










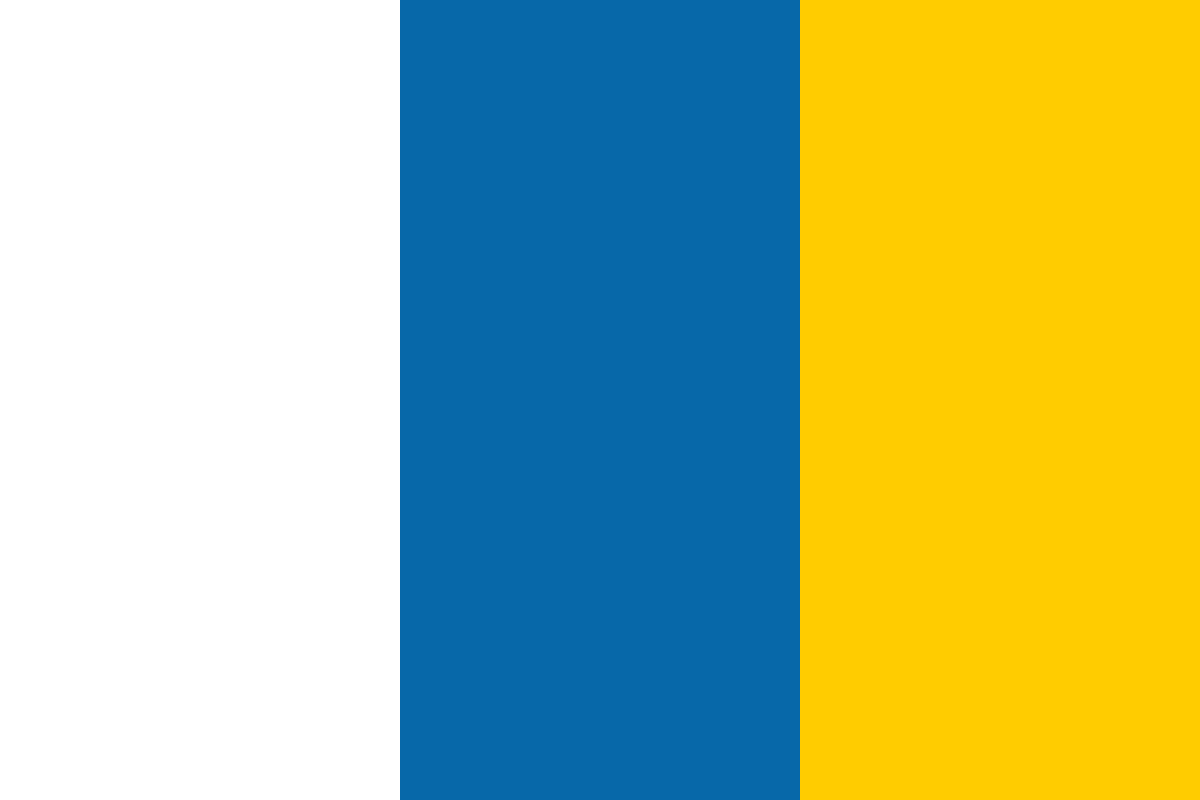

































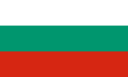








(For more resources related to this topic, see here.)
We will create our application, which will be called awesome-chat, by executing the following commands in the Node.js command line:
$ express awesome-chat $ cd awesome-chat $ npm install
This will create our application and install the express application dependencies. Open the package.json file and change the name to awesome-chat, as shown in the following code snippet:
{ "name": "awesome-chat", "version": "0.0.1", "private": true, "scripts": { "start": "node app" }, "dependencies": { "express": "3.0.0rc2express": "3.x", "jade": "*" } }
Let's modify the view to make it look like a chat room. We will need an area to display the messages, a text input for the user to enter the message, and a button to send the message. We will add some aesthetic elements, such as a header, banner, and footer. When we are done, our chat room user interface should look like the one shown in the following screenshot:
Awesome chat UI
Let's start editing layout.jade by adding a header and footer to it:
doctype 5 html block head title= title link(rel='stylesheet', href='/stylesheets/style.css') body header#banner h1 Awesome Chat block content footer Hope you enjoy your stay here
The first change we make is to add the block keyword before head. This makes head a block, to which we can append content from the extending pages.
The other change is the addition of a new header and footer. Note that we are using the header and footer tags from HTML5. This code also introduces us to a new jade syntax. When we write header#banner , it will generate headers with banner as the id value. The generated HTML code will be as follows:
<!DOCTYPE html> <html> <head> <title>{TITLE}</title> <link rel="stylesheet" href="/stylesheets/style.css" /> </head> <body> <header id="banner"> <h1>Awesome Chat</h1> </header> {CONTENT} <footer> Hope you enjoy your stay here </footer> </body> </html>
Next, we will edit index.jade to add the message area, message input, and the Send button:
extends layout block content section#chatroom div#messages input#message(type='text', placeholder='Enter your message here') input#send(type='button', value='Send')
Let's run and see what our awesome-chat application looks like. Execute the application using npm and open http://localhost:3000/ in the browser:
npm start
Hey, all the elements are there, but it doesn't look right! That's correct; to improve the look and feel of the application, we need to edit the stylesheet, which is located at public/stylesheets/style.css.
We can edit it according to our taste. Here is one that works just fine for me:
html { height: 100%; } body { font: 14px "Lucida Grande", Helvetica, Arial, sans-serif; margin: 0px; padding: 0px; height: -moz-calc(100% - 20px); height: -webkit-calc(100% - 20px); height: calc(100% - 20px); } section#chatroom { height: -moz-calc(100% - 80px); height: -webkit-calc(100% - 80px); height: calc(100% - 80px); background-color: #EFFFEC; } div#messages { height: -moz-calc(100% - 35px); height: -webkit-calc(100% - 35px); height: calc(100% - 35px); padding: 10px; -moz-box-sizing:border-box; -webkit-box-sizing:border-box; box-sizing:border-box; } input#message { width: -moz-calc(100% - 80px); width: -webkit-calc(100% - 80px); width: calc(100% - 80px); } input#send { width: 74px; } header{ background-color:#4192C1; text-align: right; margin-top: 15px; } header h1{ padding: 5px; padding-right: 15px; color: #FFFFFF; margin: 0px; } footer{ padding: 6px; background-color:#4192C1; color: #FFFFFF; bottom: 0; position: absolute; width: 100%; margin: 0px; margin-bottom: 10px; -moz-box-sizing:border-box; -webkit-box-sizing:border-box; box-sizing:border-box; } a { color: #00B7FF; }
