










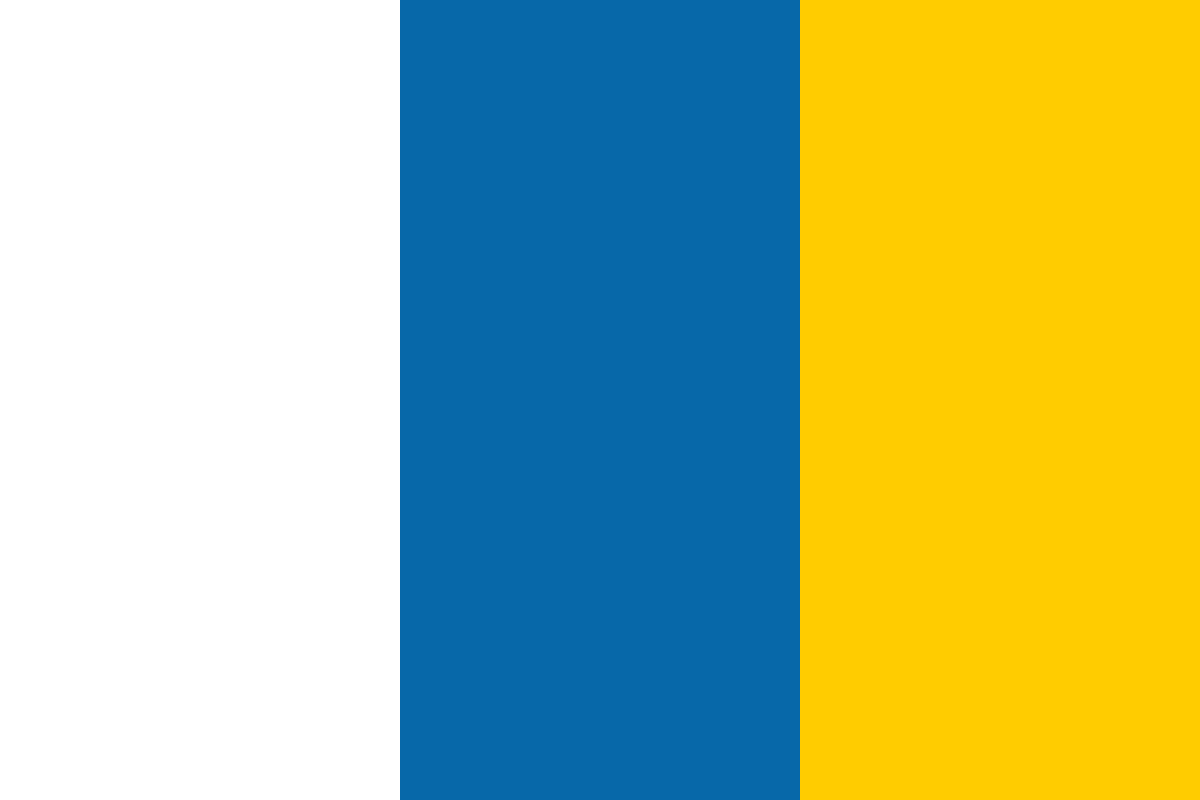

































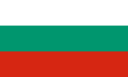








Programming in Microsoft Office Outlook differs from programming other Microsoft applications such as InfoPath and Excel. Most of the Microsoft Office applications target documents rather than data items, but Microsoft Office Outlook targets each data item stored in the database or the primary data storage used by an Outlook application. Microsoft Office Outlook stores and manages data items such as emails, appointments, notes, tasks, and contacts in tables in a structured database.
The Microsoft Office Outlook object model is based on COM (Component Object Model) and is used to interact with Outlook form regions, menus, and other application customization possibilities. It is similar to other Microsoft Office application object models when thought of with respect to the object model implementation interfaces for collections, objects, properties, methods, and events.
COM is a language-neutral way of implementing objects that can be used in different environments.
