










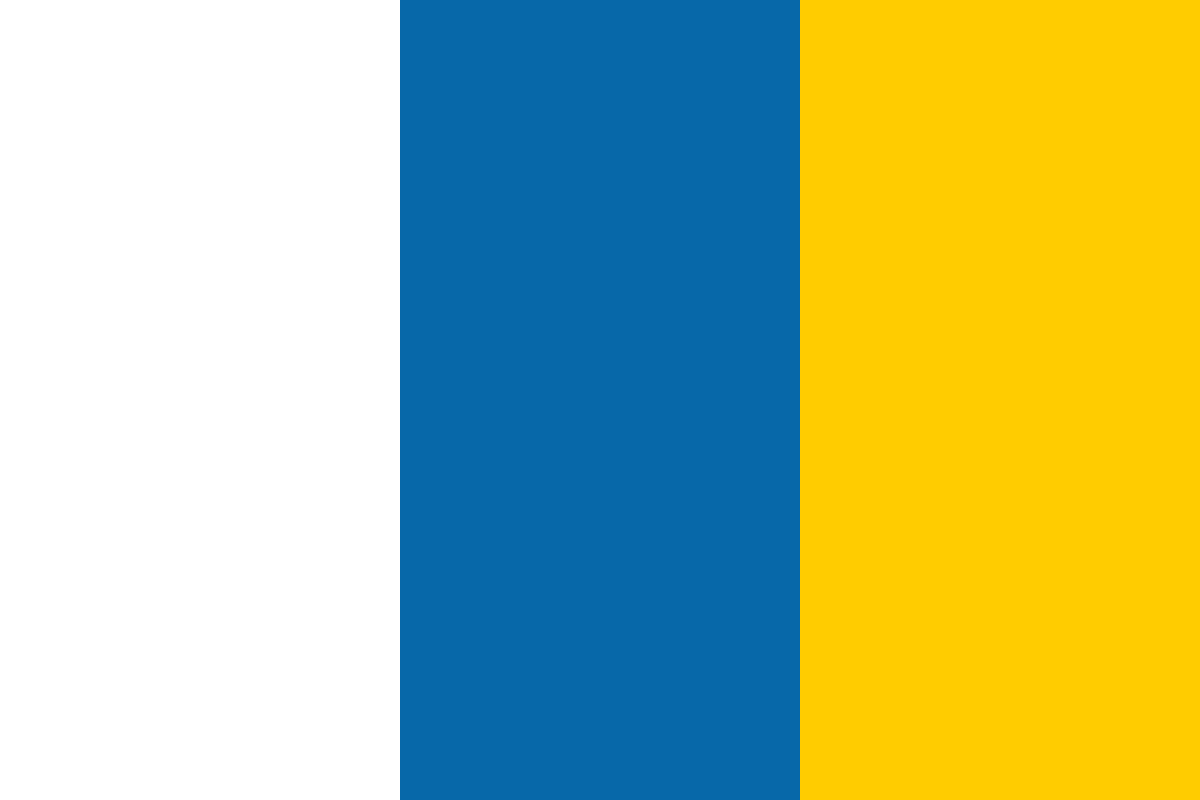

































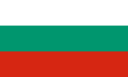








(For more resources related to this topic, see here.)
Model-View-Controller ( MVC ) is a heavily used design pattern in programming. A design pattern is essentially a reusable solution that solves common problems in programming. For example, the Namespace and Immediately-Invoked Function Expressions are patterns that are used throughout this article. MVC is another pattern to help solve the issue of separating the presentation and data layers. It helps us keep our markup and styling outside of the JavaScript; keeping our code organized, clean, and manageable—all essential requirements for creating one-page-applications. So let's briefly discuss the several parts of MVC, starting with models.
A model is a description of an object, containing the attributes and methods that relate to it. Think of what makes up a song, for example the track's title, artist, album, year, duration, and more. In its essence, a model is a blueprint of your data.
