










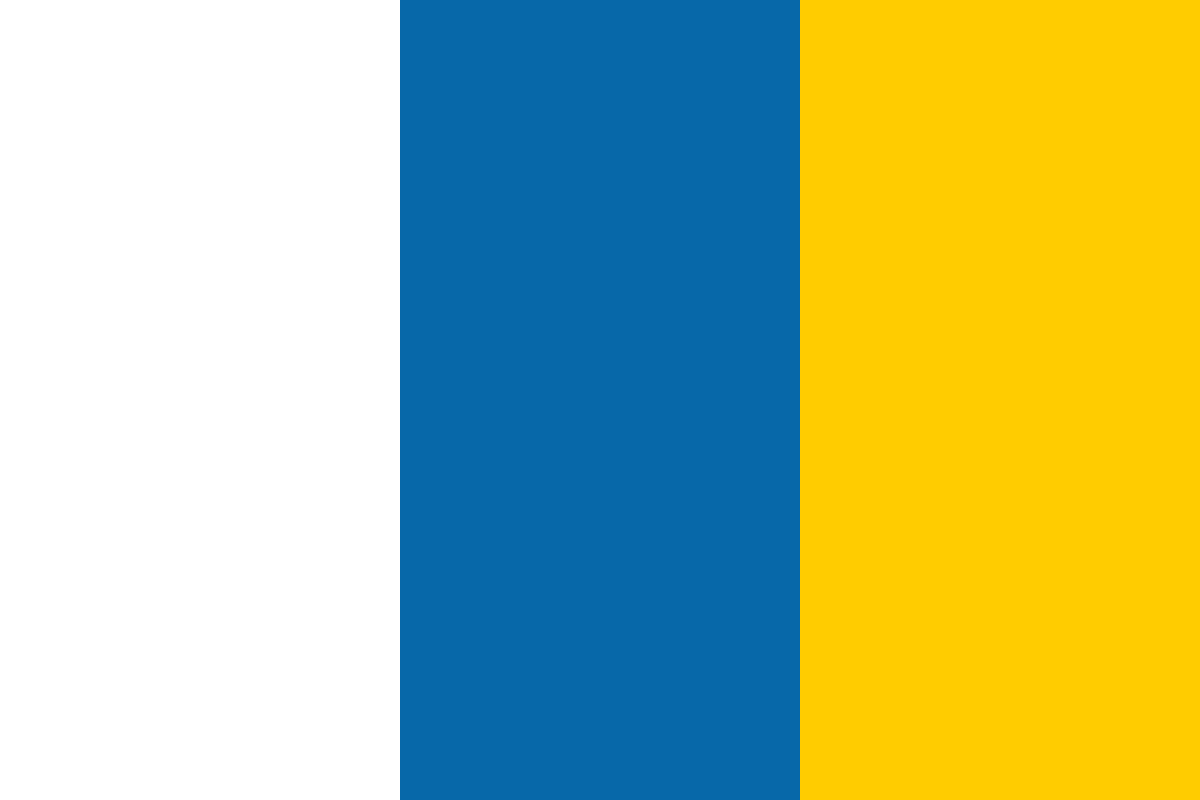

































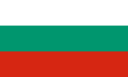








(For more resources related to this topic, see here.)
While working with location services on the Android platform, you will primarily work with an instance of LocationManager. The process is fairly straightforward as follows:
Android devices generally provide two different means for determining a location: GPS and Network. When requesting location change notifications, you must specify the provider you wish to receive updates from. The Android platform defines a set of string constants for the following providers:
Provider Name |
Description |
GPS_PROVIDER (gps) |
This provider determines a location using satellites. Depending on conditions, this provider may take a while to return a location fix. This requires the ACCESS_FINE_LOCATION permission. |
NETWORK_PROVIDER (network) |
This provider determines a location based on the availability of a cell tower and Wi-Fi access points. Its results are retrieved by means of a network lookup. |
PASSIVE_PROVIDER (passive) |
This provider can be used to passively receive location updates when other applications or services request them without actually having to request for the locations yourself. It requires the ACCESS_FINE_ LOCATION permission, although if the GPS is not enabled, this provider might only return coarse fixes. |
You will notice specific permissions in the provider descriptions that must be set on an app to be used.
App permissions are specified in the AndroidManifest.xml file. To set the appropriate permissions, perform the following steps:
To use an emulator for development, this article will require the emulator to be configured with Google APIs so that the address lookup and navigation to map app works.
To install and configure Google APIs, perform the following steps:
The LocationManager class is a system service that provides access to the location and bearing of a device, if the device supports these services. You do not explicitly create an instance of LocationManager; instead, you request an instance from a Context object using the GetSystemService() method. In most cases, the Context object is a subtype of Activity. The following code depicts declaring a reference of a LocationManager class and requesting an instance:
LocationManager _locMgr; . . . _locMgr = GetSystemService (Context.LocationService) as LocationManager;
The LocationManager class provides a series of overloaded methods that can be used to request location update notifications. If you simply need a single update, you can call RequestSingleUpdate(); to receive ongoing updates, call RequestLocationUpdate().
Prior to requesting location updates, you must identify the location provider that should be used. In our case, we simply want to use the most accurate provider available at the time. This can be accomplished by specifying the criteria for the desired provider using an instance of Android.Location.Criteria. The following code example shows how to specify the minimum criteria:
Criteria criteria = new Criteria(); criteria.Accuracy = Accuracy.NoRequirement; criteria.PowerRequirement = Power.NoRequirement;
Now that we have the criteria, we are ready to request updates as follows:
_locMgr.RequestSingleUpdate (criteria, this, null);
In this article, we stepped through integrating POIApp with location services and the Google map app. We dealt with the various options that developers have to make their apps location aware and walks the reader through adding logic to determine a device's location and the address of a location, and displaying a location within the map app.
Further resources on this subject: