










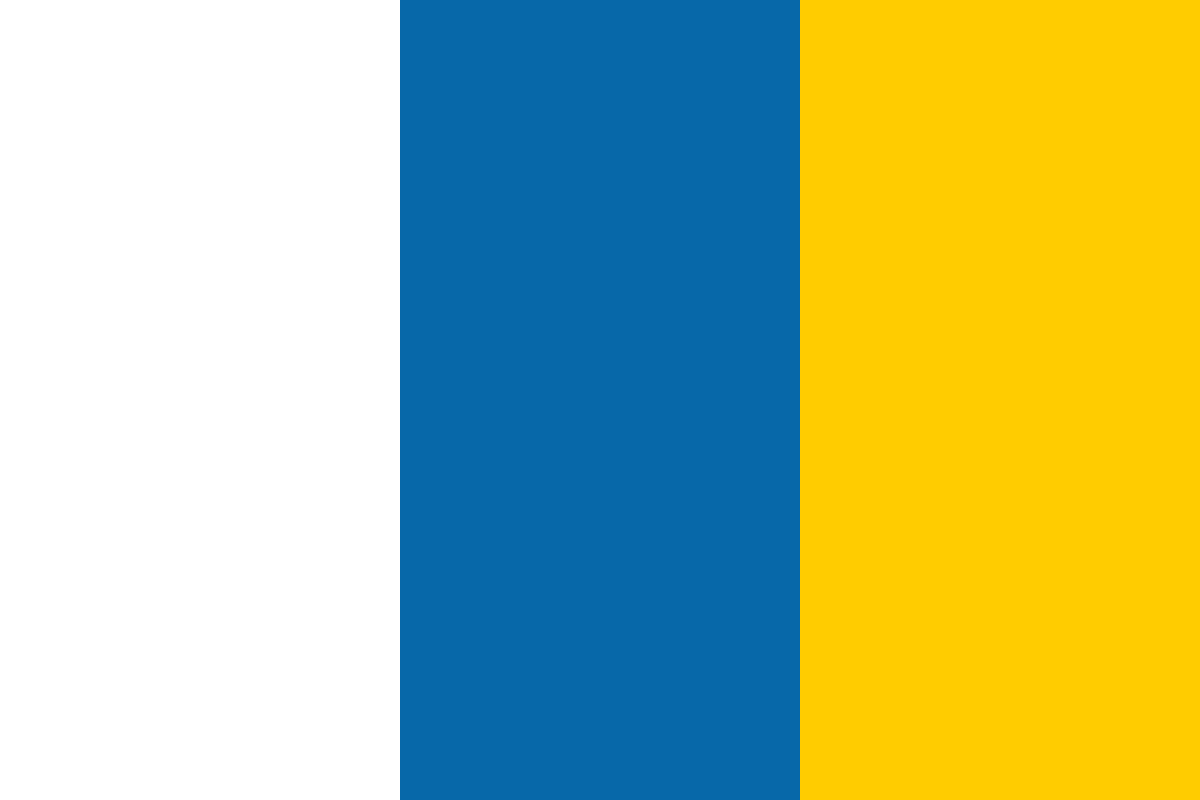

































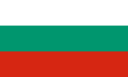








These components allow you to develop native 32-bit Windows applications without any proprietary third-party software. All components of MinGW software are produced under GNU General Public License and therefore this is a free software that you can download, use, and change as you want.
You can develop applications in C, C++, Java, Objective C, Fortran, and Ada programming languages with MinGW software. C++ application development is more typical for MinGW usage.
Besides developing new applications, you can import existing Visual C++ projects to MinGW software. It is easy to integrate MinGW with well-known third-party libraries such as DirectX, Boost, Qt, GTK, OpenGL, and SDL. If you are using any of these libraries, you can compile your application with MinGW.
MinGW software is very useful for importing Unix and Mac applications to Windows native code. It provides the same instruments that Unix and Mac developers have used in most cases. Also, you can import your MinGW-based applications to any computing platform supported by the GNU toolchain. Therefore, MinGW software is a great instruments' set for developing cross-platform applications.
Another benefit of MinGW software is modular organization. You can replace most components of the GNU toolchain with your favorite instruments (for example, debugger, profiler, or build automation system). These instruments will be integrated with existing components without any problems. Usage of the MinGW software is the first step to collecting your own developer's instruments' set for comfortable work.
