










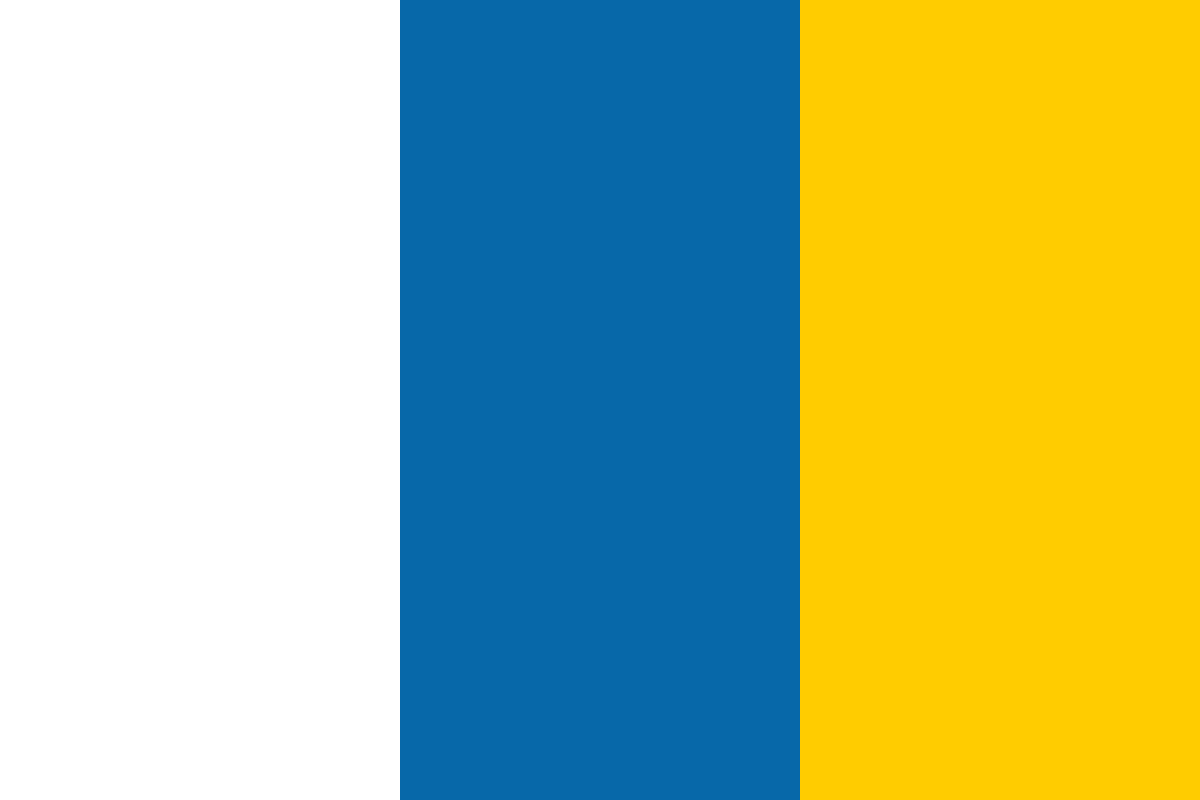

































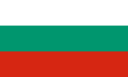








In this article by Bass Jobsen, author of the book Less Web Development Essentials - Second Edition, we will cover the following topics:
(For more resources related to this topic, see here.)
Nowadays, WordPress is not only used for weblogs, but it can also be used as a content management system for building a website.
The WordPress system, written in PHP, has been split into the core system, plugins, and themes. The plugins add additional functionalities to the system, and the themes handle the look and feel of a website built with WordPress. They work independently of each other and are also independent of the theme. The theme does not depend on plugins. WordPress themes define the global CSS for a website, but every plugin can also add its own CSS code.
The WordPress theme developers can use Less to compile the CSS code of the themes and the plugins.
Sage is a WordPress starter theme. You can use it to build your own theme. The theme is based on HTML5 Boilerplate (http://html5boilerplate.com/) and Bootstrap. Visit the Sage theme website at https://roots.io/sage/. Sage can also be completely built using Gulp.
More information about how to use Gulp and Bower for the WordPress development can be found at https://roots.io/sage/docs/theme-development/.
After downloading Sage, the Less files can be found at assets/styles/. These files include Bootstrap's Less files. The assets/styles/main.less file imports the main Bootstrap Less file, bootstrap.less.
