










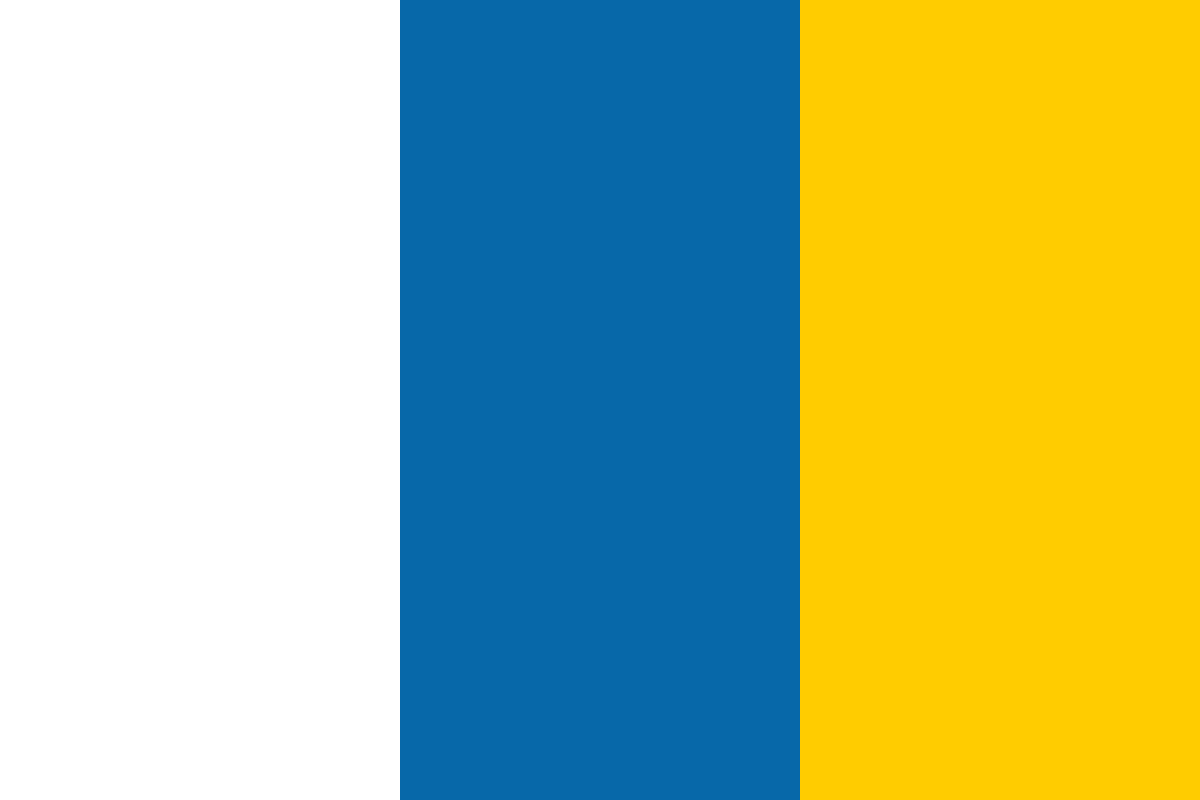

































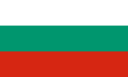








Create real time 3D applications using OGRE 3D from scratch
We will use this to create a sample quad that we can experiment with.
We will start with an empty application and insert the code for our quad into the createScene() function:
Ogre::ManualObject* manual = mSceneMgr- >createManualObject("Quad"); manual->begin("BaseWhiteNoLighting", RenderOperation::OT_TRIANGLE_ LIST);
manual->position(5.0, 0.0, 0.0);
manual->textureCoord(0,1);
manual->position(-5.0, 10.0, 0.0);
manual->textureCoord(1,0);
manual->position(-5.0, 0.0, 0.0);
manual->textureCoord(1,1);
manual->position(5.0, 10.0, 0.0);manual->textureCoord(0,0);
manual->index(0);
manual->index(1);
manual->index(2);
manual->index(0);
manual->index(3);
manual->index(1);
manual->end();
manual->convertToMesh("Quad");
Ogre::Entity * ent = mSceneMgr->createEntity("Quad");
Ogre::SceneNode* node = mSceneMgr->getRootSceneNode()-
>createChildSceneNode("Node1");
node->attachObject(ent);
We used our knowledge to create a quad and attach to it a material that simply renders everything in white. The next step is to create our own material.
Always rendering everything in white isn't exactly exciting, so let's create our first material.
Now, we are going to create our own material using the white quad we created.
manual->begin("MyMaterial1", RenderOperation::OT_TRIANGLE_LIST);
material MyMaterial1
{
technique
{
pass
{
texture_unit
{
texture leaf.png
}
}
}
}
We created our first material file. In Ogre 3D, materials can be defined in material files. To be able to find our material files, we need to put them in a directory listed in the resources.cfg, like the one we used. We also could give the path to the file directly in code using the ResourceManager.
To use our material defined in the material file, we just had to use the name during the begin call of the manual object.
The interesting part is the material file itself.
Each material starts with the keyword material, the name of the material, and then an open curly bracket. To end the material, use a closed curly bracket—this technique should be very familiar to you by now. Each material consists of one or more techniques; a technique describes a way to achieve the desired effect. Because there are a lot of different graphic cards with different capabilities, we can define several techniques and Ogre 3D goes from top to bottom and selects the first technique that is supported by the user's graphic cards. Inside a technique, we can have several passes. A pass is a single rendering of your geometry. For most of the materials we are going to create, we only need one pass. However, some more complex materials might