The compiler efficiency is one of most important parameters for software developers. There are a lot of C++ compilers' benchmarks that are available on the Internet. Unfortunately for us, developers of proprietary compilers are not interested in objective researches of this kind. Fair comparison of available compilers is impossible because of this.
The MinGW compiler efficiency is abreast to proprietary compiler efficiency today according to benchmarks of independent software developers. You can find one of them at the following website:
http://www.willus.com/ccomp_benchmark.shtml?p9+s6
The MinGW software releases are more frequent than the proprietary compilers' releases. This means that MinGW is developed and improved more dynamically. For example, the standard features of C++11 have been supported by the GCC compiler earlier than the Visual Studio one. You can find these features at the following website:
http://wiki.apache.org/stdcxx/C++0xCompilerSupport
Notice that the GNU toolchain is a product of Unix culture. This culture is earlier than GUI applications with access to any function through menus, dialogs, and icons. Unix software has been developed as a suite of little stand alone utilities. Each of these performs only one task, but this execution is optimized very well. Therefore, all these utilities have a text-based interface. This provides the simplest intercommunication mechanism with a command line shell and saves the system resources.
If the idea of a text-based interface scares you, be relieved because there are a lot of Integrated Development Environments ( IDE ) that support MinGW.
MinGW software allows you to develop applications based on any library compiled with the MinGW C++ compiler. Open source libraries are often supplied in Visual C++ and MinGW compilation variants. Moreover, you can always get the source code of these libraries and build them with your MinGW software. Several well-known open source cross-platform frameworks and toolkits are described as follows:
Qt framework
Gtk+ widget toolkit
wxWidgets framework
The same functionality example application will be developed with each of these libraries. The goal of these examples is to show the first steps to deploy and to start working with these libraries.
Our example application consists of a window with two buttons. A click on the first button leads to the display of a message and a click on the second button leads to the application termination.
The Qt framework provides not only a cross-platform widget toolkit for GUI development but also contains features for SQL database access, XML parsing, thread management, interaction over a network, and internalization support. The Qt framework has its own container classes such as Qstring or QVector and a set of well-known algorithms such as sorting, searching, and copying to process data in these containers that allow you to substitute the capabilities of STL and Boost libraries by the Qt ones. This kind of Qt framework self-sufficiency is of great advantage to develop cross-platform applications. All you need to do to import your Qt application to a new platform is building a Qt framework for this one. There are a lot of platforms that are supported by Qt framework developers:
Windows
Mac OS X
Linux
Solaris
Symbian
Android (unofficial framework port with the name Ministro)
Let's begin our work with the Qt framework. It is important to note that Qt libraries have been compiled with the specific MinGW software version. Your application must be compiled with the same MinGW version and therefore you must install it. The alternative way is the compilation of Qt libraries sources with your already installed MinGW software. You can find some helpful instructions to do this at the following website:
http://www.formortals.com/build-qt-static-small-microsoft-intel-gcc-compiler
All other toolkits described here don't need a specific MinGW software version. The following are instructions to install Qt libraries version 4.6.4 and some necessary software to start developing with it:
Download the MinGW version of the Qt libraries for Windows from the following official website:
http://qt-project.org/downloads
Note that this library has been compiled with a specific (equal in our case to 4.4) MinGW software version.
Install the downloaded Qt libraries with the setup wizard. Run the downloaded exe file to do it.
Download the MinGW software of version 4.4 from the following official download page:
http://sourceforge.net/projects/mingw/files/MinGW/Base/gcc/Version4/Previous%20Release%20gcc-4.4.0/
You need the file to be named gcc-full-4.4.0-mingw32-bin-2.tar.lzma. This is an archive with the MinGW compilers' executable files and necessary core libraries.
Extract the downloaded MinGW software archive to the directory without spaces in the path (for example, C:MinGW4.4).
You can use the 7-Zip application to extract the LZMA archive type. This application is available at the following official website:
Download the GNU Binutils for MinGW software from the following official download page:
http://sourceforge.net/projects/mingw/files/MinGW/Base/binutils/binutils-2.19
You need the archive to be named binutils-2.19-2-mingw32-bin.tar.gz.
Extract the downloaded GNU Binutils archive to the same directory as the MinGW software. This is C:MinGW4.4 in our case.
