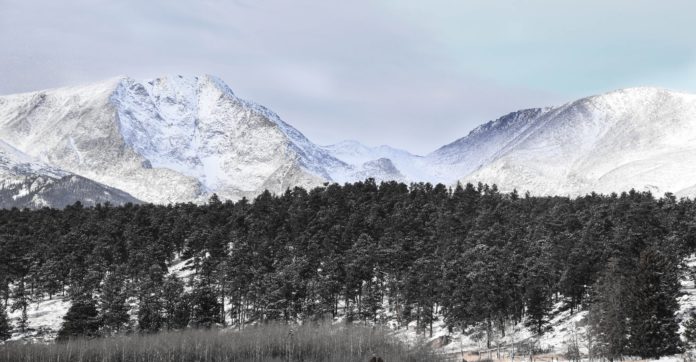
(For more resources related to this topic, see here.)
Sending a message from a website
Sending messages from a website has many uses; sending notifications to users is one good example. In this example, we’re going to present you with a form where you can enter a phone number and message and send it to your user. This can be quickly adapted for other uses.
Getting ready
The complete source code for this recipe can be found in the Chapter6/Recipe1/ folder.
How to do it…
Ok, let’s learn how to send an SMS message from a website. The user will be prompted to fill out a form that will send the SMS message to the phone number entered in the form.
- Download the Twilio Helper Library from https://github.com/twilio/twilio-php/zipball/master and unzip it.
- Upload the Services/ folder to your website.
- Upload config.php to your website and make sure the following variables are set:
<?php $accountsid = ”; // YOUR TWILIO ACCOUNT SID $authtoken = ”; // YOUR TWILIO AUTH TOKEN $fromNumber = ”; // PHONE NUMBER CALLS WILL COME FROM ?>
- Upload a file called sms.php and add the following code to it:
<!DOCTYPE html> <html> <head> <title>Recipe 1 – Chapter 6</title> </head> <body> <?php include(‘Services/Twilio.php’); include(“config.php”); include(“functions.php”); $client = new Services_Twilio($accountsid, $authtoken); if( isset($_POST[‘number’]) && isset($_POST[‘message’]) ){ $sid = send_sms($_POST[‘number’],$_POST[‘message’]); echo “Message sent to {$_POST[‘number’]}”; } ?> <form method=”post”> <input type=”text” name=”number” placeholder=”Phone Number….” /><br /> <input type=”text” name=”message” placeholder=”Message….” /><br /> <button type=”submit”>Send Message</button> </form> </body> </html>
- Create a file called functions.php and add the following code to it:
<?php function send_sms($number,$message){ global $client,$fromNumber; $sms = $client->account->sms_messages->create( $fromNumber, $number, $message ); return $sms->sid; }
How it works…
In steps 1 and 2, we downloaded and installed the Twilio Helper Library for PHP. This library is the heart of your Twilio-powered apps. In step 3, we uploaded config.php that contains our authentication information to talk to Twilio’s API.
In steps 4 and 5, we created sms.php and functions.php, which will send a message to the phone number we enter. The send_sms function is handy for initiating SMS conversations; we’ll be building on this function heavily in the rest of the article.
Allowing users to make calls from their call logs
We’re going to give your user a place to view their call log.
We will display a list of incoming calls and give them the option to call back on these numbers.
Getting ready
The complete source code for this recipe can be found in the Chapter9/Recipe4 folder in the source code for this article.