Now, you can edit main.less to customize your theme. You will have to rebuild the Sage theme after the changes you make. You can use all of the Bootstrap's variables to customize your build.
JBST is also a WordPress starter theme. JBST is intended to be used with the so-called child themes. More information about the WordPress child themes can be found at https://codex.wordpress.org/Child_Themes.
After installing JBST, you will find a Less compiler under Appearance in your Dashboard pane, as shown in the following screenshot:
JBST's built-in Less compiler in the WordPress Dashboard
The built-in Less compiler can be used to fully customize your website using Less. Bootstrap also forms the skeleton of JBST, and the default settings are gathered by the a11y bootstrap theme mentioned earlier.
JBST's Less compiler can be used in the following different ways:
h1 {color: red;}
@navbar-default-bg: blue; .btn-colored { .button-variant(blue;red;green); }
@footer_bg_color: black;
.include-custom-font(@family: arial,@font-path, @path: @custom-font-dir, @weight: normal, @style: normal);
In the preceding code, the parameters mentioned were used to set the font name (@family) and the path name to the font files (@path/@font-path). The @weight and @style parameters set the font's properties. For more information, visit https://github.com/bassjobsen/Boilerplate-JBST-Child-Theme.
More Less code blocks can also be added to a special file (wpless2css/wpless2css.less or less/custom.less); these files will give you the option to add, for example, a library of prebuilt mixins. After adding the library using this file, the mixins can also be used with the built-in compiler.
The Semantic UI, as discussed earlier, offers its own WordPress plugin. The plugin can be downloaded from https://github.com/ProjectCleverWeb/Semantic-UI-WordPress. After installing and activating this theme, you can use your website directly with the Semantic UI. With the default setting, your website will look like the following screenshot:
Website built with the Semantic UI WordPress theme
As discussed earlier, the WordPress plugins have their own CSS. This CSS will be added to the page like a normal style sheet, as shown here:
<link rel='stylesheet' id='plugin-name' href='//domain/wp-content/plugin-name/plugin-name.css?ver=2.1.2' type='text/css' media='all' />
Unless a plugin provides the Less files for their CSS code, it will not be easy to manage its styles with Less.
The WP Less to CSS plugin, which can be found at http://wordpress.org/plugins/wp-less-to-css/, offers the possibility of styling your WordPress website with Less. As seen earlier, you can enter the Less code along with the built-in compiler of JBST. This code will then be compiled into the website's CSS. This plugin compiles Less with the PHP Less compiler, Less.php.
The Play framework helps you in building lightweight and scalable web applications by using Java or Scala. It will be interesting to learn how to integrate Less with the workflow of the Play framework. You can install the Play framework from https://www.playframework.com/. To learn more about the Play framework, you can also read, Learning Play! Framework 2, Andy Petrella, Packt Publishing.
To read Petrella's book, visit https://www.packtpub.com/web-development/learning-play-framework-2.
To run the Play framework, you need JDK 6 or later. The easiest way to install the Play framework is by using the Typesafe activator tool. After installing the activator tool, you can run the following command:
> activator new my-first-app play-scala
The preceding command will install a new app in the my-first-app directory. Using the play-java option instead of the play-scala option in the preceding command will lead to the installation of a Java-based app. Later on, you can add the Scala code in a Java app or the Java code in a Scala app.
After installing a new app with the activator command, you can run it by using the following commands:
cd my-first-app activator run
Now, you can find your app at http://localhost:9000.
To enable the Less compilation, you should simply add the sbt-less plugin to your plugins.sbt file as follows:
addSbtPlugin("com.typesafe.sbt" % "sbt-less" % "1.0.6")
After enabling the plugin, you can edit the build.sbt file so as to configure Less. You should save the Less files into app/assets/stylesheets/. Note that each file in app/assets/stylesheets/ will compile into a separate CSS file.
The CSS files will be saved in public/stylesheets/ and should be called in your templates with the HTML code shown here:
<link rel="stylesheet" href="@routes.Assets.at("stylesheets/main.css")">
In case you are using a library with more files imported into the main file, you can define the filters in the build.sbt file. The filters for these so-called partial source files can look like the following code:
includeFilter in (Assets, LessKeys.less) := "*.less" excludeFilter in (Assets, LessKeys.less) := "_*.less"
The preceding filters ensure that the files starting with an underscore are not compiled into CSS.
