










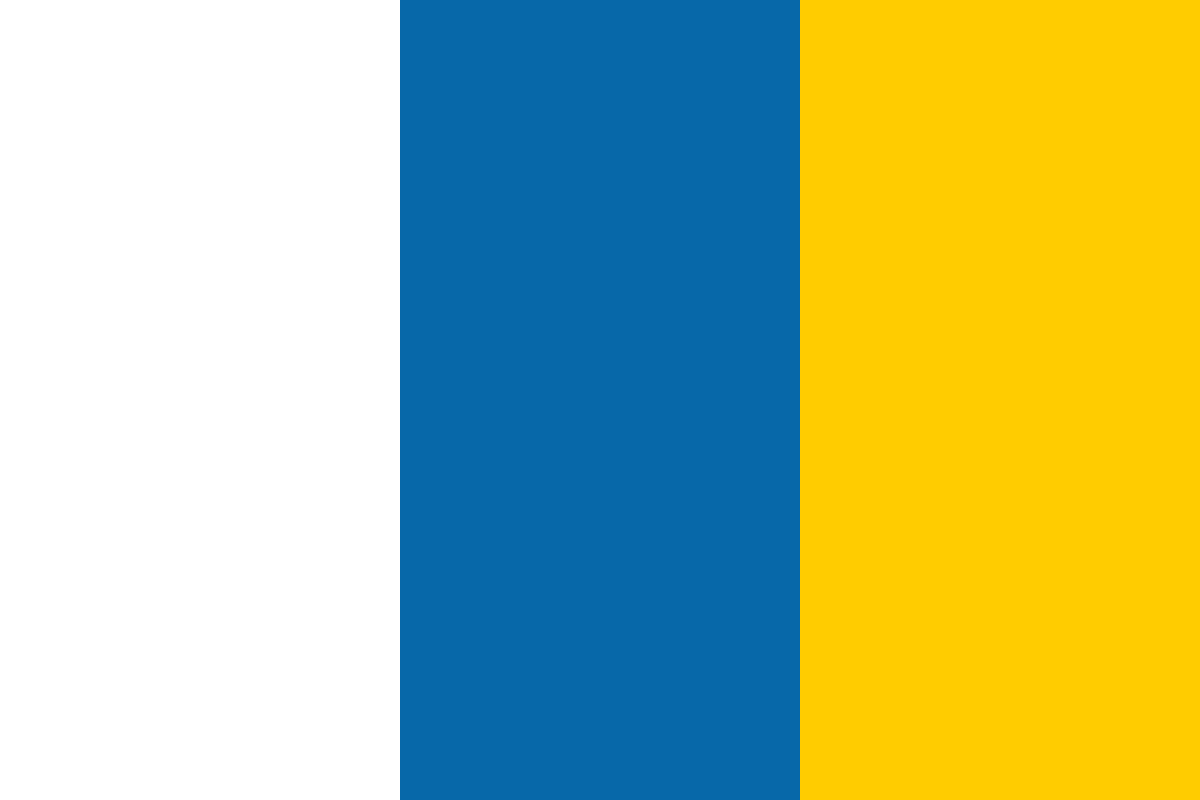

































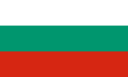








In this article by Gastón C. Hillar, the author of the book Java 9 with JShell, we will learn about one of the most exciting features of object-oriented programming in Java 9: polymorphism. We will code many classes and then we will work with their instances in JShell to understand how objects can take many different forms. We will:
(For more resources related to this topic, see here.)
We will consider the existence of an abstract base class named VirtualAnimal and the following three abstract subclasses: VirtualMammal, VirtualDomesticMammal, and VirtualHorse. Next, we will code the following three concrete classes. Each class represents a different horse breed and is a subclass of the VirtualHorse abstract class.
The three concrete classes will implement the following three abstract methods they inherited from abstract superclasses:
The following UML diagram shows the members for the three concrete classes that we will code: AmericanQuarterHorse, ShireHorse, and Thoroughbred. We don’t use bold text format for the three methods that each of these concrete classes will declare because they aren’t overriding the methods, they are implementing the abstract methods that the classes inherited.