How to do it…
Now, let’s build a section for our users to log in to using the following steps:
- Update a file called index.php with the following content:
<?php session_start(); include ‘Services/Twilio.php’; require(“system/jolt.php”); require(“system/pdo.class.php”); require(“system/functions.php”); $_GET[‘route’] = isset($_GET[‘route’]) ? ‘/’.$_GET[‘route’] : ‘/’; $app = new Jolt(‘site’,false); $app->option(‘source’, ‘config.ini’); #$pdo = Db::singleton(); $mysiteURL = $app->option(‘site.url’); $app->condition(‘signed_in’, function () use ($app) { $app->redirect( $app->getBaseUri().’/login’,!$app->store(‘user’)); }); $app->get(‘/login’, function() use ($app){ $app->render( ‘login’, array(),’layout’ ); }); $app->post(‘/login’, function() use ($app){ $sql = “SELECT * FROM `user` WHERE `email`='{$_POST[‘user’]}’ AND
`password`='{$_POST[‘pass’]}'”; $pdo = Db::singleton(); $res = $pdo->query( $sql ); $user = $res->fetch(); if( isset($user[‘ID’]) ){ $_SESSION[‘uid’] = $user[‘ID’]; $app->store(‘user’,$user[‘ID’]); $app->redirect( $app->getBaseUri().’/home’); }else{ $app->redirect( $app->getBaseUri().’/login’); } }); $app->get(‘/signup’, function() use ($app){ $app->render( ‘register’, array(),’layout’ ); }); $app->post(‘/signup’, function() use ($app){ $client = new Services_Twilio($app->store(‘twilio.accountsid’),
$app->store(‘twilio.authtoken’) ); extract($_POST); $timestamp = strtotime( $timestamp ); $subaccount = $client->accounts->create(array( “FriendlyName” => $email )); $sid = $subaccount->sid; $token = $subaccount->auth_token; $sql = “INSERT INTO ‘user’ SET `name`='{$name}’,`email`='{$email}’,
`password`='{$password}’,`phone_number`='{$phone_number}’,
`sid`='{$sid}’,`token`='{$token}’,`status`=1”; $pdo = Db::singleton(); $pdo->exec($sql); $uid = $pdo->lastInsertId(); $app->store(‘user’,$uid ); // log user in $app->redirect( $app->getBaseUri().’/phone-number’); }); $app->get(‘/phone-number’, function() use ($app){ $app->condition(‘signed_in’); $user = $app->store(‘user’); $client = new Services_Twilio($user[‘sid’], $user[‘token’]); $app->render(‘phone-number’); }); $app->post(“search”, function() use ($app){ $app->condition(‘signed_in’); $user = get_user( $app->store(‘user’) ); $client = new Services_Twilio($user[‘sid’], $user[‘token’]); $SearchParams = array(); $SearchParams[‘InPostalCode’] = !empty($_POST[‘postal_code’]) ?
trim($_POST[‘postal_code’]) : ”; $SearchParams[‘NearNumber’] = !empty($_POST[‘near_number’]) ?
trim($_POST[‘near_number’]) : ”; $SearchParams[‘Contains’] = !empty($_POST[‘contains’])?
trim($_POST[‘contains’]) : ” ; try { $numbers = $client->account->available_phone_numbers->
getList(‘US’, ‘Local’, $SearchParams); if(empty($numbers)) { $err = urlencode(“We didn’t find any phone numbers by that search”); $app->redirect( $app->getBaseUri().’/phone-number?msg=’.$err); exit(0); } } catch (Exception $e) { $err = urlencode(“Error processing search: {$e->getMessage()}”); $app->redirect( $app->getBaseUri().’/phone-number?msg=’.$err); exit(0); } $app->render(‘search’,array(‘numbers’=>$numbers)); }); $app->post(“buy”, function() use ($app){ $app->condition(‘signed_in’); $user = get_user( $app->store(‘user’) ); $client = new Services_Twilio($user[‘sid’], $user[‘token’]); $PhoneNumber = $_POST[‘PhoneNumber’]; try { $number = $client->account->incoming_phone_numbers->create(array( ‘PhoneNumber’ => $PhoneNumber )); $phsid = $number->sid; if ( !empty($phsid) ){ $sql = “INSERT INTO numbers (user_id,number,sid) VALUES
(‘{$user[‘ID’]}’,'{$PhoneNumber}’,'{$phsid}’);”; $pdo = Db::singleton(); $pdo->exec($sql); $fid = $pdo->lastInsertId(); $ret = editNumber($phsid,array( “FriendlyName”=>$PhoneNumber, “VoiceUrl” => $mysiteURL.”/voice?id=”.$fid, “VoiceMethod” => “POST”, ),$user[‘sid’], $user[‘token’]); } } catch (Exception $e) { $err = urlencode(“Error purchasing number: {$e->getMessage()}”); $app->redirect( $app->getBaseUri().’/phone-number?msg=’.$err); exit(0); } $msg = urlencode(“Thank you for purchasing $PhoneNumber”); header(“Location: index.php?msg=$msg”); $app->redirect( $app->getBaseUri().’/home?msg=’.$msg); exit(0); }); $app->route(‘/voice’, function() use ($app){ }); $app->get(‘/transcribe’, function() use ($app){ }); $app->get(‘/logout’, function() use ($app){ $app->store(‘user’,0); $app->redirect( $app->getBaseUri().’/login’); }); $app->get(‘/home’, function() use ($app){ $app->condition(‘signed_in’); $uid = $app->store(‘user’); $user = get_user( $uid ); $client = new Services_Twilio($user[‘sid’], $user[‘token’]); $app->render(‘dashboard’,array( ‘user’=>$user, ‘client’=>$client )); }); $app->get(‘/delete’, function() use ($app){ $app->condition(‘signed_in’); }); $app->get(‘/’, function() use ($app){ $app->render( ‘home’ ); }); $app->listen(); - Upload a file called dashboard.php with the following content to your views folder:
<h2>My Number</h2> <?php $pdo = Db::singleton(); $sql = “SELECT * FROM `numbers` WHERE `user_id`='{$user[‘ID’]}'”; $res = $pdo->query( $sql ); while( $row = $res->fetch() ){ echo preg_replace(“/[^0-9]/”, “”, $row[‘number’]); } try { ?> <h2>My Call History</h2> <p>Here are a list of recent calls, you can click any number to call
them back, we will call your registered phone number
and then the caller</p> <table width=100% class=”table table-hover tabled-striped”> <thead> <tr> <th>From</th> <th>To</th> <th>Start Date</th> <th>End Date</th> <th>Duration</th> </tr> </thead> <tbody> <?php foreach ($client->account->calls as $call) { # echo “<p>Call from $call->from to $call->to at
$call->start_time of length $call->duration</p>”; if( !stristr($call->direction,’inbound’) ) continue; $type = find_in_list($call->from); ?> <tr> <td><a href=”<?=$uri?>/call?number=
<?=urlencode($call->from)?>”><?=$call->from?></a></td> <td><?=$call->to?></td> <td><?=$call->start_time?></td> <td><?=$call->end_time?></td> <td><?=$call->duration?></td> </tr> <?php } ?> </tbody> </table> <?php } catch (Exception $e) { echo ‘Error: ‘ . $e->getMessage(); } ?> <hr /> <a href=”<?=$uri?>/delete” onclick=”return confirm
(‘Are you sure you wish to close your account?’);”>Delete My Account</a>
How it works…
In step 1, we updated the index.php file.
In step 2, we uploaded dashboard.php to the views folder. This file checks if we’re logged in using the $app->condition(‘signed_in’) method, which we discussed earlier, and if we are, it displays all incoming calls we’ve had to our account. We can then push a button to call one of those numbers and whitelist or blacklist them.
We also give the user the option to delete the account, which we’ll cover in the Deleting a subaccount recipe.
Summary
Thus in this article we have learned how to send messages and make phone calls from your website using Twilio.
Resources for Article:
Further resources on this subject:
- Trunks in FreePBX 2.5 [Article]
- Recording Calls in FreePBX 2.5 [Article]
- Voice Menus and IVR in AsteriskNOW [Article]