The view is a physical representation of the model. It essentially displays the appropriate attributes of the model to the user, the markup and styles used on the page. Accordingly, we use templates to populate our views with the data provided.
Controllers are the mediators between the model and the view. The controller accepts actions and communicates information between the model and the view if necessary. For example, a user can edit properties on a model; when this is done the controller tells the View to update according to the user's updated information.
The relationship established in an MVC application is critical to sticking with the design pattern. In MVC, theoretically, the model and view never speak with each other. Instead the controller does all the work; it describes an action, and when that action is called either the model, view, or both update accordingly. This type of relationship is established in the following diagram:
This diagram explains a traditional MVC structure, especially that the communication between the controller and model is two-way; the controller can send data to/from the model and vice versa for the view. However, the view and model never communicate, and there's a good reason for that. We want to make sure our logic is contained appropriately; therefore, if we wanted to delegate events properly for user actions, then that code would go into the view.
However, if we wanted to have utility methods, such as a getName method that combines a user's first name and last name appropriately, that code would be contained within a user model. Lastly, any sort of action that pertains to retrieving and displaying data would be contained in the controller.
Theoretically, this pattern helps us keep our code organized, clean, and efficient. In many cases this pattern can be directly applied, especially in many backend languages like Ruby, PHP, and Java. However, when we start applying this strictly to the frontend, we are confronted with many structural challenges. At the same time, we need this structure to create solid one-page-applications. The following sections will introduce you to the libraries we will use to solve these issues and more.
One of the libraries we will be utilizing in our sample application will be Underscore.js. Underscore has become extremely popular in the last couple of years due to the many utility methods it provides developers without extending built-in JavaScript objects, such as String, Array, or Object. While it provides many useful methods, the suite has also been optimized and tested across many of the most popular web browsers, including Internet Explorer. For these reasons, the community has widely adopted this library and continually supported it.
Underscore is extremely easy to implement in our applications. In order to get Underscore going, all we need to do is include it on our page like so:
<!DOCTYPE html> <html> <head> <meta charset="utf-8"> <meta http-equiv="X-UA-Compatible" content="IE=edge,chrome=1"> <title></title> <meta name="description" content=""> <meta name="viewport" content="width=device-width"> </head> <body> <script src = "//ajax.googleapis.com/ajax/libs/jquery/
1.9.0/jquery.min.js"></script> <script src = "//cdnjs.cloudflare.com/ajax/libs/underscore.js/
1.4.3/underscore-min.js"></script> </body> </html>
Once we include Underscore on our page, we have access to the library at the global scope using the _ object. We can then access any of the utility methods provided by the library by doing _.methodName. You can review all of the methods provided by Underscore online (http://underscorejs.org/), where all methods are documented and contain samples of their implementation. For now, let's briefly review some of the methods we'll be using in our application.
The extend method in Underscore is very similar to the extend method we have been using from Zepto (http://zeptojs.com/#$.extend). If we look at the documentation provided on Underscore's website (http://underscorejs.org/#extend), we can see that it takes multiple objects with the first parameter being the destination object that gets returned once all objects are combined.
Copy all of the properties in the source objects over to the destination object, and return the destination object. It's in-order, so the last source will override properties of the same name in previous arguments.
As an example, we can take a Song object and create an instance of it while also overriding its default attributes. This can be seen in the following example:
<script> function Song() { this.track = "Track Title"; this.duration = 215; this.album = "Track Album"; }; var Sample = _.extend(new Song(), { 'track': 'Sample Title', 'duration': 0, 'album': 'Sample Album' }); </script>
If we log out the Sample object, we'll notice that it has inherited from the Song constructor and overridden the default attributes track, duration, and album. Although we can improve the performance of inheritance using traditional JavaScript, using an extend method helps us focus on delivery. We'll look at how we can utilize this method to create a base architecture within our sample application later on in the article.
