










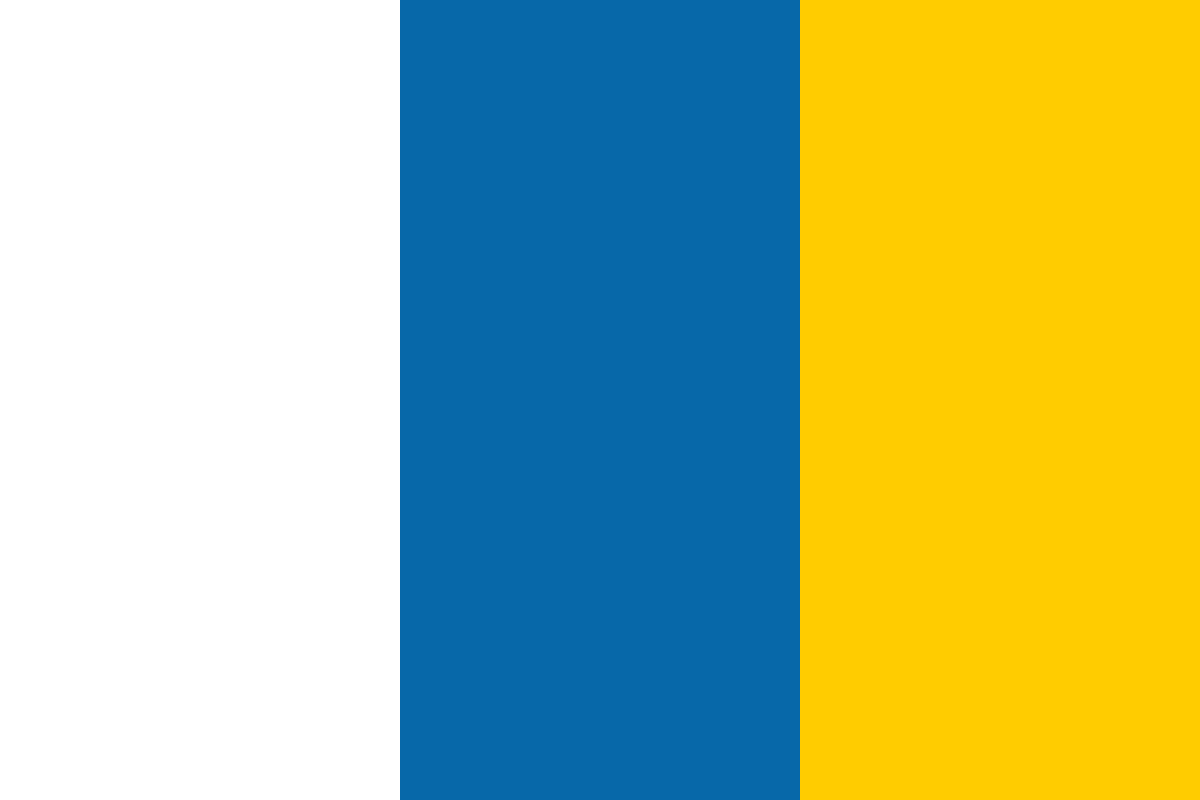

































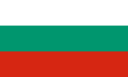








These methods are used to remove content from the DOM and replace it with new content.
Get the HTML contents of the first element in the set of matched elements.
.html()
None
A string containing the HTML representation of the element.
This method is not available on XML documents.
In an HTML document, we can use .html() to get the contents of any element. If our selector expression matches more than one element, only the first one's HTML content is returned. Consider the following code:
$('div.demo-container').html();
In order for the content of the following <div> to be retrieved, it would have to be the first one in the document.
<div class="demo-container">
<div class="demo-box">Demonstration Box</div>
</div>
The result would look like this:
<div class="demo-box">Demonstration Box</div>
Set the HTML contents of each element in the set of matched elements.
.html(htmlString)
.html(function)
The jQuery object, for chaining purposes.
The .html() method is not available in XML documents.
When we use .html() to set the content of elements, any content that was in those elements is completely replaced by the new content. Consider the following HTML code:
<div class="demo-container">
<div class="demo-box">Demonstration Box</div>
</div>
We can set the HTML contents of <div class="demo-conta iner"> as follows:
$('div.demo-container')
.html('<p>All new content. <em>You bet!</em></p>');
That line of code will replace everything inside <div class="demo-container">.
<div class="demo-container">
<p>All new content. <em>You bet!</em></p>
</div>
As of jQuery 1.4, the .html() method allows us to set the HTML content by passing in a function.
$('div.demo-container').html(function() {
var emph = '<em>' + $('p').length + ' paragraphs!</em>';
return '<p>All new content for ' + emph + '</p>';
});
Given a document with six paragraphs, this example will set the HTML of <div class="demo-container"> to <p>All new content for <em>6 paragraphs!</em></p>.
Get the combined text contents of each element in the set of matched elements, including their descendants.
.text()
None
A string containing the combined text contents of the matched elements.
Unlike the .html() method, .text() can be used in both XML and HTML documents. The result of the .text() method is a string containing the combined text of all matched elements. Consider the following HTML code:
<div class="demo-container">
<div class="demo-box">Demonstration Box</div>
<ul>
<li>list item 1</li>
<li>list <strong>item</strong> 2</li>
</ul>
</div>
The code $('div.demo-container').text() would produce the following result:
Demonstration Box list item 1 list item 2
Set the content of each element in the set of matched elements to the specified text.
.text(textString)
.text(function)
The jQuery object, for chaining purposes.
Unlike the .html() method, .text() can be used in both XML and HTML documents.
We need to be aware that this method escapes the string provided as necessary so that it will render correctly in HTML. To do so, it calls the DOM method .createTextNode(), which replaces special characters with their HTML entity equivalents (such as < for <). Consider the following HTML code:
<div class="demo-container">
<div class="demo-box">Demonstration Box</div>
<ul>
<li>list item 1</li>
<li>list <strong>item</strong> 2</li>
</ul>
</div>
The code $('div.demo-container').text('<p>This is a test.</p>'); will produce the following DOM output:
<div class="demo-container">
<p>This is a test.</p>
</div>
It will appear on a rendered page as though the tags were exposed as follows:
<p>This is a test</p>
As of jQuery 1.4, the .text() method allows us to set the text content by passing in a function.
$('ul li').text(function() {
return 'item number ' + ($(this).index() + 1);
});
Given an unordered list with three <li> elements, this example will produce the following DOM output:
<ul>
<li>item number 1</li>
<li>item number 2</li>
<li>item number 3</li>
</ul>