










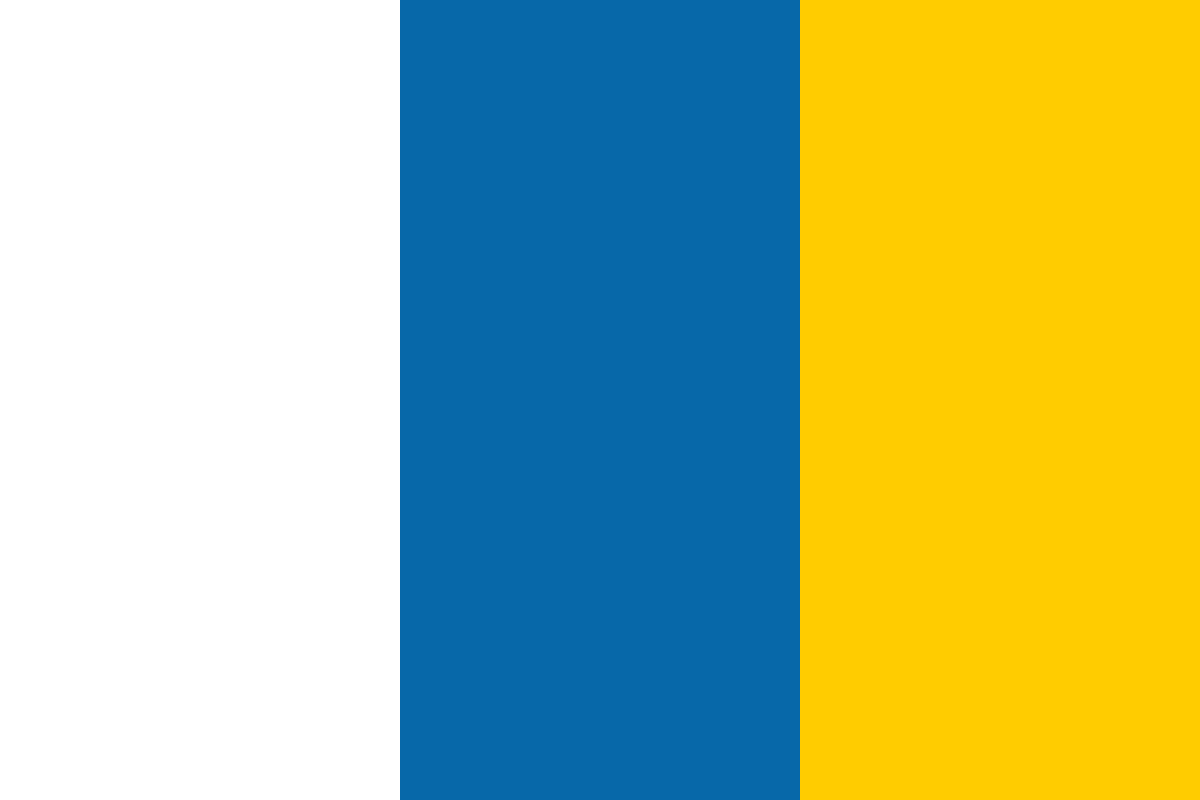

































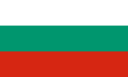








The following screenshot shows a dialog widget and the different elements that it is made of:
A dialog has a lot of default behavior built-in, but few methods are needed to control it programmatically, making this a very easy widget to use that is also highly configurable.