First, we will create the AmericanQuarterHorse concrete class. The following lines show the code for this class in Java 9. Notice that there is no abstract keyword before class, and therefore, our class must make sure that it implements all the inherited abstract methods.
public class AmericanQuarterHorse extends VirtualHorse {
public AmericanQuarterHorse(
int age,
boolean isPregnant,
String name,
String favoriteToy) {
super(age, isPregnant, name, favoriteToy);
System.out.println("AmericanQuarterHorse created.");
}
public AmericanQuarterHorse(
int age, String name, String favoriteToy) {
this(age, false, name, favoriteToy);
}
public String getBaby() {
return "AQH baby ";
}
public String getBreed() {
return "American Quarter Horse";
}
public String getAsciiArt() {
return
" >>\.n" +
" /* )`.n" +
" // _)`^)`. _.---. _n" +
" (_,' \ `^-)'' `.\n" +
" | | \n" +
" \ / |n" +
" / \ /.___.'\ (\ (_n" +
" < ,'|| \ |`. \`-'n" +
" \\ () )| )/n" +
" |_>|> /_] //n" +
" /_] /_]n";
}
}
Now, we will create the ShireHorse concrete class. The following lines show the code for this class in Java 9:
public class ShireHorse extends VirtualHorse {
public ShireHorse(
int age,
boolean isPregnant,
String name,
String favoriteToy) {
super(age, isPregnant, name, favoriteToy);
System.out.println("ShireHorse created.");
}
public ShireHorse(
int age, String name, String favoriteToy) {
this(age, false, name, favoriteToy);
}
public String getBaby() {
return "ShireHorse baby ";
}
public String getBreed() {
return "Shire Horse";
}
public String getAsciiArt() {
return
" ;;n" +
" .;;'*\n" +
" __ .;;' ' \n" +
" /' '\.~~.~' \ /'\.)n" +
" ,;( ) / |n" +
" ,;' \ /-.,,( )n" +
" ) /| ) /|n" +
" ||(_\ ||(_\n" +
" (_\ (_\n";
}
}
Finally, we will create the Thoroughbred concrete class. The following lines show the code for this class in Java 9:
public class Thoroughbred extends VirtualHorse {
public Thoroughbred(
int age,
boolean isPregnant,
String name,
String favoriteToy) {
super(age, isPregnant, name, favoriteToy);
System.out.println("Thoroughbred created.");
}
public Thoroughbred(
int age, String name, String favoriteToy) {
this(age, false, name, favoriteToy);
}
public String getBaby() {
return "Thoroughbred baby ";
}
public String getBreed() {
return "Thoroughbred";
}
public String getAsciiArt() {
return
" })\-=--.n" +
" // *._.-'n" +
" _.-=-...-' /n" +
" {{| , |n" +
" {{\ | \ /_n" +
" }} \ ,'---'\___\n" +
" / )/\\ \\ >\n" +
" // >\ >\`-n" +
" `- `- `-n";
}
}
We have more than one constructor defined for the three concrete classes. The first constructor that requires four arguments uses the super keyword to call the constructor from the base class or superclass, that is, the constructor defined in the VirtualHorse class. After the constructor defined in the superclass finishes its execution, the code prints a message indicating that an instance of each specific concrete class has been created. The constructor defined in each class prints a different message.
The second constructor uses the this keyword to call the previously explained constructor with the received arguments and with false as the value for the isPregnant argument.
Each class returns a different String in the implementation of the getBaby and getBreed methods. In addition, each class returns a different ASCII art representation for a virtual horse in the implementation of the getAsciiArt method.
We can use the same method, that is, a method with the same name and arguments, to cause different things to happen according to the class on which we invoke the method. In object-oriented programming, this feature is known as polymorphism. Polymorphism is the ability of an object to take on many forms, and we will see it in action by working with instances of the previously coded concrete classes.
The following lines create a new instance of the AmericanQuarterHorse class named american and use one of its constructors that doesn’t require the isPregnant argument:
AmericanQuarterHorse american =
new AmericanQuarterHorse(
8, "American", "Equi-Spirit Ball");
american.printBreed();
The following lines show the messages that the different constructors displayed in JShell after we enter the previous code:
VirtualAnimal created.
VirtualMammal created.
VirtualDomesticMammal created.
VirtualHorse created.
AmericanQuarterHorse created.