After saving this CSS and refreshing the page, here is what the chat room looks like:
The awesome chat room
jQuery is almost ubiquitous when it comes to JavaScript libraries, and we will use it in our application too. To add jQuery to our application, let's download the latest release from http://www.jquery.com/ and save it to public/javascript/jquery.min.js . Then, we add the script in layout.jade to pull in jQuery to our application's pages:
script(type='text/javascript', src = '/javascripts/jquery.min.js')
Ever since the onset of web applications, developers have worked towards different ways of getting duplex communication between the server and the browser. Be it using Java, Flash, Comet, or many other workarounds, all aim to do the same. But for the first time, there is a specification to build a full-duplex communication system by using HTML5 WebSockets. WebSocket is a revolutionary, new communication feature in the HTML5 specification that defines a full-duplex communication channel operating over the Web through a single socket.
Although the WebSockets RFC is published, it is not, and will never be, available on older browsers that are still in use. Socket.io is an abstraction layer for WebSockets, with Flash, XHR, JSONP, and HTMLFile fallbacks. Socket.io provides an easy server and client library for making real-time, streaming updates between a web server and a browser client.
Socket.io is a node module available through the npm, and we will add it to our package dependencies. The current release of socket.io is 0.9.10. To add this to our dependencies, add the following line to the dependencies object in package.json:
"socket.io": "0.9.10"
And install it using the npm:
$ npm install
This will bring socket.io in the node_modules folder. Now let's see how we will use it.
Since the socket.io framework has components for both the server and the client, we will use these components to code our communication on both the sides. Events emitted on a socket on one side will be handled by the corresponding event handler on the other side. Socket.io is built so that both the sides can send messages or attach handlers to process the incoming messages.
Let's begin by understanding how the messages will flow. It is important to remember that "messages" here are not the actual messages sent and received by users of the chat system, but the messages used for communication by the client and the server. There will be two types of messages, as follows:
The system messages: These messages will be sent by our chat system to the client, like when the user is connected, when others connect, or when users disconnect. Let's identify it with serverMessage.
The user messages: These messages will be sent by the client to the server and will actually carry the user's message content in the payload. We will probably want to differentiate between the messages we send and the messages other users send. So let's call them myMessage and userMessage respectively.
When a user connects for the first time, the server will send a welcome message to the user as a serverMessage message.
When a user types in a message and presses the Send button, we will send a userMessage message from the browser to the server.
On receiving the user message, the server will broadcast this message to all the other users. It will also send back the same message as myMessage to the user who originally sent the message.
On receiving any message from the server, the browser will display the contents of the message in the message area.