Generating it on the page is very simple and requires a minimal underlying mark-up structure. The following page contains the minimum mark-up that's required to implement the dialog widget:
<!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN" "http://www.w3.org/TR/html4/strict.dtd">
<html lang="en">
<head>
<link rel="stylesheet" type="text/css" href="jqueryui1.6rc2/themes/flora/flora.dialog.css">
<link rel="stylesheet" type="text/css" href="jqueryui1.6rc2/themes/flora/flora.resizable.css">
<meta http-equiv="Content-Type" content="text/html; charset=utf-8">
<title>jQuery UI Dialog Example 1</title>
</head>
<body>
<div id="myDialog" class="flora" title="This is the title">Lorem
ipsum dolor sit amet, consectetuer adipiscing elit. Aenean
sollicitudin. Sed interdum pulvinar justo. Nam iaculis volutpat
ligula. Integer vitae felis quis diam laoreet ullamcorper. Etiam
tincidunt est vitae est. Ut posuere, mauris at sodales rutrum,
turpis tellus fermentum metus, ut bibendum velit enim eu lectus.
Suspendisse potenti. Donec at dolor ac metus pharetra aliquam.
Suspendisse purus. Fusce tempor ultrices libero. Sed quis nunc.
Pellentesque tincidunt viverra felis. Integer elit mauris,
egestas ultricies, gravida vitae, feugiat a, tellus.</div>
<script type="text/javascript" src="jqueryui1.6rc2/jquery-1.2.6.js"></script>
<script type="text/javascript" src="jqueryui1.6rc2/ui/ui.core.js"></script>
<script type="text/javascript" src="jqueryui1.6rc2/ui/ui.dialog.js"></script>
<script type="text/javascript" src="jqueryui1.6rc2/ui/ui.resizable.js"></script>
<script type="text/javascript" src="jqueryui1.6rc2/ui/ui.draggable.js"></script>
<script type="text/javascript">
//define function to be executed on document ready
$(function(){
//create the dialog
$("#myDialog").dialog();
});
</script>
</body>
</html>
An options object can be used in a dialog's constructor method to configure various dialog properties. Let's look at the available properties:
Save this as dialog1.html in the jqueryui folder. A few more source files are required, specifically the ui.resizable.js and ui.draggable.js files and the flora.resizable.css stylesheet.
The JavaScript files are low-level interaction helpers and are only required if the dialog is going to be resizable and draggable. The widget will still function without them. The dialog flora theme file is a mandatory requirement for this component, although the resizable one isn't.
Other than that, the widget is initialized in the same way as other widgets. When you run this page in your browser, you should see the default dialog widget shown in the previous screenshot, complete with draggable and resizable behaviors.
One more feature that I think deserves mentioning here is modality. The dialog comes with modality built-in, although it is disabled by default. When modality is enabled, a modal overlay element, which covers the underlying page, will be applied. The dialog will sit above the overlay while the rest of the page will be below it.
The benefit of this feature is that it ensures the dialog is closed before the underlying page becomes interactive again, and gives a clear visual indicator that the dialog must be closed before the visitor can proceed.
The dialog's appearance is easy to change from the flora theme used in the first example. Like some of the other widgets, certain aspects of the default or flora themes are required to make the widget function correctly. Therefore, when overriding styles, we need to be careful to just override the rules related to the dialog's display.
When creating a new skin for the default implementation, including resizable behavior, we have a lot of new files that will need to be created. Apart from new images for the different components of the dialog, we also have to create new images for the resizing handles. The following files need to be replaced when skinning a dialog:
To make it easier to remember which image corresponds to which part of the dialog, these images are named after the compass points at which they appear. The following image illustrates this:
Note that these are file names as opposed to class names. The class names given to each of the different elements that make up the dialog, including resizable elements, are similar, but are prefixed with ui- as we'll see in the next example code.