The constructor defined in the AmericanQuarterHorse calls the constructor from its superclass, that is, the VirtualHorse class. Remember that each constructor calls its superclass constructor and prints a message indicating that an instance of the class is created. We don’t have five different instances; we just have one instance that calls the chained constructors of five different classes to perform all the necessary initialization to create an instance of AmericanQuarterHorse.
If we execute the following lines in JShell, all of them will display true as a result, because american belongs to the VirtualAnimal, VirtualMammal, VirtualDomesticMammal, VirtualHorse, and AmericanQuarterHorse classes.
System.out.println(american instanceof VirtualAnimal);
System.out.println(american instanceof VirtualMammal);
System.out.println(american instanceof VirtualDomesticMammal);
System.out.println(american instanceof VirtualHorse);
System.out.println(american instanceof AmericanQuarterHorse);
The results of the previous lines mean that the instance of the AmericanQuarterHorse class, whose reference is saved in the american variable of type AmericanQuarterHorse, can take on the form of an instance of any of the following classes:
The following screenshot shows the results of executing the previous lines in JShell:
We coded the printBreed method within the VirtualHorse class, and we didn’t override this method in any of the subclasses. The following is the code for the printBreed method:
public void printBreed() {
System.out.println(getBreed());
}
The code prints the String returned by the getBreed method, declared in the same class as an abstract method. The three concrete classes that inherit from VirtualHorse implemented the getBreed method and each of them returns a different String. When we called the american.printBreed method, JShell displayed American Quarter Horse.
The following lines create an instance of the ShireHorse class named zelda. Note that in this case, we use the constructor that requires the isPregnant argument. As happened when we created an instance of the AmericanQuarterHorse class, JShell will display a message for each constructor that is executed as a result of the chained constructors we coded.
ShireHorse zelda =
new ShireHorse(9, true,
"Zelda", "Tennis Ball");
The next lines call the printAverageNumberOfBabies and printAsciiArt instance methods for american, the instance of AmericanQuarterHorse, and zelda, which is the instance of ShireHorse.
american.printAverageNumberOfBabies();
american.printAsciiArt();
zelda.printAverageNumberOfBabies();
zelda.printAsciiArt();
We coded the printAverageNumberOfBabies and printAsciiArt methods in the VirtualAnimal class, and we didn’t override them in any of its subclasses. Hence, when we call these methods for either american or zelda, Java will execute the code defined in the VirtualAnimal class.
The printAverageNumberOfBabies method uses the int value returned by the getAverageNumberOfBabies and the String returned by the getBaby method to generate a String that represents the average number of babies for a virtual animal. The VirtualHorse class implemented the inherited getAverageNumberOfBabies abstract method with code that returns 1. The AmericanQuarterHorse and ShireHorse classes implemented the inherited getBaby abstract method with code that returns a String that represents a baby for the virtual horse breed: "AQH baby" and "ShireHorse baby". Thus, our call to the printAverageNumberOfBabies method will produce different results in each instance because they belong to a different class.
The printAsciiArt method uses the String returned by the getAsciiArt method to print the ASCII art that represents a virtual horse. The AmericanQuarterHorse and ShireHorse classes implemented the inherited getAsciiArt abstract method with code that returns a String with the ASCII art that is appropriate for each virtual horse that the class represents. Thus, our call to the printAsciiArt method will produce different results in each instance because they belong to a different class.
The following screenshot shows the results of executing the previous lines in JShell. Both instances run the same code for the two methods that were coded in the VirtualAnimal abstract class. However, each class provided a different implementation for the methods that end up being called to generated the result and cause the differences in the output.
The following lines create an instance of the Thoroughbred class named willow, and then call its printAsciiArt method. As happened before, JShell will display a message for each constructor that is executed as a result of the chained constructors we coded.
Thoroughbred willow =
new Thoroughbred(5,
"Willow", "Jolly Ball");
willow.printAsciiArt();
The following screenshot shows the results of executing the previous lines in JShell. The new instance is from a class that provides a different implementation of the getAsciiArt method, and therefore, we will see a different ASCII art than in the previous two calls to the same method for the other instances.