need two or three passes, so Ogre 3D enables us to define several passes per technique. In this pass, we only define a texture unit. A texture unit defines one texture and its properties. This time the only property we define is the texture to be used. We use leaf.png as the image used for our texture. This texture comes with the SDK and is in a folder that gets indexed by resources.cfg, so we can use it without any work from our side.
Create a new material called MyMaterial2 that uses Water02.jpg as an image.
There are different strategies used when texture coordinates are outside the 0 to 1 range. Now, let's create some materials to see them in action.
We are going to use the quad from the previous example with the leaf texture material:
manual->position(5.0, 0.0, 0.0);
manual->textureCoord(0,2);
manual->position(-5.0, 10.0, 0.0);
manual->textureCoord(2,0);
manual->position(-5.0, 0.0, 0.0);
manual->textureCoord(2,2);
manual->position(5.0, 10.0, 0.0);
manual->textureCoord(0,0);
We simply changed our quad to have texture coordinates that range from zero to two. This means that Ogre 3D needs to use one of its strategies to render texture coordinates that are larger than 1. The default mode is wrap. This means each value over 1 is wrapped to be between zero and one. The following is a diagram showing this effect and how the texture coordinates are wrapped. Outside the corners, we see the original texture coordinates and inside the corners, we see the value after the wrapping. Also for better understanding, we see the four texture repetitions with their implicit texture coordinates.
We have seen how our texture gets wrapped using the default texture wrapping mode. Our plant texture shows the effect pretty well, but it doesn't show the usefulness of this technique. Let's use another texture to see the benefits of the wrapping mode.
For this example, we are going to use another texture. Otherwise, we wouldn't see the effect of this texture mode:
material MyMaterial3
{
technique
{
pass
{
texture_unit
{
texture terr_rock6.jpg
}
}
}
}
manual->begin("MyMaterial3", RenderOperation::OT_TRIANGLE_LIST)
This time, the quad seems like it's covered in one single texture. We don't see any obvious repetitions like we did with the plant texture. The reason for this is that, like we already know, the texture wrapping mode repeats. The texture was created in such a way that at the left end of the texture, the texture is started again with its right side and the same is true for the lower end. This kind of texture is called seamless. The texture we used was prepared so that the left and right side fit perfectly together. The same goes for the upper and lower part of the texture. If this wasn't the case, we would see instances where the texture is repeated.