Let's replace these images with some of our own. In a new file in your text editor, create the following stylesheet:
.flora .ui-dialog, .flora.ui-dialog {
background-color:#99ccff;
}
.flora .ui-dialog .ui-dialog-titlebar, .flora.ui-dialog
.ui-dialog-titlebar {
background:url(../img/dialog/my-title.gif) repeat-x;
background-color:#003399;
}
.flora .ui-dialog .ui-dialog-titlebar-close, .flora.ui-dialog
.ui-dialog-titlebar-close {
background:url(../img/dialog/my-title-close.gif) no-repeat; }
.flora .ui-dialog .ui-dialog-titlebar-close-hover, .flora.ui-dialog
.ui-dialog-titlebar-close-hover {
background:url(../img/dialog/my-title-close-hover.gif) norepeat;
}
.flora .ui-dialog .ui-resizable-n, .flora.ui-dialog .ui-resizable-n {
background:url(../img/dialog/my-n.gif) repeat center top;
}
.flora .ui-dialog .ui-resizable-s, .flora.ui-dialog .ui-resizable-s {
background:url(../img/dialog/my-s.gif) repeat center top;
}
.flora .ui-dialog .ui-resizable-e, .flora.ui-dialog .ui-resizable-e {
background:url(../img/dialog/my-e.gif) repeat right center; }
.flora .ui-dialog .ui-resizable-w, .flora.ui-dialog .ui-resizable-w {
background:url(../img/dialog/my-w.gif) repeat left center;
}
.flora .ui-dialog .ui-resizable-ne, .flora.ui-dialog .ui-resizable-ne
{
background:url(../img/dialog/my-ne.gif) repeat;
}
.flora .ui-dialog .ui-resizable-se, .flora.ui-dialog .ui-resizable-se
{
background:url(../img/dialog/my-se.gif) repeat;
}
.flora .ui-dialog .ui-resizable-sw, .flora.ui-dialog .ui-resizable-sw
{
background:url(../img/dialog/my-sw.gif) repeat;
}
.flora .ui-dialog .ui-resizable-nw, .flora.ui-dialog .ui-resizable-nw
{
background:url(../img/dialog/my-nw.gif) repeat;
}
Save this as dialogTheme.css in the styles folder. We should also create a new folder within our img folder called dialog. This folder will be used to store all of our dialog-specific images.
All we need to do is specify new images to replace the existing ones used by flora. All other rules can stay the same. In dialog1.html, link to the new file with the following code, which should appear directly after the link to the resizable stylesheet:
<link rel="stylesheet" type="text/css" href="styles/dialogTheme.css">
Save the change as dialog2.html. These changes will result in a dialog that should appear similar to the following screenshot:
So you can see that skinning the dialog to make it fit in with your existing content is very easy. The existing image files used by the default theme give you something to start with, and it's really just a case of playing around with colors in an image editor until you get the desired effect.
An options object can be used in a dialog's constructor method to configure various dialog properties. Let's look at the available properties:
Property |
Default Value |
Usage |
autoOpen |
true |
Shows the dialog as soon as the dialog method is called |
bgiframe |
true |
Creates an <iframe> shim to prevent <select> elements showing through the dialog in IE6 - at present, the bgiframe plugin is required, although this may not be the case in future versions of this widget |
buttons
|
{} |
Supplies an object containing buttons to be used with the dialog |
dialogClass |
ui-dialog |
Sets additional class names on the dialog for theming purposes |
draggable |
true |
Makes the dialog draggable (use ui.draggable.js) |
height |
200(px) |
Sets the starting height of the dialog |
hide |
none |
Sets an effect to be used when the dialog is closed |
maxHeight |
none |
Sets a maximum height for the dialog |
maxWidth |
none |
Sets a maximum width for the dialog |
minHeight |
100(px) |
Sets a minimum height for the dialog |
minWidth |
150(px) |
Sets a minimum width for the dialog |
modal |
false |
Enables modality while the dialog is open |
overlay |
{} |
Object with CSS properties for the modal overlay |
position |
center |
Sets the starting position of the dialog in the viewport |
resizable |
true |
Makes the dialog resizable (also requires ui.resizable.js) |
show |
none |
Sets an effect to be used when the dialog is opened |
stack |
true |
Causes the focused dialog to move to the front when several dialogs are open |
title |
none |
Alternative to specifying title on source element |
width |
300(px) |
Sets the original width of the dialog |