A large numbers of objects are available for developing and customizing Outlook 2007. If the developer needs to develop application add-ins for Outlook, he or she can program using the objects provided by the Outlook object model. In the Outlook object model, the class will represent each object in the UI to the user. For example, the Microsoft.Office.Interop.Outlook.Application class represents the entire application, and the Microsoft.Office.Interop.Outlook.MailItem class represents an email message.
To get used to the object models in Outlook, you should be familiar with some of the top-level objects. The Application object represents an Outlook application, and it is the highest level class in the Outlook object model. The Explorer object corresponds to the window that displays the contents of a folder, and contains Outlook data items such as email messages, tasks, appointments, and so on. The MAPIFolder object represents the folder that contains emails, contacts, tasks, and other Outlook data items. By default, there are sixteen MAPIFolder objects available. The Inspector object corresponds to a window that displays a single item such as a particular email message, or a specific contact item.
Let's see how to create application-level add-ins for Microsoft Office Outlook 2007 using VSTO 3.0. We will create a Hello World application-level example for Microsoft Office Outlook 2007 using Visual Studio 2008.
// Windows forms namespace to display Message box
using System.Windows.Forms;
private void ThisAddIn_Startup(object sender, System.EventArgs e)
{
// Message box display
MessageBox.Show("Say Hello World!");
}
The result will be similar to what is shown in the following screenshot:
Outlook 2007 object models are categorized and mapped correspondingly. The following Outlook object models hold all of Outlook's objects for programming in their corresponding object model categories: Items object model, Navigation bars and Outlook bars object model, Rules object model, and Views object model. In the Application object model hierarchy, the Application object is the parent of all other Outlook objects.
Most enterprise business applications are loaded with a wide variety of features for users. Even though these applications have a wide range of features, many business requirements can be fulfilled only through customization because not all applications are designed to fit each and every enterprise's special needs. Customization for most of the application is a tough job to execute.
Outlook 2007 is loaded with a wide variety of features that will satisfy a broad range of user categories. Some organizations need more functionality and features to be added, so that the application will satisfy their custom business requirements. VSTO 3.0 helps Office developers to customize and enhance the Outlook 2007 application as per the user's business requirements.
Microsoft Office Outlook 2007 supports other Microsoft Office tools such as InfoPath and Excel, in order to provide seamless collaboration.
VSTO provides an easy way to create an application-level add-in for Outlook 2007 using Visual Studio 2008. Creating add-ins for Microsoft Office Outlook 2007 has been more complex to work out, but VSTO 3.0 offers Office developers with project templates in Visual Studio 2008 to allow them to create add-ins for Outlook 2007. Adding to the project templates, VSTO offers great support for development and deployment, which improves the development work. This provides .NET framework support for Outlook 2007 add-in programming, which includes class library support, controlled exception handling, memory management, extensibility, ClickOnce deployment, and so on.
A group of commands or lists of options from which you can choose your desired operation is known as a menu. Most of the latest applications are menu driven. Microsoft Office Outlook, which is a menu-driven application, provides the user with an easy fl owing UI for user interaction. Most of the menu-driven applications will provide you with basic customization such as choosing the menu for the default view of the application, and so on.
In Microsoft Office, all menus and toolbars are CommandBars. A CommandBar is a static collection shared by all Windows. There are standard toolbars, menu bars, context menus, and so on. A small add-in enumerates and displays all Microsoft Outlook CommandBars.
Likewise, Microsoft Office Outlook 2007 provides the option for a user to customize the menus. VSTO 3.0 provides Office developers with the ability to build custom menus and customize the existing menus using the .NET framework and support a programming language. You can even rebuild the classic menu style for Outlook 2007 by using the VSTO 3.0 application level add-ins development.
Let's create a custom menu in the menu bar of the Outlook and add a new item to the menu. This way, you will get to know about custom menu development for Microsoft Office Outlook 2007.
Open Visual Studio 2008 and create a new solution, as described in the previous example.
Let's write a program to create a menu item and call it Say Hello World.