The each method is extremely helpful when we want to iterate over an Array or Object. In fact this is another method that we can find in Zepto and other popular libraries like jQuery. Although each library's implementation and performance is a little different, we'll be using Underscore's _.each method, so that we can stick within our application's architecture without introducing new dependencies. As per Underscore's documentation (http://underscorejs.org/#each), the use of _.each is similar to other implementations:
Iterates over a list of elements, yielding each in turn to an iterator function. The iterator is bound to the context object, if one is passed. Each invocation of iterator is called with three arguments: (element, index, list). If list is a JavaScript object, iterator's arguments will be (value, key, list). Delegates to the native forEach function if it exists.
Let's take a look at an example of using _.each with the code we created in the previous section. We'll loop through the instance of Sample and log out the object's properties, including track, duration, and album. Because Underscore's implementation allows us to loop through an Object, just as easily as an Array, we can use this method to iterate over our Sample object's properties:
<script> function Song() { this.track = "Track Title"; this.duration = 215; this.album = "Track Album"; }; var Sample = _.extend(new Song(), { 'track': 'Sample Title', 'duration': 0, 'album': 'Sample Album' }); _.each(Sample, function(value, key, list){ console.log(key + ": " + value); }); </script>
The output from our log should look something like this:
track: Sample Title duration: 0 album: Sample Album
As you can see, it's extremely easy to use Underscore's each method with arrays and objects. In our sample application, we'll use this method to loop through an array of objects to populate our page, but for now let's review one last important method we'll be using from Underscore's library.
Underscore has made it extremely easy for us to integrate templating into our applications. Out of the box, Underscore comes with a simple templating engine that can be customized for our purposes. In fact, it can also precompile your templates for easy debugging. Because Underscore's templating can interpolate variables, we can utilize it to dynamically change the page as we wish. The documentation provided by Underscore (http://underscorejs.org/#template) helps explain the different options we have when using templates:
Compiles JavaScript templates into functions that can be evaluated for rendering. Useful for rendering complicated bits of HTML from JSON data sources. Template functions can both interpolate variables, using <%= … %>, as well as execute arbitrary JavaScript code, with <% … %>. If you wish to interpolate a value, and have it be HTML-escaped, use <%- … %>. When you evaluate a template function, pass in a data object that has properties corresponding to the template's free variables. If you're writing a one-off, you can pass the data object as the second parameter to template in order to render immediately instead of returning a template function.
Templating on the frontend can be difficult to understand at first, after all we were used to querying a backend, using AJAX, and retrieving markup that would then be rendered on the page. Today, best practices dictate we use RESTful APIs that send and retrieve data. So, theoretically, you should be working with data that is properly formed and can be interpolated. But where do our templates live, if not on the backend? Easily, in our markup:
<script type="tmpl/sample" id="sample-song"> <section> <header> <h1><%= track %></h1> <strong><%= album %></strong> </header> </section> </script>
Because the preceding script has an identified type for the browser, the browser avoids reading the contents inside this script. And because we can still target this using the ID, we can pick up the contents and then interpolate it with data using Underscore's template method:
<script> function Song() { this.track = "Track Title"; this.duration = 215; this.album = "Track Album"; }; var Sample = _.extend(new Song(), { 'track': 'Sample Title', 'duration': 0, 'album': 'Sample Album' }); var template = _.template(Zepto('#sample-song').html(), Sample); Zepto(document.body).prepend(template); </script>
The result of running the page, would be the following markup:
<body> <section> <header> <h1>Sample Title</h1> <strong>Sample Album</strong> </header> </section> <!-- scripts and template go here --> </body>
As you can see, the content from within the template would be prepended to the body and the data interpolated, displaying the properties we wish to display; in this case the title and album name of the song. If this is a bit difficult to understand, don't worry about it too much, I myself had a lot of trouble trying to pick up the concept when the industry started moving into one-page applications that ran off raw data (JSON).
For now, these are the methods we'll be using consistently within the sample application to be built in this article. It is encouraged that you experiment with the Underscore.js library to discover some of the more advanced features that make your life easier, such as _.map, _.reduce, _.indexOf, _.debounce, and _.clone. However, let's move on to Backbone.js and how this library will be used to create our application.