Bootstrap is a CSS framework. Bootstrap's Less code includes many files. Keeping your code up-to-date by using partials, as described in the preceding section, will not work well. Alternatively, you can use WebJars with Play for this purpose. To enable the Bootstrap WebJar, you should add the code shown here to your build.sbt file:
libraryDependencies += "org.webjars" % "bootstrap" % "3.3.2"
When using the Bootstrap WebJar, you can import Bootstrap into your project as follows:
@import "lib/bootstrap/less/bootstrap.less";
AngularJS is a structural framework for dynamic web apps. It extends the HTML syntax, and this enables you to create dynamic web views. Of course, you can use AngularJS with Less. You can read more about AngularJS at https://angularjs.org/.
The HTML code shown here will give you an example of what repeating the HTML elements with AngularJS will look like:
<!doctype html> <html ng-app> <head> <title>My Angular App</title> </head> <body ng-app> <ul> <li ng-repeat="item in [1,2,3]">{{ item }}</li> </ul> <script src="https://ajax.googleapis.com/ajax/libs/angularjs/1.3.12/& angular.min.js"></script> </body> </html>
This code should make your page look like the following screenshot:
Repeating the HTML elements with AngularJS
The ngBoilerplate system is an easy way to start a project with AngularJS. The project comes with a directory structure for your application and a Grunt build process, including a Less task and other useful libraries.
To start your project, you should simply run the following commands on your console:
> git clone git://github.com/ngbp/ngbp > cd ngbp > sudo npm -g install grunt-cli karma bower > npm install > bower install > grunt watch
And then, open ///path/to/ngbp/build/index.html in your browser.
After installing ngBoilerplate, you can write the Less code into src/less/main.less. By default, only src/less/main.less will be compiled into CSS; other libraries and other codes should be imported into this file.
Meteor is a complete open-source platform for building web and mobile apps in pure JavaScript. Meteor focuses on fast development. You can publish your apps for free on Meteor's servers.
Meteor is available for Linux and OS X. You can also install it on Windows.
Installing Meteor is as simple as running the following command on your console:
> curl https://install.meteor.com | /bin/sh
You should install the Less package for compiling the CSS code of the app with Less. You can install the Less package by running the command shown here:
> meteor add less
Note that the Less package compiles every file with the .less extension into CSS. For each file with the .less extension, a separate CSS file is created. When you use the partial Less files that should only be imported (with the @import directive) and not compiled into the CSS code itself, you should give these partials the .import.less extension.
When using the CSS frameworks or libraries with many partials, renaming the files by adding the .import.less extension will hinder you in updating your code. Also running postprocess tasks for the CSS code is not always possible.
Many packages for Meteor are available at https://atmospherejs.com/. Some of these packages can help you solve the issue with using partials mentioned earlier. To use Bootstrap, you can use the meteor-bootstrap package. The meteor-bootstrap package can be found at https://github.com/Nemo64/meteor-bootstrap. The meteor-bootstrap package requires the installation of the Less package. Other packages provide you postprocsess tasks, such as autoprefixing your code.
Ruby on Rails, or Rails, for short is a web application development framework written in the Ruby language.
Those who want to start developing with Ruby on Rails can read the Getting Started with Rails guide, which can be found at http://guides.rubyonrails.org/getting_started.html.
In this section, you can read how to integrate Less into a Ruby on Rails app.
After installing the tools and components required for starting with Rails, you can launch a new application by running the following command on your console:
> rails new blog
Now, you should integrate Less with Rails. You can use less-rails (https://github.com/metaskills/less-rails) to bring Less to Rails. Open the Gemfile file, comment on the sass-rails gem, and add the less-rails gem, as shown here:
#gem 'sass-rails', '~> 5.0' gem 'less-rails' # Less gem 'therubyracer' # Ruby
Then, create a controller called welcome with an action called index by running the following command:
> bin/rails generate controller welcome index
The preceding command will generate app/views/welcome/index.html.erb. Open app/views/welcome/index.html.erb and make sure that it contains the HTML code as shown here:
<h1>Welcome#index</h1> <p>Find me in app/views/welcome/index.html.erb</p>
The next step is to create a file, app/assets/stylesheets/welcome.css.less, with the Less code. The Less code in app/assets/stylesheets/welcome.css.less looks as follows:
@color: red; h1 { color: @color; }
Now, start a web server with the following command:
> bin/rails server
Finally, you can visit the application at http://localhost:3000/. The application should look like the example shown here:
The Rails app
In this article, you learned how to use Less WordPress, Play, Meteor, AngularJS, Ruby on Rails.