// Defining new Menubar
private Office.CommandBar PacktOldMenuBar;
// Defining old Menubar
private Office.CommandBarPopup PacktNewMenuBar;
// Defining instance of button for menu item
private Office.CommandBarButton PacktButton1;
// Tag string for our Menu item
private string strMenuString = "Outlook AddIn #1";
private void ThisAddIn_Startup(object sender,
System.EventArgs e)
{
// Define the Old Menu Bar
PacktOldMenuBar = this.Application.ActiveExplorer().
CommandBars.ActiveMenuBar;
// Define the new Menu Bar into the existing menu bar
PacktNewMenuBar = (Office.CommandBarPopup)PacktOldMenuBar.
Controls.Add(Office.MsoControlType.msoControlPopup,
missing, missing, missing, false);
//If PacktNewMenuBar not found then the code will add it
if (PacktNewMenuBar != null)
{
// Set caption for the Menu
PacktNewMenuBar.Caption = "Packt Menu Item 1";
// Tag string value passing
PacktNewMenuBar.Tag = strMenuString;
// Assigning button type
PacktButton1 = (Office.
CommandBarButton)PacktNewMenuBar.
Controls.Add(Office.MsoControlType.
msoControlButton, missing, missing, 1, true);
// Setting up the button style
PacktButton1.Style = Office.MsoButtonStyle.
msoButtonIconAndCaptionBelow;
// Set button caption
PacktButton1.Caption = "Say Hello World";
// Set the menu visible
PacktNewMenuBar.Visible = true;
}
}
The following screenshot displays the resulting menu developed by you using the preceding code:
You can also build more custom menus for your Outlook 2007 with functionality as per your requirements. VSTO will speed up development and provide support for a hassle free environment for the developer to work on Outlook 2007 add-ins and other customization.
Generally, toolbars provide easy access to the functionality of the application by using buttons and menus. Most application's user interfaces have a toolbar that has buttons, menus, and input or output control elements for user interaction with the application. Even applications allow users to do visual customization of toolbars as per the users' needs.
Microsoft Office Outlook 2007 provides a very good visual representation of toolbars for user interaction with the application. Outlook provides support for toolbar customization to improve custom visual interaction for users. VSTO 3.0 offers wide options to build custom toolbars and to customize existing toolbars as per the user's needs.
Remember that menus and CommandBars are not VSTO features, but are in the Microsoft.Office namespace. VSTO is making it easier to program for the Office object model.
Let's see a demonstration of creating a custom toolbar with a button.
// Namespace reference for message box
using System.Windows.Forms;
Office.CommandBar PacktCustomToolBar;
// Declare the button
Office.CommandBarButton PacktButtonA;
private void ThisAddIn_Startup(object sender, System.EventArgs e)
{
// Verify the PacktCustomToolBar exist and add to the application
if (PacktCustomToolBar == null)
{
// Adding the commandbar to Active explorer
Office.CommandBars PacktBars = this.Application.
ActiveExplorer().CommandBars;
// Adding PacktCustomToolBar to the commandbars
PacktCustomToolBar = PacktBars.Add("NewPacktToolBar",
Office.MsoBarPosition.msoBarTop, false, true);
}
// Adding button to the custom tool bar
Office.CommandBarButton MyButton1 = (Office.
CommandBarButton)PacktCustomToolBar.Controls.Add(1,
missing, missing, missing, missing);
// Set the button style
MyButton1.Style = Office.MsoButtonStyle.msoButtonCaption;
// Set the caption and tag string
MyButton1.Caption = "PACKT BUTTON";
MyButton1.Tag = "MY BUTTON";
if (this.PacktButtonA == null)
{
// Adding the event handler for the button in the toolbar
this.PacktButtonA = MyButton1;
PacktButtonA.Click += new Office.
_CommandBarButtonEvents_ClickEventHandler(ButtonClick);
}
}
// Button event in the custom toolbar
private void ButtonClick(Office.CommandBarButton ButtonContrl,
ref bool CancelOption)
{
// Message box displayed on button click
MessageBox.Show(ButtonContrl.Caption + " Says Hello World!");
}
The following image shows the results of adding a custom toolbar with button control:
The CommandBars object helps you to build variants of toolbars to your Outlook 2007 application's user interface. Microsoft Office Outlook 2007 is the only tool in the Microsoft Office 2007 family to support both standard toolbars and the new Ribbon in their UI.