The following lines call the neigh method for the instance named willow with a different number of arguments. This way, we take advantage of the neigh method that we overloaded four times with different arguments. Remember that we coded the four neigh methods in the VirtualHorse class and the Thoroughbred class inherits the overloaded methods from this superclass through its hierarchy tree.
willow.neigh();
willow.neigh(2);
willow.neigh(2, american);
willow.neigh(3, zelda, true);
american.nicker();
american.nicker(2);
american.nicker(2, willow);
american.nicker(3, willow, true);
The following screenshot shows the results of calling the neigh and nicker methods with the different arguments in JShell:
We called the four versions of the neigh method defined in the VirtualHorse class for the Thoroughbred instance named willow. The third and fourth lines that call the neigh method specify a value for the otherDomesticMammal argument of type VirtualDomesticMammal. The third line specifies american as the value for otherDomesticMammal and the fourth line specifies zelda as the value for the same argument. Both the AmericanQuarterHorse and ShireHorse concrete classes are subclasses of VirtualHorse, and VirtualHorse is a subclass or VirtualDomesticMammal. Hence, we can use american and zelda as arguments where a VirtualDomesticMammal instance is required.
Then, we called the four versions of the nicker method defined in the VirtualHorse class for the AmericanQuarterHorse instance named american. The third and fourth lines that call the nicker method specify willow as the value for the otherDomesticMammal argument of type VirtualDomesticMammal. The Thoroughbred concrete class is also a subclass of VirtualHorse, and VirtualHorse is a subclass or VirtualDomesticMammal. Hence, we can use willow as an argument where a VirtualDomesticMammal instance is required.
We will code the VirtualDomesticCat abstract class and its concrete subclass: MaineCoon. Then, we will code the VirtualBird abstract class, its VirtualDomesticBird abstract subclass and the Cockatiel concrete subclass. Finally, we will code the VirtualDomesticRabbit concrete class. While coding these classes, we will use Java 9 features that allow us to decide whether the subclasses can or cannot override specific members.
All the virtual domestic cats must be able to talk, and therefore, we will override the talk method inherited from VirtualDomesticMammal to print the word that represents a cat meowing: "Meow". We also want to provide a method to print "Meow" a specific number of times. Hence, at this point, we realize that we can take advantage of the printSoundInWords method we had declared in the VirtualHorse class.
We cannot access this instance method in the VirtualDomesticCat abstract class because it doesn’t inherit from VirtualHorse. Thus, we will move this method from the VirtualHorse class to its superclass: VirtualDomesticMammal.
We will use the final keyword before the return type for the methods that we don’t want to be overridden in subclasses. When a method is marked as a final method, the subclasses cannot override the method and the Java 9 compiler shows an error if they try to do so.
Not all the birds are able to fly in real-life. However, all our virtual birds are able to fly, and therefore, we will implement the inherited isAbleToFly abstract method as a final method that returns true. This way, we make sure that all the classes that inherit from the VirtualBird abstract class will always run this code for the isAbleToFly method and that they won’t be able to override it.
The following UML diagram shows the members for the new abstract and concrete classes that we will code. In addition, the diagram shows the printSoundInWords method moved from the VirtualHorse abstract class to the VirtualDomesticMammal abstract class.
First, we will create a new version of the VirtualDomesticMammal abstract class. We will add the printSoundInWords method that we have in the VirtualHorse abstract class and we will use the final keyword to indicate that we don’t want to allow subclasses to override this method. The following lines show the new code for the VirtualDomesticMammal class.
public abstract class VirtualDomesticMammal extends VirtualMammal {
public final String name;
public String favoriteToy;
public VirtualDomesticMammal(
int age,
boolean isPregnant,
String name,
String favoriteToy) {
super(age, isPregnant);
this.name = name;
this.favoriteToy = favoriteToy;
System.out.println("VirtualDomesticMammal created.");
}
public VirtualDomesticMammal(
int age, String name, String favoriteToy) {
this(age, false, name, favoriteToy);
}
protected final void printSoundInWords(
String soundInWords,
int times,
VirtualDomesticMammal otherDomesticMammal,
boolean isAngry) {
String message = String.format("%s%s: %s%s",
name,
otherDomesticMammal == null ?
"" : String.format(" to %s ",
otherDomesticMammal.name),
isAngry ?
"Angry " : "",
new String(new char[times]).replace(" ",
soundInWords));
System.out.println(message);
}
public void talk() {
System.out.println(
String.format("%s: says something", name));
}
}
After we enter the previous lines, JShell will display the following messages:
| update replaced class VirtualHorse which cannot be referenced until this error is corrected:
| printSoundInWords(java.lang.String,int,VirtualDomesticMammal,boolean) in VirtualHorse cannot override printSoundInWords(java.lang.String,int,VirtualDomesticMammal,boolean) in VirtualDomesticMammal
| overridden method is final
| protected void printSoundInWords(String soundInWords, int times,
| ^---------------------------------------------------------------...
| update replaced class AmericanQuarterHorse which cannot be referenced until class VirtualHorse is declared
| update replaced class ShireHorse which cannot be referenced until class VirtualHorse is declared
| update replaced class Thoroughbred which cannot be referenced until class VirtualHorse is declared
| update replaced variable american which cannot be referenced until class AmericanQuarterHorse is declared
| update replaced variable zelda which cannot be referenced until class ShireHorse is declared
| update replaced variable willow which cannot be referenced until class Thoroughbred is declared
| update overwrote class VirtualDomesticMammal
JShell indicates us that the VirtualHorse class and its subclasses cannot be referenced until we correct an error for this class. The class declares the printSoundInWords method and overrides the recently added method with the same name and arguments in the VirtualDomesticMammal. We used the final keyword in the new declaration to make sure that any subclass cannot override it, and therefore, the Java compiler generates the error message that JShell displays.
Now, we will create a new version of the VirtualHorse abstract class. The following lines show the new version that removes the printSoundInWords method and uses the final keyword to make sure that many methods cannot be overridden by any of the subclasses. The declarations that use the final keyword to avoid the methods to be overridden are highlighted in the next lines.
public abstract class VirtualHorse extends VirtualDomesticMammal {
public VirtualHorse(
int age,
boolean isPregnant,
String name,
String favoriteToy) {
super(age, isPregnant, name, favoriteToy);
System.out.println("VirtualHorse created.");
}
public VirtualHorse(
int age, String name, String favoriteToy) {
this(age, false, name, favoriteToy);
}
public final boolean isAbleToFly() {
return false;
}
public final boolean isRideable() {
return true;
}
public final boolean isHervibore() {
return true;
}
public final boolean isCarnivore() {
return false;
}
public int getAverageNumberOfBabies() {
return 1;
}
public abstract String getBreed();
public final void printBreed() {
System.out.println(getBreed());
}
public final void printNeigh(
int times,
VirtualDomesticMammal otherDomesticMammal,
boolean isAngry) {
printSoundInWords("Neigh ", times, otherDomesticMammal,
isAngry);
}
public final void neigh() {
printNeigh(1, null, false);
}
public final void neigh(int times) {
printNeigh(times, null, false);
}
public final void neigh(int times,
VirtualDomesticMammal otherDomesticMammal) {
printNeigh(times, otherDomesticMammal, false);
}
public final void neigh(int times,
VirtualDomesticMammal otherDomesticMammal,
boolean isAngry) {
printNeigh(times, otherDomesticMammal, isAngry);
}
public final void printNicker(int times,
VirtualDomesticMammal otherDomesticMammal,
boolean isAngry) {
printSoundInWords("Nicker ", times, otherDomesticMammal,
isAngry);
}
public final void nicker() {
printNicker(1, null, false);
}
public final void nicker(int times) {
printNicker(times, null, false);
}
public final void nicker(int times,
VirtualDomesticMammal otherDomesticMammal) {
printNicker(times, otherDomesticMammal, false);
}
public final void nicker(int times,
VirtualDomesticMammal otherDomesticMammal,
boolean isAngry) {
printNicker(times, otherDomesticMammal, isAngry);
}
@Override
public final void talk() {
nicker();
}
}
After we enter the previous lines, JShell will display the following messages:
| update replaced class AmericanQuarterHorse
| update replaced class ShireHorse
| update replaced class Thoroughbred
| update replaced variable american, reset to null
| update replaced variable zelda, reset to null
| update replaced variable willow, reset to null
| update overwrote class VirtualHorse
We could replace the definition for the VirtualHorse class and the subclasses were also updated. It is important to know that the variables we declared in JShell that held references to instances of subclasses of VirtualHorse were set to null.
In this article, we created many abstract and concrete classes. We learned to control whether subclasses can or cannot override members, and whether classes can be subclassed.
We worked with instances of many subclasses and we understood that objects can take many forms. We worked with many instances and their methods in JShell to understand how the classes and the methods that we coded are executed. We used methods that performed operations with instances of different classes that had a common superclass.
Further resources on this subject: