be viewed on a mobile device. Not at all! But it may not be usable on a mobile device. It may be hard to read (text too small). It may be too wide. It may use forms that don't work well on a touch screen. We don't know what kinds of problems we will have at all until we start testing. (And we've all tested our websites on mobile devices to see how well they work, right?)
Let's have a look at the following code snippet:
<h1>Welcome</h1> <p> Welcome to our first mobile web site. It's going to be the best
site you've ever seen. Once we get some content.
And a business plan. But the hard part is done! </p> <p> <i>Copyright Megacorp© 2013</i> </p> </body> </html>
As we said, there is nothing too complex, right? Let's take a quick look at this in the browser:
Not so bad, right? But let's take a look at the same page in a mobile simulator:
Wow, that's pretty tiny. You've probably seen web pages like this before on your mobile device. You can, of course, typically use pinch and zoom or double-click actions to increase the size of the text. But it would be preferable to have the page render immediately in a mobile-friendly view. This is where jQuery Mobile comes in.
In the preface we talked about how jQuery Mobile is just a set of files. That isn't said to minimize the amount of work done to create those files, or how powerful they are, but to emphasize that using jQuery Mobile means you don't have to install any special tools or server. You can download the files and simply include them in your page. And if that's too much work, you have an even simpler solution. jQuery Mobile's files are hosted on a Content Delivery Network (CDN). This is a resource hosted by them and guaranteed (as much as anything like this can be) to be online and available. Multiple sites are already using these CDN hosted files. That means when your users hit your site they may already have the resources in their cache. For this article, we will be making use of the CDN hosted files, but just for this first example we'll download and extract the files we need. I recommend doing this anyway for those times when you're on an airplane and wanting to whip up a quick mobile site.
To grab the files, visit http://jquerymobile.com/download. There are a few options here but you want the ZIP file option. Go ahead and download that ZIP file and extract it. (The ZIP file you downloaded earlier from GitHub has a copy already.) The following screenshot demonstrates what you should see after extracting the files from the ZIP file:
Notice the ZIP file contains a CSS and JavaScript file for jQuery Mobile, as well as a minified version of both. You will typically want to use the minified version in your production apps and the regular version while developing. The images folder has five images used by the CSS when generating mobile optimized pages. You will also see demos for the framework as well as theme and structure files. So, to be clear, the entire framework and all the features we will be talking about over the rest of the article will consist of a framework of 6 files. Of course, you also need to include the jQuery library. You can download that separately at www.jquery.com. At the time this article was written, the recommended version was 1.9.1.
As a final option for downloading jQuery Mobile, you can also use a customized Download Builder tool at http://jquerymobile.com/download-builder. Currently in Alpha (that is, not certified to be bug-free!), the web-based tool lets you download a jQuery Mobile build minus features your website doesn't need. This creates smaller files which reduces the total amount of time your application needs to display to the end user.
Ok, we've got the bits, but how do we use them? Adding jQuery Mobile support to a site requires the following three steps at a minimum:
Let's look at a modified version of our previous HTML file that adds all of the above:
code 1-2: test2.html <!DOCTYPE html> <html> <head> <title>First Mobile Example</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet"href="jquery.mobile-1.3.2.min.css" /> <script type="text/javascript"src = "http://code.jquery.com/jquery-
1.9.1.min.js"></script> <script type="text/javascript"src = "jquery.mobile-1.3.2.min.js">
</script> </head> <body> <h1>Welcome</h1> <p> Welcome to our first mobile web site. It's going to be the best site
you've ever seen. Once we get some content. And a business plan.
But the hard part is done! </p> <p> <i>Copyright Megacorp© 2013</i> </p> </body> </html>
For the most part, this version is the exact same as Code 1-1, except for the addition of the DOCTYPE, the CSS link, and our two JavaScript libraries. Notice we point to the hosted version of the jQuery library. It's perfectly fine to mix local JavaScript files and remote ones. If you wanted to ensure you could work offline, you can simply download the jQuery library as well.
So while nothing changed in the code between the body tags, there is going to be a radically different view now in the browser. The following screenshot shows how the iOS mobile browser renders the page now:
Right away, you see a couple of differences. The biggest difference is the relative size of the text. Notice how much bigger it is and easier to read. As we said, the user could have zoomed in on the previous version, but many mobile users aren't aware of this technique. This page loads up immediately in a manner that is much more usable on a mobile device.
As we saw in the previous example, just adding in jQuery Mobile goes a long way to updating our page for mobile support. But there's a lot more involved to really prepare our pages for mobile devices. As we work with jQuery Mobile over the course of the article, we're going to use various data attributes to mark up our pages in a way that jQuery Mobile understands. But what are data attributes?
HTML5 introduced the concept of data attributes as a way to add ad-hoc values to the DOM ( Document Object Model). As an example, this is a perfectly valid HTML:
<div id="mainDiv" data-ray="moo">Some content</div>
In the previous HTML, the data-ray attribute is completely made-up. However, because our attribute begins with data-, it is also completely legal. So what happens when you view this in your browser? Nothing! The point of these data attributes is to integrate with other code, like JavaScript, that does whatever it wants with them. So for example, you could write JavaScript that finds every item in the DOM with the data-ray attribute, and change the background color to whatever was specified in the value.
This is where jQuery Mobile comes in, making extensive use of data attributes, both for markup (to create widgets) and behavior (to control what happens when links are clicked). Let's look at one of the main uses of data attributes within jQuery Mobile—defining pages, headers, content, and footers:
code 1-3: test3.html <!DOCTYPE html> <html> <head> <title>First Mobile Example</title> <meta name="viewport" content="width=device-width, initial-scale=1"> <link rel="stylesheet"href="jquery.mobile-1.3.2.min.css" /> <script type="text/javascript"src = "http://code.jquery.com/jquery-
1.9.1.min.js"></script> <script type="text/javascript"src = "jquery.mobile-1.3.2.min.js">
</script> </head> <body> <div data-role="page"> <div data-role="header"><h1>Welcome</h1></div> <div data-role="content"> <p> Welcome to our first mobile web site. It's going to be the best
site you've ever seen. Once we get some content.
And a business plan. But the hard part is done! </p> </div> <div data-role="footer"> <h4>Copyright Megacorp© 2013</h4> </div> </div> </body> </html>
Compare the previous code snippet to code 1-2, and you can see that the main difference was the addition of the div blocks. One div block defines the page. Notice it wraps all of the content inside the body tags. Inside the body tag, there are three separate div blocks. One has a role of header, another a role of content, and the final one is marked as footer.
All the blocks use data-role, which should give you a clue that we're defining a role for each of the blocks. As we stated previously, these data attributes mean nothing to the browser itself. But let's look what at what jQuery Mobile does when it encounters these tags:
Notice right away that both the header and footer now have a black background applied to them. This makes them stand out even more from the rest of the content. Speaking of the content, the page text now has a bit of space between it and the sides. All of this was automatic once the div tags with the recognized data-roles were applied. This is a theme you're going to see repeated again and again as we go through this article. A vast majority of the work you'll be doing will involve the use of data attributes.
In this article, we talked a bit about how web pages may not always render well in a mobile browser. We talked about how the simple use of jQuery Mobile can go a long way to improving the mobile experience for a website. Specifically, we discussed how you can download jQuery Mobile and add it to an existing HTML page, what data attributes mean in terms of HTML, and how jQuery Mobile makes use of data attributes to enhance your pages.
Further resources on this subject: