










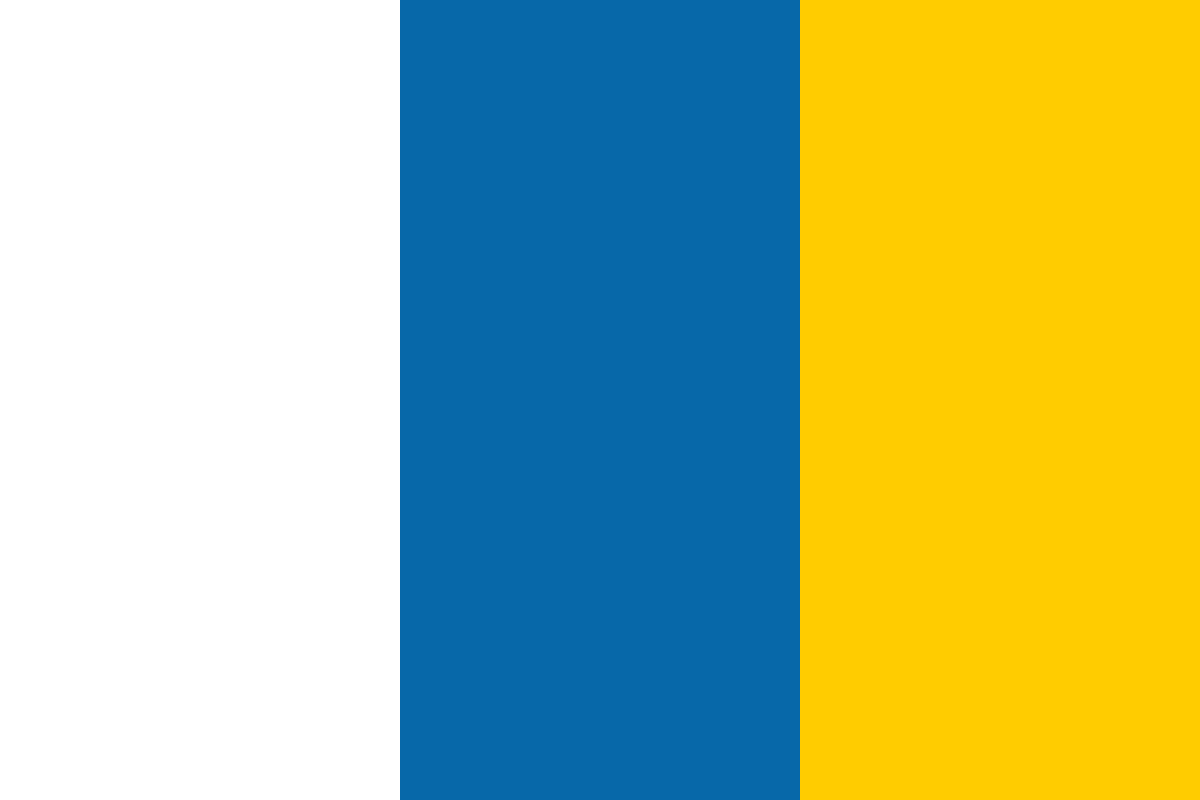

































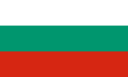








Ext JS has an extensive library of validation functions. This is how it can be used to validate URLs, email addresses, and other types of data.
The following screenshot shows email address validation in action:
This screenshot displays URL validation in action:
Ext.QuickTips.init();
Ext.onReady(function() {
var commentForm = new Ext.FormPanel({
frame: true,
title: 'Send your comments',
bodyStyle: 'padding:5px',
width: 550,
layout: 'form',
defaults: { msgTarget: 'side' },
items: [
{ xtype: 'textfield',
fieldLabel: 'Name',
name: 'name',
anchor: '95%',
allowBlank: false
},
{
xtype: 'textfield',
fieldLabel: 'Email',
name: 'email',
anchor: '95%',
vtype: 'email'
},
{
xtype: 'textfield',
fieldLabel: 'Web page',
name: 'webPage',
vtype: 'url',
anchor: '95%'
},
{
xtype: 'textarea',
fieldLabel: 'Comments',
name: 'comments',
anchor: '95%',
height: 150,
allowBlank: false
}],
buttons: [{
text: 'Send'
},
{
text: 'Cancel'
}]
});
commentForm.render(document.body);
});
The vtype configuration option specifies which validation function will be applied to the field.
Validation types in Ext JS include alphanumeric, numeric, URL, and email formats. You can extend this feature with custom validation functions, and virtually, any format can be validated. For example, the following code shows how you can add a validation type for JPG and PNG files:
Ext.apply(Ext.form.VTypes, {
Picture: function(v) {
return /^.*.(jpg|JPG|png|PNG)$/.test(v);
},
PictureText: 'Must be a JPG or PNG file';
});
If you need to replace the default error text provided by the validation type, you can do so by using the vtypeText configuration option:
{
xtype: 'textfield',
fieldLabel: 'Web page',
name: 'webPage',
vtype: 'url',
vtypeText: 'I am afraid that you did not enter a URL',
anchor: '95%'
}
Frequently, you face scenarios where the values of two fields need to match, or the value of one field depends on the value of another field.
Let's examine how to build a registration form that requires the user to confirm his or her password when signing up.
Ext.QuickTips.init();
Ext.apply(Ext.form.VTypes, {
password: function(val, field) {
if (field.initialPassField) {
var pwd = Ext.getCmp(field.initialPassField);
return (val == pwd.getValue());
}
return true;
},
passwordText: 'What are you doing?<br/>The passwords entered
do not match!'
});
var signupForm = { xtype: 'form',
id: 'register-form',
labelWidth: 125,
bodyStyle: 'padding:15px;background:transparent',
border: false,
url: 'signup.php',
items: [
{ xtype: 'box',
autoEl: { tag: 'div',
html: '<div class="app-msg"><img src="img/businessman
add.png" class="app-img" />
Register for The Magic Forum</div>'
}
},
{ xtype: 'textfield', id: 'email', fieldLabel: 'Email',
allowBlank: false, minLength: 3,
maxLength: 64,anchor:'90%', vtype:'email'
},
{ xtype: 'textfield', id: 'pwd', fieldLabel: 'Password',
inputType: 'password',allowBlank: false, minLength: 6,
maxLength: 32,anchor:'90%',
minLengthText: 'Password must be at least 6 characters
long.'
},
{ xtype: 'textfield', id: 'pwd-confirm',
fieldLabel: 'Confirm Password', inputType: 'password',
allowBlank: false, minLength: 6,
maxLength: 32,anchor:'90%',
minLengthText: 'Password must be at least 6 characters
long.',
vtype: 'password', initialPassField: 'pwd'
}
],
buttons: [{
text: 'Register',
handler: function() {
Ext.getCmp('register-form').getForm().submit();
}
},
{
text: 'Cancel',
handler: function() {
win.hide();
}
}]
}
Ext.onReady(function() {
win = new Ext.Window({
layout: 'form',
width: 340,
autoHeight: true,
closeAction: 'hide',
items: [signupForm]
});
win.show();