Download the GNU Make utility from the following web page:
http://sourceforge.net/projects/mingw/files/MinGW/Extension/make/mingw32-make-3.80-3
You need to download the mingw32-make-3.80.0-3.exe file.
Install GNU Make utility to the MinGW software directory (C:MinGW4.4). Run the downloaded exe file and use the setup wizard to do it.
Add the installation MinGW software path to the PATH Windows environment variable. Remove the existing paths of other MinGW software installations from there. The C:MinGW4.4bin path must be added in our example.
Now you have the necessary libraries and specific MinGW software version to start developing an application based on the Qt framework.
Our example application is implemented in the main.cpp source file. The following is the main function definition:
int main(int argc, char* argv[]) { QApplication application(argc, argv); QMainWindow* window = CreateWindow(); CreateMsgButton(window); CreateQuitButton(window, application); return application.exec(); }
First of all, the object of the QApplication class named application is created here. This object is used to manage the application's control flow and main settings. After that, the window of the QMainWindow class named window is created by the CreateWindow function. Two window buttons are created by the CreateMsgButton and CreateQuitButton functions. The exec method of the application object is called to enter the main event loop when all user interface objects have been created. Now the application starts processing events such as button pressing.
The following is the CreateWindow function that encapsulates the main application window creation:
QMainWindow* CreateWindow() { QMainWindow* window = new QMainWindow(0, Qt::Window); window->resize(250, 150); window->setWindowTitle("Qt Application"); window->show(); return window; }
The main application window is an object of the QMainWindow class named window . It is created by a constructor that has two input parameters. The first parameter is of the QWidget* type. This is a pointer to the window's parent widget. It equals to zero in our case which means that there is no parent widget. The second parameter is of the Qt::WindowFlags type and defines the window style. It equals to Qt::Window that matches the standard window appearance with the system frame and title bar.
After the main window creation its size is set with the resize method of the window object. Then the window title is set with the setWindowTitle method of the window object. The next action is to make the main window visible by using the show method of the window object. The function returns the pointer to the created window object.
The following code shows the CreateMsgButton function that encapsulates the creation of button with message showing action:
void CreateMsgButton(QMainWindow* window) { QMessageBox* message = new QMessageBox(window); message->setText("Message text"); QPushButton* button = new QPushButton("Message", window); button->move(85, 40); button->resize(80, 25); button->show(); QObject::connect(button, SIGNAL(released()), message, SLOT(exec())); }
At first, we need to create a message window to display it when we click on the button. This message window is an object of the QMessageBox class named message. It is created by a constructor with one input parameter of the QWidget* type. This is a pointer to the parent widget. The text displayed in the message window is set by the setText method of the message object.
Now we need a button that will be placed at the main window. This button is the object of the QPushButton class named button. The constructor with two input parameters is used here to create this object. The first parameter is a string with the button text of the QString class. The second parameter is the parent widget pointer. The position and size of the button object are set by the move and resize methods. After configuring the button we make it visible by using the show method.
Now we need to bind the button press event and message window displaying. The connect static method of the QObject class is used here for this goal. It allows to create a connection between the button object's release signal and the exec method of the message object. The exec method causes the displaying of window with message.
The following code shows the CreateQuitButton function that encapsulates the creation of the close application button:
void CreateQuitButton(QMainWindow* window, QApplication& application) { QPushButton* quit_button = new QPushButton("Quit", window); quit_button->move(85, 85); quit_button->resize(80, 25); quit_button->show(); QObject::connect(quit_button, SIGNAL(released()), &application, SLOT(quit())); }
This function is similar the CreateMsgButton function. But the message window isn't created here. The close application button is an object of the QPushButton class named quit_button. The release signal of the quit_button button is connected to the quit method of the application object. This method leads to the application closing with successful code.
Now you are ready to build an example application. You need to perform the following instructions:
Create a Qt project file with the following command:
$ qmake -project
You will get a file with the pro extension and name of the current directory.
$ qmake
Create Makefile , service files, and subdirectories with the following command:
Compile the project with the GNU Make utility:
$ mingw32-make
The GNU Make utility executable name is mingw32-make in the official distribution. This one has been installed with the MinGW software of version 4.4.
You will get the qt.exe executable file in the debug subdirectory after compilation.
This is the debugging version of our example application. Type the following command to build the release version:
$ mingw32-make release
The qt.exe executable file will be created in the release subdirectory after this compilation.
We learned how to develop applications based on Qt framework with the help of the libraries and the MinGW software mentioned in this article.
Further resources on this subject: