










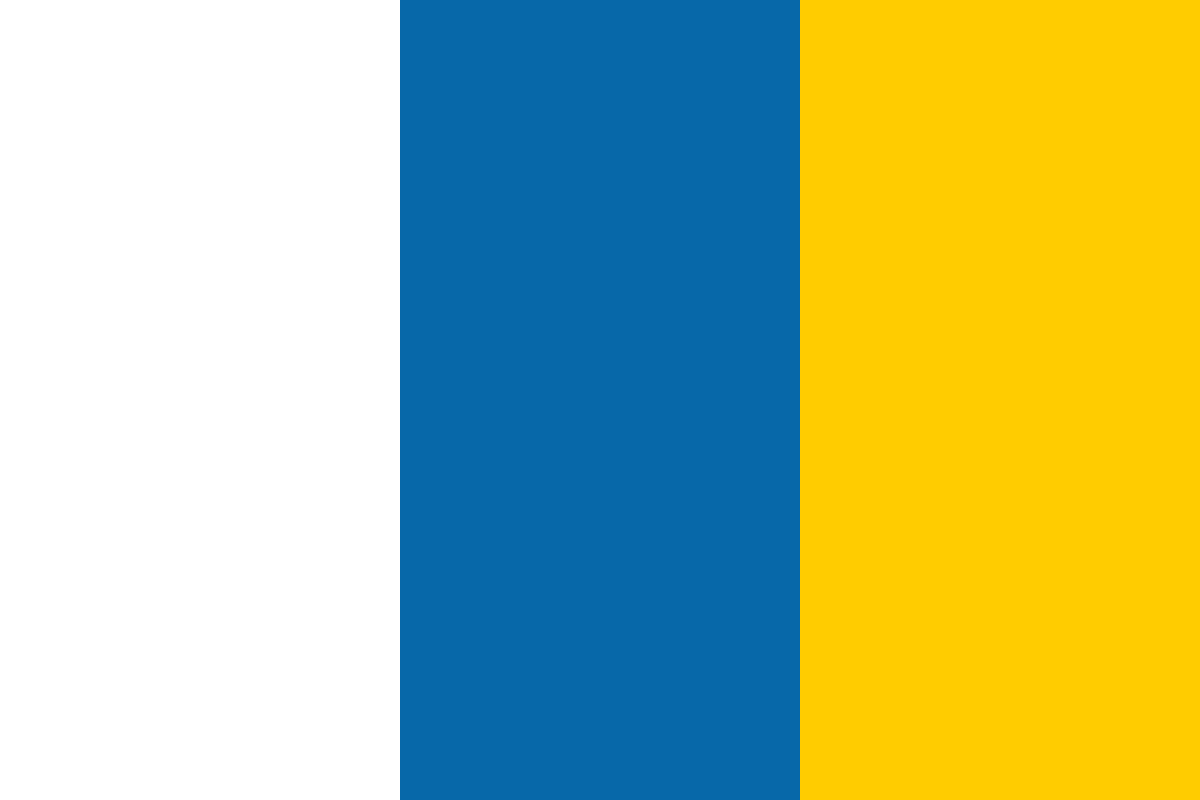

































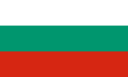








Security is a big topic in distributed communication applications. When the client consumers call a service operation through an intranet or the Internet, it is necessary to consider how we will secure the communication between the two sides, or how we can make sure that we are talking to the correct service or the correct client consumers.
WCF provides a lot of built-in features for developers to address all these kinds of problems in service application development. The most commonly used WCF security features include authentication, authorization, and message protection (signing and encrypting).
In this article, we will use 5 recipes to demonstrate some useful security scenarios in WCF service development. These recipes will focus on various authentication use cases, including Windows authentication, username authentication, and so on.
WCF supports various authentication types and Windows authentication is a common authentication method used in existing distributed communication components on the Windows platform. A very common use case is to enable Windows authentication at transport layer without an additional secure connection like SSL (just like what the traditional ASMX Web Service uses).
In this recipe, we will demonstrate how to apply Windows authentication for a WCF service endpoint using plain HTTP as the transport protocol, without additional security.
After the service endpoint has been properly configured as ad hoc Windows authentication mode, the client consumer can use the generated proxy class or ChannelFactory to invoke the target service operations. Also, either the service proxy or ChannelFactory provides the ClientCredential property for the caller to supply their Windows credentials (see the following code snippet):
static void CallService()
{
TestProxy.Service1Client client = new TestProxy.Service1Client();
client.ClientCredentials.Windows.ClientCredential =
System.Net.CredentialCache.DefaultNetworkCredentials;
Console.WriteLine( client.GetData(11));
}
WCF provides various built-in authentication methods either at the transport layer or at the message layer. The client consumer can use a WCF service proxy or ChannelFactory to supply certain client credentials to the service. The following MSDN reference lists all the built-in credential types supported by WCF:
After the service request passes authentication, a valid identity will be associated with each service-operation execution context and the service operation code can retrieve the identity information within the operation context.
This recipe will show you how to programmatically retrieve the client authenticated identity information in service operation code.
WCF runtime provides an OperationContext object associated with each request processing so that the developers can access some operation/request context-specific data from it. For example, we can access and manipulate SOAP headers or other underlying transport protocol properties through OperationContext. For operation authentication, the authenticated identity is also accessible through OperationContext, and the minor difference is that we need to get the identity by a ServiceSecurityContext member of the OperationContext object. The ServiceSecurityContext type contains several member properties, which represent security information transferred from the client side.
Name
Description
Anonymous
Returns an instance of the ServiceSecurityContext class that contains an empty collection of claims, identities, and other context data usually used to represent an anonymous party.
AuthorizationContext
Gets the authorization information for an instance of this class. The AuthorizationContext contains a collection of ClaimSet that the application can interrogate, and retrieve the information of the party.
AuthorizationPolicies
Gets the collection of policies associated with an instance of this class.
Current
Gets the current ServiceSecurityContext.
IsAnonymous
Gets a value that indicates whether the current client has provided credentials to the service.
PrimaryIdentity
Gets the primary identity associated with the current setting.
WindowsIdentity
Gets the Windows identity of the current setting.
The WindowsIdentity and PrimaryIdentity properties are the corresponding members which contain the authentication identity information of the client service caller. We can inspect the identity details such as identity name or authentication type from the two properties. The following screenshot shows the code for obtaining main authentication identity information from the PrimaryIdentity property.
Likewise, we can use the Windows Identity property to get the Windows security identity associated with the current operation call (as shown in the following screenshot):